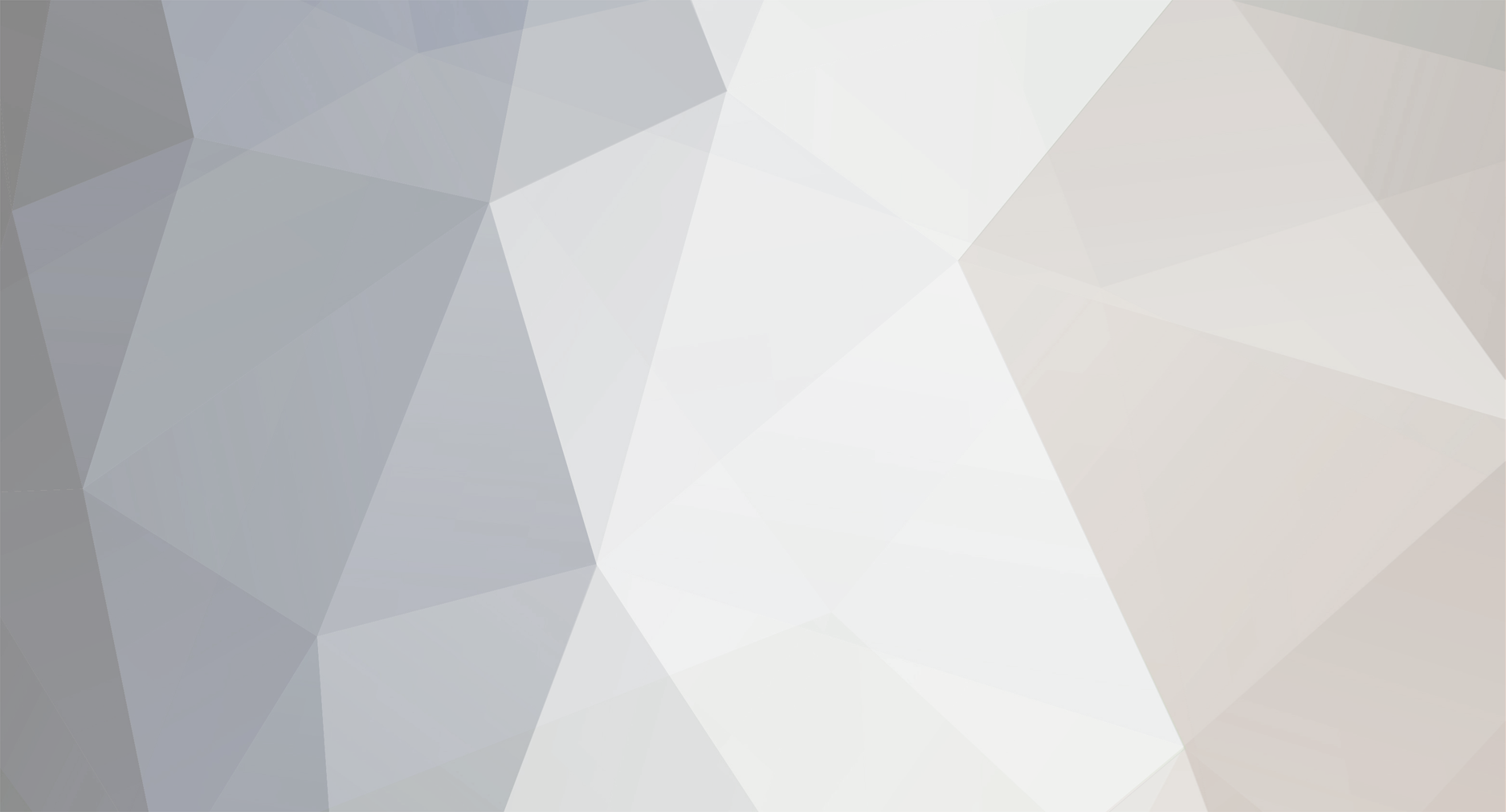
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
hmm...question on mysql db id number auto_increment..
