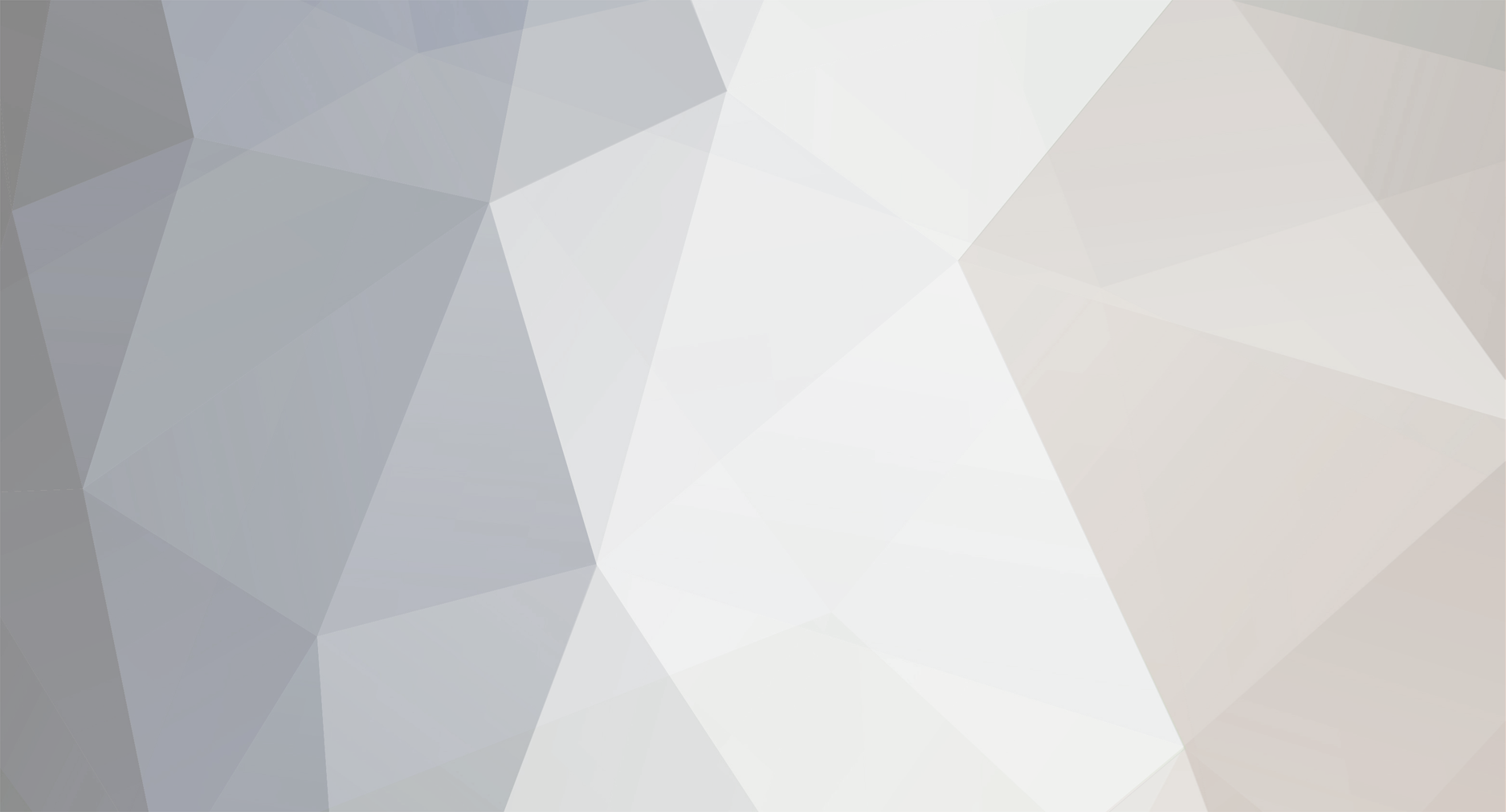
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
Problem with error message when login
jcbones replied to adhbvklwqdbviabjiawdnbij's topic in PHP Coding Help
How do you expect PHP held data to appear on a page without any PHP on it? index.php <?php session_start(); if(isset($_SESSION['ERRMSG_ARR'])) { $errors = implode('<br />',$_SESSION['ERRMSG_ARR']); }else { $errors = NULL; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Login</title> <link href="loginmodule.css" rel="stylesheet" type="text/css" /> </head> <body> <h1>Login</h1> <form id="loginForm" name="loginForm" method="post" action="actions/login.php"> <table width="300" border="0" align="left" cellpadding="2" cellspacing="0"> <tr> <td width="112"><b>Email</b></td> <td width="188"><input name="email" type="text" class="textfield" id="email" /></td> </tr> <tr> <td><b>Senha</b></td> <td><input name="password" type="password" class="textfield" id="password" /></td> </tr> <tr> <td></td> <td><p><a href="resend_password.php">Esqueceu sua senha?</a></p></td> </tr> <tr> <td> </td> <td><input type="submit" name="Submit" value="Login" /> <?php echo $errors; ?></td> </tr> </table> </form> </body> </html> -
Got to include some whitespace in there: $offset = strpos($data["content"], ' ', 15) ;
-
This will get you started: <?php //include connection info. include('config.php'); //point to file that holds database connection info. //function to show all results from a table. function browseData($tbl) { $result = mysql_query("SELECT * FROM `$tbl`"); $output = NULL; $output .= mysql_num_rows($result) . " Results!"; $c = mysql_num_fields($result); $output .= "<table class=\"search\">"; for ($i=0; $i < $c; $i++) { $output .= '<th style="background-color:blue;">'.ucwords(mysql_field_name($result, $i)).'</th>'; } $d = 1; while($r = mysql_fetch_array($result)) { $output .= '<tr style="background-color:'; $output .= (($d % 2) == 0) ? '#ACCDE2;' : '#0088E2;' ; $output .= '">'; $i = 0; while($i < $c) { $output .= "<td>" . $r[$i] . "</td>"; $i++; } $output .= "</tr>"; $d++; } $output .= "</table><br/><br/>"; return $output; } //call the function, and print the results to the page. $db_table = 'test'; //Your table name. echo browseData($db_table); ?> This is an old function that will get the results from a specific table in your database. You will have to include your database connection file, and edit the $db_table variable. For editing, I would link the row id to a script that would use the database to populate a form for editing.
