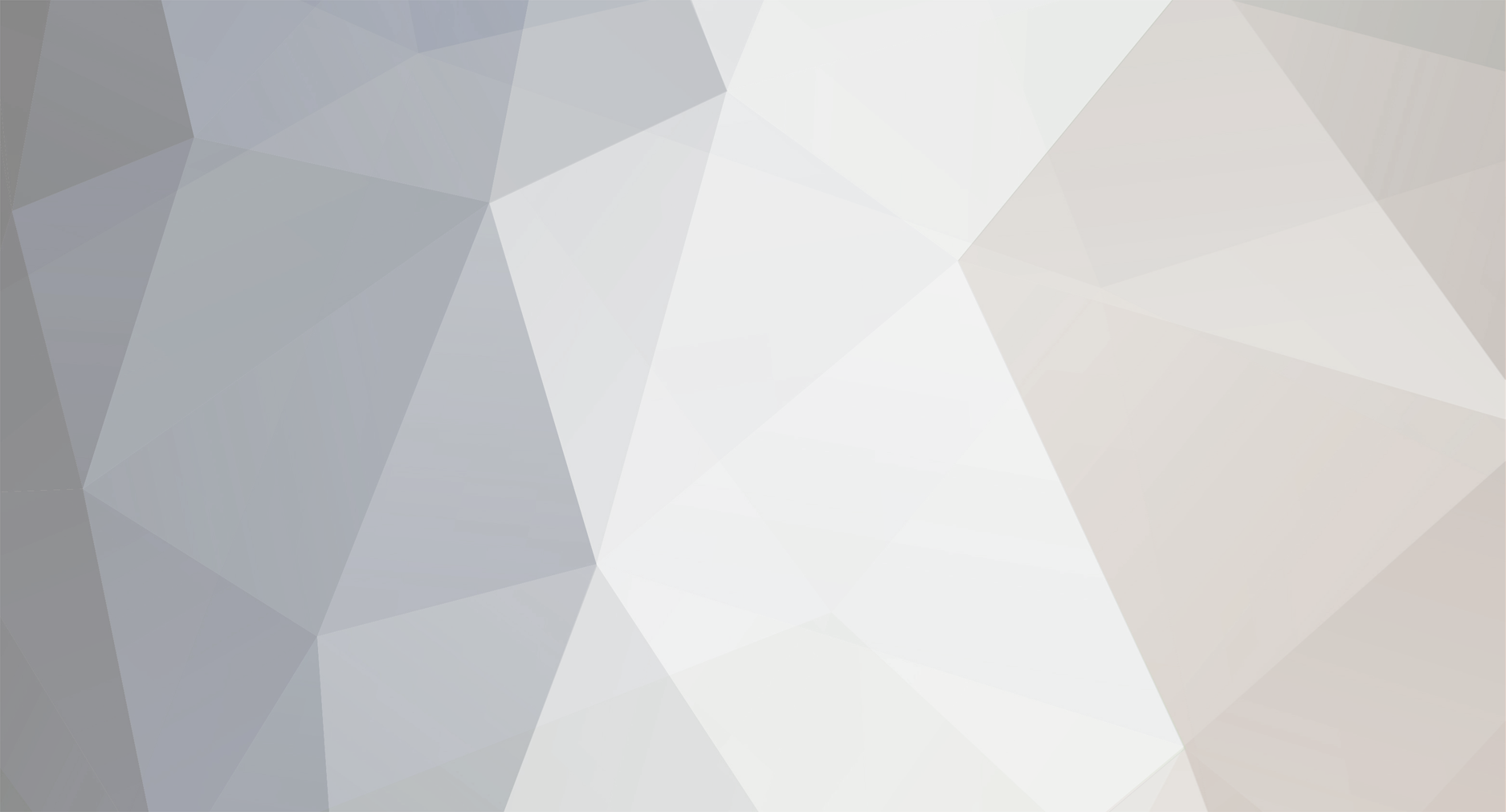
DeX
Members-
Posts
258 -
Joined
-
Last visited
Everything posted by DeX
-
function getTotalQuantityBuildingProduct($productId, $showInsulation, $showPerma) { $quantity = 0; foreach ($this->getQuantityBuildingProduct($productId, $showInsulation, $showPerma) as $productQuantity) $quantity += $productQuantity; return $quantity; } The getQuantityBuildingProduct() is the method I already posted. Since a product can have multiple quantities (3 doors at one width and 5 doors at a different width), the getQuantityBuildingProduct is an array of the quantity groups. The getTotalQuantityBuildingProduct simply adds together all the quantities from the array and gets a total. That total is multiplied by the price for a total cost of that type of product.......for most products. Some products use the widths and lengths to calculate the final cost as well if it is dependent on price per square foot (tiles, poly or sheets of metal).
