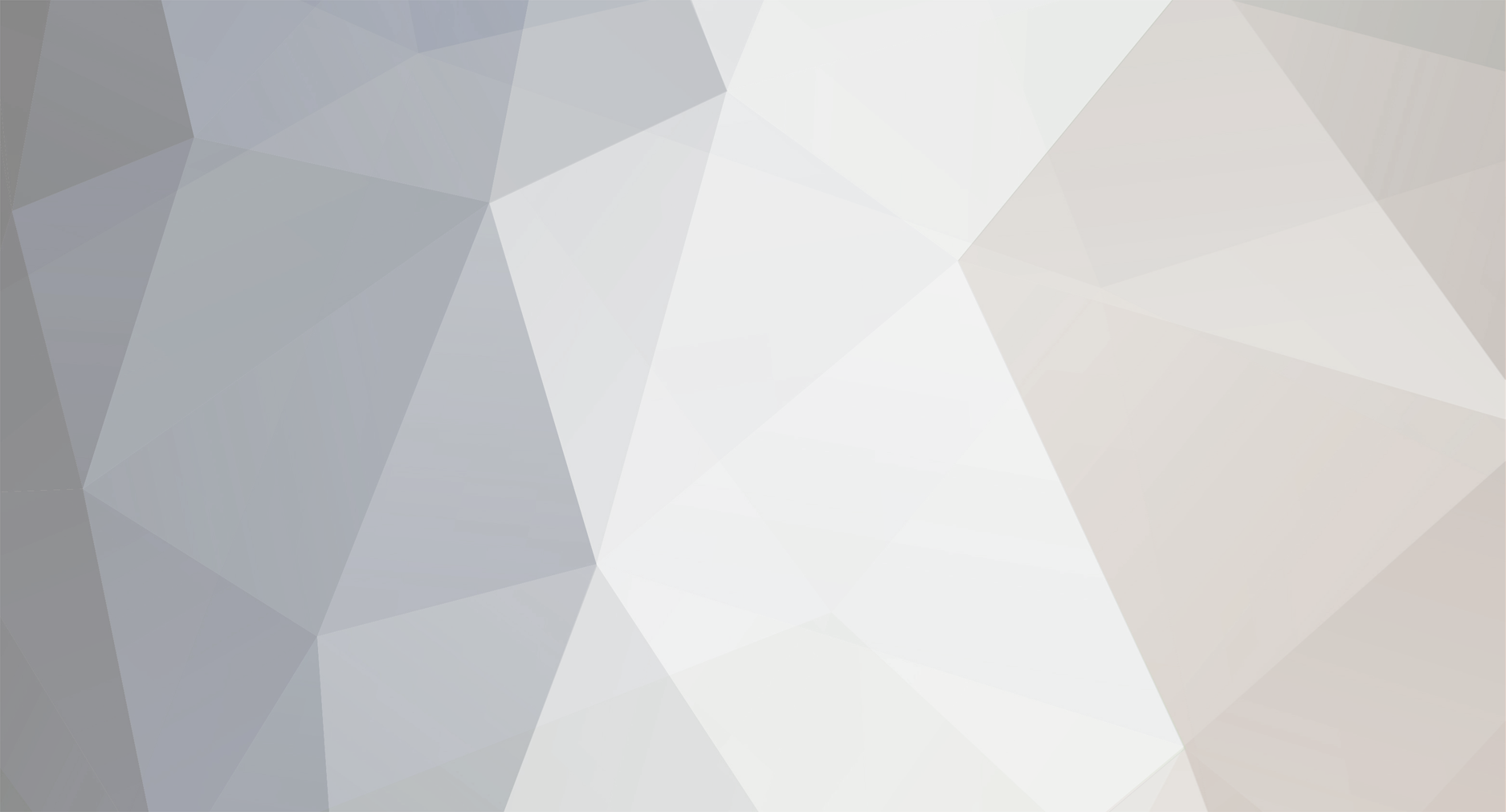
DeX
Members-
Posts
258 -
Joined
-
Last visited
Everything posted by DeX
-
Why use things like Java to create web applications?
