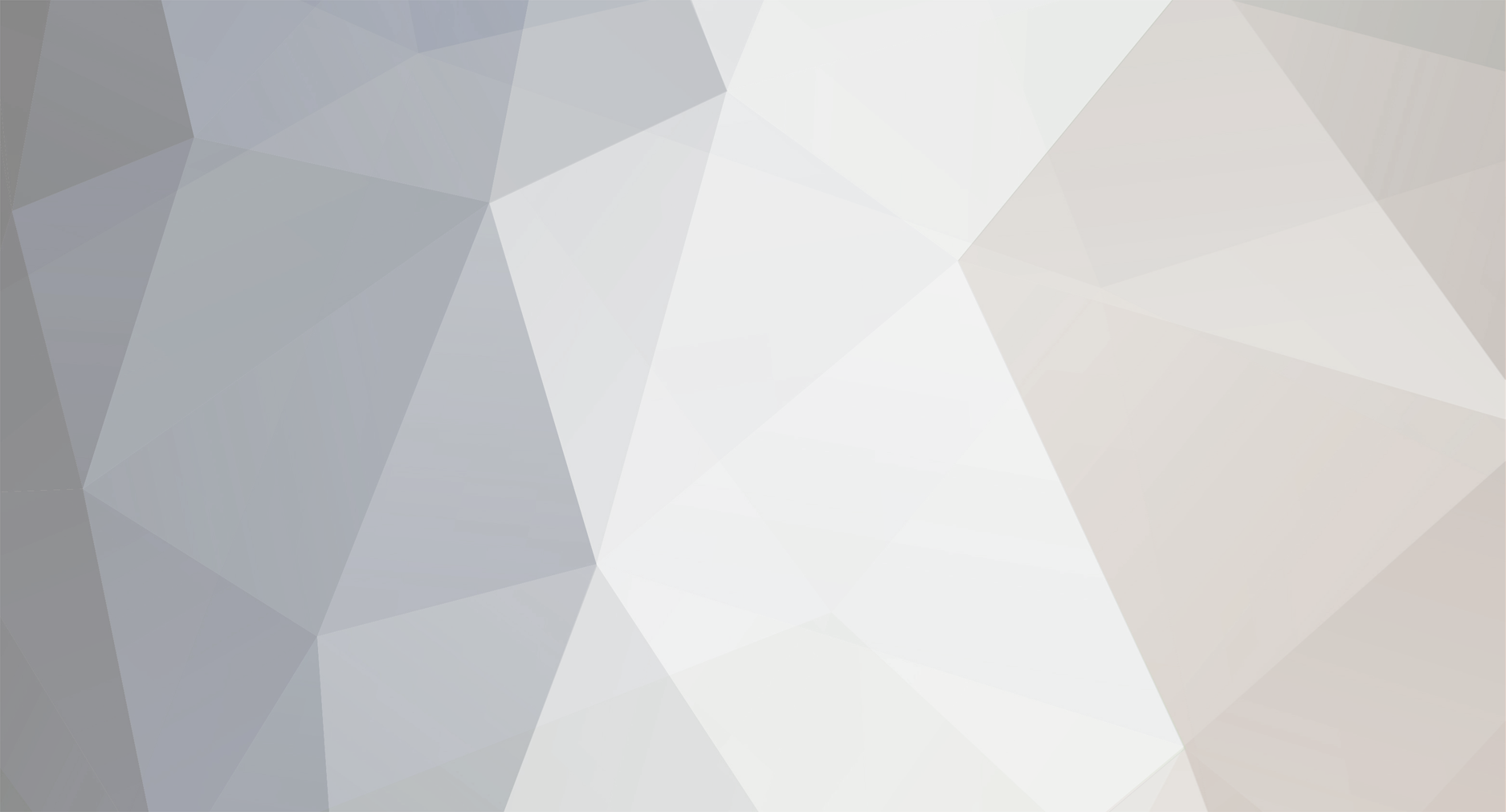
DeX
Members-
Posts
258 -
Joined
-
Last visited
Everything posted by DeX
-
Okay, here's the big picture. I've got a table for building materials, everything from lumber to sheet metal to soffit to screws. Each one has a unit_price stored in the table and a quantity is calculated at quote time based on square footage. When the quote is done, I store all the required building materials for that quote along with each of their individual unit prices and the calculated quantities for each material. Here is an example of everything I just went over: building_products: id | name | unit_price | price_per_k | board_feet | purchase_order_id 1 | 2x4x10 | 0 | 420 | 800 | 5 2 | exterior metal | 2.33 | 0 | 0 | 3 3 | trim screws | .057 | 0 | 0 | 3 4 | ridge cap | 10.34 | 0 | 0 | 2 So all the products are in there. Lumber price is calculated with a formula using board_feet and price_per_k and all the others have their own unit_price. The last column is for which purchase order it gets displayed on. Different purchase orders are for lumber, metal, doors and more. When the quote happens I need to store every building material used for that building: match_quote_building_materials (I made up the numbers for simplicity, they would normally match their foreign keys): id | building_product_id | quote_id | unit_price | board_feet | price_per_k | quantity 1 | 1 | 1001 | 2.12 | 0 | 0 | 12 2 | 5 | 1001 | 1.87 | 0 | 0 | 74 3 | 6 | 1001 | 0 | 800 | 420 | 34 And this is where I'm confused, I currently have a QUOTE table which stores the information from the actual quote itself where the user entered information. Currently it works like this: QUOTE (again, numbers made up): id | customer_id | salesman_id | date_quoted | is_insulated | drawing_price | interior_labour_rate | exterior_labour_rate | height_premium 1001 | 2001 | 3001 | 01-01-2001 | 1 | 300 | 2.34 | 3.45 | 600 1002 | 2002 | 3001 | 01-01-2001 | 0 | 300 | 1.79 | 2.21 | 400 And before I explain that table, there is an area in the program where the salesman can set the various labour rates based on various square footages as well as what the drawing price and height premiums currently are. They are constantly changing these values in the program (first table in first post above) based on market trends and seasonal changes. So of course I have to store the value of that option at the time of quote. Hence the drawing_price, labour_rates and height_premium columns in the quote table. There are also other things I'm storing there such as the current commission rate for the salesman, the current markup percentage on their buildings, the current commission rate for the operations manager and the list continues. They all go in this quote table. My question is whether I should keep this as strictly a quote table or if I should have a separate match_quote_options table to add all these random values into or if I should have a separate match table for each value. Or feel free to tell me if I'm way off.
