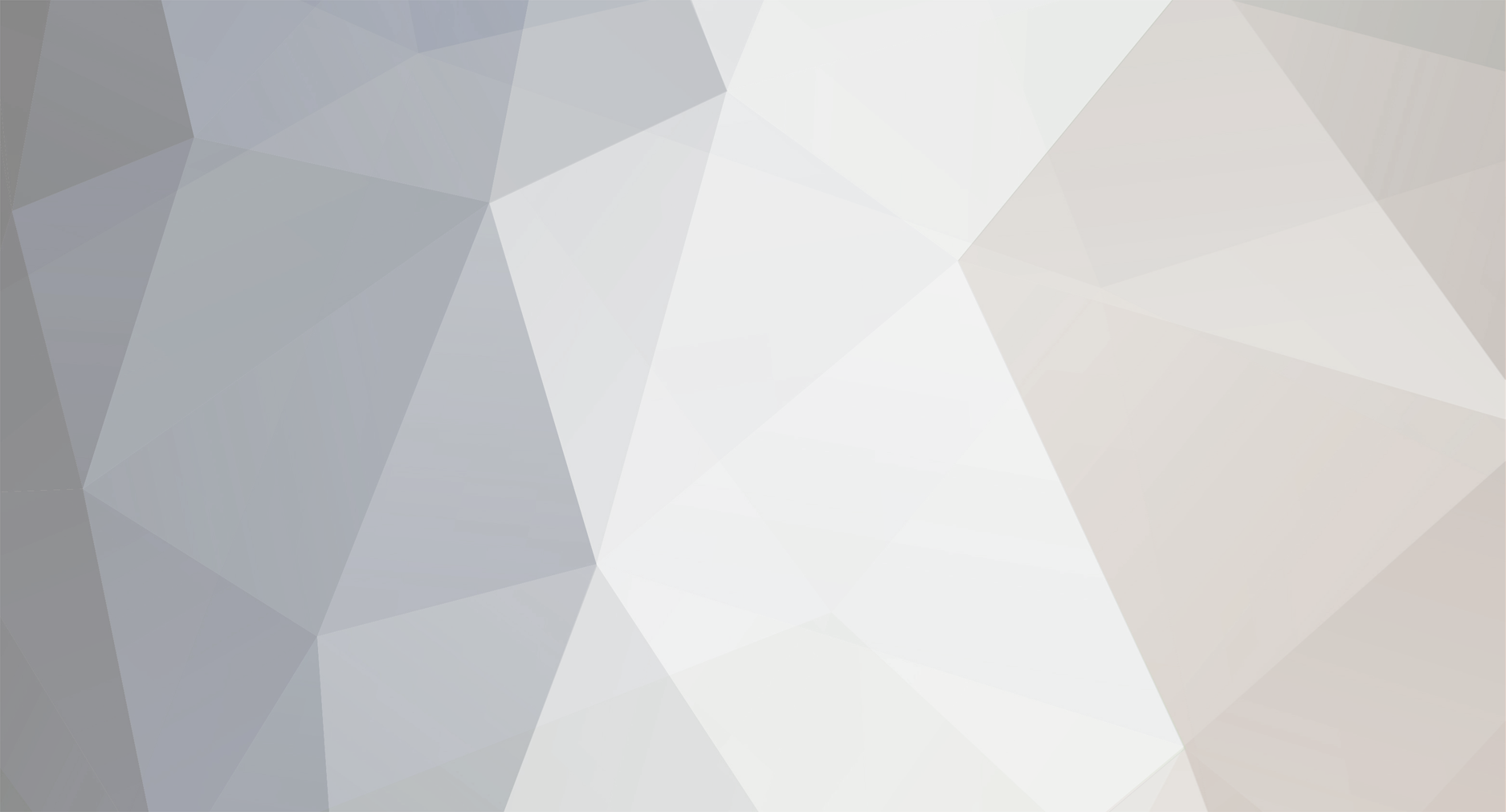
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
Try running this: Let us know what the error says. <?php error_reporting(E_ALL); ini_set('display_errors',1); if(!$_POST) exit; $email = $_POST['email']; //$error[] = preg_match('/\b[A-Z0-9._%-]+@[A-Z0-9.-]+\.[A-Z]{2,4}\b/i', $_POST['email']) ? '' : 'INVALID EMAIL ADDRESS'; if(!eregi("^[a-z0-9]+([_\\.-][a-z0-9]+)*" ."@"."([a-z0-9]+([\.-][a-z0-9]+)*)+"."\\.[a-z]{2,}"."$",$email )){ $error.="Invalid email address entered"; $errors=1; } if($errors==1) echo $error; else{ $values = array ('name','email','message'); $required = array('name','email','message'); $your_email = "myaddress@gmail.com"; $email_subject = "New Message: ".$_POST['subject']; $email_content = "new message:\n"; foreach($values as $key => $value){ if(in_array($value,$required)){ if ($key != 'subject' && $key != 'company') { if( empty($_POST[$value]) ) { echo 'PLEASE FILL IN REQUIRED FIELDS'; exit; } } $email_content .= $value.': '.$_POST[$value]."\n"; } } if(mail($your_email,$email_subject,$email_content) !== false) { header('Status: 200'); //for Chrome. header("Location: http://www.temporary.com"); } else { echo 'ERROR!'; } } ?>
-
Because date() expects a format for the first argument. Try: <?php $T_End_Date = date('Y-m-d', strtotime("+ $P_Days days",$T_Start_Date));
-
Two ways off the top of my head: <?php //using date function. (not recommended, cause I think it is dirty). $timestamp = '2011-08-22 18:02:00'; //notice the timestamp change from the format provided. $time = strtotime($timestamp); $month = date('m',$time); $day = date('d',$time); $year = date('Y',$time); $hour = date('H',$time); $minute = date('i',$time); $second = date('s',$time); echo 'Month: ' . $month . '<br />Day: ' . $day . '<br />Year: ' . $year . '<br />Hour: ' . $hour . '<br />Minute: ' . $minute . '<br />Second: ' . $second . '<hr />'; //using explode $timestamp = '08-22-2011 18:02:00'; $split = explode(' ',$timestamp); list($month, $day, $year) = explode('-',$split[0]); list($hour, $minute, $second) = explode(':',$split[1]); echo 'Month: ' . $month . '<br />Day: ' . $day . '<br />Year: ' . $year . '<br />Hour: ' . $hour . '<br />Minute: ' . $minute . '<br />Second: ' . $second;
-
Pick an item from database based on a number
jcbones replied to joecooper's topic in PHP Coding Help
Run it like a real lotto, where the jackpot stacks up until someone wins. Then you could generate a random number to put into the row, or let them pick their own number. If two numbers come up, they split the prize, or make the row unique, kicking it back if it fails. If you MUST have a winner, draw until you find one. -
Yep, I think that is exactly what Mj wants. If the page was 4 sub directories deep, getting the web_root directory wouldn't do that page a bit of good. Therefore, you always need to make sure the page knows EXACTLY where it resides.