DeX replied to DeX's topic in Other Programming Languages
Okay I got a few more questions about this now. // structure/calc-mvc/CalcMVC.java -- Calculator in MVC pattern. // Fred Swartz -- December 2004 import javax.swing.*; public class CalcMVC { //... Create model, view, and controller. They are // created once here and passed to the parts that // need them so there is only one copy of each. public static void main(String[] args) { CalcModel model = new CalcModel(); CalcView view = new CalcView(model); CalcController controller = new CalcController(model, view); view.setVisible(true); } } So this completely makes sense, just initialize the constructors for each class and then load the view. Will the main class pretty much look like this for every MVC application? // structure/calc-mvc/CalcView.java - View component // Presentation only. No user actions. // Fred Swartz -- December 2004 import java.awt.*; import javax.swing.*; import java.awt.event.*; class CalcView extends JFrame { //... Constants private static final String INITIAL_VALUE = "1"; //... Components private JTextField m_userInputTf = new JTextField(5); private JTextField m_totalTf = new JTextField(20); private JButton m_multiplyBtn = new JButton("Multiply"); private JButton m_clearBtn = new JButton("Clear"); private CalcModel m_model; //======================================================= constructor /** Constructor */ CalcView(CalcModel model) { //... Set up the logic m_model = model; m_model.setValue(INITIAL_VALUE); //... Initialize components m_totalTf.setText(m_model.getValue()); m_totalTf.setEditable(false); //... Layout the components. JPanel content = new JPanel(); content.setLayout(new FlowLayout()); content.add(new JLabel("Input")); content.add(m_userInputTf); content.add(m_multiplyBtn); content.add(new JLabel("Total")); content.add(m_totalTf); content.add(m_clearBtn); //... finalize layout this.setContentPane(content); this.pack(); this.setTitle("Simple Calc - MVC"); // The window closing event should probably be passed to the // Controller in a real program, but this is a short example. this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); } void reset() { m_totalTf.setText(INITIAL_VALUE); } String getUserInput() { return m_userInputTf.getText(); } void setTotal(String newTotal) { m_totalTf.setText(newTotal); } void showError(String errMessage) { JOptionPane.showMessageDialog(this, errMessage); } void addMultiplyListener(ActionListener mal) { m_multiplyBtn.addActionListener(mal); } void addClearListener(ActionListener cal) { m_clearBtn.addActionListener(cal); } } So this simply loads the view that is seen by the end user and doesn't contain any of the logic used by the application. But I'm wondering why the view is calling the setText methods, shouldn't these be done by the controller? What's the controller's job if not to facilitate the communication between the view and the model? // structure/calc-mvc/CalcModel.java // Fred Swartz - December 2004 // Model // This model is completely independent of the user interface. // It could as easily be used by a command line or web interface. import java.math.BigInteger; public class CalcModel { //... Constants private static final String INITIAL_VALUE = "0"; //... Member variable defining state of calculator. private BigInteger m_total; // The total current value state. //============================================================== constructor /** Constructor */ CalcModel() { reset(); } //==================================================================== reset /** Reset to initial value. */ public void reset() { m_total = new BigInteger(INITIAL_VALUE); } //=============================================================== multiplyBy /** Multiply current total by a number. *@param operand Number (as string) to multiply total by. */ public void multiplyBy(String operand) { m_total = m_total.multiply(new BigInteger(operand)); } //================================================================= setValue /** Set the total value. *@param value New value that should be used for the calculator total. */ public void setValue(String value) { m_total = new BigInteger(value); } //================================================================= getValue /** Return current calculator total. */ public String getValue() { return m_total.toString(); } } This also makes sense, it's the basic functions of get, set and multiply used by the application. // stucture/calc-mvc/CalcController.java - Controller // Handles user interaction with listeners. // Calls View and Model as needed. // Fred Swartz -- December 2004 import java.awt.event.