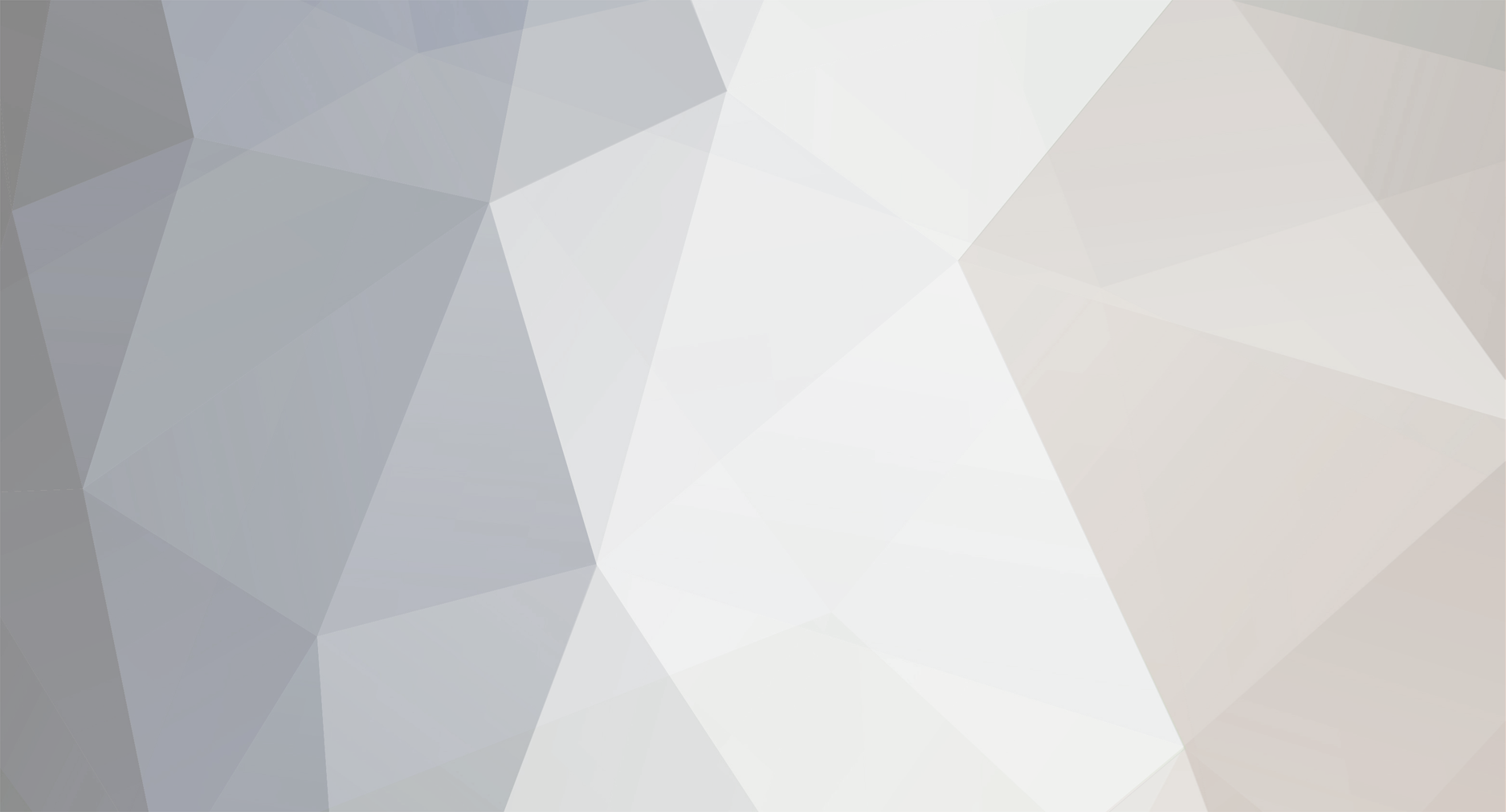
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
Getting data from mysql and then converting it to json with php
jcbones replied to borden0108's topic in PHP Coding Help
Your query is failing, this should tell you why. <?php error_reporting(-1); ini_set('display_errors',1); $link = mysql_connect('localhost', 'root', ''); if (!$link) { die('Could not connect: ' . mysql_error()); } echo 'Connected successfully<br />'; $arr = array(); $rs = "SELECT `id`, `title`, `author`, `date`, `imageUrl`, `text` FROM `items`"; $rs_return = mysql_query($rs) or trigger_error($rs . ' has encountered an error: <br />' . mysql_error()); while($obj = mysql_fetch_array($rs_return)) { $arr[0] = $obj['id']; $arr[1] = $obj['title']; $arr[2] = $obj['author']; $arr[3] = $obj['date']; $arr[4] = $obj['imageUrl']; $arr[5] = $obj['text']; } //numbering the array indexes, will only return 5 array indexes EVER. All indexes will be over written on each loop. echo '{"items":'.json_encode($arr).'}'; ?> -
look at your page source, that should tell you if the PHP variable is working. Other than that, you should be using CSS, and not depreciated attributes.