-
I'll lay out my whole issue here just in case I'm incorrect about where the problem is. I wrote some software in PHP to build a quote for a building company. I have a manual quantity calculation for every product they have in inventory and each time they modify the inputs, like the building dimensions, it recreates the quote and spits out a new price based on the quantity calculations for each individual product being used. There are a number of inputs on the page and I make an AJAX call to the server to recreate the quote, then display the new quote once the AJAX return is done. This includes running all the quantity calculations. When requesting the quantities, it goes into a switch statement consisting of 300 cases until it finds the matching ID of the product being requested. It then runs the quantity calculation I have coded in there which may or may not rely on quantities of other products in the system as well. I have statements at the top and bottom of the switch statement to check an overall array to see if that item ID is already in the overall array (if the quantity has been calculated already). If so, it returns that quantity instead of recalculating it. If not, at the bottom of the switch statement it adds that quantity to the overall array in case that product quantity is requested again. Here's how it looks: function getQuantityBuildingProduct($productId, $showInsulation, $showPerma) { $quantities = array(); if (isset($this->overallQuantities[$productId])) return $this->overallQuantities[$productId]; switch($productId) { case ($this->cornerMoulding) : $quantity = 0; if ($this->isInsulated() || $showInsulation) { $quantity += ceil( $this->getTotalQuantityBuildingProduct($this->threeByThreeFixedWindow, $showInsulation, $showPerma) * 1.5 + $this->getTotalQuantityBuildingProduct($this->fourByThreeFixedWindow, $showInsulation, $showPerma) * 1.5; ); $quantity += $this->getTotalQuantityBuildingProduct($this->threeFootStandardDoor, $showInsulation, $showPerma) * 2.5; } $quantities[] = ceil($quantity); break; case ($this->smokeDamper) : $quantities[] = ceil(($this->getTotalQuantityBuildingProduct($this->smokeStop, $showInsulation, $showPerma) + $this->getTotalQuantityBuildingProduct($this->fireStop, $showInsulation, $showPerma)) * 3); break; case ($this->tuckTape) : $quantities[] = ceil($this->getTotalQuantityBuildingProduct($this->poly, $showInsulation, $showPerma) / 2000); break; case ($this->ceilingOnlyLabour) : if ($this->isCeilingOnly()) $quantities[] = 1; break; default: break; } $this->overallQuantities[$productId] = $quantities; return $quantities; } It's taking about 10 seconds to get the entire quote back from the server since I implemented these switch statements. I have others to also get the widths, heights and lengths of the products just like I get these quantities. I used to have a separate function to get every single quantity, width, length and height but that was a lot of individual functions so I found this easier to add new functions to. Is this the cause my the slowdown? How can I make it faster? My dream goal is to somehow store all of the quantity calculations in the database but I have no idea how I can do that since they're all different and sometimes rely on quantities of other products. I think having them in the database may speed things up (or slow them down) but I'm just not sure how to accomplish that right now. For now I'd just like to speed up the current way.
-
No, they're using pagination to display 20 results at a time. You click the arrow and it displays the next 20 results. I want to somehow get all the results using a HTTP GET request on my own PHP page.
-
Yes, I'm trying to pull a list of stores from a mall directory but they have the listing split across 3 pages and you browse the pages using javascript links. I want to pull all the stores from the 3 pages. More specifically they have a Javascript link for returning all stores, I want that one. EDIT: This one - http://www.crossironmills.com/shopping/store-directory/alpha/
-
I'm writing a PHP script to pull information from another website which I don't own. That website uses javascript for pagination, splitting data across 3 pages. They also have a function to list all their data on one page but it requires clicking the link on the page which runs a javascript AJAX call. I want to pull all that data with PHP but how do I emulate the Javascript AJAX call in my code so I can get all that data instead of just the first page? Even if I could just call that function and then parse the information it returns to me. Thanks.
-
I built a Bootstrap site but when I view it on my phone (Samsung Galaxy S3), it doesn't collapse the navigation menu into the mobile button like I thought it would. I did some research and realized that my phone is actually the same resolution as my desktop (1920x1080) so it's giving me the desktop view. Bootstrap, to my surprise, only seems to be responsive to resolutions, not physical screen sizes. How do I overcome this? I want to build a site which is responsive to physical screen size, regardless of resolution.
