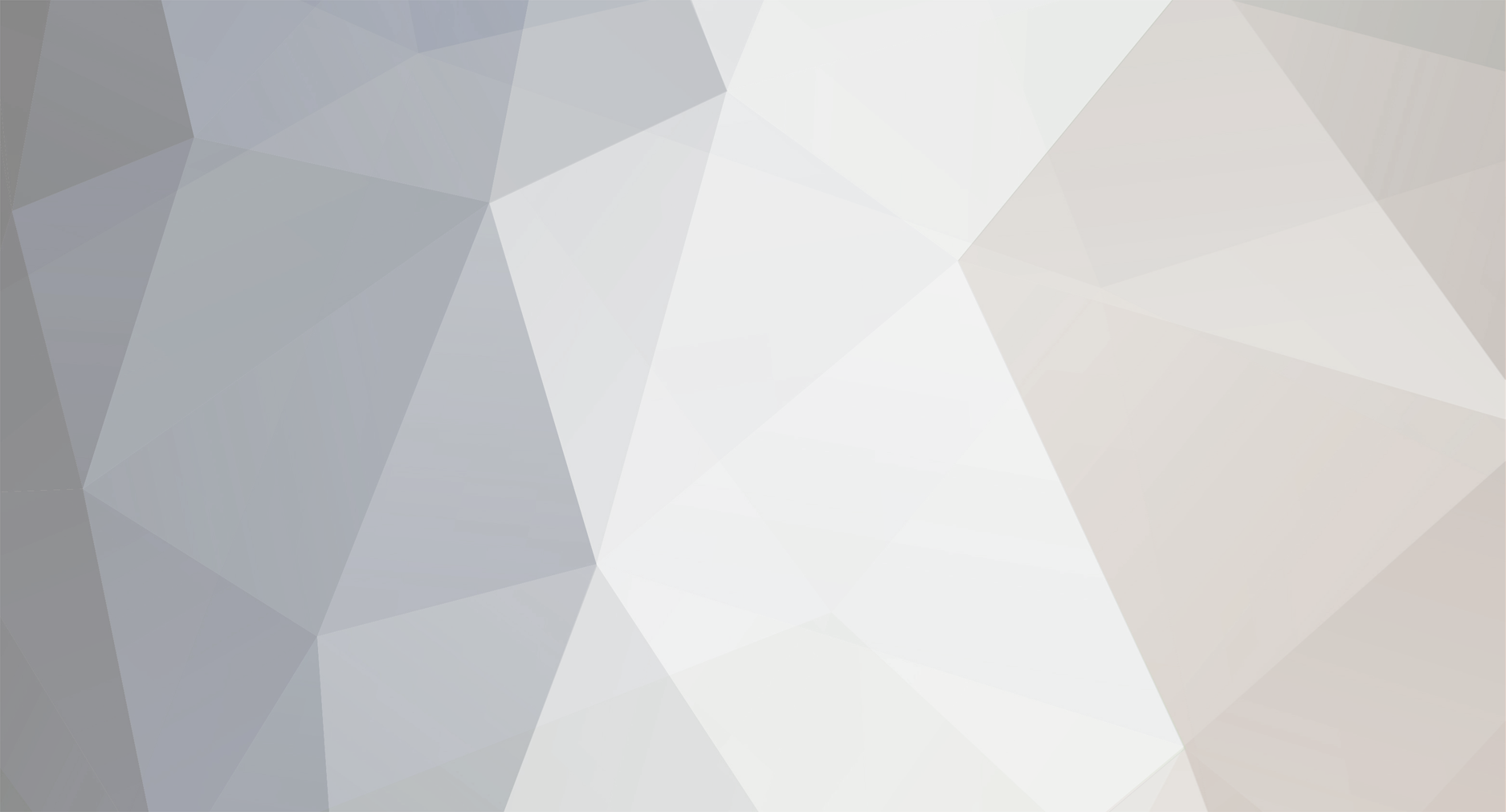
Alex
Staff Alumni-
Posts
2,467 -
Joined
-
Last visited
Everything posted by Alex
-
Whoops, unintentional post. :-\
-
Just check "Topic Solved" in the bottom left, Thank you.
