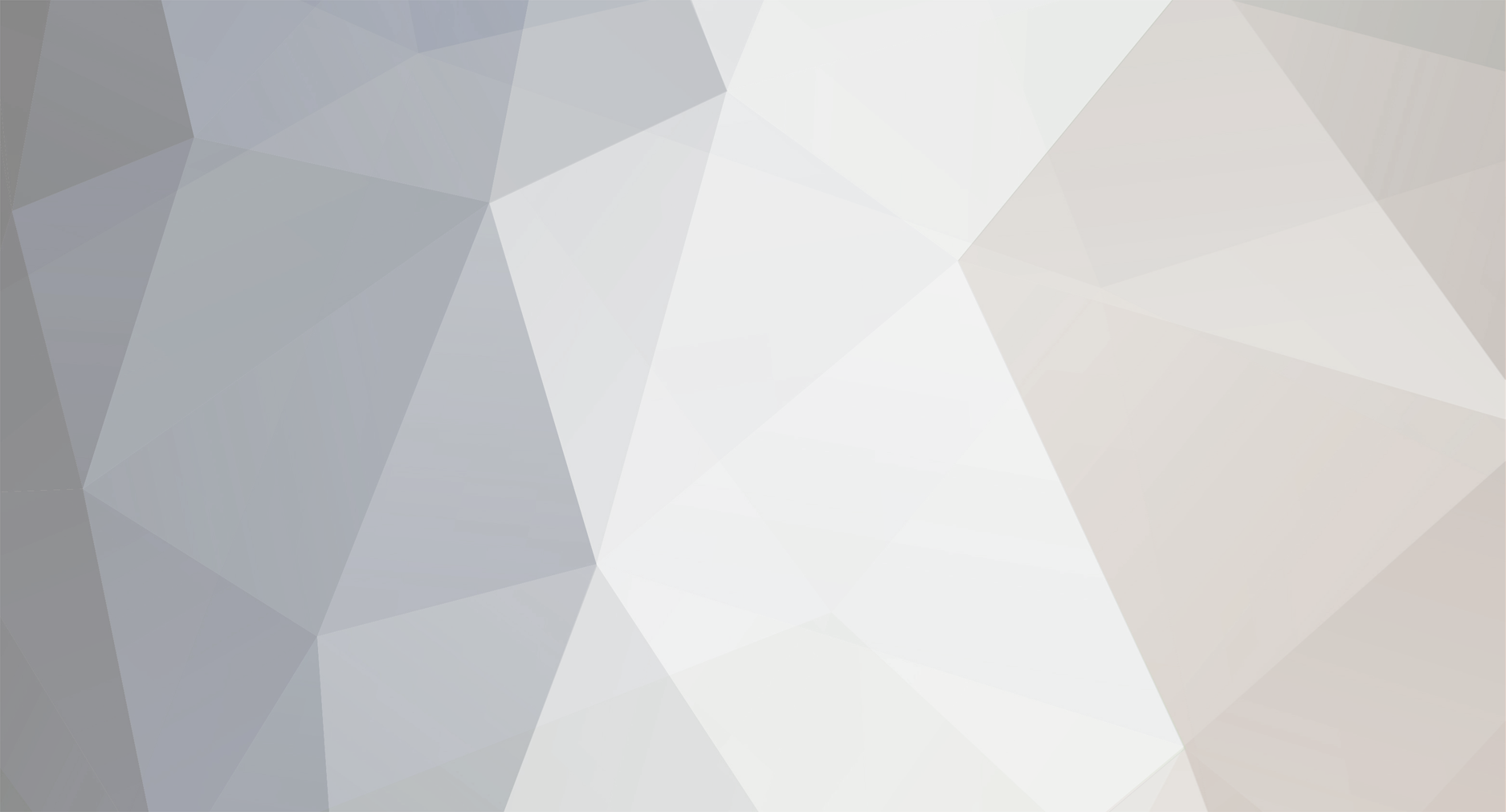
foucquet
Members-
Posts
48 -
Joined
-
Last visited
Everything posted by foucquet
-
Thanks, that's so obvious now that I see it. I was busy playing around with str functions and getting nowhere...