-
Okay, I've had success with mod_rewrite and .htaccess, I can now redirect URL of http://www.example.com/users/bob to point to http://www.example.com/some-page.php?action=getUser&user=bob. So now that I've been successful with that I'm a little confused how I would use this. If I wanted to display a page all about Bob and his associated information in the database, what do I do with this? Am I right to assume I would just have a regular user.php page which I would rewrite to and that would load all the information using $_GET['user'] to figure out which user to display? If so then that's simple enough but what about the API I keep reading about? I keep reading that I should create a rest API file so what would this look like and what is it used for? As of now I have this, is this pointing in the right direction? <?php require_once('include/include.php'); ?> <?php $action = $_GET['action']; $userId = $_GET['user']; switch($action) { case("get"): $controller::getUserById($userId); break; case("delete"): $controller::deleteUser($userId); break; } ?> My include.php file has the following: <?php require_once('controller/mainController.php'); require_once('model/mainModel.php'); require_once('view/mainView.php'); require_once('controller/loginController.php'); // call on every page to start the session MainController::sec_session_start(); $model = new MainModel(); //It is important that the controller and the view share the model $controller = new MainController($model); $loginController = new LoginController($model); $view = new MainView($controller, $model); // if (isset($_GET['action'])) // $controller->{$_GET['action']}(); ?> This is the same model/view/controller setup I use for all my sites, I find it easy to separate the code this way. So I'm using this controller in my API to call methods. If the method requires database access I pass it to the model. I need direction on the API though, do I need one? If I use it to delete a user, how do I then display the resulting page after the user is deleted? Just do a PHP redirect?
-
Cool, I'm off to do some research on how to change the URL for those pages. Can you tell me what it's called or somehow point me in the right direction to find examples on that?
-
Very interesting, thank you. One last question, is it acceptable web programming for me to be using example.com/user.php?id=123 instead of example.com/users/123 I'd put a question mark at the end of that question but it looks confusing so I left it off. I'm hoping my site in question is going to blow up big, something like Kijiji and be used quite a lot on a daily basis. I want it to be as perfect as possible.
-
I'm quite good at PHP but I thought it was time to learn this ReST thing people are talking about. As of right now I've got a web application written entirely in PHP and has a MySQL database full of......let's say users. Anyone logged in can browse a list of the other users (users.php) and can then visit that user's profile (user.php). There are no security limitations for the sake of our example. Right now when the user clicks another user, it brings them to a URL (http://www.example.com/user.php?user=123) and the page (user.php) makes a call to the model which checks the database for a user with ID 123, then returns any information for that user. I'm wondering if it would be more professional to have the user go to a more friendly URL (http://www.example.com/users/bob) which would load user.php with all the information about Bob (only a single Bob in the database). Is it reasonable to switch from my way to this ReST way? Can someone point me (or provide) a tutorial for the simplest example you can imagine? I would just need it to get to the SQL call, I can do all the database authentication and calling myself, I just want to know how everything is set up and I can build on it from there. I can't find anything this simple online, they're all too complicated with extra features I don't want. Thanks.
-
How do I get database values for currently logged in users?
DeX replied to DeX's topic in PHP Coding Help
Session hijacking and poisoning? Is that something I should worry about? -
How do I get database values for currently logged in users?
DeX replied to DeX's topic in PHP Coding Help
How do I keep track of the currently logged in user if I don't store the ID in a session variable? If it's in a session variable then the user is free to change it as they wish. -
I'd like to display a user profile for the currently logged in user so they can edit their contact details. I already have a secure login scripted which works well and it stores the user_id, username and a login_string into session variables. The login_string is a long alphanumeric string of hashed values used when checking the logged in status of the user but it's not stored in the database. My concern is if I pass the user_id from the session variable to the model to get the user contact information, the user can easily change that variable in order to display someone else's information. So should I continuously hash the same variables to compare the login_function or should I just store it in the database once the user logs in so that's it's readily available to do a comparison on any time I need database information. Does that make sense?
-
Is sha512 an acceptable encryption algorithm for passwords?
DeX replied to DeX's topic in PHP Coding Help
So just a random string generator for every salt and store it in the user table? -
Is sha512 an acceptable encryption algorithm for passwords?
