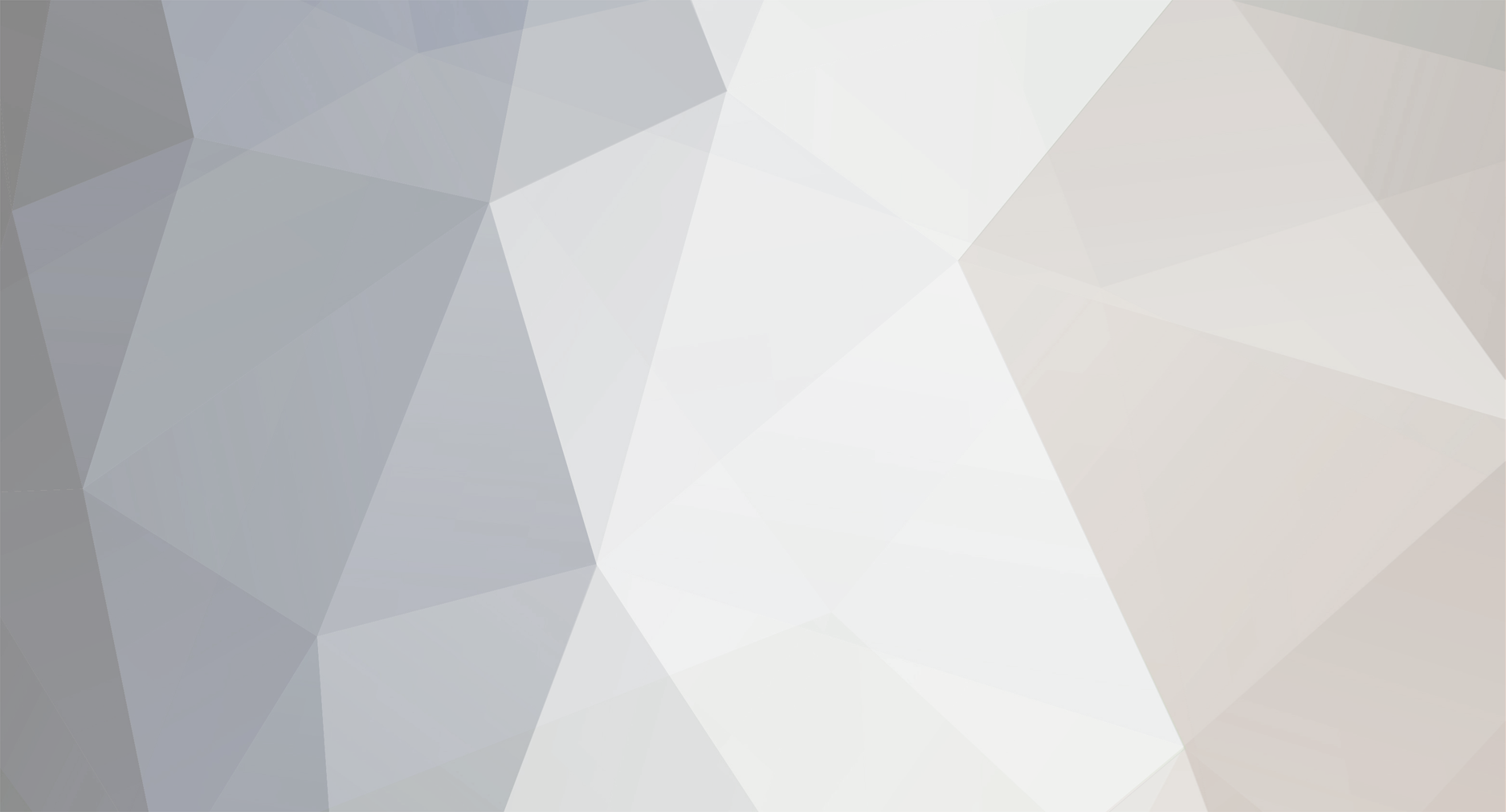
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
Not if kaveman50 followed my suggestion.
-
Things like: <br/><input type="text" name="num1" value = "<?=$_POST['num']?> " /> Notice you have a space after <?=$_POST['num']?> before "
-
Database Design and segregation of reponsiblilites
Alex replied to flash gordon's topic in Application Design
I would definitely go with the three separate tables. You don't want to be storing unrelated data together in the same table. Trying to manage all of this inside 1 table would be a very bad design flaw that would just make things much harder for you in the long run. I suggest reading up on database normalization -
You didn't listen to what I said before. You can leave the values that you have set if you want (although, if you have error reporting high enough those will throw and undefined index warning, so ideally you should use isset() for those as well), but you might want to remove some of the spaces you have in there.
