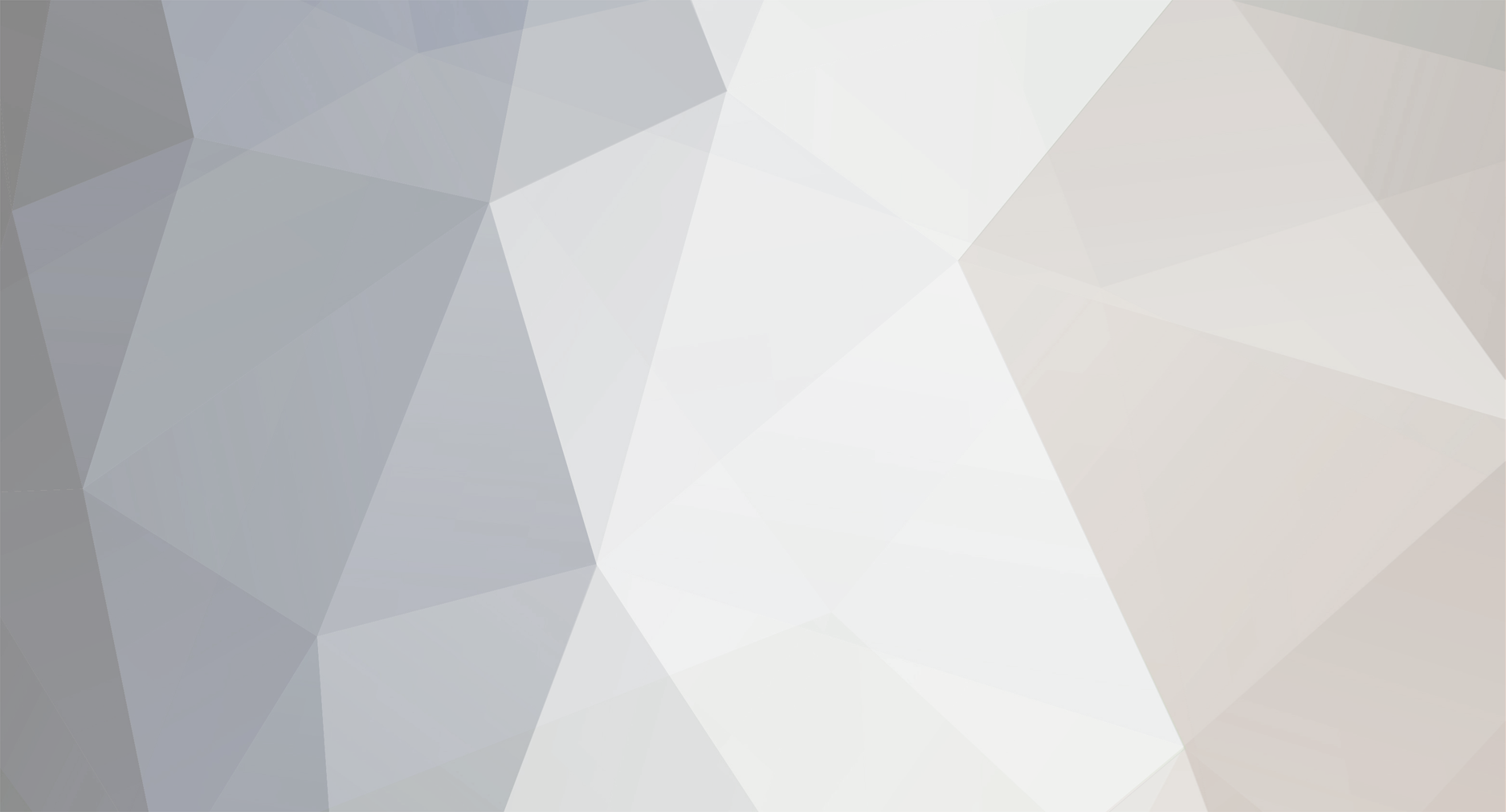
ChemicalBliss
Members-
Posts
719 -
Joined
-
Last visited
Never
Everything posted by ChemicalBliss
-
Common Practice to Make Avatar and Nickname Permanently Visible?