-
I've got a system where the user fills out a form on a website in order to get a quote for a building. Depending on the square footage of the building they inquire about, it will price it with a number of different variables based on this square footage. So I've got a table in my database which has columns for minimum / maximum square footage and a price for each. The final price of the building is calculated using the price of the size which falls in between the proper minimum / maximum square footage: name | minimum | maximum | price Interior Labour | 0 | 10000 | 2.75 Interior Labour | 10001 | 20000 | 2.45 Interior Labour | 20001 | 30000 | 2.13 Interior Labour | 30001 | 40000 | 1.98 Interior Labour | 40001 | 50000 | 1.86 Exterior Labour | 0 | 15000 | 2.34 Exterior Labour | 15001 | 30000 | 2.04 Exterior Labour | 30001 | 45000 | 1.93 Exterior Labour | 45001 | 50000 | 1.88 There are more in the table but this is an example. My first question is if I should have a separate table for each of these options based on square footage or if I should have them all in the same table like this. There are probably 4-5 distinct options like this. My second question is if I should store the values into a table like so when the quote is completed: quote_id | interior_labour_rate | exterior_labour_rate | customer_id 1000 | 2.45 | 2.34 | 25 1001 | 1.86 | 1.88 | 26 There are much more columns in this table such as the price of the quote and such. I'm wondering if I should do it like this or if I should have match tables for each individual option of the quote. So there would be a match table for the interior rate, one for the exterior rate and another for the customer_id. Thanks.
-
Nice, thank you. I have 2 questions though. 1. What does this part do? Where is the getInstance() method? what exactly is this doing? 2. How does this interact with the model to get the name from the database? Thanks again.
-
Okay so let me know if I got this right. I navigate to edit the quote and it goes to home.php?quote=555. HTML - input tag with value of $this->view->customer_first_name VIEW - customer_first_name method which returns $this->controller->get_customer_first_name() CONTROLLER - get_customer_first_name method which returns $this->model->get_customer_first_name() MODEL - get_customer_first_name method which makes a call to the database with some SQL and returns the first name of the customer associated with the quote number from $_GET['quote'] Is this right? Where does the quote number get accessed? Is it retrieved by the controller and passed as a parameter to the model? Or does the model get it directly? Thanks.