jcbones replied to shane85's topic in PHP Coding Help
Instead of x, just use number 1. Mysql will auto adjust to the next available increment. -
To read from a file and return the last 10 lines: $file = 'file.txt'; $res = file($file); $count = count($res); for($i = ($count - 10); $i <= $count; $i++) { echo $res[$i]; }
-
http://www.php.net/manual/en/function.fopen.php http://www.php.net/manual/en/function.fwrite.php http://www.php.net/manual/en/function.fclose.php
-
You cannot keep people from changing $_GET variables. You can sanitize them to the best of your ability, but someone can always type in something different. With a variable that changes alot (product ids), you run into more problems in sanitation. Namely, the more strict the sanitation, the more limited you are when adding product Id's. If you have a product Id 129874321, and part of your sanitation includes (int)$_GET['product_id']. You have just lost the ability to add a product with the id of 129874321b. Just keep in mind that your script needs to be written as secure as possible, but with the ability to expand for future use. You don't want to have to re-write a script, just to add a product id.
-
My take on this problem: <?php $January = 0; $February = 0; $March = 0; $April = 0; $May = 0; $June = 0; $July = 0; $August = 0; $September = 0; $October = 0; $November = 0; $December = 0; $sql = "SELECT * FROM `$blog`"; $result = mysql_query($sql); if(mysql_num_rows($result) > 0) { while($r = mysql_fetch_assoc($result)) { @$$r['month'] += 1; } echo '<ul id="monthul"> <li><a href="index.php?month=January">January</a> ('.$January.')</li> <li><a href="index.php?month=February">February</a> ('.$February.')</li> <li><a href="index.php?month=March">March</a> ('.$March.')</li> <li><a href="index.php?month=April">April</a> ('.$April.')</li> <li><a href="index.php?month=May">May</a> ('.$May.')</li> <li><a href="index.php?month=June">June</a> ('.$June.')</li> <li><a href="index.php?month=July">July</a> ('.$July.')</li> <li><a href="index.php?month=August">August</a> ('.$August.')</li> <li><a href="index.php?month=September">September</a> ('.$September.')</li> <li><a href="index.php?month=October">October</a> ('.$October.')</li> <li><a href="index.php?month=November">November</a> ('.$November.')</li> <li><a href="index.php?month=December">December</a> ('.$December.')</li> </ul>'; ?>
-
Counting the number of times something is in the table
jcbones replied to Potatis's topic in PHP Coding Help
Don't give up yet, I just seen your Db structure. Try this: <?php include('includes/admin_session.php'); ?> <?php require_once("includes/connection.php"); // Set variables from search query /*$tutor_name = $_POST['tutor_name']; $school_name = $_POST['school']; $term = $_POST['term']; $year = $_POST['year'];*/ $tutor_name = "Krismack"; $school_name = "MPS"; $term = "1"; $year = "2010"; // Query database with the above variables to get all stored data belonging to the tutor, at X school during X term and X year $sql = "SELECT * FROM student_roll WHERE (tutor_name='$tutor_name' AND school='$school_name' AND term='$term' AND year='$year') ORDER BY last_name ASC"; $result = mysqli_query($connection,$sql); if(mysqli_num_rows($result)) { while($row = mysqli_fetch_assoc($result)) { $student_first_name = $row['first_name']; $student_last_name = $row['last_name']; //set students in an array, so we increment a count to keep total rows. @$students[$student_first_name][$student_last_name] += 0; if($row['week1'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week2'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week3'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week4'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week5'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week6'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week7'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week8'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week9'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week10'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } } } if(is_array($students)) { foreach($students as $fname => $dem2) { foreach($dem2 as $lname => $count) { echo $fname . " " . $lname . ": "; echo $count; echo "<br />"; echo "<br />"; } } } else { echo ucwords(strtolower($tutor_name)) . ' does not have any students to display!'; } ?> -
Yes, I added it into the wrong section. My mistake, but the problem has been identified already. I knew better, but I insist on doing this stuff when I can barely hold my eyes open.
