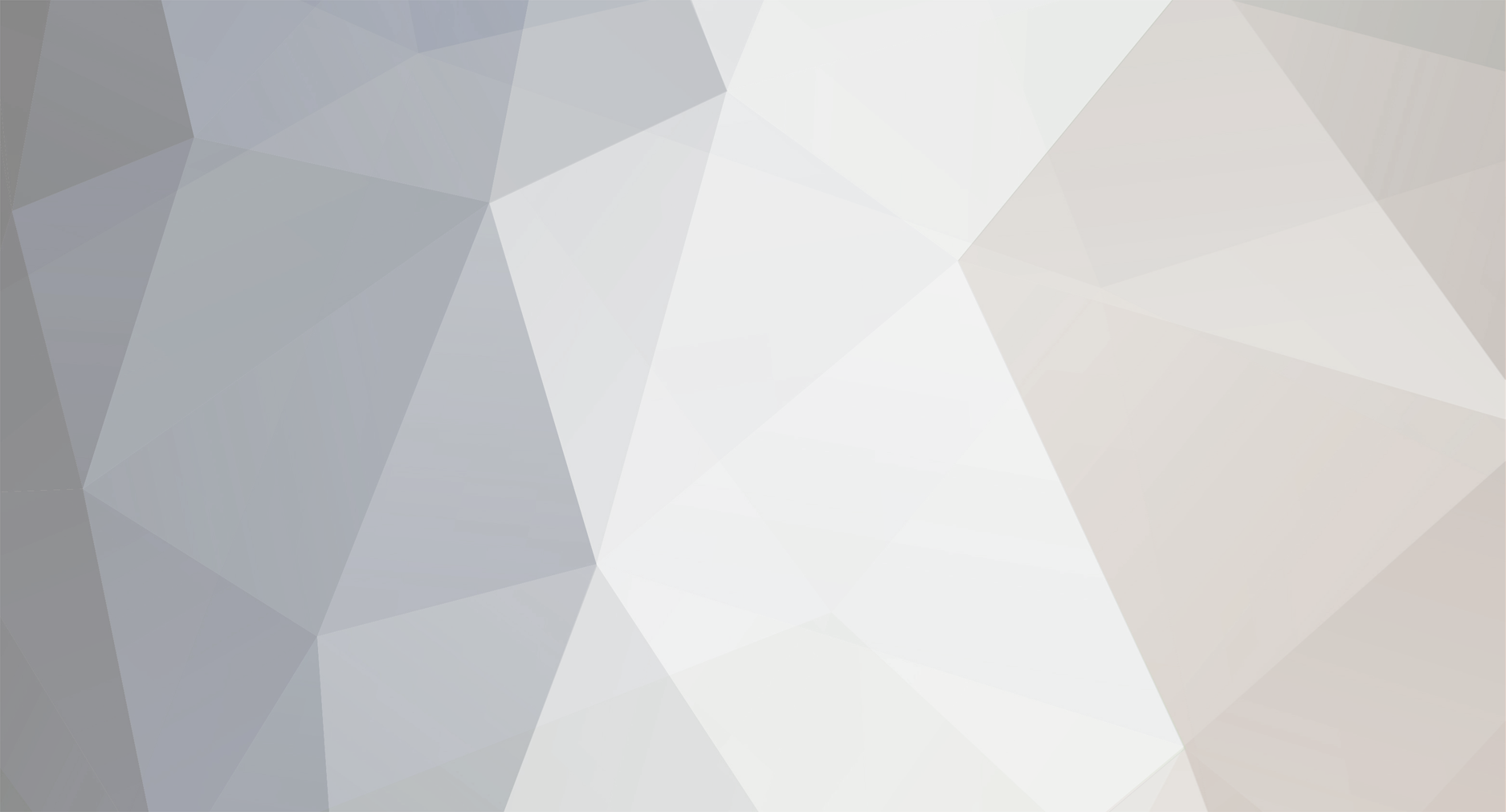
AndyB
-
Posts
5,465 -
Joined
-
Last visited
Never
Posts posted by AndyB
-
-
php variables are 'loosely typed'. If you add zero to a string you get a number.