*; public class CalcController { //... The Controller needs to interact with both the Model and View. private CalcModel m_model; private CalcView m_view; //========================================================== constructor /** Constructor */ CalcController(CalcModel model, CalcView view) { m_model = model; m_view = view; //... Add listeners to the view. view.addMultiplyListener(new MultiplyListener()); view.addClearListener(new ClearListener()); } ////////////////////////////////////////// inner class MultiplyListener /** When a mulitplication is requested. * 1. Get the user input number from the View. * 2. Call the model to mulitply by this number. * 3. Get the result from the Model. * 4. Tell the View to display the result. * If there was an error, tell the View to display it. */ class MultiplyListener implements ActionListener { public void actionPerformed(ActionEvent e) { String userInput = ""; try { userInput = m_view.getUserInput(); m_model.multiplyBy(userInput); m_view.setTotal(m_model.getValue()); } catch (NumberFormatException nfex) { m_view.showError("Bad input: '" + userInput + "'"); } } }//end inner class MultiplyListener //////////////////////////////////////////// inner class ClearListener /** 1. Reset model. * 2. Reset View. */ class ClearListener implements ActionListener { public void actionPerformed(ActionEvent e) { m_model.reset(); m_view.reset(); } }// end inner class ClearListener } This is the controller that listens to the buttons and performs actions when the user interacts with the application. Is this the sole purpose of the controller or is it supposed to do the calls for get/set as well? I pretty much got it now, just have these last few questions, thanks. -
I know most programming languages and run a web development business but haven't dealt much with struts yet. I've spent this evening reading up on them and I have a few questions as to why someone might use them. 1. I see they are sort of like an API extension to the Java EE platform allowing you to create more scalable applications. Since Java's main selling point is that it's cross platform, why would it be useful in a web environment where the platform is irrelevant? Why not use PHP instead? Or .NET? 2. It seems to be most helpful when developing a MVC type architecture which makes sense but isn't it easy enough to create this tiered architecture with the API we already have available? Why do we need these struts? 3. I see these were used to create Drupal, Joomla! and Ruby, why couldn't those applications simply be created with PHP?
-
I honestly don't understand this at all. I put this on the page: <div style="position:relative;width:500px;"> <div style="float:left;display:inline;"> <select style="display:inline;"></select> </div> <div style="float:left;display:inline;"> <select style="display:inline;"></select> </div> </div> which is a select inside a div, next to another select inside its own div, all inside a div. Then I constructed a Javascript function to spit out the same thing and when I use the Inspect Element function in Chrome it shows me the exact same code: <div style="position:relative;width:500px;"> <div style="float:left;display:inline;"> <select style="display:inline;"></select> </div> <div style="float:left;display:inline;"> <select style="display:inline;"></select> </div> </div> The crazy problem is when I view the first one that is coded into the page, they appear side by side as they're supposed to. When I click the + button and run the Javascript to add the second code, the new select boxes appear above/below one another. Why is that? Why do they show the same code in Chrome and display differently in Internet Explorer?
-
Anyone?
-
Are you sure? I'm using this very basic Javascript function now and it's still loading them below one another in Internet Explorer 7. <script type="text/javascript"> function addRow(id) { if (!document.getElementsByTagName) return; formBody = document.getElementsByTagName("form").item(0); formElement = document.getElementById("formitem" + id); divRow = document.createElement("div"); select1 = document.createElement("select"); select2 = document.createElement("select"); ddd1 = document.createElement("div"); ddd2 = document.createElement("div"); ddd3 = document.createElement("div"); ddd1.setAttribute("style", "display:inline;"); ddd2.setAttribute("style", "display:inline;"); ddd3.setAttribute("style", "display:inline;"); ddd1.appendChild(select1); ddd2.appendChild(select2); ddd3.appendChild(ddd1); ddd3.appendChild(ddd2); formBody.insertBefore(ddd3, formElement.nextSibling); } </script>
-
I'm just using "float:left;" in my CSS so it should be fine. But in the piece of code I posted, I'm not using any CSS and it's still not working.
-
Here's my PHP form code: <form method='POST' action="<?php basename($_SERVER['PHP_SELF']);?>" onSubmit="return stripInputBoxes(1)"> <?php echo "<div id = 'purchaseOrderRow1' style = 'display:none;border: 1px solid black;'>"; echo $integrityBuildingProductsPDF; echo '<input type = "hidden" id = "pdf1" value = "" name = "pdf1" />'; echo '<button name = "savePurchaseOrder" type = "submit">Save Purchase Order</button>'; echo "</div>"; ?> </form> Here's my Javascript: function stripInputBoxes(pdfNumber) { if (!document.getElementsByTagName) return; for (i = 0; i < document.getElementById('tableBody').getElementsByTagName('tr').length - 3; i++) { document.getElementById(i).parentNode.innerHTML = document.getElementById(i).value; } document.getElementById("pdf" + pdfNumber).value = "ljkasdkljf"; return true; } Will this set the value of the hidden variable? I'm trying to echo the value of $_POST['pdf1'] but it comes out to nothing when the page reloads. How do I do this? What I'm trying to do here is display a purchase order on the screen and the user can edit the quantities in the purchase order. They click SAVE to save the new updated quantities and it auto generates a PDF that's saved on the server with those new quantities. The problem is I need to run the stripInputBoxes function to take the <input> tags out of the HTML code before creating the PDF because the PDF generator doesn't recognize the tags.