-
I am parsing an rss feed from my flickr photostream using this:- <?php $url = "http://api.flickr.com/services/feeds/photos_public.gne?id=49466419@N05&lang=en-us&format=rss_200"; $rss = simplexml_load_file($url); if($rss) { echo '<h1>'.$rss->channel->title.'</h1>'; echo '<li>'.$rss->channel->pubDate.'</li>'; $items = $rss->channel->item; foreach($items as $item) { $title = $item->title; $link = $item->link; $published_on = $item->pubDate; $description = $item->description; echo '<h3><a href="'.$link.'">'.$title.'</a></h3>'; echo '<span>('.$published_on.')</span>'; echo '<p>'.$description.'</p>'; } } ?> which gives me this as the description for each image:- public 'description' => string ' <p><a href="http://www.flickr.com/people/alfthomas/">Alf Thomas</a> posted a photo:</p> <p><a href="http://www.flickr.com/photos/alfthomas/14064465890/" title="harlaw_12"> <img src="http://farm6.staticflickr.com/5077/14064465890_83c02ecec6_m.jpg" width="240" height="110" alt="harlaw_12" /> </a> </p> <p>A view of Harlaw Reservoir.</p>' (length=338) What I actually want is the photo (linked back) without the "Alf Thomas posted a photo" bit, does anyone have any idea how I would go about cloning that bit out?
-
Of course! What a dummy, Iknew a fresh pair of eyes would help - thanks.
-
I am trying to put an rss feed from my Flickr account into my website I have got thus far with my class:- <?php // Flickr Class class flickr { // Properties var $feed; //=================== // Construct Flickr function __construct($feed) { $this->feed = $feed; } //=================== // Parse Method function parse() { $rss = simplexml_load_file($this->feed); $photodisp = array(); foreach ($rss->channel->item as $item) { $link = (string) $item->link; // Link to this photo $title = (string) $item->title; // Title of this photo $media = $item->children('http://search.yahoo.com/mrss/'); $thumb = $media->thumbnail->attributes(); $url = (string) $thumb['url']; // URL of the thumbnail $width = (string) $thumb['width']; // Width of the thumbnail $height = (string) $thumb['height']; // Height of the thumbnail $photodisp[] = <<<________________EOD {$title} ________________EOD; } return $photodisp; } //=================== // Display Method function display($numrows=6,$head="Photos on Flickr") { $photodisp = $this->parse(); $i = 0; $thumbs = <<<____________EOD $head ____________EOD; while ( $i < $numrows ) { $thumbs .= $photodisp[$i]; $i++; } $trim = str_replace('http://api.flickr.com/services/feeds/photos_public.gne?id=', '',$this->feed); $user = str_replace('〈=en-us&format=rss_200','',$trim); $thumbs .= <<<____________EOD View All Photos on Flickr ____________EOD; return $thumbs; } //=================== } //End of Class ?> // <- Line 66 and it gives me "Parse error: syntax error, unexpected end of file in C:\wamp\www\image-a-nation\test4_class.php on line 66". I thought it might be an unclosed brace/bracket or something like that, but no amount of checking finds anything - would appreciate a fresh pair of eyes...
-
Hmm, didn't spot that one - definitely an autopilot typo... Thanks
-
I am trying to iterate through an array of images and am having trouble with next/prev links. I thought that this should basically work as a starting point:- $images = array(); $img_loc = 'images/'; if ($handle = opendir($img_loc)) { while (false !== ($file = readdir($handle))) { if ($file != "." && $file != "..") { $images[] = $file; } } closedir($handle); } //>>> ==================================================================================== //>>> Block of code for Next/Prev links on images if (isset($_REQUEST['id'])) { $id = $_REQUEST['id']; } else { $id = 0; // Whatever the default starting value should be } $self = $_SERVER['PHP_SELF'] . "?$id="; $first = 0; // Because arrays are numbered from 0 $last = 7; $pic = $img_loc . $images[$id]; echo "<p><img src=\"$pic\"></p>"; if ($id < $last) { echo "<a href=".$self.($id + 1)."><h3>Next</h3></a>"; } if ($id > $first) { echo "<a href=".$self.($id - 1)."><h3>Previous</h3></a>"; } //=====================================================================================<<< //>>> ==================================================================================== //>>> Checking on what the variables are doing var_dump ($images); echo "File location = " . $pic . "<br>"; echo "First = " . $first . "<br>"; echo "Last = " . $last . "<br>"; echo "File id = " . $id . "<br>"; echo "Self = " . $self . "<br>"; echo "Server = " . $_SERVER['PHP_SELF'] . "<br>"; //=====================================================================================<<< and to an extent it does giving me:- IMAGE Next array (size = 8 ) 0 => string 'decisions.jpg' (length=13) 1 => string 'dual_concentration.jpg' (length=22) 2 => string 'generation_gap.jpg' (length=18) 3 => string 'just_looking.jpg' (length=16) 4 => string 'mid_stride.jpg' (length=14) 5 => string 'no7.jpg' (length=7) 6 => string 'plugged.jpg' (length=11) 7 => string 'runner.jpg' (length=10) File location = images/decisions.jpg First = 0 Last = 7 File id = 0 Self = /imageanation/next_prev_link_test.php?0= Server = /imageanation/next_prev_link_test.php However when I click next it changes the url from localhost/imageanation/next_prev_link_test.php to localhost/imageanation/next_prev_link_test.php?0=1 and reloads the same image. Hours of head scratching has not provided me with any solution, which I am sure will be quite simple if I could only see it.
