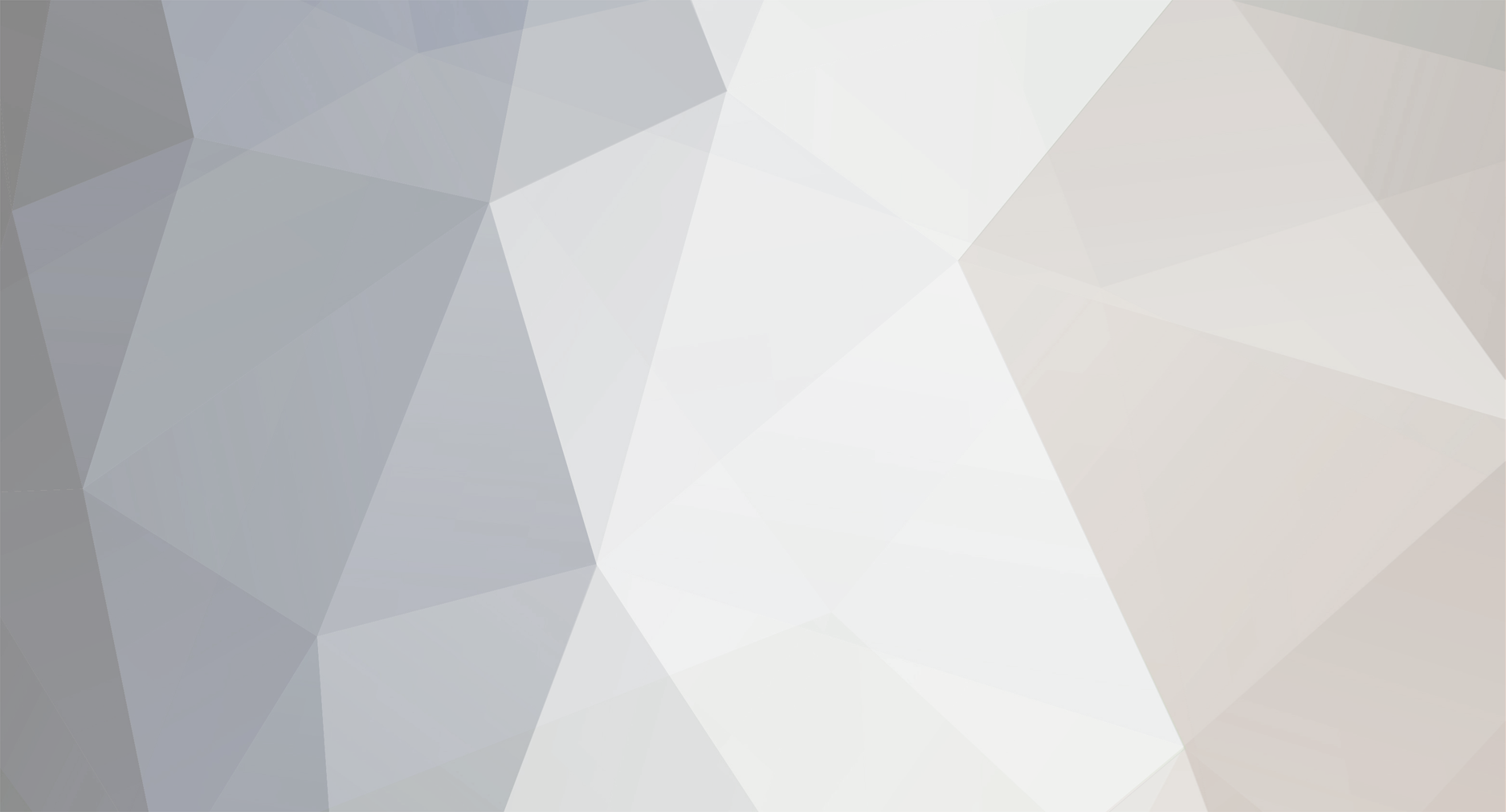
MDanz
-
Posts
728 -
Joined
-
Last visited
Posts posted by MDanz
-
-
I have a large array. Is there an alternate method to check if $value in the array is present in the MySql table vote and if not then insert $value into vote. This is what i am doing currently. Is there a better method?