-
I've got a pretty large form with inputs but cut it down here to post. I have an input form that has coveralls and the user selects a size, selects a quantity, then enters the name to be sewn onto them. Then if they want more, they hit the + button and the javascript adds another line for them to do all the options again. In Chrome, Firefox and Internet Explorer 8 it loads all the elements onto the same row, side by side. In Internet Explorer 7 for some reason it loads them all above one another and I cannot figure out why. There's no style sheet in this snippet, here's what I have for you to check out: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Enerplus</title> <script type="text/javascript"> var count = 41; function addRow(id, names) { if (!document.getElementsByTagName) return; formBody = document.getElementsByTagName("form").item(0); formElement = document.getElementById("formitem" + id); quantityText = document.createElement("input"); divDiv = document.createElement("div"); divRow = document.createElement("div"); divFirstNameColumn = document.createElement("div"); divLastNameColumn = document.createElement("div"); divQuantityColumn = document.createElement("div"); firstNameInput = document.createElement("input"); lastNameInput = document.createElement("input"); divDiv.setAttribute("id", "formitem1"); divRow.setAttribute("class", "row"); divRow.setAttribute("style", "clear:both;"); divQuantityColumn.setAttribute("class", "column"); divQuantityColumn.setAttribute("style", "float:left;"); divFirstNameColumn.setAttribute("class", "column"); divFirstNameColumn.setAttribute("style", "float:left;"); divLastNameColumn.setAttribute("class", "column"); divLastNameColumn.setAttribute("style", "float:left;"); firstNameInput.setAttribute("type", "text"); lastNameInput.setAttribute("type", "text"); quantityText.setAttribute("type", "text"); quantityText.setAttribute("name", "form1[" + count + 1 + "][quantity]"); div1 = formElement.getElementsByTagName("div").item(0); div2 = div1.getElementsByTagName("div"); for (var i = 0; i < div2.length; i++) { dc = document.createElement("div"); dc.setAttribute("class", "column"); dc.setAttribute("style", "float:left;"); selectArray = div2[i].getElementsByTagName("select"); // check first if there is a select tag there if (selectArray.length > 0) { select = div2[i].getElementsByTagName("select").item(0); selectElement = document.createElement("select"); selectElement.setAttribute("name", "form1[" + count + "][" + select.id + "]"); selectElement.setAttribute("id", select.id); selectElement.setAttribute("style", "float:left;"); for (var j = 0; j < select.options.length; j++) { text = document.createTextNode(select.options[j].text); optionElement = document.createElement("option"); optionElement.setAttribute("value", select.options[j].value); optionElement.appendChild(text); selectElement.appendChild(optionElement); } dc.appendChild(selectElement); divRow.appendChild(dc); count = count + 1; } } divDiv.appendChild(divRow); formBody.insertBefore(divDiv, formElement.nextSibling); } </script> </head> <body> <form id="login" method="POST" action="ty.php"> <div id = "formitem1"> <div class="row" style="clear:both;"> <div class="column"><span>length</span><br /> <select name="form1[50][Coverall Length]" id="Coverall Length" value=" "> <option value = ""></option> <option value="S">S</option> <option value="R">R</option> <option value="T">T</option> </select> </div> <div class="column"><span>length2</span><br /> <select name="form1[51][Coverall Length2]" id="Coverall Length2" value=" "> <option value = ""></option> <option value="S">S</option> <option value="R">R</option> <option value="T">T</option> </select> </div> <div class="column"><span>quantity</span><br /> <input type="text" name="form1[29][Coverall Quantity]" id="Coverall Quantity" style="width:40px;" /> </div> <div class="column"><span>employee first name</span><br /> <input name="form1[30][Employee First Name]" type="text" id="Employee First Name" value="" /> </div> <div class="column"><span>employee last name</span><br /> <input name="form1[31][Employee Last Name]" type="text" id="Employee Last Name" value="" /> </div> <div class="column"><br /> <button onClick="addRow(1, true);return false;">+</button></div> </div> </div> </form> </body> </html>
-
I have a purchase order displaying on a site that the user can edit quantities for. I want them to be able to hit submit and it takes that form and submits it to some code that auto creates a PDF with it. The PDF part is easy, I use DOMPDF to create that and it works fine, I just can't seem to get the values passed through. I can easily pass the values of just the input boxes through the $_POST but I don't want to have to recreate the PDF setup after the post. I'd rather just use some Javascript or something to grab the innerHTML of a <div> element and somehow make it available after the form is submitted so the PHP code can use it to send to DOMPDF. The form submits to itself and I have a piece of code at the top of the page which will create the PDF, I just need the <div> contents and I'm done. How do I get it? I could set it into a hidden variable but the reason I'm not doing that is because for some reason when I assign the big table which contains the whole purchase order into the hidden variable and then try to print it, it comes out all messed up. I don't know why and I'd rather just find another way than attempt to figure that out.