-
I'm really trying to convert my messy code into a more readable MVC architecture because it's quite a mess right now. I've been doing some research on MVC and trying to figure out how it works. I'll explain here how my web application works and I'd like someone to please tell me if I've got the right idea for setting it up for MVC. Right now I have a system where a building company owner can enter customer details along with some building dimensions into a web page and it will calculate the cost of that building for that customer. It's way more complicated than this but right now I'm starting simple with the MVC conversion. When they do the quote, it gets assigned a number from the database and they can then edit this quote with new details if the customer wants another price on the building with different dimensions. So the owner goes to home.php, does the quote and the quote is generated with a database ID number. When they edit the quote, they get sent back to home.php?quote=123 but this time all the input fields are filled in with the values from the quote and the owner can now change the one thing the customer wants changed and resubmit. So here's where I start asking questions. Let's just work with one of these input boxes, the customer's first name. If I understand it correctly I would have the HTML code with a call to the view to get the text for the text inside that input box. Somehow it traverses through the controller to the model where the model would check to see if the quote variable is set in the URI (home.php?quote=123). Since it is, the model would then make a call to the database to get the customer's first name associated with that quote with some SQL and a getter function would return the value. It would then traverse back through the controller to the view and be passed to the HTML code which asked for it. Why do I need the controller here? Do I use it at all? Am I right in the role of the model? Would I do this for every one of the input boxes on the page? There are probably 40-50. Thanks.
-
I have this. I have a customer table which stores all the customer information when the quote is done. There's also a building_products table which stores every product used and all associated attributes/prices for each one. Then there's a match_building_product table which matches each quote with all the products/prices/sizes used for that quote. It's this match_building_product table which information is pulled from in order to create each purchase order. So thank you, it's good to know I did it correctly. This is what I've been trying to explain since the beginning that I'm trying to avoid. Right now I have one single function (or it could be a page) which has the layout for the purchase order and generates it as needed. If I have one page to display it and another to edit it, any changes to the layout of the purchase order would need to be done in both places. I have a single function to avoid this, I want to use just the one function for both so any edits only need to be done in the one place.
-
Javascript - How can I replace a textarea with its contents?
DeX replied to DeX's topic in Javascript Help
There is only 1 text area on the screen at any time but the id/name could change depending on which AJAX function is called to display it. Here is the exact layout, no shortening: <table class = "site-table"> <thead> <tr> <th>Notes</th> </tr> </thead> <tbody> <tr> <td> <textarea id = "notes5" rows = "3" cols = "105" value = "" ></textarea> </td> </tr> </tbody> </table> -
Research is telling me this has to be done in Adobe InDesign prior to exporting it as a PDF file. At the design stage. Anyone have any experience with this?
-
Javascript - How can I replace a textarea with its contents?
DeX replied to DeX's topic in Javascript Help
Yes, sorry, not sure how I left that out. var textareaBoxes = document.getElementById("purchase-order-area").getElementsByTagName("textarea"); var textareaBoxesCount = document.getElementById("purchase-order-area").getElementsByTagName("textarea").length; -
I have a textarea <textarea id = "notes5" rows = "3" cols = "105" value = "" ></textarea> and I have some javascript for (i = 0; i < textareaBoxesCount; i++) { textareaBoxes[i].parentNode.innerHTML = textareaBoxes[i].value; } Whenever this runs it always replaces the textarea with nothing, regardless of what I type into it. Is the textarea value attribute blank until the form is submitted? I don't want to submit the form until after it is replaced, is this possible? Just so you know the rest of it works, changing it to a value like this: for (i = 0; i < textareaBoxesCount; i++) { textareaBoxes[i].parentNode.innerHTML = "asdfasdf"; } does replace the textbox with "asdfasdf". So it does work, it just isn't getting the value of the textarea like it should. Any suggestions?
-
One of my clients just sent me a .pdf file she created using InDesign and asked me to add an interactive menu to the bottom of it. Has anyone heard of this? How do you put code into a PDF file? I just don't get it, a PDF is supposed to just be like a rich text file, correct? I mean, can I edit the PDF code somehow?