foreach($rowids as $value) { $select = mysql_query("SELECT voteid FROM vote WHERE username='$username' AND voteid='$value' LIMIT 1",$this->connect); if(mysql_num_rows($select)==0) { $insert = mysql_query("INSERT INTO vote VALUES ('','$value','$username')",$this->connect); } }
-
Here's some of my code. The fault is when AJAX sends the dataString to sendpm.php, it should call the function. But nothing is happening. If i put the sendprivatemessage query in sendpm.php page, the data is sent to mysql. What is the solution, so i don't have to put the query on sendpm.php?
jquery - some of it
$.ajax({ type: "POST", url: "/sendpm.php", data: dataString, success: function() { window.location.href = 'http://www.stackway.com/cp/messages=outbox'; } });
sendpm.php
include "database.php"; $to = $_POST['to']; $from = $_POST['from']; $subject = $_POST['subject']; $body = $_POST['body']; $database2 = new Database(); $database2->opendb(); $database2->sendprivatemessage($to,$from,$subject,$body); $database2->closedb();
sendprivatemessage function
function sendprivatemessage($to,$from,$subject,$body) { $insert = mysql_query("INSERT INTO privatemessage VALUES ('','$subject','$body','$to','$from','1',NOW(),'','','')",$this-connect); }
-
nvm ..use plus instead of array merge
-
i want to preserve the numeric keys. When i print the array this is the result
Array ( [0] => one [1] => two [2] => three )
it should be
Array ( [0] => two [1] => one [2] => three )
$test1[2] = "one"; $test2[1] = "two"; $test2[3] = "three"; $test = array_merge($test1,$test2); print_r($test);
How do i solve this?
-
i get this error Warning: current() [function.current]: Passed variable is not an array or object..
for this
$lastblock = current( ${"s".$row} ); print_r($lastblock);
when i change to this it works..
$lastblock = current( $s0 ); print_r($lastblock);
The problem is i won't know the $row seeing as it is in a while loop. Solution?
-
this if statement isn't working..
$newid is an integer, which is the name of a variable of an array e.g. $53. The below code should check if the array exist. it should echo yes.. instead it is blank.
if(isset(${"$newid"})) { echo "yes"; //code }
-
while($row=mysql_fetch_assoc($query)) { $id = $row['id']; $col1 = $row['name1']; $col2= $row['name2']; $col3= $row['name3']; ${"$id"} = array("$col1","$col2","$col3"); ${"$s".$i}[] = ${"$id"}; }
This is just a brief example of what i'm trying to accomplish,$i is incremented somewhere else. I'm trying to implode the arrays in the array. So below i have imploded the main array but how do i implode the other arrays?
for($i=0;$i<11;$i++) { $array = ${"s" . $i}; $outcomes = implode("",$array); //implodes main array }
-
$logged = $_SESSION['logged']; $construct = "SELECT child.* FROM products child LEFT JOIN products parent on parent.sid=child.sid WHERE parent.id = (SELECT productid FROM subscribed WHERE username='".$logged."') AND parent.keyword != child.name ORDER BY child.id DESC";
I'm having trouble getting the subquery to work i get the wrong results. The parent query is fine because i have used it before. This is my first time using a sub query so bare with me.
I want the sub query to get all productids from the table 'subscribed' where username=$logged.
This is what i want but in one query, check WHERE clause for differences.
$construct = "SELECT child.* FROM products child LEFT JOIN products parent on parent.sid=child.sid WHERE parent.id= 'SUB QUERY RESULT 1' AND parent.keyword != child.name ORDER BY child.id DESC"; $construct = "SELECT child.* FROM products child LEFT JOIN products parent on parent.sid=child.sid WHERE parent.id= 'SUB QUERY RESULT 2' AND parent.keyword != child.name ORDER BY child.id DESC"; $construct = "SELECT child.* FROM products child LEFT JOIN products parent on parent.sid=child.sid WHERE parent.id= 'SUB QUERY RESULT 3' AND parent.keyword != child.name ORDER BY child.id DESC";
How do I do this in one query without looping the query. Do I even need a subquery?