-
OK, OK, I'm a complete dummy - didn't even spot those errors, too busy looking for everything but the bleedin' obvious! That indeed now works (hides head in shame!)
-
I am trying to bring a flickr feed into a webpage, and can't work out why this:- <?php // >>> Set up the variables needed <<< $photos = array(); $encode_params = array(); $maximgs = 19; // >>> This need to be 1 less than actual req'd No. as array counts from 0 <<< // >>> Set up the parameters for the API <<< $api_params = array( 'api_key' => 'my api key', 'method' => 'flickr.photos.search', 'user_id' => 'my id', 'format' => 'php_serial', ); //>>> Encode the parameters ready to make API request <<< foreach($api_params as $k => $v){ $encode_params[] = urlencode($k) . '=' . urlencode($v); } var_dump($encode_params); // >>> Construct API url and make the request <<< $api_url = 'http://api.flickr.com/services/rest/?' . implode('&', $encode_params); var_dump($api_url); // >>> Retrieve images <<< $rsp = file_get_contents($api_url); $rsp_obj = unserialize($rsp); echo $rsp_obj; var-dump($rsp_obj['photos']['photo']); //>>> Line 37 <<< ?> gives me "Parse error: syntax error, unexpected T_VAR in C:\wamp\www\php\flickr tests\prev3~.php on line 37".
-
What a dummy! Didn't spot the underscore, so bleedin' obvious when pointed out. That works perfectly MMDE thanks.
-
I am trying to pull images from my Flickr account with some success except that of accessing the description to be used as a caption for the image. What I have so far is:- <?php //>>> Define some variables $maximgs = 5; $images = array(); $params = array( 'api_key' => '***********************', 'method' => 'flickr.photos.search', 'user_id' => '****************', 'sort' => 'date-posted-desc', 'format' => 'php_serial', 'extras' => 'description', ); $encoded_params = array(); foreach ($params as $key => $value){ $encoded_params[] = urlencode($key) . '=' . urlencode($value); } //>>> Make request and decode the response $url = 'http://api.flickr.com/services/rest/?' . implode('&', $encoded_params); $response = file_get_contents($url); $response_object = unserialize($response); //var_dump($response_object['photos']['photo']); //>>> Why is this returning 100 results? if($response_object['stat'] !== 'ok'){ echo "Something went wrong!"; } //>>> Select the required number of images ($maximgs) for($loop = 0; $loop <= $maximgs; $loop++){ $images = $response_object['photos']['photo'][$loop]; var_dump($images); //>>> Url of the individual image location for the img src. $imgurl = 'http://farm'.$images['farm'].'.'.'static'.'.'.'flickr.com/'. $images['server'].'/'.$images['id'].'_'.$images['secret'].'_n.jpg'; //>>> Url of the individual image for the link. $flkrurl = 'http://www.flickr.com/photos/'.$images['owner'].'/'.$images['id'].'/'; /*The complete Url (<a href="link($flkrurl)"> <img alt="$images['title']" src="image location"($imgurl) width="required width of image" /> </a>) */ $compurl = '<a href="'.$flkrurl.'" target="_blank">'.'<img alt="'. $images['title'].'" src="'.$imgurl.'" width="320px" /></a>'; //>>> Display Images echo $compurl . '<br />' . $images['description']['content']; //<<<<<< Line 66 } ?> Which annoyingly throws this error:- Notice: Undefined index: content in C:\wamp\www\php\flickr_test1.php on line 66 The result of a var_dump():- array 'id' => string '6914498038' (length=10) 'owner' => string '************' (length=12) 'secret' => string '601a885f31' (length=10) 'server' => string '7137' (length=4) 'farm' => float 8 'title' => string 'newhaven_15' (length=11) 'ispublic' => int 1 'isfriend' => int 0 'isfamily' => int 0 'description' => array '_content' => string 'Great reflections found in Newhaven Harbour.' (length=44) with the info that I want to extract underlined. What I can't figure out is how to find out the name of the array below "description =>". I have been scratching my head for several hours now and am no nearer to working it out. Maybe a genius here will have the solution.