-
I didn't really know what views were so I looked them up. It seems like a stored SQL query always returning the same rows from the same tables which might help out in some situations but I would need to pull all items with a specific purchase_order_id (belonging to that specific purchase order) and a specific quote_id (belonging to that specific quote). Can I use a view to view a table of items with a certain quote_id and purchase_order_id? There are about 10,000 quotes in the system right now. Each one has about 100 separate pieces of material in its relevant quote-material match table. Related to my original question, would I be able to parse the HTML if it contains PHP calls? Would the calls be replaced by their return values or would they be passed through as raw PHP? To explain it better, I have function getPurchaseOrder() which returns all the HTML with a few other PHP calls in there like getting the customer's name and phone number. Is the name already obtained when I get the return from getPurchaseOrder() or is it done when rendered by the browser?
-
When the quote is confirmed it takes all the materials used to quote that item from the database and creates all the purchase orders, saving each one's HTML layout into the database and also auto creating all the PDF for each one. If they click any of the purchase order links, it'll load up the editable purchase order (HTML from database) on the screen with input boxes for them to edit certain columns if they want. If they do edit, they can save and the new purchase order HTML is sent again to the database and that PDF is generated again with new values. The process is as follow: - salesman quotes a job, then another, then another, then another - one of the quotes is confirmed as a sale - when confirmed, a function is called to generate HTML in a table format which is sent to the PDF generator which creates the purchase order PDF and saves them in a folderon the server. This is done recursively for each purchase order so they're all created and ready to go. - the purchase orders are not used for anything up to this point and may sit there for a day or two until the guy who orders the buildings looks at it - guy ordering buildings comes upon this confirmed quote and wants to send out all purchase orders to retailers to order materials - he clicks the link to view the lumber purchase order so the HTML (same as before) is generated to display on the screen so he can see it including the input boxes. - he decides an extra 2x4x10 is required for this particular building so he changes the quantity and resaves it. - this sends the generated HTML once again to the database to be saved and also sends the same HTML to the PDF generator to recreate the new PDF - the customer calls back and says he'll supply the windows from a local seller - the guy ordering materials reloads the most recent purchase order and modifies quantities once again, resaving, sending new HTML to the database and recreating the PDF - most recent PDF is emailed to retailer to order materials The reason the HTML is saved to the database is for ease of editing. In the second last step the guy ordering materials wants to load the purchase order with all the most recent changes, not the original one that gets generated from the original materials list when the quote is first confirmed. So either I create another couple of tables and store every item and every quantity/width/height/price/length again every time they modify a purchase order or I just store the HTML. Then load the HTML so they can edit it again. So basically a PHP function creates the initial purchase order HTML and any subsequent saving is done by just sending the HTML from the screen which is easy. I'm dealing with the inital creation which is done with a PHP function and doesn't have anything to do with what's on the screen.
-
All the items are already stored in a database table, that's where they're pulled from to create the purchase order in the first place. But if I have 2 separate functions, one to pull the purchase order with input boxes and another to pull without input boxes, then I have 2 to modify any time a change is made. I want just one function creating the purchase order so modifications are kept to a minimum. Should I reconsider?
-
It's in this basic format, just a regular table: <table> <thead> <tr><th></th></tr> </thead> <tbody> <tr> <td></td> <td><input type = "text">information</input></td> <td></td> <td></td> </tr> </tbody> </table> That's basically it with many more rows. I want to strip those input boxes but keep the information inside them. Easy with Javascript but I have no way to call the Javascript function inside a PHP function. LONG EXPLANATION: Here's the long version of what I'm trying to do, don't read this unless you're really interested in what's happening. I got a site where my client can create quotes, they do 100 or more per day. Whenever a quote is confirmed as a sale, they click a button which creates all the purchase orders and also auto-generates the PDF for each one and saves it to the server. That way they can click a button on the page and it sends out all the purchase orders for that quote to order all the materials from each retailer.......the retailer gets an email with a link to the PDF on the server. I'm calling a PHP function which puts together that specific purchase order including all styling and relevant materials, and stores the HTML into a table so it can be viewed/edited later. I would also like to have the PDF generated at this time but the HTML has the input boxes in it. The reason the input boxes are there is because my client can click any purchase order they want and edit quantities/prices, then resave it which stores the new HTML into the database and creates the PDF again with the new values. Just in case they want an extra couple of pieces of material on that order or the customer is going to be supplying some of it on his own. Then if the customer changes his mind again, my client can go in once again, pull up the purchase order, edit it a second time and resave everything again putting all the HTML into the database again and creating the purchase order for a third time. Now if everything is good they send that purchase order to the retailer to order materials.