-
The site won't load for me, but it doesn't matter. The reason that A is being put inside of it's own row is because the two conditionals ($i % 100) == 0 are only being fired when $i = 0. If you want to make them all be inside one <tr>, you should remove that from the loop. I don't see the point of if(strlen($head_letters[$i]) > 0) because that would only evaluate to true if one of the elements in $head_letters is empty, which since that array is hard-coded shouldn't be a problem. Try: echo '<tr>'; for($i = 0;$i < count($head_letters);$i++) echo "<td align='center' style='cursor:pointer;'><a href=\"browse-{$head_letters[$i]}_page-0.html\" class='menualpha'><b>{$head_letters[$i]}</b></a></td>"; echo '</tr>';
-
If you're only picking 1 record from the database there's no need to create a loop. Additionally you should take advantage of ordering and limiting. $query = "SELECT pin, id FROM `pins` ORDER BY id DESC LIMIT 1"; $result = mysql_query($query) or die(mysql_error()); $row = mysql_fetch_assoc($result); echo "The highest id is " . $row['id'] . " and has pin number: " . $row['pin'];
-
You can use this website to see how your website looks in various versions of IE.
-
It doesn't matter either way because ob_start() doesn't output anything (So you won't get a headers already sent error if you call ob_start() first). So.. session_start(); ob_start(); or ob_start(); session_start(); There will be no difference.
-
ob_start() isn't related to session_start(), ob_start() is just to turn output buffering on so that you can catch all the output of the page and set it to a session variable later on through ob_get_contents(). Check them out, ob_start(), ob_get_contents()
-
I'm confused as to why you're using preg_match().. Why don't you just do: if($realtoken == $token) { //Valid } else { //Invalid }
-
Oh, I see. Well you can do something like this: <?php session_start(); ob_start(); echo <<<END All your page's output here END; $_SESSION['var'] = ob_get_contents(); ?> $_SESSION['var'] will then include everything that was output on the page.
-
Through PHP GD you can load fonts and use them in images, but not on a website's text.
-
The reason you're getting that is because you're trying to use a MySQL resource in preg_match, which makes no sense. You should have: $result = mysql_query("SELECT token FROM data WHERE email='$email'") or die(mysql_error()); $row = mysql_fetch_assoc($result); $realtoken = $row['token'];
-
I'm not exactly sure what you mean.. Do you mean something like this? <?php session_start(); if(isset($_POST['var'])) $_SESSION['var'] = $_POST['var']; if(isset($_SESSION['var'])) echo $_SESSION['var'];
-
help auto deleting row from table after x ammount of time
Alex replied to Jnerocorp's topic in MySQL Help
Assuming your unix time stamp field is called time_created you can do this: mysql_query("DELETE FROM `table` WHERE time_created + 600 <= UNIX_TIMESTAMP()"); -
[SOLVED] PHP Not Writing To MySQL Database
Alex replied to CrownVictoriaCop's topic in PHP Coding Help
You're getting that error because the number of rows to insert data into don't match number of values you provided. Edit: More specifically you don't have a value listed for BirthDay. -
[SOLVED] PHP Not Writing To MySQL Database
Alex replied to CrownVictoriaCop's topic in PHP Coding Help
If you have a database created you can try this SQL query on it to create the table. CREATE TABLE IF NOT EXISTS `Students` ( ID INT NOT NULL AUTO_INCREMENT, ParentFirstName VARCHAR(32) NOT NULL, ParentLastName VARCHAR(32) NOT NULL, StudentName VARCHAR(32) NOT NULL, Class VARCHAR(32) NOT NULL, Address VARCHAR(64) NOT NULL, City VARCHAR(32) NOT NULL, State VARCHAR(32) NOT NULL, Zip INT NOT NULL, Phone VARCHAR(32) NOT NULL, BirthMonth VARCHAR(32) NOT NULL, BirthDay VARCHAR(16) NOT NULL, BirthYear VARCHAR(16) NOT NULL, School VARCHAR(32) NOT NULL, PaymentMethod VARCHAR(32) NOT NULL, PRIMARY KEY(`ID`) ); It's not optimized, it could (and should) be done differently but I'm not sure how of your form is setup and the format of the data. Note: This will throw an error if you leave any of the fields blank; so you should also put in some form validation (currently the SQL query is assuming that you want every field required). -
[SOLVED] PHP Not Writing To MySQL Database
Alex replied to CrownVictoriaCop's topic in PHP Coding Help
Where'd you get the rest of this code from? If it's from a third-party source they should've supplied you with the SQL query to create the table. It would probably look something like.. CREATE TABLE IF NOT EXISTS `Students` ( ParentFirstName VARCHAR(32) NOT NULL, ... ); -
[SOLVED] PHP Not Writing To MySQL Database
Alex replied to CrownVictoriaCop's topic in PHP Coding Help
You'll need to run a query to create the table in the database. -
What do you mean by 'not working'? You should try to be more specific; it'll help us help you more effectively.