-
<?php $column_to_search = 'column'; $search = $_POST['search']; $ex = explode(' ',$search); foreach($ex as $value) { $str[] = "column LIKE '%$value'"; } $search = implode(' || ',$str); $q = "SELECT * FROM Table WHERE $search";
-
<?php require("connections/db.php"); require("connections/require.php"); $que = mysql_query("SELECT * FROM `staff` WHERE `playername` = '$player' LIMIT 1")or die(mysql_error()); $arr = mysql_fetch_array($que); $lvl = $arr['level']; if ($lvl == 4){ if ($_POST['clean']) { $result_dead=mysql_query("SELECT * FROM `players` WHERE `status` = 'dead'"); while ($row_dead = mysql_fetch_array($result_dead, MYSQL_ASSOC)) { $dead=$row['owner field from players']; } mysql_query ("DELETE FROM `garage` WHERE `owner` = '$dead'"); echo "Success"; } ?> <html> <head> <title>DataBase Cleaner</title> <link href="style.css" rel="stylesheet" type="text/css"> </head> <body> <br /> <form action="clean.php" method="post"> Clean the Database <br /> <br /> <br /> <br /> <input name="clean" class="submit" type="submit" value="Submit" id="clean"> <? } else{ echo "No Access"; die(); } ?>
-
Change the switch to: switch($_GET['orderby']) { case "contact": $orderby="$arrEntry[contact_next]"; break; case "date": $orderby="$arrEntry[tstamp]"; break; case "company": $orderby="$arrEntry[company_name]"; break; case "city"; $orderby="$arrEntry[city]"; break; default: $orderby="prospect_id"; break; }
-
From post number one, replace this function with the one provided. function query($sql) { $sql = mysql_real_escape_string($sql); $this->Query_ID=mysql_query($sql,$this->connection); if(!$this->Query_ID) { echo "Query Failed".mysql_error(); } else { return $this->Query_ID; } }
-
No, It can be done. You would need a script that looks something like: <?php if(isset($_POST['submit'])) { include('config.php'); //path to your database connection. $save_path = 'upload/'; //path you would like to save your database.sql file. $target = $save_path . $_FILES['file']['name']; if(move_uploaded_file($_FILES['file']['tmp_name'], $target)) { } else { echo "Sorry, there was a problem uploading your file. <br/> Importing Data has been halted!<br/>"; } $file = file_get_contents($target); //removing the standard comments added to a .sql file. $pattern[] = '/--(.*)$/m'; $pattern[] = '/^\/\*(.*?)$/m'; $pattern[] = '/SET SQL_MODE="NO_AUTO_VALUE_ON_ZERO";/'; //replacing the ';' with a newline statement. This will let us seperate the inserts without bothering any semi-colons inside the queries. $pattern[] = '/;$/m'; $replace[] = ''; $replace[] = ''; $replace[] = ''; $replace[] = '__NEWLINE__'; $file = preg_replace($pattern,$replace,$file); //split the queries. $sql = explode('__NEWLINE__',$file); foreach($sql as $value) { $value = trim($value); if($value != '') { if(mysql_query($value)) { $import[] = 1; } else { echo 'Query failed: ' . trim($value) . '<br/>'; $import[] = 2; } } } if(in_array(2,$import)) { echo 'A part of the import process has failed! Please try again!'; } else { echo 'Database Updated!'; } } ?> <form action="" method="post" enctype="multipart/form-data"> <input type="file" name="file"/> <input type="submit" name="submit" value="Upload"/> </form> And then do a db dump to a non-compressed file, one table per file. Of course, there could be errors in there. But, it will get you started.
-
Few things. 1. You need a "type" for your input fields. type="submit", type="text", type="hidden", type="password", etc. 2. You need to pass your database values to a variable. After the last row in the database, the pointer increments to fail the while statement, allowing the script to continue. So, there is no longer a $row[$database_column] to pull from. 3. You are trying to access database information, even if the database returns 0 rows of data. Not a good idea, as you will consistently have inputs with empty value's.