-
I have a string of html on a page with some <input> tags in it and when the user is done entering information into the boxes, then can hit submit and it fires off the html to a PDF generator. The problem is the generator simply ignores the <input> tags so the whole column is just blank. I need to strip the tags but keep what the user entered in them prior to sending it to the generator. Is there any way I can get rid of the tags surrounding the data in that column? Everything is done in a PHP function in a separate class so it's totally separate from the page the data is displayed on. There is no form being submitted anywhere to run a javascript function on, all data is pulled from the PHP function.
-
This should be very simple I know but here is why it doesn't work for me. I have a purchase order displaying on the screen and I give my client the option to edit some of the fields before: a) the purchase order HTML gets sent to a PDF generator on the server to auto create and save it in PDF format. b) the purchase order HTML is also sent to the database to be saved in case they want to bring it up in the future and edit it again and re-save. Regardless of all that, I have the purchase order HTML on the screen with input (text) boxes around fields they can edit. When they edit the field and the HTML is sent to the database to be saved for later, the actual value property of the input element hasn't changed. So loading it up later shows the old value, not the one the client entered. So I tried to assign the .value property of the input element in Javascript prior to saving it to the database and it does indeed change the value visible inside the text box to whatever I have it set at but it doesn't actually change the .value property of the element. If I do an "inspect element" in Chrome, I can see the .value property hasn't changed from the original value. So how can I change the actual .value property associated with the element so that it displays with the new value when I pull the HTML code back out of the database for later use? Or any other suggestions welcome as well, thanks.
-
No, the input boxes are loaded with default values. I want to send it all to DOMPDF to create the base PDF file without any changes to it. I also want to give the user the ability to edit any of those fields and save it again.
-
Short question: How can I get a string of HTML code and replace all the <input> tags with their values? Long question: I have a web page with a purchase order on it in HTML format and one or more of the columns is editable via input boxes. So the user can change the quantity/length of the material before submitting it. Once they choose to save it, it sends the string of HTML to a PDF generator (DOMPDF) which converts the HTML to a PDF file and saves it to the server. The user then has a hard copy of the purchase order PDF saved for each job. The problem is DOMPDF doesn't do well with <input> tags, it just leaves them as a blank space. So I want to take that string of HTML and replace all input boxes with their values before sending it to DOMPDF. Currently I'm using Javascript to do just that when the user clicks to save the modified purchase order. The problem with this is it only works for when the user modifies the purchase order and saves a new modified copy. I need to save a base unmodified copy of it when the page loads so this would strip all the input boxes from the page as soon as it loads and the user would no longer be able to edit anything. So I need something to send the string to DOMPDF when the page loads but not modify what's on the page. Any ideas? Thanks.
-
I'm trying to make a page where users can select a company which will be assigned to their purchase order. So they have a purchase order for lumber and one for metal. They choose lumber, then select which company is associated with that and that company's information with logo will show up when that purchase order is printed out. The question I have about the page is I'd like to have all the companies listed on the left and when you click the company, the right side changes to show the information already entered including contact information and logo. They can edit this information. What is the best way to load the information on the right side based on left side selection? Javascript? Ajax? Some PHP based system I'm unaware of? All the information will be coming from the database. Thanks
-
Use PHP to Populate HTML Form Drop Down Boxes With MySql Data
DeX replied to hobbiton73's topic in PHP Coding Help
I've coded this a few times but I'm not sure if there is a better way to do it. This is how I did it: Use PHP to pull all the values from the database and put them into 3 separate arrays, there are lots of tutorials around for this. Populate the first SELECT box with the proper values and attach an onchange function to it to call Javascript. Inside your Javascript, you'll grab the other SELECT box by its ID and inject the proper values into it. If I confused you, this might be a little too advanced for you. -
<div id="wrapper"> <div id="header"> <div id="headerwrapper"> <div id="nav"> </div> </div> </div> </div>
-
You need to understand that PHP script is executed by the server but Javascript is executed by the client browser. So it's like if I asked you to take an array from my computer and pass it to your computer, it doesn't work. However you could build the Javascript with PHP so the variables are passed to the client as was already suggested. You would actually echo out the code: <?php echo "<script language = 'Javascript1.1'>"; var var1 = " . $phpVariable . ";"; Do things with variable here echo "</script>"; ?>
-
Redirecting to Paypal.com is professional, I do it for all my client sites. Customers will probably feel more secure checking out on a paypal.com branded page rather than something custom on your domain. Plus, if you tunnel the Paypal API to your site, you're going to need SSL and a valid site certificate in order to secure it. You can't just drop in the code and that's it.