-
i tried that and now it is removing peter from results. So the results are carl, john and david.
SELECT child.* FROM mytable child LEFT JOIN mytable parent on parent.sid=child.sid WHERE parent.name LIKE '%peter%' AND parent.name != child.name ORDER BY child.id ASC
-
I'm doing a left join query for the table below and it is not getting the correct results. It should return the results peter, john and david because they have the same sid and the wildcard is peter.
Instead it is returning carl, peter, john and david. What do i have to change to correct this?
SELECT child.* FROM mytable child LEFT JOIN mytable parent on parent.sid=child.sid WHERE parent.name LIKE '%peter%' ORDER BY child.id ASC
[td]1
0rickmike -
nevermind , i used an left join
-
I want a query where searching for 'dave', will display the top three rows. 'mike' and 'carl' both have the parentid of dave. So the three rows displayed will be the top three.
+----+----------+------------+ | id | parentid| user +----+----------+------------+ | 1 | null | dave | 2 | 1 | mike | 3 | 1 | carl | 4 | 2 | rick | 5 | 4 | mike
What type of query will accomplish this? A nested query?
-
By calling function two will it break the loop?; or do i have to echo what is returned?
functione one() { $getids = mysql_query("SELECT * FROM table"); while($row=mysql_fetch_assoc($getids); { $this->two(); } } function two() { return 'break;'; }
-
nope nothing wrong in firebug.. AJAX only works again when i reload the page.
-
AJAX only works once, i have to reload the page for it to work again. How do i fix this?
echo "<div class='unsubscribe'><a id='us$id' href='javascript:unsubscribe($id);'><img src='/unsubscribe.jpg' alt='unsubscribe' /></a></div>";
function unsubscribe(number) { if (window.XMLHttpRequest) {// code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp=new XMLHttpRequest(); } else {// code for IE6, IE5 xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange=function() { if (xmlhttp.readyState==4 && xmlhttp.status==200) { document.getElementById("us"+number).innerHTML="<img src='/subscribe.jpg' alt='subscribe' />"; } } xmlhttp.open("GET","/unsubscribe.php?id="+number,true); xmlhttp.send(); }
-
so i should never use JavaScript with AJAX? always jQuery and AJAX? I was following a tutorial, is their a specific reason?
-
i've simplified it.
This should be simple. I click on the subscribe.jpg image and the image changes to unsubscribe.jpg. and then subscribe.php is called. But nothing is happening when i click the subscribe image??
echo "<a id='s1' href='javascript:subscribe(1)'><img src='/subscribe.jpg' alt='subscribe' /></a>";
function subscribe(number) { if (window.XMLHttpRequest) {// code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp=new XMLHttpRequest(); } else {// code for IE6, IE5 xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange=function() { if (xmlhttp.readyState==4 && xmlhttp.status==200) { document.getElementById("s"+number).innerHTML="<img src='/unsubscribe.jpg' alt='unsubscribe' />"; } } xmlhttp.open("GET","subscribe.php?id="+number,true); xmlhttp.send(); }
-
where did i go wrong? It should update mysql(insertsuscribe function) and change the image in the anchor tag. This is my first time doing AJAX, what did i do wrong?
php
$id= $row['id']; echo "<div class='suscribe'><a id='s$id' href='javascript:suscribe($id);'><img src='/suscribe.jpg' alt='suscribe' /></a></div>";
ajax
function suscribe(number) { if (window.XMLHttpRequest) {// code for IE7+, Firefox, Chrome, Opera, Safari xmlhttp=new XMLHttpRequest(); } else {// code for IE6, IE5 xmlhttp=new ActiveXObject("Microsoft.XMLHTTP"); } xmlhttp.onreadystatechange=function() { if (xmlhttp.readyState==4 && xmlhttp.status==200) { document.getElementById("s"+number).innerHTML="<img src='/unsuscribe.jpg' alt='unsuscribe' />"; } } xmlhttp.open("GET","suscribe.php?id="+number,true); xmlhttp.send(); }
suscribe.php
<?php session_start(); include "database.php"; $id = $_GET['id']; $database = new Database(); $database->opendb(); $database->insertsuscribe($id); $database->closedb(); ?>
-
ok i added a condition but it still doesn't work. Can you help me correct the code below? You can just copy and paste and try it yourself. onmouseover the red boxes appear. If i click(X) the red box should close but it doesn't. Only doubleclicking (X) closes the red box. Onmouseover it should always display the red box though.