-
<html> <head> </head> <body> <?php // Define db details //db details mysql_connect(DB_HOST, DB_USER, DB_PASSWORD) or trigger_error(mysql_error()); mysql_select_db(DB_DATABASE) or trigger_error(mysql_error()); $tbl_name="prospects"; //your table name // How many adjacent pages should be shown on each side? $adjacents = 3; /* First get total number of rows in data table. If you have a WHERE clause in your query, make sure you mirror it here. */ $query = "SELECT COUNT(*) as num FROM $tbl_name"; $total_pages = mysql_fetch_array(mysql_query($query)); $total_pages = $total_pages['num']; /* Setup vars for query. */ $targetpage = "view_prospect.php"; //your file name (the name of this file) $limit = 5; //how many items to show per page $page = (isset($_GET['page'])) ? (int)$_GET['page'] : 1; $start = ($page - 1) * $limit; //first item to display on this page $queryLimit = 'LIMIT ' . $start . ',' . $limit; /* Get data. */ // $sql = "SELECT prospect_id FROM $tbl_name LIMIT $start, $limit"; // $result = mysql_query($sql); /* Setup page vars for display. */ if ($page == 0) $page = 1; //if no page var is given, default to 1. $prev = $page - 1; //previous page is page - 1 $next = $page + 1; //next page is page + 1 $lastpage = ceil($total_pages/$limit); //lastpage is = total pages / items per page, rounded up. $lpm1 = $lastpage - 1; //last page minus 1 /* Now we apply our rules and draw the pagination object. We're actually saving the code to a variable in case we want to draw it more than once. */ $pagination = ""; if($lastpage > 1) { $pagination .= "<div class=\"pagination\">"; //previous button if ($page > 1) $pagination.= "<a href=\"$targetpage?page=$prev\">« previous</a>"; else $pagination.= "<span class=\"disabled\">« previous</span>"; //pages if ($lastpage < 7 + ($adjacents * 2)) //not enough pages to bother breaking it up { for ($counter = 1; $counter <= $lastpage; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } } elseif($lastpage > 5 + ($adjacents * 2)) //enough pages to hide some { //close to beginning; only hide later pages if($page < 1 + ($adjacents * 2)) { for ($counter = 1; $counter < 4 + ($adjacents * 2); $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } $pagination.= "..."; $pagination.= "<a href=\"$targetpage?page=$lpm1\">$lpm1</a>"; $pagination.= "<a href=\"$targetpage?page=$lastpage\">$lastpage</a>"; } //in middle; hide some front and some back elseif($lastpage - ($adjacents * 2) > $page && $page > ($adjacents * 2)) { $pagination.= "<a href=\"$targetpage?page=1\">1</a>"; $pagination.= "<a href=\"$targetpage?page=2\">2</a>"; $pagination.= "..."; for ($counter = $page - $adjacents; $counter <= $page + $adjacents; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } $pagination.= "..."; $pagination.= "<a href=\"$targetpage?page=$lpm1\">$lpm1</a>"; $pagination.= "<a href=\"$targetpage?page=$lastpage\">$lastpage</a>"; } //close to end; only hide early pages else { $pagination.= "<a href=\"$targetpage?page=1\">1</a>"; $pagination.= "<a href=\"$targetpage?page=2\">2</a>"; $pagination.= "..."; for ($counter = $lastpage - (2 + ($adjacents * 2)); $counter <= $lastpage; $counter++) { if ($counter == $page) $pagination.= "<span class=\"current\">$counter</span>"; else $pagination.= "<a href=\"$targetpage?page=$counter\">$counter</a>"; } } } //next button if ($page < $counter - 1) $pagination.= "<a href=\"$targetpage?page=$next\">next »</a>"; else $pagination.= "<span class=\"disabled\">next »</span>"; $pagination.= "</div>\n"; } function findColor($n) { return ($n % 2 == 0) ? '#0026FF' : '#0094FF'; } // Create function to display the news entries function print_prospectEntry($arrEntry,$i) { // convert \n linebreaks to HTML formatted <br> breaks $arrEntry['comments'] = str_replace("\n", '<br>', $arrEntry['comments']); ?> <table style="background-color:<?php echo findColor($i); ?>" width="100%" border="0" cellspacing="0" cellpadding="0"> <tr> <td width="10"></td> <td width="300">Representative:</td> <td style="padding: 5px;"> <?php echo $arrEntry['representative']; ?> </td> </tr> <tr> <td width="10"></td> <td width="300">Company Name:</td> <td style="padding: 5px;"> <a href="prospect.php?prospect_id=<?php echo $arrEntry['prospect_id']; ?>"><?php echo $arrEntry['company_name']; ?