-
Landing page looks good, and clean. Little bit of alignment problem in FF5 on the right side of the lower division. I also love a site that flows, and holds it's design throughout. So the products page should mimic the landing page in it's overall design. As it stands you have borders on the products page, and the divisions don't quite line up like they do on the Landing page. On the products page, I don't like having to scroll to see all the info. I think it would be better if you had some javascript tabs for the features, etc. Same goes for the menu. The colors are not a bad combination, and very easy on the eyes. I would look into smoothing the site out a little, just seems to boxy. Use some CSS to round off those corners. Of course, this is all my opinion!
-
I don't "see" anything that would cause an error, unless the data you are pulling has an illegal character in it. You will have to use some de-bugging tools (echo mainly) to find it. Or look at the page source.
-
What is the error?
-
I would suggest writing one query to do the work you have two doing. It eliminates the headaches. Un-tested: SELECT a.CITYID, b.City FROM Address AS a JOIN Cities AS b ON a.CITYID = b.CITYID GROUP BY a.CITYID ORDER BY b.City ASC It can be tricky (sometimes) getting a group by and an order by running on the same query. So let us know if that doesn't work, we may have to go with a sub-query to get it working correctly.
-
Un-Tested: <?php error_reporting(-1); ini_set('display_errors',1); include('mysql_connection_info.php'); if(isset($_POST['submit'])) { $state = mysql_real_escape_string(trim($_POST['state'])); $county = mysql_real_escape_string(trim($_POST['county'])); foreach($_POST['city'] as $value) { if(empty($value)) { continue; } $city = mysql_real_escape_string($value); $parts[] = "('$state','$county','$city')"; } if(is_array($parts)) { $sql = "INSERT INTO locations (state,county,city) VALUES " . implode(',',$parts); if(mysql_query($sql)) { echo 'Successful<br />'; } else { echo $sql . ' has encountered an error: <br /> ' . mysql_error(); } } else { echo 'You must submit at least one city.'; } ?> <form action="" method="post"> <input type="text" name="state" id="state" placeholder="State" /><br /> <input type="text" name="county" id="county" placeholder="County" /><br /> <?php for($i = 0; $i < 5; $i++) { echo '<input type="text" name="city[]" id="city' . $i . '" placeholder="City" /><br />'; } ?> <input type="submit" name="submit" value="Submit" /> </form> Table structure CREATE TABLE IF NOT EXISTS `locations` ( `id` int(11) NOT NULL AUTO_INCREMENT, `state` varchar(100) NOT NULL, `county` varchar(100) NOT NULL, `city` varchar(100) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM ;
-
What are you looking for in the string? This function will have to be changed from a POSIX pattern to a PRCE pattern.
-
Reading comprehension is required to understand the manual.
-
http://www.php.net/manual-lookup.php?pattern=mysqli_stmt_close&lang=en&scope=404quickref
-
There is absolutely no reason to use globals. Using a standard variable, and passing arguments to functions is how it should be done. Passing a global to a function defeats the purpose of using a function, as you have just tied a specific variable to a function. Dropping that function into another script, will cause headaches, or a function re-write. All other super-global arrays are available in functions anyway ($_POST,$_GET,$_REQUEST), but due to the reasons stated above, shouldn't be used in functions. *hope I didn't mis-understand the question*.
-
Did you move your header call outside of the foreach loop, the table may not be finished updating before you select the rows.
-
Good Tut for PHP search results - Paged and Ordered
jcbones replied to mat3000000's topic in PHP Coding Help
You will need to do the calculations in MySQL, because you need to order by the distance. I would suggest http://www.goondocks.com/blog/08-01-22/zip_code_radius_search_using_mysql.aspx to start, and maybe http://www.scribd.com/doc/2569355/Geo-Distance-Search-with-MySQL to optimize. -
You need to echo the query out, and make sure everything is in it that needs to be. That is why you should store the query string in a variable, so you can echo the string out in case of an error. <?php $sql = "UPDATE checklist SET $fieldName = $val WHERE project = $id"; mysql_query($sql) or trigger_error("Query failed: <br /> $sql <br /> ERROR: <br />" . mysql_error());
-
Reading Image Code And Storing In Database
jcbones replied to N-Bomb(Nerd)'s topic in PHP Coding Help
I believe you should be using send_long_data() for this operation. -
Put your fieldnames in backticks (`), cause the hyphen seems to be causing an issue.