-
Change the while statement to a foreach: foreach($_POST['company'.$val] as $ic) {
-
I would remove the id=$id part, and just ban on the masterplayerid, that way, every account would be banned, and not just one row.
-
You should be developing with error's own full. error_reporting(E_ALL); ini_set('display_errors',1);
-
Still not quite there, but closer: <?php $search = "PLEl25o5"; $swap=array('1'=>"l", '5'=>"s",'0'=>"o","l"=>'1',"s"=>'5',"o"=>'0'); //all entries must be strings in order for in_array to work. $len = strlen($search); //get length of search parameters. $arr = array(); //new array. $searched = array_pad($arr,$len,$search); //fill the array with the search according to the string length. for($i = 0, $index = 1; $i < $len; $i++) { //for loop. $letter = substr($search,$i,1); //get current letter. if(in_array($letter,$swap,true)) { //if it is in the swap array. $key = array_search(substr($search,$i,1),$swap,true); //get the key. $searched[$index][$i] = $key; //store the new search to the searched array. ++$index; } } $query="('". implode("' or '",$searched)."')"; echo $query; ?> OUTPUT: ('PLEl25o5' or 'PLE125o5' or 'PLEl2so5' or 'PLEl2505' or 'PLEl25os' or 'PLEl25o5' or 'PLEl25o5' or 'PLEl25o5')
-
2 where's in a query will fail.
-
Really vague there. We don't know what MASTERPLAYERID is, where it is stored, or how it is populated. If you ban a player in the players table, and the database is normalized, then you should have no problem enforcing the ban anywhere in the script.
-
http://php.net/manual/en/function.date-default-timezone-set.php It should be set at the top of the script, then all date functions used in the script would be based on the set timezone. supported timezones
-
$match_time = date('Y-m-d H:i:s',time() - $time_count); //should work, but Un-tested
-
You will most likely have to modify the `hosts` file on your computer. Here is a tutorial on how to do that: http://support.apple.com/kb/TA27291 Here is why you have to do that: Scroll to Resolving DNS issue
-
Impossible! You should get an array on that print_r, as I changed the variable it pointed to. Something else is wrong if that is it's actual output.
-
<?php $_POST['qty'][] = 3; $_POST['qty'][]=4; if(!empty($_POST['qty'])) { $item_count = COUNT($_POST['qty']) - 1; } echo $item_count; //should echo 1; ?>
-
What does: echo '<pre>' . print_r($_POST['company'.$val],true) . '</pre>'; return?
-
What makes you think there is a problem with that line?
-
Post exactly what that print_r function outputs to the screen. echo '<pre>' . print_r($ic,true) . '</pre>';
-
Try this: <?php $doc = new DomDocument(); $doc->validateOnParse = true; if(!$doc->load("users/users.xml")) { exit('Error occured!'); } $userList = $doc->getElementsByTagName('username'); $userCnt = $userList->length; for ($idx = 0; $idx < $userCnt; $idx++) { echo 'Username: ' . $userList->item($idx)->nodeValue . "<br />"; } ?>
-
insert multiple database table row from dynamic checkboxes from db
jcbones replied to sir_amin's topic in PHP Coding Help
Remove the array from the following input element. It isn't in a while loop so there is no need for it, and it should cure your problems. <input name="lid[]" type="text" id="lid[]" value="<?php echo $row_rslesson['lid']; ?>" size="32" readonly="readonly" /> Change to: <input name="lid" type="text" id="lid" value="<?php echo $row_rslesson['lid']; ?>" size="32" readonly="readonly" /> -
remove the line break to br function in your code. nl2br()
-
The simplest way is to use the simpleImage class available from white-hat. It is free, and works well. The fastest way, is to use imageMajik, but not all servers have that installed. SimpleImage ImageMajik
-
Post a database table structure dump of the `teams` table. If using phpMyAdmin, choose table, goto Export , un-check everything but 'Structure', make sure you DO NOT check 'Save as File'. Copy and paste what is printed to the screen. Post it here.
-
Try adding de-bugging to see where you are going wrong. $sales=$row[wfdollarsales]; echo 'Sales starts as:' . $sales . '<br />'; $sales = preg_replace("/[^a-zA-Z0-9\s]/", "", $sales); echo 'After preg_replace, it is now: ' . $sales . '<br />'; $sales = intval($sales); $salesly=$row[wfdollarsalesly]; echo 'Salesly starts as: ' . $salesly . '<br />'; $salesly = preg_replace("/[^a-zA-Z0-9\s]/", "", $salesly); echo 'After preg_replace it is now: ' . $salesly . '<br />'; $salesly = intval($salesly); $subtraction=$sales-$salesly; echo 'Sales minus Salesly = ' . $subtraction . '<br />'; $percent=$subtraction/$salesly; echo 'Subtraction divided by salesly = ' . $percent . '<br />'; Using that, I think you will see where you are going wrong.