></a> </td> </tr> <tr> <td width="10"></td> <td width="300">Phone Number:</td> <td style="padding: 5px;"> <?php echo $arrEntry['phone']; ?> </td> </tr> <tr> <td width="10"></td> <td width="300">First Name:</td> <td style="padding: 5px;"><?php echo $arrEntry['firstname']; ?> </td> </tr> <tr> <td width="10"></td> <td width="300">Last Name:</td> <td style="padding: 5px;"> <?php echo $arrEntry['lastname']; ?> </td> </tr> <tr> <td width="10"></td> <td width="300">Last Contacted:</td> <td style="padding: 5px;"> <?php echo $arrEntry['last_contact']; ?> </td> </tr> <tr> <td width="10"></td> <td width="300">Comments:</td> <td style="padding: 5px;"> <?php echo $arrEntry['comments']; ?> </td> </tr> </table> <br> <br> <?php } // Get all news entries $prospectEntries = mysql_query('SELECT * FROM prospects ORDER BY prospect_id DESC ' . $queryLimit) or trigger_error(mysql_error()); $i = 1; while ($prospectEntry = mysql_fetch_array($prospectEntries)) { // Your while loop here print_prospectEntry($prospectEntry,$i++); // when printing here, it does 5 results per page like I want, however it just prints the same record //echo $row; } ?> <?php echo $pagination; ?> </body> </html>
-
Counting the number of times something is in the table
jcbones replied to Potatis's topic in PHP Coding Help
Try something like this: Un-tested of course. <?php include('includes/admin_session.php'); ?> <?php require_once("includes/connection.php"); // Set variables from search query $tutor_name = $_POST['tutor_name']; $school_name = $_POST['school']; $term = $_POST['term']; $year = $_POST['year']; // Query database with the above variables to get all stored data belonging to the tutor, at X school during X term and X year $result = mysqli_query($connection,"SELECT * FROM student_roll WHERE tutor_name='$tutor_name' AND school='$school_name' AND term='$term' AND year='$year' ORDER BY last_name ASC") or die(mysqli_error($connection)); while($row = mysqli_fetch_array($result)) { //get first and last name of all students belonging to tutor at X school, term and year $student_first_name= $row['first_name']; $student_last_name= $row['last_name']; // Use student names to loop through table the names of all students who had a lesson on week 1 and display 1 // as the number of lessons they had. $result = mysqli_query($connection,"SELECT * FROM student_roll WHERE first_name='$student_first_name' AND last_name='$student_last_name'") or die(mysqli_error($connection)); if(mysqli_num_rows($result)) { while($row = mysqli_fetch_array($result)) { //set students in an array, so we increment a count to keep total rows. $students[$student_first_name][$student_last_name] = 0; if($row['week1'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week2'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week3'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week4'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week5'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week6'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week7'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week8'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week9'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } if($row['week10'] == 'y') { $students[$student_first_name][$student_last_name] += 1; } } } } if(is_array($students)) { foreach($students as $fname => $dem2) { foreach($dem2 as $lname => $count) { echo $fname . " " . $lname . ": "; echo $count; echo "<br />"; echo "<br />"; } } } else { echo ucwords(strtolower($tutor_name)) . ' does not have any students to display!'; } ?> -
Date help...pulling info from db and seperating into 3 fields
jcbones replied to shane85's topic in PHP Coding Help
How is it stored? //data stored m-d-y; $date = explode('-',$db_data); $date[0] = m; $date[1] = d; $date[2] = y; -
Notice: A session had already been started - ignoring session_start() in /www/zxq.net/p/a/i/paidtoclick/htdocs/data.php on line 2 = There is already a call to session_start(), this can be another script, or the server automatically starts the session. Notice: Undefined index: r in /www/zxq.net/p/a/i/paidtoclick/htdocs/data.php on line 11 = The key 'r' doesn't exist in the $_GET array. Notice: Undefined index: ucNick in /www/zxq.net/p/a/i/paidtoclick/htdocs/data.php on line 15 = The key 'ucNick' doesn't exist in the $_COOKIE array.