-
Well, this is truly weird! Out of sheer desperation I deleted the oldest post, making the second oldest post the oldest, and it seems to have solved the problem, why I haven't a clue. All I can assume is that there is some sort of problem with the image attached to that particular post - requires some investigation. I can't think why I didn't do this in the first place to test if it was a problem with the particular post or a programming problem. The next step is to post the same image as the newest post and see if it throws the same error, if it does then I know it is the image, but if it doesn't then I will be even more puzzled that at present... Update:- Curiouser and curiouser, just posted the problem image as the newest post and it is behaving exactly how I would expect - WTF!"
-
I had a quick look and everything seems to be right, I am beginning to suspect that it is because the versions of PHP are different, 5.3.4 on the laptop and 5.3.8 on the PC. Not even going to think about all ths for the next week or so, too busy with Xmas related stuff...
-
If it helps any I inserted - var_dump($photo, $photos, $result, $imgurl); below $imgurl = $result; - and it returned the following:- $photos= object(stdClass)[301] public 'ID' => int 71 public 'post_author' => string '1' (length=1) public 'post_date' => string '2011-12-09 08:25:38' (length=19) public 'post_date_gmt' => string '2011-12-09 08:25:38' (length=19) public 'post_content' => string '' (length=0) public 'post_title' => string 'loch-linnhe-at-port-appin' (length=25) public 'post_excerpt' => string 'Loch Linnhe at Port Appin' (length=25) public 'post_status' => string 'inherit' (length=7) public 'comment_status' => string 'open' (length=4) public 'ping_status' => string 'open' (length=4) public 'post_password' => string '' (length=0) public 'post_name' => string 'loch-linnhe-at-port-appin' (length=25) public 'to_ping' => string '' (length=0) public 'pinged' => string '' (length=0) public 'post_modified' => string '2011-12-09 08:25:38' (length=19) public 'post_modified_gmt' => string '2011-12-09 08:25:38' (length=19) public 'post_content_filtered' => string '' (length=0) public 'post_parent' => int 78 public 'guid' => string 'http://localhost/wordpress/wp-content/uploads/2011/12/loch-linnhe-at-port-appin.jpg' (length=83) public 'menu_order' => int 0 public 'post_type' => string 'attachment' (length=10) public 'post_mime_type' => string 'image/jpeg' (length=10) public 'comment_count' => string '0' (length=1) public 'filter' => string 'raw' (length=3) $photo= array empty $result= string 'http://localhost/wordpress/wp-content/uploads/2011/12/loch-linnhe-at-port-appin.jpg' (length=83) $imgurl= string 'http://localhost/wordpress/wp-content/uploads/2011/12/loch-linnhe-at-port-appin.jpg' (length=83) However on hitting the last image the return was the following:- $photos= null $photos= array empty $result= null $imgurl= null everything empty.