DeX replied to DeX's topic in PHP Coding Help
Great, thanks, so I'll incorporate SSL, send the unhashed password, then salt and hash it on the server side. Which things should I be salting with the password for best security? The IP? The browser session ID? Timestamp? And lastly, should I be storing the salt in the database or should I just use the same method each time (for example every second letter of their name or something)? -
I'm writing a huge PHP secure login script for a heavily used website so I need to do 2 things securely: a) transmit the password from the form to the webserver. b) store the password in the database. I'm using a JavaScript sha512 function I downloaded in order to encrypt the password prior to sending it to the webserver and I'm storing encrypted passwords in the database using hash("sha512", $password) as an encryption function. Is this good enough? Is there a different encryption algorithm I should be using? The process I'll be using is encrypting with javascript, sending to the webserver, adding a salt and encrypting again for storage.
-
I'm building a website with a layout I've never tried before but I've seen it work well on a lot of other websites. I'm wondering if someone here can help me accomplish doing this, I haven't been able to figure it out. This is the design I got from my designer which I need to code: I removed the middle white area so you wouldn't know my website idea. What I want is a 1200 pixel wide center area and I want the outside parts to fill the remaining portions of the page, regardless of the screen width. So (correct me if I'm wrong), I was thinking I need the left div, center div and right div. The center is fixed width and the left/right parts are variable but how do I accomplish this?
-
This is my very first MVC style PHP scripting so I'm wondering if I've done it the very best I can. I've done a lot of reading and this is what I just got working. First I'll explain how it works: - User goes to www.example.com/mvctest.php and gets a login prompt. - User tries to skip that and go directly to the home2.php page to try and view the site without logging in first. He gets automatically redirected back to mvctest.php for login. - User gives bad credentials, he gets routed back to mvctest.php for a bad login to try again - User gives proper credentials and gets logged in, gets redirected to home2.php on successful login. - User closes their browser window, then opens another and goes back to the homepage at mvctest.php. Instead of getting the login prompts again, he gets redirected straight to home2.php because he's already logged in (cookies remain until midnight). - User clicks logout button. Their cookie is set to a time in the past and they are redirected back to mvctest.php and prompted for login credentials. That's all the actions I could think of. Now on to the code. It all works except I commented out the disconnecting from the database because it was doing that prematurely and I wasn't able to log in using my mysql link because it had been closed before I got to use it. That's small stuff though, I'm wondering if people can comment on everything, I want this to be as good as it can be. Thanks. mvctest.php: <?php require_once('controller/login.php'); require_once('model/database.php'); require_once('view/login.php'); include('includes/include.php'); ?> <form action="?action=login" method="post"> Username: <input type="text" name="username"> Password: <input type="password" name="password"> <input type="submit"> </form> include.php: <?php $model = new DatabaseModel(); //It is important that the controller and the view share the model $controller = new LoginController($model); $view = new LoginView($controller, $model); if (isset($_GET['action'])) $controller->{$_GET['action']}(); ?> home2.php: <?php require_once('controller/login.php'); require_once('model/database.php'); require_once('view/login.php'); include('includes/include.php'); ?> <button type ="button" onclick ="window.location = '<?php echo $_SERVER['PHP_SELF'] ?>?action=logout'" >Log Out</button> controller/login.php: <?php class LoginController //extends Controller { public $view; public $databaseModel; public $loginModel; /** * Initializes the Vew and the Model. */ public function __construct($model) { require_once('model/login.php'); $this->connection = $model->connectToDatabase(); $this->loginModel = new LoginModel($model); $this->view = new LoginView($this, $model); if (!$this->loginModel->checkLogin() && !$this->isLoginPage()) { $this->view->showLoginPage(); $model->disconnectDatabase(); } else if ($this->loginModel->checkLogin() && $this->isLoginPage())// must wait for loginModel to return before disconnecting { $model->disconnectDatabase(); $this->view->loginSuccess(); // go somewhere else if already logged in } else $model->disconnectDatabase(); } /** * The "index" action. * Called by default if no action is defined. */ public function index() { $this->show(); } /** * The "show" action. * Simply instructs the View to display the form. */ public function show() { $this->view->showForm(); } public function isLoginPage() { $urlPath = explode("/", $_SERVER['PHP_SELF']); if ($urlPath[count($urlPath) - 1] == "mvctest.php") return true; else return false; } /** * The "process" action. * Processes the form data, either sending it to the model to be * saved into the database, or displays errors if the required * fields are not present. */ public function login() { $requiredFields = array('username', 'password'); $data = array(); $error = false; foreach($requiredFields as $_field) { if(!isset($_POST[$_field]) || empty($_POST[$_field])) { $error = true; $this->view->showLoginError("Field '{$_field}' needs to be filled."); } else { // Skipping any sort of validation, for the sake of // simplicity. $data[$_field] = trim($_POST[$_field]); } } if($error) { $this->view->showForm(); } else { if($this->loginModel->login()) { $this->view->loginSuccess(); } else { $this->view->showLoginError("Username or password is invalud. Please try again."); } } } public function logout() { if ($this->loginModel->logout()) $this->view->showLoginPage (); } } ?