-
Try: $id = (isset($_GET['prospect_id'])) ? (int)$_GET['prospect_id'] : die('error!'); $qry = "UPDATE prospects SET representative='$representative', prospect_feeling='$prospect', firstname= '$f_name', lastname= '$l_name' WHERE prospect_id='$id'";
-
include ('database'); class blogPost { function __construc() { $this->db = new database(); } }
-
PHP redirect to error page has error on server but not localhost
jcbones replied to shortysbest's topic in PHP Coding Help
Make sure there is no spaces before <?php -
Try something like this: <?php // Contact subject $subject ="Customer Quote Form"; // Details $name = (isset($_POST['name'])) ? strip_tags(ucwords(strtolower($_POST['name']))) : NULL; // Mail of sender $mail_from= (isset($_POST['customer_mail'])) ? strip_tags($_POST['customer_mail']) : NULL; $info['Customer Phone'] = (isset($_POST['phone'])) ? strip_tags($_POST['phone']) : 'Phone number not listed'; $info['Piano Make and Model'] = (isset($_POST['make'])) ? strip_tags($_POST['make']) : 'Make & Model not listed.'; $info['Piano Size'] = (isset($_POST['size'])) ? strip_tags($_POST['size']) : 'Size not listed.'; $info['Stairs'] = (isset($_POST['stairs'])) ? (int) $_POST['stairs'] : 0; $info['Estimated Mileage'] = (isset($_POST['mileage'])) ? (int) $_POST['mileage'] : 0; $info['Truck Access'] = (isset($_POST['access']) && strtolower($_POST['access']) == 'yes') ? 'Yes' : 'No, or not stated.'; $info['Other Details'] = (isset($_POST['detail'])) ? strip_tags($_POST['detail']) : NULL; $message = 'Customer: ' . $name . PHP_EOL; foreach($info as $key => $value) { $message .= $key . ': ' . $value . PHP_EOL; } // From $header="from: $name <$mail_from>"; // Enter your email address $to ='<my email here>'; $send_contact=mail($to,$subject,$message,$header); // Check, if message sent to your email // display message "We've recived your information" if($send_contact){ header('Status: 200'); header('Location: thankyou.php'); //This should be a full URI as in 'Location: http://mysite.com/thankyou.php' } else { $comment = "Error: Please email us directly: <customer email here>."; } ?><!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta content="text/html; charset=utf-8" http-equiv="Content-Type" /> <title>Contact Form</title> </head> <body> <?php echo $comment; ?> </body> </html>
-
Using the above syntax def. $qry = "UPDATE prospects SET representative='$representative', prospect_feeling='$prospect', firstname= '$f_name', lastname= '$l_name'"; //You would probably want to add a WHERE clause onto that, or every row in the table will update.
-
Same way you would with HTML. As you see, HTML is a hypertext language that you MUST use for the browser to correctly display a website. PHP outputs anything you want, but if you want a website to look correct, PHP will have to output HTML. Therefore, a favicon file MUST be included in the head section of the HTML output that reaches the browser.
-
//Should be $doc->save($xmlfile);