-
[b]You[/b] might always know exactly what's going on with your scripts, but your scripts (sometimes) have to deal with user input ... and users are the most unreliable things in the universe. If a user 'forgets' to provide a form input, wouldn't it be nice to know that an important variable had been set (by the user's input) before you processed their data?
-
I think there's some confusion here between javascript (client side), jsp (java server pages, i.e. server-side), and java .. all of which are different.
-
Basically, put what users enter into your database 'as is' and then when you display it use the [a href=\"http://ca.php.net/manual/en/function.nl2br.php\" target=\"_blank\"]nl2br() function[/a] to convert 'new lines' into html <br> tags:
[code]$nice_text = nl2br($text);
echo $nice_text;[/code] -
[!--quoteo--][div class=\'quotetop\']QUOTE[/div][div class=\'quotemain\'][!--quotec--]You are a Genius [/quote]
Not exactly. I spend time reading here, time looking through the manual, time looking through other people's scripts, and have gradually reduced the number of crazy mistakes I make to manageable proportions :) -
[!--quoteo(post=365709:date=Apr 17 2006, 05:58 PM:name=businessman332211)--][div class=\'quotetop\']QUOTE(businessman332211 @ Apr 17 2006, 05:58 PM) [snapback]365709[/snapback][/div][div class=\'quotemain\'][!--quotec--]so what would the purpose of taking strings out of a specific file without pulling the whole file.[/quote]
It's called modular design (among other things). By using an included file instead of entering the same content into every page of a site, you can change one file and have every page change. Also, you never need to debug a piece of code that works only the stuff outside the included parts.
An approximate HTML equivalent to an included file is an external javascript or external CSS file.
-
Simply, you cannot have any output to the browser before the header() function. In the script you gave, just delete the title line (and anything above it that you didn't include here). That should fix it.
-
The link you gave to the validator output (as well as the online code itself) are both the same. They use HREF (incorrect) and should be href, they have an image alt tag missing, and they pass a parameter in the link using & which needs to be & which is what causes all the errors on line 73.
If you fix those online and revalidate there should not be any errors.
-
[code]<div class='random-image'><a HREF='index.php?page=viewgallery&category=Random'><img src='gallery/tn_mr_bean1.jpg' /></a></div> [/code]
Change to:
[code]<div class='random-image'><a href='index.php?page=viewgallery&category=Random'><img src='gallery/tn_mr_bean1.jpg' /></a></div> [/code]
That should clear all your errors. -
[code]$doit = $_GET['action'];
if ($doit == "rename") {
echo "Rename ....";
... more code ...
}
[/code] -
[code] $result1 = mysql_query($query,$connect1)[/code]
That should be:
[code] $result1 = mysql_query($query1,$connect1)[/code] -
The value of $row used in the first loop is being reset in the second loop. Try this:
while([b]$row1[/b] = mysql_fetch_array($result1))
{
echo "<td><b>";
echo ''.$[b]row1[/b]['quantity'].''; -
Your code/life will be simplest if you create an array of all the countries, and then use a simple piece of code like the below to generate your drop-down and show the selected country:
[code]include("country_array.php");
echo "<select size='1' name='country'>";
for ($i=0;$i<count($countries);$i++ {
echo "<option value='". $countries[$i]. "'";
if ($country_from_db==$countries[$i]) { echo " selected style='background-color:#6ff'"; }
echo ">". $countries[$i]. "</option>\n";
}
echo "</select>";[/code]
The countries array file looks like:
[code]
$countries = array("Afghanistan", "Albania", lots more sountries, "Zambia", "Zimbabwe");[/code] -
Suggestions.