-
Make sure the post was submitted: if(isset($_POST['Submit'])) { echo getAverage($_POST['num']); } isset()
-
Values for parameters given in the function declaration designate default values if that specific parameter happens to not be passed, if it is passed it'll just be overlooked. Example: function doSomething($var = 50) { echo $var; } /* Because I don't pass the first parameter in this example it assumes the default value designated in the function declaration. ($var = 50). As a result this function will echo '50'. */ doSomething(); /* This time I do supply the first parameter in the function call so the default value of 50 is overlooked, and it just assumes the value that I supply in the function call. As a result this time the function echos 'Hello'. */ doSomething('Hello');
-
Something like this would also work: <?php function getAverage($arr) { asort($arr); array_shift($arr); array_shift($arr); return array_sum($arr) / count($arr); } To use it in your form you'd make all the text inputs named 'num[]', then do something like: echo getAverage($_POST['num']);
-
[SOLVED] Class could not be converted to string
Alex replied to XpertWorlock's topic in PHP Coding Help
If you do want to use an instance of a class like a string it's useful to utilize the __toString() magic method. Ex: class someClass { public function __toString() { return 'Some name'; } } $in = new someClass; echo $in; // Some name -
Strange issue with custom function and image drawing
Alex replied to patrick89's topic in PHP Coding Help
When working with images if you can't figure out the problem it's usually a good idea to comment out the Content-type header so that you can see the error you're getting. In your case you have a scope problem. Neither $timelineGraph nor $red is set within the scope of that function. You can either make them globals (which is a bad practice), or preferably pass those variable as parameters. Ex: <?php header("Content-type: image/png"); $timelineGraph = ImageCreate(300,300) or die("Cannot Initialize new GD image stream"); // Formatting $black = ImageColorAllocate($timelineGraph, 0, 0, 0); $red = ImageColorAllocate($timelineGraph, 255, 0, 0); function drawTimeline($im, $color, $startWeek, $startDay, $endWeek, $endDay) { ImageLine($im, 0, 0, 299, 299, $color); } drawTimeline($timelineGraph, $red, 1,1,1,1); ImagePng($timelineGraph); ImageDestroy($timelineGraph); ?> -
You can't do this with PHP alone, but with some JavaScript you can. Here's an example: <script type="text/javascript"> window.onload = setTimeout('document.getElementById("something").innerHTML = ""', 1000); </script> <?php echo '<span id="something">Some text here..</span>'; ?>
-
Yes, you can declare properties like that. It doesn't have anything to do with the referencing. class someClass { public function __construct($str) { $this->str = $str; } public function echoStr() { echo $this->str; } } $something = 'hello'; $instance = new someClass($something); $instance->echoStr(); Would work as well. Or am I misunderstanding the question?
-
You didn't listen to what he said. When grabbing only 1 random key it doesn't return an array. So $keys[0] and $keys[1] are non-existent. If you were only doing one you'd have to do: echo $rand[$keys];
-
Is it just a special font? Why not just use imagettftext() or imageloadfont(). Technically you can use your custom image, pretty easily, but it'll be pretty slow.
-
Can you tell me the benefit of using multiple browsers (besides having their own processes and the fact that you're just used to it)? Or just use the best web browser, whos tabs are separated into their own processes.
-
Never use $_SERVER['PHP_SELF'] as a form action, it leaves you vulnerable to XSS attacks. If you want to set the action to the same page just leave action blank (action="").
-
Nope. You'll have to use strtotime().
-
You're not defining $n_time within your list: list($n_id, $n_vid, $n_pic, $n_title) = explode('-', $n_line); Add it if you know how, or show us the structure of $n_line.
-
Yea, I noticed that myself. I was considering making all the tabs the same length to avoid the snapping when adding or removing the scrollbar, but I thought that might not look so great because there would be empty space on tabs will less content. Another idea was to adjust the entire layout's position based on if the scrollbar is present or not with some js to avoid the snapping, but that too seems a bit unnecessary. I'll shorten the smiley display area to show just 2 rows at a time and that should fix this problem on most resolutions, and decide on how to completely address the issue a bit later.
-
Changing é into html entities without touching the html elements ?
Alex replied to Peuplarchie's topic in Third Party Scripts
html_entity_decode() -
within the or die() put mysql_error() and post what it says. It's usually not suggested to use back ticks unless you're using reserved words, in which case they're required. Also, if 'usrID' is an auto_increment field there is no need to include it in the query, it'll be inserted automatically.
-
[SOLVED] How do I select only the lowest price for each unique ID?
Alex replied to daydreamer's topic in MySQL Help
I think you mean: SELECT ID, MIN(Price) FROM tblPrices GROUP BY Product ID -
Your code would only work if register_globals is on, which is never a good idea. Instead you should be using the $_GET superglobal. So it would be $_GET['fruit']. Additionally you should be using isset() to confirm that the fruit index is defined within the $_GET superglobal otherwise (depending on your error reporting level) a warning will be thrown. Something like this would work: if(isset($_GET['fruit'])) { echo '<p>You clicked ' . $_GET['fruit'] . '</p>'; }
-
[SOLVED] Simple validation function makes PHP to stop respond
Alex replied to nvee's topic in PHP Coding Help
Technically the for statement is just looping through incrementing the variable $i from 0 until it gets to number of the highest element in the array. Here's an example that explains it better: $array = Array('something', 'something else', 'something else'); for($i = 0;$i < count($array);$i++) { echo "$i<br />"; } If you run that you'll notice you get 0, 1, 2 which are the indexes of the array. So if you do: $array = Array('something', 'something else', 'something else'); for($i = 0;$i < count($array);$i++) { echo $array[$i] . '<br />'; } You're getting all the elements of the array, it's the same as: echo $array[0] . '<br />'; echo $array[1] . '<br />'; echo $array[2] . '<br />'; -
[SOLVED] Simple validation function makes PHP to stop respond
Alex replied to nvee's topic in PHP Coding Help
Actually no, mikesta707, there's a reason it will not work. empty($value) would never even get a chance to run, because if $value was empty, the while statement wouldn't evaluate to true in the first place. Making this an ineffective way to loop through an array that may contain empty values. -
[SOLVED] Simple validation function makes PHP to stop respond
Alex replied to nvee's topic in PHP Coding Help
Post your entire code.