> model/database.php: <?php class DatabaseModel { public $connection; public function __construct() { } public function connectToDatabase() { require_once('includes/config/config.inc.php'); $connection = mysql_connect(HOSTNAME, USERNAME, PASSWORD); if (!$connection) die('Could not connect: ' . mysql_error()); $database = mysql_select_db(DATABASE, $connection); if (!$database) die('Could not select database: ' . mysql_error()); $this->connection = $connection; return $connection; } public function disconnectDatabase() { // mysql_close($this->connection); } public function getConnection() { return $this->connection; } } ?> model/login.php: <?php class LoginModel { public $connection; public function __construct($databaseModel) { $this->connection = $databaseModel->getConnection(); } public function checkLogin() { if (isset($_COOKIE['login'])) { if ($_COOKIE['login']) { return true; } else { return false; } } } /* public function inputs($fieldNames, $data) { foreach ($fieldNames as $field) { echo "data: " . $data[$field] . ", field: " . $field . "<br />"; } } */ public function login() { $sql = mysql_query("select salt, password from user where username = '" . $_POST['username'] . "'", $this->connection); if (mysql_num_rows($sql) > 0) { $sqlRow = mysql_fetch_assoc($sql); if (sha1($_POST['password'] . $sqlRow['salt']) == $sqlRow['password']) { setcookie("login", true, $this->getLoginCookieExpiry()); return true; } else return false; } else return false; } public function logout() { setcookie("login",false,time()-10); return true; } public function getLoginCookieExpiry() { return mktime(23, 59, 59, date("m"), date("d"), date("y")); } } ?> view/login.php: <?php class LoginView { protected $model; protected $controller; public function __construct(LoginController $controller, DatabaseModel $model) { $this->controller = $controller; $this->model = $model; } public function showLoginError($error) { return $error; } public function loginSuccess() { header("location: home2.php"); } public function showLoginPage() { header("location: mvctest.php"); } } ?>
-
How Do I Handle The Multiple Database Connects And Requests With Mvc?
DeX replied to DeX's topic in PHP Coding Help
Okay, so this would be option B from what I posted earlier? -
How Do I Handle The Multiple Database Connects And Requests With Mvc?
DeX replied to DeX's topic in PHP Coding Help
So according to this website: [http://www.potstuck.com/2009/01/08/php-dependency-injection/], does that mean I would need a database setter method in every new model file I make and also a databaseConfig setter? That seems like a lot of unnecessary and redundant code. -
How Do I Handle The Multiple Database Connects And Requests With Mvc?
DeX replied to DeX's topic in PHP Coding Help
Same database but multiple models which connect to various tables in that database. I could either:a) connect to the database inside each separate model. b) connect to the database in the controller and pass the returned connection into each model used c) extend the database_connect model in every other model which uses it (I couldn't figure this out)Which is best? -
I just started a MVC style web application and I have a model which has a sole job of creating a connection to the database. I also have more models which connect to various tables to do various jobs but they're separate files in the model class. How do I use the connection in the database_connection_model in all the other model classes? Do I pass it into every model I use from the controller or is there some other way? I've considered just connecting in the model constructor but then where do I close the connection? Please help. Thanks.
-
Is this what I have with my match_quote_building_materials table I outlined above?
-
Correct me if I'm wrong but I need to store the pricing of each material at the time of the quote because the pricing changes weekly. So if the building is a confirmed sale 2 weeks later and we recalculate the pricing to send out all the purchase orders for materials, all the total pricing will be off because it'll be using the new prices.
-
I have a web application created in PHP where super users can moderate user profiles. When they make a change and save it to the system, I'd like to show a small notification in the lower window to show the outline the change they made and that it was saved. The window should be styled with CSS and only display for a couple of seconds. I don't expect anyone to write out all the code for me, does anyone know what this is called so I can research it? I keep getting tutorials on alert boxes but those aren't what I want. Would I just use a DIV and hide / show it?