-
Aye, I use both echo and var_dump() when debugging, but neither of these tell me why this works on my laptop setup but not on the PC setup, surely if it works on one it should work on the other. I agree that this is the case with the PC, but it is not on my laptop, and I can't figure out why the PC set up is throwing this error. To make it just a little clearer, this works to pick up the exif data from each sucessive image, working Previous/Next, which is fine as long as I do not go back to the first (oldest) image; when it hits the oldest post it throws the error, at least on my PC setup. Surely if it works on one then it should, in theory, work on both :-\
-
OK, I am developing a Wordpress theme, not getting much response fromt their support, so I thought I would ask here especially as is does not seem to be a pure Wordpress problem. Can anyone explain why this:- $size = "full"; $photos = get_children( array( 'post_parent' => $post->ID, 'post_status' => 'inherit', 'post_type' => 'attachment', 'post_mime_type' => 'image', 'order' => 'ASC', 'orderby' => 'menu_order ID') ); if ($photos) { $photo = array_shift($photos); $result = wp_get_attachment_url($photo->ID, $size); } $imgurl = $result; $exif = exif_read_data($imgurl, 'EXIF'); //<<<<<< Line 67 $exifdata = array(); format_exif_data($exif); should work on my laptop, but give me a "Warning: exif_read_data() [function.exif-read-data]: Filename cannot be empty in C:\wamp\www\wordpress\wp-content\themes\image-a-nation\index.php on line 67" on my PC? I have the same version of WAMP installed on both, so this strikes me a just a little weird, and much head scratching over the weekend has not shed any light at all. Hopefully someone can save me from insanity...
-
Thanks for all of the suggestions, in the end I used the PHP exif_read_data() function (don't know why I didn't think of it in the first place ) rather than the inbuilt Wordpress function, the PHP function actually returns the exif data as wanted except that one then has to split the DateTime into two variables rather than the one returned, but that's simple
-
When pulling exif data from an image I have pretty much everythiung nailed down except for the Shutter Speed value. When pulled from the image this is returned as:- 'Shutter' => string '0.0015625' Now I know that the actual shutter speed at the time of shooting was 1/640 sec; does anyone have any ideas about how one would convert... 'Shutter' => string '0.0015625' to 1/640 sec... I have been scratching my head over this problem for a few days now and am stiull no wiser
-
I think you hit the nail on the head there M, I also have age agaisnt me old dog, new tricks etc. :-\
-
Thanks again for the help mjdamato. I agree with you to a point, which is why I tend to 'echo' everything along the way to ensure that what I want to happen is happening, but I know from long experience as an artist that even the best plans are sometimes impossible to implement, and that one has to work around one's limtations to get a framework which one can then work on to improve. Given that I am not writing all this for any other reason than keeping a very old brain active maybe a few 'hidden bugs' would be good, as it would make this very old brain of mine work harder to sort them out. I don't advocate anyhting other than working to best principles in all things, but sometimes best principles are not quite as obvious as they should be - but that is a whole other debate...
-
I knew it was something simple - I had just not seen that second insertion... I did say that it was no pretty, but cmon guys we all have to learn somewhere along the line... Thanks for pointing out the bleedin' obvious Btw.
-
As part of an image gallery I am trying to INSERT data into a table to make accessible the relevant info of individual images - the problem I have encountered is that each query seems to be being inserted twice, and I can't figure out why. The code = <?php /*============================================== Connect to MySQL and select the correct database ==============================================*/ mysql_connect("localhost", "uname", "pwd") or die(mysql_error()); echo "Connected to MySQL<br />"; mysql_select_db("gallery_db") or die(mysql_error()); echo "Connected to Database gallery_db.<br />"; /*============================================= Include the files needed to undertake this task =============================================*/ include("functions.php"); /*========================= Set up arrays and variables =========================*/ $store = array(); $exif = array(); $folder = "./images/"; $insrt = 'INSERT INTO images VALUES (img_id, '; $file_name = ''; $file_location =''; $copyright =''; $caption = ''; $file_date = ''; $file_time = ''; $camera = ''; $speed = ''; $fNo = ''; $ISO = ''; /*================================= Open folder and read relevant files =================================*/ if ($handle = opendir('./images')) { while (false !