-
[SOLVED] PHP Not Writing To MySQL Database
Alex replied to CrownVictoriaCop's topic in PHP Coding Help
Is ID an auto_increment field? If so it's not even necessary to include it in the query. Try: $result = mysql_query("INSERT INTO Students (ParentFirstName, ParentLastName, StudentName, Class, Address, City, State, Zip, Phone, BirthMonth, BirthDay, BirthYear, School, PaymentMethod) VALUES ('".$PFName."', '".$PLName."', '".$SFName."', '".$Class."', '".$Address."', '".$City."', '".$State."', '".$Zip."', '".$Phone."', '".$BMonth."', '".$BYear."', '".$School."', '".$PaymentMethod."')"); echo ($result) ? 'Success!' : mysql_error(); -
[SOLVED] PHP Not Writing To MySQL Database
Alex replied to CrownVictoriaCop's topic in PHP Coding Help
Oh, You're missing a ( right after VALUES. Try this: mysql_query("INSERT INTO Students (ID, ParentFirstName, ParentLastName, StudentName, Class, Address, City, State, Zip, Phone, BirthMonth, BirthDay, BirthYear, School, PaymentMethod) VALUES ('NULL', '".$PFName."', '".$PLName."', '".$SFName."', '".$Class."', '".$Address."', '".$City."', '".$State."', '".$Zip."', '".$Phone."', '".$BMonth."', '".$BYear."', '".$School."', '".$PaymentMethod."')"); -
[SOLVED] PHP Not Writing To MySQL Database
Alex replied to CrownVictoriaCop's topic in PHP Coding Help
I don't see any problem with that code (besides that fact that you should be securing all data being inserted into the database with mysql_real_escape_string()). Try changing the query to this and tell us if it outputs any error: mysql_query("INSERT INTO Students (ID, ParentFirstName, ParentLastName, StudentName, Class, Address, City, State, Zip, Phone, BirthMonth, BirthDay, BirthYear, School, PaymentMethod) VALUES 'NULL', '".$PFName."', '".$PLName."', '".$SFName."', '".$Class."', '".$Address."', '".$City."', '".$State."', '".$Zip."', '".$Phone."', '".$BMonth."', '".$BYear."', '".$School."', '".$PaymentMethod."')") or die(mysql_error()); -
help auto deleting row from table after x ammount of time
Alex replied to Jnerocorp's topic in MySQL Help
You could create a row to store a UNIX time stamp on each record then run a CRON job every X minutes to check and see if that time stamp is older than 10 minutes. -
You can use file_get_contents() $content = file_get_contents('http://phpfreaks.com'); echo $content;
-
You were missing )"; at the end. <?php $connect=mysql_connect("localhost","dbuser","dbpassword"); $sql="INSERT INTO`dbase`.`auto`( `name`, `last`, `mi`, `phone`, `cp`, `wp`, `email`' `add`, `add2`, `city`, `state`, `zip`, `password` ) VALUES ( '{$_POST['name']}', '{$_POST['last']}', '{$_POST['mi']}', '{$_POST['phone']}', '{$_POST['cp']}', '{$_POST['wp']}', '{$_POST['email']}', '{$_POST['add']}', '{$_POST['add2']}', '{$_POST['city']}', '{$_POST['state']}', '{$_POST['zip']}', '{$_POST['password']}')"; $res=mysql_query($sql)or die(mysql_error()); ?>