ChemicalBliss replied to chaseman's topic in PHP Coding Help
Well now that's much more clear . Ok so for such a basic script you would move the mysql portion to each page, or make it a function that each page has accses to (via an include perhaps). This is much easier imo with objects but to keep it simple, I would make a file named mysql.func.php, this will hold the functions to connect/query and any other specific code you dont want repeated, like a select statement for your contributions table. Then each page, at the start will "include('mysql.func.php');", Then you can use any mysql on any page as long as it includes all the mysql functions from this file. You could also make the mysql.func.php auto-connect when it's included (basically a file that connects to mysql and provides some functions that do some erpeated tasks). hope this helps -
Common Practice to Make Avatar and Nickname Permanently Visible?
ChemicalBliss replied to chaseman's topic in PHP Coding Help
From your post here is what I think I understand: You have a page on which you only display Avatars with nicknames of the members that have logged in. This is not possible with sessions as each session is unique to each user so you will only ever see your own avatar. If you meant: Geenrally showing avatars of members on any page at any time, it depends on where the avatar data is stored, the url or the image path, and the nickname, if it's in a database it should be very simple, and even if not - storing the data in a file is easy too but tbh i don't know exactly what you're asking. Is this for some sort of message board or something? -
use microtime() - it's a built-in function of PHP that gives you the number of seconds + milleseconds since the UNIX Epoch (1st January 1970) according to the server time. you can use time() for just the seconds but in case someone orders something on the same second you will want microtime to differentiate between the two orders. Although usually you would implement your own unique numbering system. hope this helps
-
The easiest method is a "lookup" array - otherwise you would pretty much rewrite the whole "redirector" code. Make the lookup array like so: // MAKE SURE that the values correspond to the keys in the $offers array! $loopkup = array( "yahoo" => 1, "google" => 2 ); Then make a little switch that will use the lookup table, you can code it so you can use numbers or strings quite easily. // This will let your code take EITHER; the number, or the string; if(isset($_GET['mn']) && is_string($_GET['mn'])){ // Is string, use lookup array // First always error-check, does this item exist? if(isset($lookup[$_GET['mn']])){ $offer_id = $lookup[$_GET['mn']]; }else{ // Error - The requested redirect wasn't found } }else if(isset($_GET['mn']) && is_numeric($_GET['mn'])){ // Is number, use as-is $offer_id = $_GET['mn']; }else{ // Error handling - not a number OR a string. } Then you just edit this part: if (isset($offer_id)) { $magic_number = $offer_id - $safemode; Hope this helps
-
Dont return inside a for loop its never a good idea, if you have to use a break; statement. With your "inconsistent" results - reproduce the poblem with a little code as possible - then give us that, with examples of input and output.
-
No problem, You need to use the "Concatenation-Operand" in your $out assignment line: $out .= ... hope this helps PS, Globals in PHP is a nasty word and i would try to avoid it as best as possible .
