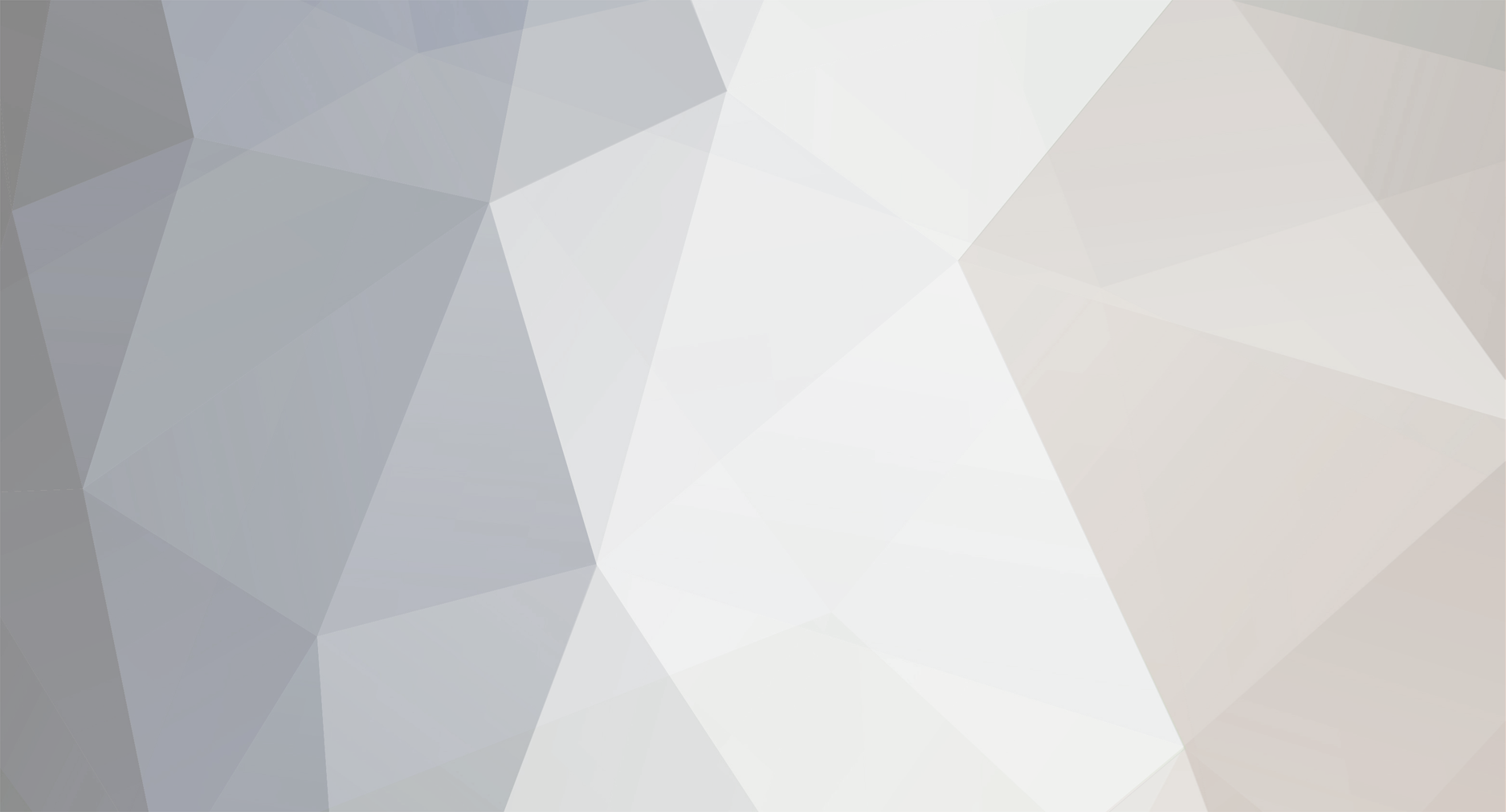
eugene2009
Members-
Posts
109 -
Joined
-
Last visited
Never
Everything posted by eugene2009
-
Yes, thats using the GET method.
