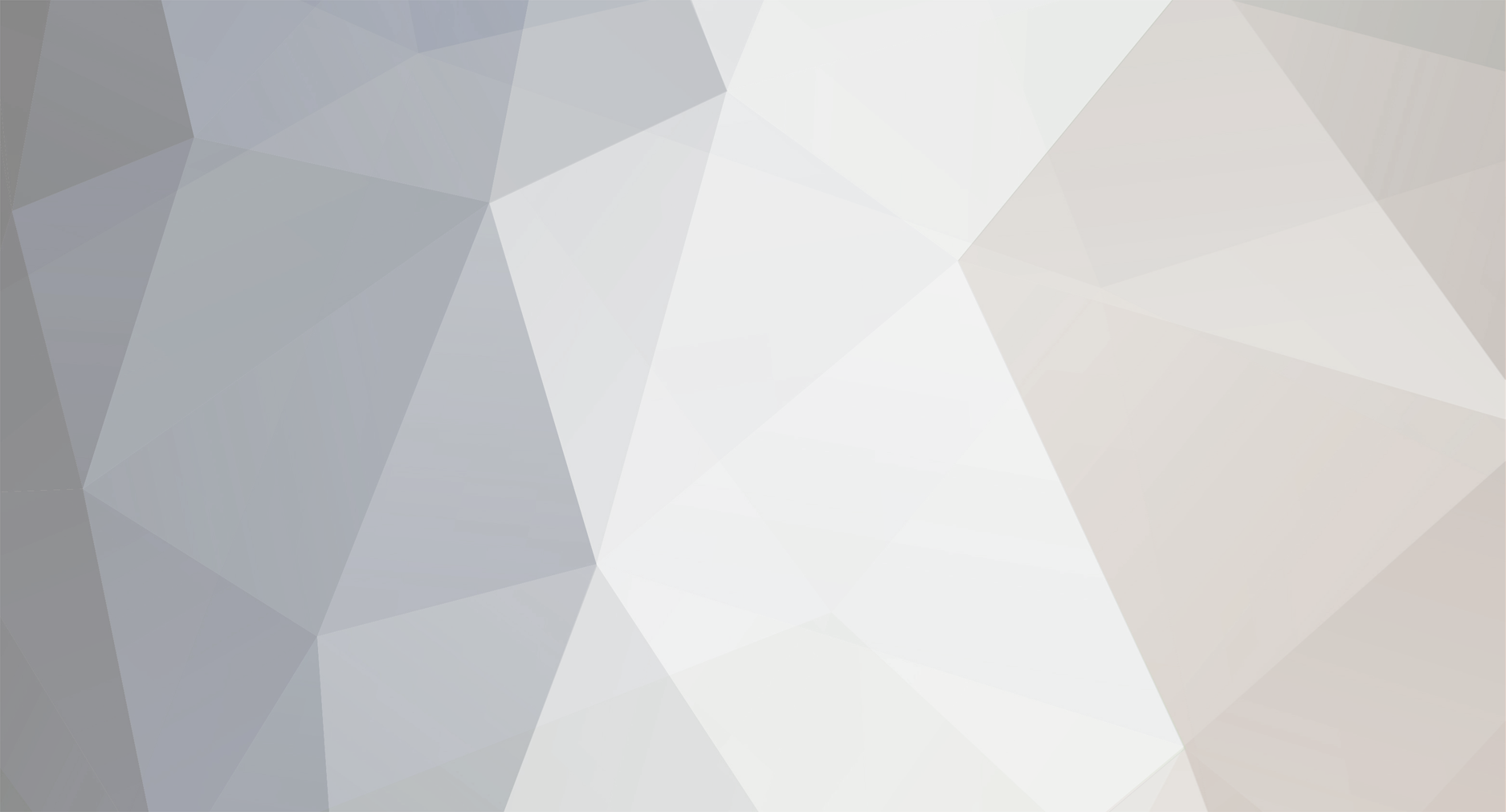
RopeADope
Members-
Posts
157 -
Joined
-
Last visited
Everything posted by RopeADope
-
Well...I've read the tutorials and did my best but I still feel I've made a terrible mess of what OOPHP should be. Due to lack of experience, I'm not entirely sure this makes sense but it does in my head so here it goes.... class auth { var $username; var $password; function connect(){ $db="main_db"; $dbuser="db_user"; $dbpass="db_password"; $host="localhost"; mysql_connect($host, $dbuser, $dbpass)or die('Error establishing connection!'); mysql_select_db($dbname)or die('Error connecting to the database!'); } function clean_input($username,$password){ $regex="'/^[A-Za-z0-9]+$/'"; //Expression to match $repex=""; //Replace unmet characters with nothing, i.e. remove them from the string preg_replace($regex, $repex, $username); preg_replace($regex, $repex, $password); } function auth_user(){ $sql=mysql_query("SELECT * FROM users WHERE username='$username' AND password='$password'"); $result=mysql_result($sql); if($result==1) { header('Location:main.php'); }else{ header('Location:index.php'); }; }; }; Sneaking suspicions: 1) I should have a setter method somewhere. 2) The connect function should take arguments 3) clean_input function is off somehow...RegEx off?(goal is to only allow alphanumeric characters) Mainly looking for ways to improve efficieny, make it more reusable, and more importantly that it works. Thanks in advance!