-
Need help image verification won't display on mail form
ChemicalBliss replied to domskidan's topic in PHP Coding Help
Should be simple enough to remove, Though i would fix it rather than remove a security feature . Write a simple gd script to make suer gd works, if nto then contact your hosting providers for support. Removing the verification: Page A. (The Form) <form action="contactus_send.php" method="post"> <div align="left"><strong>Name: </strong><br> <input name="name" type="text"> <br> <strong>Phone Number:</strong><br> <input name="phone" type="text"> <br> <strong>E-Mail: </strong><br> <input name="email" type="text"> <br> <br> <strong>Reason For Contacting Us:</strong><br> <select name="reason" id="reason" onChange="MM_jumpMenu('parent',this,0)"> <option>Sales Question</option> <option>Tech Question</option> <option>Website Question</option> <option>Suggestion</option> <option>Feedback</option> </select> <br> <br> <strong>Message:</strong><br> <textarea name="message" cols="60" rows="10"></textarea> <br> </div> <input name="Submit" type="submit" value="Submit"> </form> Page B (the mailer) <?php // ----------------------------------------- // The Web Help .com // ----------------------------------------- // remember to replace you@email.com with your own email address lower in this code. // load the variables form address bar $subject = $_REQUEST["subject"]; $name = $_REQUEST["name"]; $phone = $_REQUEST["phone"]; $email = $_REQUEST["email"]; $reason = $_REQUEST["reason"]; $message = $_REQUEST["message"]; $from = $_REQUEST["from"]; $break = "\n\n"; // remove the backslashes that normally appears when entering " or ' $eventtype = stripslashes($eventtype); $phone = stripslashes($phone); $name = stripslashes($name); $message = stripslashes($message); $subject = stripslashes($subject); $from = stripslashes($from); // check to see if verificaton code was correct // if verification code was correct send the message and show this page mail("domskidan@gmail.com", 'New Contact Message: '.$subject, "\n".'****** New Contact Message ******'.$break.'Name: '.$name.$break.'Phone Number: '.$phone.$break.'E-mail: '.$email.$break.'Reason For Contact: '.$reason.$break.'Message: '.$message.$break, "From: $from");?> hope this helps -
creating a routing system (mvc framework)
ChemicalBliss replied to Destramic's topic in PHP Coding Help
I would use an extended class that would basically create the functionality of a static controller. The interface should already be defined by its parent abstract class. For example, you could have a controller object for web requests, which would have every method needed for extracting the input data from the client request. Then another for remote commands, by shell for ex, which would hold everything needed for getting arguments passed via a command line. You could even further extend the web request controller to add certain Hard Coded (independent of mod-rewrite) "friendly URL" formats. hope this helps -
Also, I had a bug in my code i gave you - a logic error . Here it is in it's C++ mimic glory <?php $User = 'itsausername'; $key = 56; for($i=0;$i<strlen($User);$i++) { $User = substr($User, 0, $i).chr(ord($User{$i}) ^ $key).substr($User, $i + 1, (strlen($User) - ($i + 1))); } echo($User); ?> hope this helps
-
It is as i expected - PHP's different approach to "Characters" and "Strings"; For example; The ASCII id of A is 65: PHP: A is stored a literal character rather than a number C++: A is stored as it's ASCII id Results: PHP: "A" (the string) XOR "56" (also string) - note php cannot XOR between different variable types (int+string). C++ "65" (integer) XOR "56" (also integer) Simple Demonstration: C++ Mimic: <?php $User = ord('A'); // ord() gets the ASCII id of this character $key = 56; echo "Result Integer: ".($User ^ $key). "Character: ".chr($User ^ $key); PHP Native: <?php $User = 'A'; $key = '56'; echo "Result: ".($User ^ $key); hope this helps
-
Can you give us some more information, The original string would be nice so we can try to replicate the c++ results in our own php environment. Also check if the variables $User is the same in each program and that $key is the same type (PHP String vs Integer).
-
creating a routing system (mvc framework)
ChemicalBliss replied to Destramic's topic in PHP Coding Help
The controller object should have an interface to the route object, though, IMO an extension object of an abstract controller object should be all that is needed to implement a static controller, and have enough flexibility to exchange it for a dynamic controller extension object in the future. -
Sometimes you can "over simplify" problems, looks cool - but in the long run won't do you any favors . Still, I cannot think of a single mysql query that could get those 6 seperate counts into a variable that isn't an array and is on 1 line .
-
Since your not trying to code this yourself i gues your wanting to start from this script and work around it. Go use google . http://lmgtfy.com/?q=php+image+upload
-
That is the empty array . The numbers on the left are the "KEYS" of each "item" of the array, whereas the numbers on the right are the "VALUE"s of each item in the array. So, we are using the array "KEYS" as the "vendor_type_ids", this way we can more easily "increment" each value as neccessary in the loop. for instance, if you only wanted to count the vendor type ids 3,5 and 6 then; Query: // Query - Note: only select the vendor_type_id field as thats all we really need to seperate the data. $query = "SELECT vendor_type_id FROM vendors WHERE vendor_type_id = 3 OR vendor_type_id = 5 OR vendor_type_id = 6 GROUP BY vendor_type_id"; // Note: GROUP BY - will group vendor ids together in blocks. Empty Array: // Setup an empty array we can use to count the different vendor ids, change keys here to reflect what vendor type ids your using $vendor_counts = array( 3 => 0, 5 => 0, 6 => 0 ); hope this clears things up PS: The code I originally gave you should be able to replace the code you originally gave us . - though the variable names etc will be different and the print_r() is there to show you the results - look in the "source Code" of the html result.