-
So im building a search form.. everything is up and running.. and it works perfectly. i just have one quick question. in my mysql database, i have a photo named bmw... and the description for it is "this is a black bmw 325i" for example.. when i search "black bmw" or "bmw 325i" the results show.. but what if i wanted to search "black 325i"? how would i be able to do that? heres me code if (isset($_GET['search'])) { $searchTerms = trim($_GET['search']); $searchTerms = strip_tags($searchTerms); // remove any html/javascript. if (strlen($searchTerms) < 3) { $error[] = "Search terms must be longer than 3 characters."; }else { $searchTermDB = mysql_real_escape_string($searchTerms); // prevent sql injection. } // If there are no errors, lets get the search going. if (count($error) < 1) { $searchSQL = "SELECT id, name, description, category FROM cars WHERE "; // grab the search types. $types = array(); $types[] = isset($_GET['name'])?"`name` LIKE '%{$searchTermDB}%'":''; $types[] = isset($_GET['description'])?"`description` LIKE '%{$searchTermDB}%'":''; $types[] = isset($_GET['category'])?"`category` LIKE '%{$searchTermDB}%'":''; $types = array_filter($types, "removeEmpty"); // removes any item that was empty (not checked) if (count($types) < 1) $types[] = "`name` LIKE '%{$searchTermDB}%'"; // use the body as a default search if none are checked $andOr = isset($_GET['matchall'])?'AND':'OR'; $searchSQL .= implode(" {$andOr} ", $types) . " ORDER BY `name`"; // order by title. $searchResult = mysql_query($searchSQL) or die("There was an error.<br/>" . mysql_error() . "<br />SQL Was: {$searchSQL}"); if (mysql_num_rows($searchResult) < 1) { $error[] = "The search term provided {$searchTerms} yielded no results."; }else { $results = array(); // the result array $i = 1; while ($row = mysql_fetch_assoc($searchResult)) { $results[] = "{$i}: {$row['name']}<br />{$row['description']}<br />{$row['category']}<br /><br />"; $i++; } } } } function removeEmpty($var) { return (!empty($var)); }
-
Google xampp, it's a Free open source server with php and mysql support
-
dont mind the page id.. just say theres 1 picture per page and i want that 5 stars linked with the id of the picture.. how exactly am I going to plug this function into my existing code? im having trouble getting it to work with the code.. please help me and yes i have the tracking information set up..
-
Anyone... Any suggestions ?????
-
The only thing I need help with is how to make individual id's for each page.thank u
-
Hello, so im following this tutorial.. My tables are created and everything works great!! But my problem is.. i want this rating script to work in my gallery for every individual photo.. every picture is /picture.php?id=23 , or id=2, etc.. anyways.. Ive been trying to do a lot of different things where it will save to the database under different id's so every page has its own rating count.. somebody please help me. here are the 2 pages that need to be edited.. thanks in advance.. //_drawrating.php <?php function rating_bar($id,$units='',$static='') { require('_config-rating.php'); // get the db connection info //set some variables $ip = $_SERVER['REMOTE_ADDR']; if (!$units) {$units = 10;} if (!$static) {$static = FALSE;} // get votes, values, ips for the current rating bar $query=mysql_query("SELECT total_votes, total_value, used_ips FROM $rating_dbname.$rating_tableName WHERE id='$id' ")or die(" Error: ".mysql_error()); // insert the id in the DB if it doesn't exist already // see: http://www.masugadesign.com/the-lab/scripts/unobtrusive-ajax-star-rating-bar/#comment-121 if (mysql_num_rows($query) == 0) { $sql = "INSERT INTO $rating_dbname.$rating_tableName (`id`,`total_votes`, `total_value`, `used_ips`) VALUES ('$id', '0', '0', '')"; $result = mysql_query($sql); } $numbers=mysql_fetch_assoc($query); if ($numbers['total_votes'] < 1) { $count = 0; } else { $count=$numbers['total_votes']; //how many votes total } $current_rating=$numbers['total_value']; //total number of rating added together and stored $tense=($count==1) ? "vote" : "votes"; //plural form votes/vote // determine whether the user has voted, so we know how to draw the ul/li $voted=mysql_num_rows(mysql_query("SELECT used_ips FROM $rating_dbname.$rating_tableName WHERE used_ips LIKE '%".$ip."%' AND id='".$id."' ")); // now draw the rating bar $rating_width = @number_format($current_rating/$count,2)*$rating_unitwidth; $rating1 = @number_format($current_rating/$count,1); $rating2 = @number_format($current_rating/$count,2); if ($static == 'static') { $static_rater = array(); $static_rater[] .= "\n".'<div class="ratingblock">'; $static_rater[] .= '<div id="unit_long'.$id.'">'; $static_rater[] .= '<ul id="unit_ul'.