== ($file = readdir($handle))) { if ($file != "." && $file != "..") { $file_name = $file; //>>> File Name <<< $file_location = $folder . $file; //>>> File Location <<< $exif = exif_read_data($file_location, 'EXIF'); //>>> Read exif data <<< /*============================================================== Check if the 'Copyright' info existe in the exif data, and if it doesn't set the $copyright variable to the relevant value. ==============================================================*/ if (array_key_exists('Copyright', $exif)) { $copyright = $exif['Copyright']; } else { $copyright = "copy name"; } $caption = $exif['COMMENT'][0]; //>>> Get the Caption <<< //>>> Re-format DateTime into separate date and time var's $file_date & $file_time <<< $temp = substr($exif['DateTime'], 0, 10); $file_date = substr($temp, 8, 2) . substr($temp, 4, 4) . substr($temp, 0, 4); $file_time = substr($exif['DateTime'], 11, ; //>>> Set the rest of the variables <<< $camera = $exif['Model']; //>>> Get the camera make and model <<< $speed = $exif['ExposureTime']; //>>> Get the shutter speed <<< $fNo = $exif['COMPUTED']['ApertureFNumber']; //>>> Get the f No. <<< $ISO = $exif['ISOSpeedRatings']; //>>> Get the ISO rating <<< //>>> Fill the $store[] array with the relevant bits for each seperate query <<< $store[] = $insrt . "'" . $file_name . "', " . "'" . $file_location . "', " . "'" . $copyright . "', " . "'" . $caption . "', " . "'" . $file_date . "', " . "'" . $file_time . "', " . "'" . $camera . "', " . "'" . $speed . "', " . "'" . $fNo . "', " . "'" . $ISO . "')"; } } closedir($handle); } /*============================================================================= Get individual queries from $store[] and INSERT INTO images the relevant VALUES =============================================================================*/ $num_queries = count($store); //>>> Check the number of queries in the array $store $loop = 0; //>>> Starting point for query No. bearing in mind that array keys are numbered from zero while ($loop <= $num_queries - 1) { $query = $store[$loop]; if (!mysql_query($query)){ die('Error: ' . mysql_error()); } mysql_query($query); $loop++; } ?> I know it's not pretty, but that can be attended to once working properly. I am quite sure that it will be a very simple solution, probably so simple that I haven't even thought of it.
-
I am trying to create a gallery page, one photo on the page with Next/Previous links either above or below but am having a little local difficulty. This is what I have:- //>>> Set up arrays and variables <<< $piccies = array(); $exif = array(); $exifdata = array(); $pic = 0; $img =''; $date = ''; $time = ''; $caption = ''; $copyright =''; $imageid = 1; $first = 1; $last = 5; $self = $_SERVER['PHP_SELF'] . "?imageid="; //>>> Read images from folder and assign to the array $piccies[] <<< $piccies = read_folder(); //>>> Find image and extract the Exif info <<< $exif = read_exif_info($piccies, $pic); //>> Populate with required info from $exifdata with required info from $exif $exifdata = populate_exif_data($exif, $date, $time); $img = substr_replace($img, "", 0, 2); ?> <div id="pict"> <img src="<?php echo $img; ?>" /> <?php echo "<p>" . $caption . "</p>"; if ($imageid < $last) { echo "<a href=" . $self . ($imageid + 1) . "><h2>Next</h2></a>"; } if ($imageid > $first) { echo "<a href=" . $self . ($imageid - 1) . "><h2>Previous</h2></a>"; } ?> </div> It puts the 'Next' link in fine, but when clicked the link goes to the Parent directory:- Index of /php/gallery [iCO] Name Last modified Size Description [DIR] Parent Directory - [ ] functions.php 27-Oct-2011 09:25 2.1K [ ] gallery index.php 27-Oct-2011 09:45 1.8K [DIR] images/ 26-Oct-2011 16:00 - and when I look at the source I see that the link has not been constructed as I expected. This is the source:- <div id="pict"> <img src="images/blue sky.jpg" /> <p>Blue Sky over Auld Reekie</p><a href=/php/gallery test.php?imageid=2><h2>Next</h2></a></div> where '/php/gallery test.php' is showing I expected 'images/filename.jpg' etc. and I just can't figureout what I have done wrong...
-
OK, that worked, thanks. Although it doesn't quite give the ouptput I want, it comes out like this:- This image is the copyright of Alf Thomas and may not be used without prior permission. Camera - Canon PowerShot G9 Shutter - 1/800 fNo - f/5.6 ISO - 200 Date - 07:10:2011 Time - 09:05:26 but I can play around with that and force it to comply with my needs in some way, and get the firsy line to wrap, and get an extra tab in the fNo and ISO lines...
-
I am trying to line up info using '\t', but it doesn't seem to be working at all. I have also tried several other escaped characters and none of them work either, any ideas? Just to make sure I have got it right this is what I have done. echo "This image is the copyright of " . $copyright . " and may not be used without prior permission.<br /><br />"; while( $field = each( $exifdata ) ) { echo $field[ 'key' ]; echo " - \t"; //>>> Tab across to line everything up... echo $field[ 'value' ]; echo '<br />'; }
-
I just knew I had done summat stupid - so bleeding obvious! Thanks Buddski