-
Just had a few PHP include questions. Is there a "best practice" when using PHP include, inlcude_once, require(e.g. when to use each one)? When including a file, does it have to be .php and should it be "echoed out"? Q2 Example: File to be included <!-- somefile.txt --> <html> vs. <?php echo "<html>"; ?> File doing the include //index.php <?php include('inc.php'); ?>
-
Sorry for the long delay. Decided to take some time off. So idk why I didn't realize it earlier but you're right, I shouldn't be using mysql_real_escape_string(). My goal is to basically protect against injection as this project will eventually be hosted online. With that said, what's the best method to prevent against said injection? I assume the best way would be to clean the data of any dangerous characters before comparing it with database values, correct?
-
I did that. As a test, I commented out the mysql_real_escape lines and just echoed the $_POST variables and they showed up. It seems like something happens when they mysql_real_escape lines are executed because if I try to echo the $usr and $pwd variables, nothing shows up, but the $_POST variables will.
-
Hi all. This is my first time working with .htaccess files so I searched around, and didn't find anything helpful. I'm trying to use an .htaccess file in a subdirectory of my document root to stop the browser from showing the document list. I'm also trying to change the DirectoryIndex from the default to dashboard.php, but its not working. Do I have to restart apache to have it recognize the .htaccess file? .htaccess Options -Index DirectoryIndex dashboard.php (dashboard.php and .htaccess are in the same directory)
-
So I've figured out that for some reason, the mysql_real_escape_string() is causing problems. When I put my $_POST variables in the function, the variables $usr and $pwd wind up having no value. Anybody know why this might be?
-
Hi all. Just wanted to post this snippet to make sure I wrote it correctly. My intent is that upon clicking "Enter" on the home page, the login request will get sent to this snippet, escape the dangerous characters, then validate according to the result returned from the users table. <?php include('connect.php'); $usr=mysql_real_escape_string($_POST['usr']); $pwd=mysql_real_escape_string($_POST['pwd']); $sql="SELECT FROM users WHERE username='$usr' AND password='$pwd'"; $query=mysql_query($sql); $num=mysql_num_rows($query); if($num!=1){ header('Location: index.php'); } ?>
-
Help with TRIGGERS/Best practices for CASCADE/UPDATE/DELETE
RopeADope replied to RopeADope's topic in MySQL Help
That's the nature of Microsoft? lol. This was created by my boss, whom had to read a book on Access before creating it, so I'm sure this is not the first issue I'll run into...So anyway, my previous question, keep it in the DB or make it app based?(the above query is just one of many) -
Help with TRIGGERS/Best practices for CASCADE/UPDATE/DELETE
RopeADope replied to RopeADope's topic in MySQL Help
According to me, yes. Gotcha. So one thing I should probably mention(if I haven't already) is I'm converting this from MSAccess to MySQL. I didn't design the initial database or the queries(written in SQL). So here's a sample of the kind of queries I'm dealing with... SELECT Availability.AvailabilityID, Availability.AvailDate, Availability.ClientID, Availability.KPIAvailMinID, Availability.KPIAvailObjID, Availability.BusParaID, Availability.AvailIncid, Availability.AvailUnavil, Availability.AvailDownCServ, Availability.AvailDownCSys, Availability.AvailDownAppDev, Availability.AvailDownInfra, Availability.UtilUnavail, Availability.AvailAuto, Availability.Availproc, Availability.TotalAvail, Availability.VendMissed, Availability.CSrvOLATargets, Availability.CSysOLATargets, Availability.AppDevOLATargets, Availability.InfraOLATargets, Availability.InfSecOLATargets, Availability.VendTargets, Availability.CSrvOLAMissed, Availability.CSysOLAMissed, Availability.AppDevOLAMissed, Availability.InfraOLAMissed, Availability.InfSecOLAMissed, Availability.SecIncidents, Availability.HwSwNetCIs, Availability.HSNCIOrphans, Availability.NoAvailPlan, Availability.NoAvailReview, Availability.AvailComments, BusPara.P1Services, BusPara.P2Services, BusPara.P3Services, (1-[AvailUnavil]/[TotalAvail]) AS ServiceRelX, ([P1Services]+[P2Services])*30*24 AS TotalAvailability, (1- ([CSrvOLAMissed]+[CSysOLAMissed]+[AppDevOLAMissed]+[infraOLAMissed]+[infSecO LAMissed])/([CSrvOLATargets]+[CSysOLATargets]+[AppDevOLATargets]+[infraOLATar gets]+[infSecOLATargets])) AS IntsupRel, (1-([VendMissed]/[VendTargets])) AS VendSupRel, ([HSNCIOrphans]/[HwSwNetCIs]) AS ServabilX, ([NoAvailPlan]/[busPara]![ActiveServices]) AS AvailRiskX, (1- ([NoAvailPlan]/[busPara]![ActiveServices])) AS CQIX, ([utilUnavail]/[TotalUtilAvail]) AS AvailUtil So with that in mind, is your advice to still confine the logic to my app? -