-
hotscripts.com to name one. Google to name a thousand other good sources of PHP scripts . Though, I've not used any image upload scripts from external sources I've created them mysql for absolute peace-of-mind. hope this helps
-
creating a routing system (mvc framework)
ChemicalBliss replied to Destramic's topic in PHP Coding Help
That could work fine, Though it all depends on your projects structure. This is something you would design into your application, depending on how abtract you want your components. (I'm guessing you've read a little about OOP and MVC structures). You could have it in config as you suggested/In the page controller class itself/in a database such as mysql for dynamic pages. It is entirely your choice depending on how flexible you want your framework to be. hope this helps -
Just a couple things to note; 1. External resources (mysql) is really expensive in PHP resources. Every call costs a lot. Benchmark our scripts to find which is fastest . 2. Also code readability is very important is reusable code (which you should eb aiming for ).
-
Don't forget to post your findings it could be useful to someone else .
-
Don't be afraid to try your ideas... Just be prepared to get attacked by spammers/script kiddies etc. Live and learn in PHP is the best way as your own mistakes stick with you much easier than someones advice .
-
Ahh, this is much better than my suggestion. A lot less lines of code needed for the selection of vendors. I didn't think about using a loop. doh. Yes but too many mysql queries imo
-
I really dont know C++ well but the differences seem to be that in your PHP exampel you have to concatenate the string, whereas on C++ you change the character independently. To try to mimic this in PHP you could try: for($i=0;$i<strlen($User);$i++) { $XOR = $User{$i} ^ $key; $startString = substr($User, 0, $i); $endString = substr($User, $i + 1, (strlen($User) - $i) - 1); $User = $startString.$XOR.$endstring; } Also, Try a simple XOR, one character, in each program: c++ int key = 10; char* input = "g"; input = input ^ key; php $key = 10; $input = "g"; $input =$input ^ $key; Also check out http://php.net/manual/en/language.operators.bitwise.php hope this helps, Good luck
-
No, For simplicity im goign to call your "ghosts" => "guests". On most posting boards, guests have very limited power and that is for good reason, among many other vulnerabilities your website has no way of spam control, since the user is not forced to sign up with an email or some other form of external object it makes it very easy to just clear cache/cookies and change ip - ie. Spam spam spam crash. That aside if you really want guests to be able to post just let them post, I would highly discourage this though unless it's something like a shout-box with limited messages and good security. good luck, though
-
Why yes, there is indeed . A) always use <?php ?> - its my pet hate to see a shorthand. B) The less mysql calls (and also the smaller the manipulated data) the better. Here's how i would do it: <?php // Get all rows with all vendors // Query - Note: only select the vendor_type_id field as thats all we really need to seperate the data. $query = "SELECT vendor_type_id FROM vendors WHERE vendor_type_id = 1 OR vendor_type_id = 2 OR vendor_type_id = 3 OR vendor_type_id = 4 OR vendor_type_id = 5 OR vendor_type_id = 6 GROUP BY vendor_type_id"; // Note: GROUP BY - will group vendor ids together in blocks. $result = mysql_query($query); // Setup an empty array we can use to count the different vendor ids, change keys here to reflect what vendor type ids your using $vendor_counts = array( 1 => 0, 2 => 0, 3 => 0, 4 => 0, 5 => 0, 6 => 0 ); // Now we count each vendor id; while($row = mysql_fetch_assoc($result)){ $vendor_counts[$row['vendor_type_id']]++; } // Now print results: print_r($vendor_counts); ?> One mysql query. Counts stored in an array for easy and quick access. Could easily be made dynamic b incorporating variable vendor type ids. hope this helps
-
I dont know the SOAP api but to get a readable result from testUser: print_r $testUser; print_r $testUser->return->ErrorStr; print_r $testUser->return->ErrorID; - View in the page source hope this helps