First, I assume that id is sent to this page from a page (contact_menu.php presumably) which contains a form using the method="get" since your commented code suggests checking form values. If the form uses method="post" the $_GET array value will always be absent.
Second, there's no way to tell at which stage the script tries to find a page that doesn't exist. For debugging purposes I'd suggest changing each instance of the header/location function to something simple like:
[code]echo "failed at line whatever";
exit;[/code]
At least then you'll know what's causing it to fail.
Third, add Barand's check in your script.
And if a page can't be found, then the script is looking for a file that doesn't exist with the name you expect in the location you expect. -
Show us your code, please.
-
If it used to work perfectly and then stopped working AND you didn't make any changes to it, then possibly your host upgraded php to a version where register_globals is OFF and your code assumed it was ON. How are the variables retrieved by your script?
-
It's an html question. Use textarea, not text, as the input type. Adjust the number of rows and number of columns (horizontal size) to suit. For example:
[code]
<textarea name="something" rows="3" cols="30"><?php echo $something; ?></textarea>
[/code] -
[!--quoteo(post=365192:date=Apr 15 2006, 08:33 PM:name=hpughLW)--][div class=\'quotetop\']QUOTE(hpughLW @ Apr 15 2006, 08:33 PM) [snapback]365192[/snapback][/div][div class=\'quotemain\'][!--quotec--]What do you mean by a relative page address?[/quote]
This link explains ... [a href=\"http://www.thuto.org/ubh/web/html/relad1.htm\" target=\"_blank\"]http://www.thuto.org/ubh/web/html/relad1.htm[/a]
In your case, if contact_menu.php is in the same folder as the script with the problem, then this will work [relative address]
[code]header("Location: contact_menu.php");[/code]
-
[a href=\"http://localhost/contact_menu.php\" target=\"_blank\"]http://localhost/contact_menu.php[/a] is the only page that wouldn't be displayed. Are you sure it exists with that name in that folder? Personally, I'd change the redirect to a relative page address so when the script is uploaded to the server it'll still work as expected.
-
OK, so what's the error message?
-
The stray character isn't visible with Firefox ... but the layout breaks horribly using Firefox at 1024px resolution. That's a more significant problem, unfortunately.
-
Assuming both these variables exist in the database and that the db contains data, what does the [b]generated html [/b]look like if you use this:
[code]echo "<OPTION VALUE='". $record['fname']. "'>". $record['name']. "</option>";[/code] -
Move the select closing tag ahead of the submit input and close the option tags.
[code]<html>
<head>
<title>search2</title>
<body>
search me via search box
<form method="post" action="search.php>
<SELECT NAME="name">
<?
$db=mysql_connect("localhost","xxx","xxx");
mysql_select_db("freedating", $db);
$query="select * from pro_membersu";
$result=mysql_query($query);
while($record=mysql_fetch_assoc($result)){
echo "<OPTION VALUE='".$record["fname"]."'>".$record[name]. "</option>";
}
echo "</select>";
?>
<input type="submit" value="submit">
</form>
</body>[/code]
-
Try replacing each occurrence similar to this:
[code]<?php echo '$companys[avatar]'; ?>[/code]
With this type of code:
[code]<?php echo $companys['avatar']; ?>[/code]
isset()
in PHP Coding Help
Posted
One reason why some people might like to use client-side validation is that they create scripts in two parts: the data input script and the data processing script. In that case they may find they want the user to go back to a blank form just because one field was invalid. Bad design. Better to have the data input and processing in the same script so you can echo back user inputs when you need them to resubmit the form without filling it out again. Then you'll need some conditional branching to test whether the form has been submitted or whether this is the first visit to the form. isset() is one way of testing for specific input to use for such branching.
And, of course, when you pass data via URL (click a link like showstuff.php?id=27) you can't validate that client-side if your visitors edit what's in the browser address bar to showstuff.php (for example). Isn't isset() nice to have?