<html xmlns="http://www.w3.org/1999/xhtml"> <head> </head> <style type="text/css"> .container { display:block; width:500px; height:200px; border:1px solid green; } .advert { float:right; overflow:hidden; width:100px; height:30px; border:1px solid red; } .close { float:right; width:20px; height:28px; cursor:pointer; border:1px solid black; } </style> <body> <div class="container" onmouseover='getad(39);' onmouseout='hidead(39);changeback(39);'> <div class='advert' id="39" style="display:none;"><div class="close"><a href="javascript:closead(39)">X</a></div></div> <input type="text" value="1" id="ad39" /> </div> <div class="container" onmouseover='getad(40);' onmouseout='hidead(40);changeback(40);'> <div class='advert' id="40" style="display:none;"><div class="close"><a href="javascript:closead(40)">X</a></div></div> <input type="text" value="1" id="ad40" /> </div> <script type="text/javascript"> function getad(number) { if(document.getElementById('ad'+number).value==1) { if(document.getElementById(number).style.display == "none") { document.getElementById(number).style.display = "block"; } } } function hidead(number) { if(document.getElementById('ad'+number).value==1) { if(document.getElementById(number).style.display == "block") { document.getElementById(number).style.display = "none"; } } } function closead(number) { document.getElementById('ad'+number).value = 0; if(document.getElementById(number).style.display == "block") { document.getElementById(number).style.display = "none"; } } function changeback(number) { if(document.getElementById('ad'+number).value==0) { document.getElementById('ad'+number).value = 1; } } </script> </body> </html>
-
onmouseover = null disables onmousever.. How do i renable it again? I tried not null but it didn't enable onmouseover. I didn't include the functions btw.
document.getElementById('row'+number).onmouseover =null; document.getElementById('row'+number).onmouseover !=null;
When i try disabled=true or false it doesn't disable onmouseover, so the below didn't work.
document.getElementById('row'+number).onmouseover.disabled =true; document.getElementById('row'+number).onmouseover.disabled =false;
-
This is a function for a slideshow,onmouseover i want it to stop. Instead of stopping the slideshow onmouseover, it speeds up?? How can i correct this to stop onmouseover?
<body onload="nextslide();">
function nextslide() { // Hide current slide var object = document.getElementById('slide' + current); //e.g. slide1 object.style.display = 'none'; // Show next slide, if last, loop back to front if (current == last) { current = 1; } else { current++ } object = document.getElementById('slide' + current); object.style.display = 'block'; var timeout = setTimeout(nextslide, 2500); object.onmouseover = function(){ clearTimeout( timeout ) }; object.onmouseout = nextslide; }
-
<form method='POST' action='http://www.example.com/submit' name='know'><input type='hidden' name='id' value='$id' /><a href='#' onclick='document.know.submit()'>test</a></form>
when i click "test" it should go to the submit page but nothing happens.
-
this is frustrating...
i changed the code to this to test. Everytime it goes to the next slide the alert appears but i haven't clicked on anything????
function nextslide() { // Hide current slide object = document.getElementById('slide' + current); //e.g. slide1 object.style.display = 'none'; // Show next slide, if last, loop back to front if (current == last) { current = 1; } else { current++ } object = document.getElementById('slide' + current); object.style.display = 'block'; var next = setTimeout(nextslide, 2500); object.onclick = alert("hello"); object.onmouseout = next; }
-
thanks i tried that code and the slideshow stopped working?
Alternative to foreach Mysql help
in MySQL Help
Posted
blank is the auto incremented primary key id of the mysql table vote.