-
Interesting, maybe I can simplify all the different material management pages into one single page and have a submit button for each section of materials. Or would you have one single submit button at the bottom to save all the materials on the page and their prices/sku/weight accordingly? By the way, what would be the benefit to having a single table instead of all separated like I have it now? And also, would it matter that some materials have a material price as well as a labour price and others just have a material price? Then others have lengths, some don't, and others have attributes like a window can be sliding or fixed. I'd have a lot of NULL cells for materials that don't have those attributes. I think I'm the only one right now that can add new materials because of the way the building price is calculated. When the quote is done, there are algorithms run on each material to figure out how many of each are needed, like for each walk-in door in the quote, it would add 3 pieces of 2x4 to build the frame of that door, get the price from the lumber table and multiply it by the quantity to get the total price of 2x4, then add that to the total price of the building. And right now I've got to add caulking so we figured it out that they use 2 tubes of caulking per door and also 1 tube for every 10 feet of length on the eve. So I need to write the code to get all those variables and do the math, then add it to the building price. Unless you have a way they can add a new material (like tubes of caulking) and enter the calculations for it themselves? That would be amazing but I haven't figured out a way yet. So like I said up top here, just one single page to edit the material attributes? And have them all listed in a long table or divs with their price, length, sku, weight....? Is that the terms for what I'm trying to do here? If that's what I need to do then thanks for getting me started.
-
This is awesome, thanks guys. The criticism is welcomed, I'm not going to attack anyone for telling me the way it is. I know my database is trash but I'm here to find out how I can make it better. Or avenues of where I can start my research to make it better. I should also have asked another question I've had for the last few weeks.....should I be using stored procedures or JQuery? I've heard those terms thrown around but don't mind learning it if it's useful. I should also mention that I have more than enough skills to finish this, I'm actually going to incorporate all of their billing systems and everything else into it as well, so I don't need to contract it out. I just want to rewrite anything now if it helps the future of the project. Interesting. So you'd put all the materials into one table and load them based on the type ID? I might do that but how would I edit the pricing of each product then? What I have now is a materials management page where the owners can go in and click 'lumber' or click 'exterior metal' and view all of the pricing for each piece of material with textboxes to edit the prices. The elements are set up like so: $all_rows = $db->fetch_all_array($sql); foreach($all_rows as $column) { echo "<tr>"; echo "<td>" . $column['name'] . "</td>"; echo "<td><input type = 'text' name = 'form1[" . $column['id'] . "' value ='"."' /></td> <input type = 'hidden' name = 'form1[" . $column['id'] . "][price]' id = '" . $column['id'] . "' value = '" . $column['price'] . "' /> ............ for the rest of the attributes echo "</tr>"; } I just hand typed that from looking at my laptop, hopefully it's exact. And the submit button on the form redirects to itself where in the PHP I have: if (isset($_POST['saveChanges'])) { $form = $_POST['form1']; foreach ($form as $rowid => $columnarray) { foreach ($columnarray as $column => $value) { // create sql query here to update all values in the lumber table with all values on the page // this works fine, just don't want to type it all out here } } }
-
I've been on a major project for a client for the last 5 months and I have a website almost completely finished where the owner can log into an admin section and run a quote on a building for a customer. The quote gives builds a PDF quote with a price, they print it off and the client signs. It also builds all the purchase orders with the entire material list required for the building and they can click a button to email those purchase orders out to their respective building supply stores. They can also adjust the material prices, markup, add new users and such. I don't have any formal PHP training, just learning as I go but I'm an extremely fast learner and know a pile of other languages. But let me tell you how I did this and you tell me if there is anything I should rewrite a proper way and avoid on any future projects: - When creating a new user they have to enter the user's username and password they wish to use. The password is hashed and stored in the database (md5 I think?). The login page checks their username/password combination