-
Try this: <?php // database connection info $conn = mysql_connect('dbhost, dbusername, dbpassword') or trigger_error("SQL", E_USER_ERROR); $db = mysql_select_db('inventorymanage',$conn) or trigger_error("SQL", E_USER_ERROR); if(isset($_POST['search'])) { $item = mysql_real_escape_string($_POST['item']); } elseif(isset($_GET['item'])) { $item = mysql_real_escape_string($_GET['item'); } else { $item = 'a'; } // find out how many rows are in the table $sql = "SELECT COUNT(*) FROM invsearch WHERE item_name LIKE '%$item%'"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); $r = mysql_fetch_row($result);$numrows = $r[0]; // number of rows to show per page $rowsperpage = 10; // find out total pages $totalpages = ceil($numrows / $rowsperpage); // get the current page or set a default if (isset($_GET['currentpage']) && is_numeric($_GET['currentpage'])) { // cast var as int $currentpage = (int) $_GET['currentpage']; } else { // default page num $currentpage = 1;} // end if // if current page is greater than total pages... if ($currentpage > $totalpages) { // set current page to last page $currentpage = $totalpages;} // end if// if current page is less than first page... if ($currentpage < 1) { // set current page to first page $currentpage = 1;} // end if // the offset of the list, based on current page $offset = ($currentpage - 1) * $rowsperpage; // get the info from the db $sql = "SELECT Item_name, system, item_num, price FROM invsearch WHERE item_name LIKE '%$item%' ORDER BY item_name LIMIT $offset, $rowsperpage"; $result = mysql_query($sql, $conn) or trigger_error("SQL", E_USER_ERROR); // while there are rows to be fetched... echo "<div>\n<form action=\"\" method=\"post\">\n<label for=\"item\">Search</label>\n <input type=\"text\" name=\"item\" id=\"item\" /> <input type=\"submit name=\"submit\" value=\"Search\" />\n </form>\n<table border='2' cellpadding='10' align='center'>"; while ($list = mysql_fetch_assoc($result)) { // echo data echo "<tr>"; echo "<td width='500px'>"; echo "<p style=margin:0px><font color='black' size='5px'>" . $list['Item_name'] . "</font></p>"; echo "<p style=margin:0px><font color='black' size='3px'>" . $list['system'] . "</font></p>"; echo "<p style=margin:0px><font color='black' size='2px'>Item Number: " . $list['item_num'] . "</font></p>"; echo "</td>"; echo "<td><font color='red' size='3px'>" . $list['price'] . "</font></p"; echo "</td>"; echo "</tr>"; } echo "</table>"; echo "<p align='center'>"; // end while/****** build the pagination links ******/ // range of num links to show $range = 3; // if not on page 1, don't show back links if ($currentpage > 1) { // show << link to go back to page 1 echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=1&item=$item'>First<<</a> "; // get previous page num $prevpage = $currentpage - 1; // show < link to go back to 1 page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$prevpage&item=$item'>Prev<</a> ";} // end if // loop to show links to range of pages around current page for ($x = ($currentpage - $range); $x < (($currentpage + $range) + 1); $x++) { // if it's a valid page number... if (($x > 0) && ($x <= $totalpages)) { // if we're on current page... if ($var == $currentpage) { // 'highlight' it but don't make a link echo " [<b>$x</b>] "; // if not current page... } else { // make it a link echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$x&item=$item'>$x</a> "; } }// end else } }// end if } // end for // if not on last page, show forward and last page links if ($currentpage != $totalpages) { // get next page $nextpage = $var + $currentpage + 1; // echo forward link for next page echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$nextpage&item=$item'>Next></a> "; // echo forward link for lastpage echo " <a href='{$_SERVER['PHP_SELF']}?currentpage=$totalpages&item=$item'>Last>></a> "; } echo "</P>"; // end if/****** end build pagination links ******/ ?>
-
Sometimes the http:// protocol will fail because sites require that a user_agent exist. Since PHP defines this user_agent string as empty, then you would need to make sure it is populated.
-
You may need to check and make sure your host has fopen wrappers enabled.
-
How about: [/code] $fact_sent = '<input type="checkbox" ' . ($row['fact-sent'] == true ? 'checked="checked"' : NULL) . ' />'; [/code] ETC...
-
$nfri = strtotime("next Friday");
-
Because you are storing post as a varchar, and it should be stored as an integer. If you look at the table I showed you, you would see that it is set as an integer.
-
That puts it about as plain as you would want to get. Lots of different jargon out there, but this is clear and concise and to the point. A lot of other variables go with it, but this is the general breakdown.
-
I set up a test database, with your values entered in, and ran it, coming up with the correct values. db table -- -- Table structure for table `rank` -- CREATE TABLE IF NOT EXISTS `rank` ( `id` int(11) NOT NULL AUTO_INCREMENT, `post` int(11) NOT NULL, `rank` varchar(20) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM DEFAULT CHARSET=latin1 AUTO_INCREMENT=6 ; -- -- Dumping data for table `rank` -- INSERT INTO `rank` (`id`, `post`, `rank`) VALUES (1, 0, 'Noob'), (2, 10, 'Member'), (3, 50, 'Metal'), (4, 100, 'Silver'), (5, 200, 'Gold'); Query SELECT rank FROM `rank` WHERE post <= 55 ORDER BY post DESC LIMIT 1
-
<?php function userRank($userRank) { $rank = array(0=>'Noob',10=>'Member',50=>'Metal',100=>'Silver',200=>'Gold'); foreach($rank as $k => $v) { if($userRank > $k) { $ranking = $v; } else { break; } } return $ranking; } Although mysql will work.