$id.'" class="unit-rating" style="width:'.$rating_unitwidth*$units.'px;">'; $static_rater[] .= '<li class="current-rating" style="width:'.$rating_width.'px;">Currently '.$rating2.'/'.$units.'</li>'; $static_rater[] .= '</ul>'; $static_rater[] .= '<p class="static">'.$id.'. Rating: <strong> '.$rating1.'</strong>/'.$units.' ('.$count.' '.$tense.' cast) <em>This is \'static\'.</em></p>'; $static_rater[] .= '</div>'; $static_rater[] .= '</div>'."\n\n"; return join("\n", $static_rater); } else { $rater =''; $rater.='<div class="ratingblock">'; $rater.='<div id="unit_long'.$id.'">'; $rater.=' <ul id="unit_ul'.$id.'" class="unit-rating" style="width:'.$rating_unitwidth*$units.'px;">'; $rater.=' <li class="current-rating" style="width:'.$rating_width.'px;">Currently '.$rating2.'/'.$units.'</li>'; for ($ncount = 1; $ncount <= $units; $ncount++) { // loop from 1 to the number of units if(!$voted) { // if the user hasn't yet voted, draw the voting stars $rater.='<li><a href="db.php?j='.$ncount.'&q='.$id.'&t='.$ip.'&c='.$units.'" title="'.$ncount.' out of '.$units.'" class="r'.$ncount.'-unit rater" rel="nofollow">'.$ncount.'</a></li>'; } } $ncount=0; // resets the count $rater.=' </ul>'; $rater.=' <p'; if($voted){ $rater.=' class="voted"'; } $rater.='>'.$id.' Rating: <strong> '.$rating1.'</strong>/'.$units.' ('.$count.' '.$tense.' cast)'; $rater.=' </p>'; $rater.='</div>'; $rater.='</div>'; return $rater; } } ?> and heres the page where to display.. //test.php <?php require('_drawrating.php');?> <head> <title>Multiple Ajax Star Rating Bars</title> <script type="text/javascript" language="javascript" src="js/behavior.js"></script> <script type="text/javascript" language="javascript" src="js/rating.js"></script> <link rel="stylesheet" type="text/css" href="css/default.css" /> <link rel="stylesheet" type="text/css" href="css/rating.css" /> </head> <body> <?php echo rating_bar('2id',5); ?> </body> </html> Can somebody please help me? THanks
-
isnt that a software? .. i want it to automatically be generated on my page for a small preview.. where the user can click on that to see the actual live page..
-
Is it possible to generate thumbnails (.gif) for html pages with php? If so, how? Thank you.
-
It displays the same category over and over.. when i only need it to show once.. It gets it from every row.. I need to know how to set up the mysql table the right way..
-
greatt.. just like i said
-
thats probably because you are not having the images sent also.. try putting those pictures on a server and just including the whole url e.g. <img src="http://www.your_domain.com/side.gif">
-
Hello, I have this site that displays different galleries of cars.. On there, I have an admin page where it allows the administrators to upload different pictures of different cars. So heres my problem.. just to start browsing the viewer would have to go to something called.. categories.php Here I would like all the different types of makes displayed that are hyperlinked to the next page on where to select a model... So say my admin wants to add a new photo to a category that doesnt exist.. he selects lamborghini for example and uploads the pic into that category.. that way in categories.php it would automatically show up a new category because of the mysql fetch function. Something like this: $query = "SELECT * FROM cars ORDER BY makeid"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)){ echo "<a href=\"view_categories.php?id=' . $row['makeid'] . "\">' . $row['makeid'] . '</a>"; So pretty much where it will fetch all the categories and link them all on one page.. I have it all figured out but the way my mysql database is setup it displays the same link x number of pictures there are because i do not understand how to set it up correctly.. i googled it over 100 times and I cant find what im looking for.. somebody please help me..
-
i know how to connect to the database.. i got this.. $query = "SELECT * FROM comments ORDER BY tutorialid"; //get the customer list $result = mysql_query($query); //perform the query //display the customer list $array = array(); while($row = mysql_fetch_array($result)){ $array[] = $row; } $midPoint = ceil(count($array) / 2); echo "<table border=\"1\">"; for($i = 0; $i < $midPoint; $i++){ echo "<tr>"; echo '<td><a href="category.php?tutorialid='.$array[$i]['tutorialid'].'">'.$array[$i]['tutorialid'].'</a>'; echo "</td>"; if (isset($array[$i + $midPoint])) echo '<td><a href="category.php?tutorialid='.$array[$i+$midPoint]['tutorialid'].'">'.$array[$i+$midPoint]['tutorialid'].'</a></td'; echo "</td>"; echo "</tr>"; } My problem is it displays the same category over and over.. i want it to display it only once.. thats where im stuck at..