with what's in the database and sets a $_SESSION['xxxxx'] variable on success. At the top of every page (should be an include, I know) is a small script which checks to see if the login session variable is set and if it is, it proceeds to load the page. If not, it redirects to the login page. - Database access, this is a big one, should I be writing some sort of data access layer for this? When the user inputs all the specifics of the building (width, height, length, type of post, insulation....) and clicks submit, it submits to another page that auto generates the PDF, saves it on the server and displays all of the input information in HTML format but identical to how the PDF looks. It's actually displaying the HTML that the PDF was built using (with the dompdf tool). The problem here is there is 1000 - 2000 lines of code prior to this where it has to go and pull the price of every single piece of material from the database, do calculations to find out how many of each material are required for that building and multiply it by the price per piece to get the final price. And do this for every single piece of material. Here is how I'm doing this: $sql = @mysql_query('select price from lumber where id = '1'"); while($sqlRow = mysql_fetch_object($sql)) { twoByFourPrice = $sqlRow->price; } $sql = @mysql_query('select price from lumber where id = '2'"); while($sqlRow = mysql_fetch_object($sql)) { twoBySixPrice = $sqlRow->price; } ................. And that's just to get the prices for the lumber. Then I have to calculate the total pieces of 2x4 and multiply the price per piece by the total pieces and store the total price of all 2x4. It's a long process but it's done for every quote they do, hundreds per week. So by the time the quote is generated, the page has a few hundred variables stored with values. How can I make this better code? What's the proper way to do it? - If they want to go back and view a quote they did last week, they go into the quotes section, scroll through them (or search) and click to open it. I'm doing the database access method above to now pull all the information that was stored for that quote in order to display it again, so I don't recalculate everything but I do still have to pull the quote price, number of windows, number of doors, width, height........and all the rest. Should I be pulling this stuff into an array or something instead of grabbing each value with its own SQL statement? - This is how I have the database set up. Lumber - id, name, price, sku, weight Exterior Metal - id, name, price, sku, weight Interior Metal - id, name, price, sku, weight ..............and so on with all the different types of building products And then I store everything done in each quote: quotes - id, customer_id, width, length, height, date, salesman_id........ And all the materials required for that quote: quote_lumber - id, name, price, quantity, length, quote_id quote_exterior_metal - id, name, price, quantity, length, quote_id So when I load the quote, I just search each table for any materials where quote_id = the id of the quote I want to see. Am I doing this as simply as I can? I just want to know what better methods are out there. I'm also wondering if I should be using something like Yii or CakePHP for something like this but I have only looked at those for 2 minutes each. I'm not exactly sure what it is they would do for me here. Thanks guys.
-
You'd be surprised how many high end corporate websites have their websites done in tables.
-
Actually I'm getting closer with the mailto() function, that takes an attachment parameter that allows you to specify a file to send with the email and will open it in the client's email program. The only problem with this is it looks for the file on my local machine, is there a way to specify a file on the server?
-
This is for a single client that will be sending quotes to their own clients so they want to use their own Thunderbird system to: 1. Keep a copy in their SENT items folder. 2. Write a personalized message in the email prior to sending it the attached PDF. So right now I'm using DOMPDF to auto generate the PDF version of the quote and send it automatically via PHP using the mail() function. They can't do the things above with this method so I just need to move everything over to create the email in their email client prior to them hitting SEND.
-
Right, so I guess I'd have to use Javascript to do that.
-
That's great, thanks guys. I also found out I can use some <nobr></nobr> tags though this isn't W3C compliant.
-
I have a form on my website that currently uses the mail() function to send the information it collects and all works fine. I want to switch it over to open the email in Thunderbird on the user's machine so they can view it prior to sending. This should be much less complicated but I can't find answers anywhere, all I want to do is just open the email in the user's email client with the proper information/attachments included. Anyone? Thanks!