Help with TRIGGERS/Best practices for CASCADE/UPDATE/DELETE
RopeADope replied to RopeADope's topic in MySQL Help
--:: Sorry. I need a trigger that will cascade an id to a child table from its parent table, when a new entry is made in the parent table. So something like "insert into parent_table, then cascade the insert to child_table". --So if I can keep the logic in my app, I should do that instead of confining the logic to the DB? -
Hi all. My post is twofold today. 1st Q: Can anyone provide me with a ridiculously simple snippet of code for a trigger that cascades a single field from tableA to tableB? I've searched around but my brain just goes haywire with the examples that I've seen. If not a snippet, then a variable representation would work(e.g. CREATE TRIGGER trigger_name INSERT table.field............END) 2nd Q: My goal is to basically cascade keys from parent to child tables(MyISAM). I'm aware that InnoDB does this automagically but from what I've read, its fairly easy to write a trigger(even though they confuse me :'(). Are triggers the best way to enforce the keys or would it be easier to write some PHP to do multiple update statements in SQL via the UNION option?(Just ask if clarification is needed on this one. I'm not entirely sure I got my point across)
-
Ok, so OOPHP and PHPDB need to be essentially put on the same level, as in they coexist instead of one relying on or replacing the other, correct? My question arises from my current project, a car audio website. I'm trying to figure out how to apply OOPHP to that car audio website. Right off the bat, I know I can apply OO concepts to a shopping cart, and the car audio items, but I'm not sure how much further it can go(as I have limited knowledge of OO). Hypothetical Q: Could you treat an entire website as an object? e.g. class website{ var $my_website __construct(my_website){ function header(){ } function body(){ } Ok, so OOPHP and PHPDB need to be essentially put on the same level, as in they coexist instead of one relying on or replacing the other, correct? My question arises from my current project, a car audio website. I'm trying to figure out how to apply OOPHP to that car audio website. Right off the bat, I know I can apply OO concepts to a shopping cart, and the car audio items, but I'm not sure how much further it can go(as I have limited knowledge of OO). Hypothetical Q: Could you treat an entire website as an object? e.g. class website{ var $my_website __construct(my_website){ function header(){ } function body(){ } Ok, so OOPHP and PHPDB need to be essentially put on the same level, as in they coexist instead of one relying on or replacing the other, correct? My question arises from my current project, a car audio website. I'm trying to figure out how to apply OOPHP to that car audio website. Right off the bat, I know I can apply OO concepts to a shopping cart, and the car audio items, but I'm not sure how much further it can go(as I have limited knowledge of OO). Hypothetical Q: Could you treat an entire website as an object?(or am I making this way too abstract? lol) e.g. class website{ var $my_website __construct(my_website){ function header(){ } function body(){ } function footer(){ } }
-
Hi all. I found a previous thread on here with someone asking about MyISAM vs InnoDB(I have this same question). After reading through the thread, the basic conclusion was that even though MyISAM doesn't have direct support for foreign keys, you can manually set up MyISAM to enforce/cascade the updates and deletes. Am I on the right track here or am I still missing some vital info? And am I okay sticking with MyISAM even though I'm using foreign keys, or should I switch to InnoDB?