-
Lets start with this code.. CREATE TABLE category( category_id INT AUTO_INCREMENT PRIMARY KEY, name VARCHAR(20) NOT NULL, parent INT DEFAULT NULL); INSERT INTO category VALUES(1,'ELECTRONICS',NULL),(2,'TELEVISIONS',1),(3,'TUBE',2), (4,'LCD',2),(5,'PLASMA',2),(6,'PORTABLE ELECTRONICS',1), (7,'MP3 PLAYERS',6),(8,'FLASH',7), (9,'CD PLAYERS',6),(10,'2 WAY RADIOS',6); SELECT * FROM category ORDER BY category_id; So look.. i create this table.. but how do I actually create more inputs for items under that category for prices, and other information.. How will I be able to have a page named categories.php and have it list only the categories linked with the get method going to the products in each category.. somebody please help..
-
Awesome! it worked! How would I be able to paginate this so it will display 3 rows per page? Thank you
-
yea.. it doesnt work.. hmmm.. emopoops.. how would you plug yours in? sorry its just that i learn when i ask for help.
-
Sorry, I am very new to this.. i am having trouble on how to replace my array with this one.. may you please help? heres the code that is working right now.. $query = "SELECT * FROM comments ORDER BY date DESC"; //get the customer list $result = mysql_query($query); //perform the query //display the customer list while($row = mysql_fetch_array($result)){ echo '<a href="test1.php?id='.$row['commentid'].'">'.$row['name'].'</a><BR>'; //create a link with the customers id as the $_GET['id'] } Thank you so much for your help.
-
well heres the thing.. that was just an example code to make things easier.. I have an upload form where users upload pictures into the database and this page is the one that displays them you know? theres 10 results per page.. i have it paginated.. but i want it to display 2 pictures per row and then go to the next.. how is this possible..
-
is it possible to do it like this??? 1 6 2 7 3 8 4 9 5 10 instead of 1 2 3 4 5 6 etc...
-
Hello Heres my code: $query = "SELECT * FROM comments ORDER BY date DESC"; //get the customer list $result = mysql_query($query); //perform the query //display the customer list while($row = mysql_fetch_array($result)){ echo '<a href="test1.php?id='.$row['commentid'].'">'.$row['name'].'</a><BR>'; //create a link with the customers id as the $_GET['id'] } What if I want 2 names per line.. how do i make it fetch all the rows displaying them 2 per line still in descending order? Thank you for all your help.
-
What is the proper wat to retrieve the variable in the mysql_query? I am having tons of trouble getting this little code to work. Please help $id = $_GET['id']; $result = mysql_query("SELECT * FROM comments WHERE id ='$id' "); $row = mysql_fetch_assoc($result); echo 'The customers name is, '.$row['name'].' and he made a purchase on, '.$row['date'].'.<BR>' ; Please help me. Thank you
-
i ran into a problem... Just a quick narrowed down example.. $query = mysql_connect ($db_hostname, $db_username, $db_pass) or die ('I cannot connect to the database because: ' . mysql_error()); mysql_select_db ($db_name); $id = $_GET['id']; //gets the id from url $query = "SELECT * FROM comments WHERE id='$id'"; //select records with passed id $result = mysql_query($query); //perform the query $row = mysql_fetch_row($result); echo 'The customers name is, '.$row['name'].' and he made a purchase on, '.$row['date'].'.<BR>'; ?> What am I doing wrong?
-
i ran into a problem... Just a quick narrowed down example.. $query = mysql_connect ($db_hostname, $db_username, $db_pass) or die ('I cannot connect to the database because: ' . mysql_error()); mysql_select_db ($db_name); $id = $_GET['id']; //gets the id from url $query = "SELECT * FROM comments WHERE id='$id'"; //select records with passed id $result = mysql_query($query); //perform the query $row = mysql_fetch_row($result); echo 'The customers name is, '.$row['name'].' and he made a purchase on, '.$row['date'].'.<BR>'; ?> What am I doing wrong?