-
I'm doing a pretty complex site for a client and it involves a lot of tables. I have some questions regarding the way they want to go with them and the way I should go according to standards. 1. Sometimes they'll have something in a column that flows over onto the next line at the wrong place. For example, they'll have something like: Oversize (width x length x height) and because of the table column spacing, it'll come out on the website as Oversize (widthx length x height) We need that to look more like Oversize(width x length x height) so what they want to do is put a <br> tag in there to force the line break where they want it, before the brackets. Is it correct to use a <br> tag or should we be using <p> tags as better programming? Is there another way to do it? Can we force the column to expand to the end of the line in the CSS instead? I need to set this globally on the site to not wrap text for certain strings. 2. Is there a way to set a minimum column width in the CSS? I have widths set to AUTO right now but I'd like the columns to be at least 95 pixels wide or expand as needed.
-
Thanks guys, I figured it out. I'm using Cascade as a content management system so it's modifying the code a little bit to conform to W3C standards, so it is putting the embedded list inside the parent <li> tag like you suggested. That's not causing it though, I looked through the global.css file that we have and it's adding a background bullet image to all <ol><li> tags so I just took it out. Problem solved!
-
I have an unordered list with an ordered list embedded like so: <ul> <li>point 1</li> <li> <ol> <li>embedded point 1</li> <li>embedded point 2</li> </ol> </li> <li>point 3</li> </ul> Because the <ol> is inside a <li> tag, it adds an extra bullet at the top of the ordered list. Is this normal HTML or would it be something that is haywire inside the CSS? I didn't write the CSS so I'm basically looking for a starting point to look for how to fix this. Thanks!
-
I have a form on a page and I'm trying to cycle through all the div elements in a row and add all those elements again on a new row. I've created a very simplified version stripped down and it still doesn't work. Here's my code: Inside the HTML body tag, nothing else on the page: <form> <formitem> <div></div> </formitem> </form> Javascript function: function addRow2(id, names) { if (!document.getElementsByTagName) return; formBody = document.getElementsByTagName("form").item(0); formElement = formBody.getElementsByTagName("formitem").item(0); div1 = formElement.getElementsByTagName("div"); alert("div1 items: " + div1.length); } Okay, there is one more thing on the page....a button: <div class="column"><br /> <button onClick="addRow2(0, true);return false;">+</button></div> The problem here is Chrome and Firefox (go figure) are returning a 1 to the user because they're picking up the <div> tag perfectly. Internet Explorer is returning 0 every time and I can't figure out why. Why is it not getting the <div> tag? Thanks!
-
I have a drop down box like so: <select name="form1[20][Coverall Type]" id="Coverall Type" value="" onmouseover = "hint('Select overall type', 'coverallHint')" onmouseout = "nohint('coverallHint')" > <option value = "Short (5'2 - 5'7, 2'7 inseam)">Short (5'2" - 5'7", 27" inseam)</option> <option value = "Regular (5'8" - 6'0", 28.5" inseam)">Regular (5'8" - 6'0", 28.5" inseam)</option> <option value = "Long (6'1" - 6'2", 30" inseam)">Long (6'1" - 6'2", 30" inseam)</option> <option value = "X-Long">X-Long</option> </select> And I email it with PHP like so: $message = ""; $form = $_POST['form1']; // Loop array foreach($form as $rowid => $columnarray) { // Loop each column for this row foreach($columnarray as $column => $value) { $message .= $column . ": " . $value . "\n"; } } //define the headers we want passed. Note that they are separated with \r\n $headers = "From: ******@*****.com\r\nReply-To: *****@******.com"; //send the email $mail_sent = @mail( $to, $subject, $message, $headers ); But when I get the email, it looks like so: Coverall Size: 30 Coverall Type: Short (5\'2 - 5\'7, 2\'7 inseam) So as you can see, it inserts the slashes to escape the quote characters. I'm also trying to put double quotes in there for inches but I'm just working on the single quotes right now. Any idea why it's doing this? How do I stop it? Thanks.
-
I've got a form that submits values to itself and the user input values are saved into the database in a table with an auto incrementing ID. After SUBMIT is clicked, it reloads the page and all the values are gone, I'm wondering how I can get it to keep the values so that the user can make a single change and resubmit again. I know I can save all the values into the session or in the URL or pass them through $_POST in an array but I'm wondering what is the proper way to do this. Thanks.
-
I looked at that and it looks like it just downloads it to your local machine, it doesn't allow me to use any sort of mailer with it. I can just click PRINT... and then Save As PDF to do the same thing. Is there maybe a way to just use the PHP mail() function to mail a HTML email version of the current page?