-
I've never done any OOPHP and I'm trying to get my head wrapped around the concept. I think I've got it but I have a question. Isn't OOPHP like databasing without actually using a database? This is my understanding of how it works... OOPHP class X{ functionY(p1,p2,p3){ property1 property2 property2 } } do_functionY(p1,p2,p3); PHP&DB (input to DB here) for(xxxxx){ mysql_result(p1); mysql_result(p2); mysql_result(p3); } while(i>0){ echo "p1,p2,p3"; } I know the examples aren't syntactically correct but I was trying to be brief, and they get my point across. I've read a bunch of tutorials and such for OOPHP but I don't understand the purpose when databasing does the same thing(at least in my mind ). Any help would be much appreciated, I've been debating this for some time now...
-
More tables vs fewer tables, question of efficiency
RopeADope replied to RopeADope's topic in MySQL Help
It does, thanks. I've done some more research since posting and what I've found is basically that new computers are so fast, searching a few thousand+ records is quicker than searching a few thousand+ tables. -
Right, user auth is my main bullet point when I say security. Do you have a link or some snippets of code that show how to execute the various methods?
-
Ah, I apologize, perhaps I was unclear. I guess in a nutshell: Is there a better way to protect pages that are supposed to be viewable only upon login than checking for session variables? My initial question about the security token was just my brain wondering if you could take a bunch of session variables and wrap them up into a package. I think we've digressed from that however
-
Congrats on the progress. Do you have a website where I could check out the yet-to-be-named service you're offering? Two Cents: Imo there's no reason, in almost any situation, to not offer something via SaaS. There internet isn't going to shrink anytime soon, lol.
-
So pretty much just stick with the traditional method of security?(session variables?) If there's a more secure alternative, I'd appreciate a link. I've only ever learned security via sessions and/or cookies.
-
Go with SaaS. In the long run it'll save you time and the less you have to do for clients directly, is money in the bank.
-
So I'm still fairly new to PHP. Been working with it for several years but most of my projects are/were simple websites. I've started to dabble in OOPHP and I've got a question related to security. I know the old way(well its old to me anyway ) is to use session variables. 1st Q: Would it be a good practice to put several session variables into a "security token" object? Advantages/disadvantages? 2nd Q: If it is a good idea to created said security token, would it provide an extra measure of security to check the session variables against a cookie?(e.g. would this provide a check and balance type security measure? {check cookie, if !security_token[0][1][etc] in cookie, destroy session, set cookie null}) Any advice would be much appreciated
-
Ah, I see. Thanks for clearing it up!
-
Ah. This isn't really a phpMyAdmin type program, its more an interface for viewing and working with data, not actual table structure or anything that in depth. So my next question would be, can I have 50-100 users connected to the DB with a generic MySQL user at the same time? For instance, can I have Fred, Ted, and Bob all logged into the dashboard and INSERT/UPDATE/VIEW data with the same MySQL user?
-
The users will have access to the dashboard, as well as be able to enter/update/view data. So for this purpose, would they need to be in MySQL.users or can I use a generic MySQL.users user with select/update/insert privileges?
-
Hi all. My end result to my current project is basically a dashboard type deal, PHP driven with a MySQL database. My question is--"When setting up users to generate info from the database, should these users be established in the MySQL DB, or should they be in a "users table" that PHP checks for authentication?" I realize the question sounds like its asking the same thing twice, but I guess the essence of what I'm getting at is "MySQL.users vs MyDB.users?"