-
Please post your form, inside the bbcode [ code ] [ /code ] tags! In the meantime, try changing. $part1 .= sprintf("%20s: %s\n", $fields[$a], $b); To: $body .= sprintf("%s: %s\n", $fields[$a], $b);
-
The best way to get help is to post all of the relevant code. Being that you are using PHP (PRE-HYPERTEXT PROGRAMMING), looking at the end product doesn't help with a coding problem. I would start with echo'ing out the $query, and pasting that into my database program (ie. phpMyAdmin) or typing it straight into the console. This would remove the doubts as to what rows are returned.
-
.htaccess is very powerful, you can allow or deny any domain you wish to, to whatever folder you wish.
-
Then go Here
-
Yes, you can restrict anything using an IP address from getting your images. Scroll down to "blocking bandwidth leeches" To find the bandwidth leeches, look at your server logs to see which sites are hitting your images.
-
1.) Why is using a Regex "un-needed overhead"? 2.) No, I'm trying to learn how to do something multiple ways. 3.) I prefer Regex because I can do in one line what code usually takes several likes and often isn't as thorough. So, now I know how to do what I want using PHP functions and code, but I'd still be curious to see how to do this using Regex, because it seems like a very common need?! Debbie I ran a regex on a string containing 93 characters, and then ran the same string through strlen AND trim. Here is the results. This is a string that contains more than 2 characters but less than 100 characters in length. 10,000 runs of preg_match takes 0.0234 seconds 10,000 runs of strlen() and trim() takes 0.0096 seconds.
-
So use: $variable = trim($variable); $length = strlen($variable); if($length > 1 && $length < 101) { //do something } You are trying to use a function, for no other reason than you WANT to use it. Even though it would be using un-needed overhead.
-
How about: if (strlen($trimmed['htmlTitle']) > 2 && strlen($trimmed['htmlTitle']) < 100){ $htmlTitle = $trimmed['htmlTitle']; }else{ $errors['htmlTitle'] = 'HTML Title must be 2-100 characters (A-Z \' . -)'; } strlen()
-
If you look at the manual http://php.net/manual/en/function.mysql-result.php You would find that you are NOT getting the first row in your results, because that first row starts at 0. So you will have to start $x = 0; Then change: echo ($x % 3 == 0)? "</tr><tr>" : ""; To echo ($x > 0 && ($x % 3 == 0))? "</tr><tr>" : "";
-
With the few rows of data you gave, this query returned one row of data for each athlete_id, based on the highest date in the programs_for_atheletes table.
-
Sorry, I plugged in just a couple of returns so that I could see everything without having to scroll. Just change the column selection to *, but make sure to leave the MAX() in place. SELECT a.*, MAX(p.date) AS `mdate`, p.* FROM t_athletes AS a JOIN programs_for_athletes AS p ON a.athlete_id = p.athlete_id_prog GROUP BY a.athlete_id
-
You may do it how you wish, but I coded a function to be used as a function should. Doing it your way, would defeat the purpose of making it a function. <?php $result=mysql_query('select `date` from `specialevent`'); while($row=mysql_fetch_assoc($result)) { $days[] = date('l',strtotime($row['date'])); } //Start counting at 7am, count till 5pm, for 20 hours, skip monday. $updated_time = strtotime('+' . hoursLeft($database_date,7,17,20,$days) . ' hours',strtotime($database_date)); echo date('m-d-Y, H:i:s',$updated_time); ?>
-
Do you see how this query gets it's info? BTW, it is very in-secure, I would suggest looking into sanitation and validation of client provided data. (another topic). $query = "SELECT * FROM seniors WHERE sen_id=$_GET[sen_id]"; //query gets it's info from the _GET array (URL). How all of this info is past between pages, is in the URL you have a "?sen_id=2". Where 2 is the row number (name) you are looking for from the database. So, these pages actually only send 1 bit of info between them, in order to get ALL of the info you wish to see. The above code, with the lightbox removed. echo "<form action ="/tally.php?sen_id=$row[sen_id]" method="post"> $row[first_name] $row[last_name] - $row[school] <br /> <input name="submit" align="right" type="submit" value="Vote" > </form><br/>"; I think that is it.
-
Simple problems, need simple changes, but over-analyzing leads to headaches. This should address your issues, and I hope it works 100%. I've tested it, but not in every way possible. Changes MARKED <?php function hoursLeft($time,$work_starts,$work_ends,$time_to_email,$skip_days = array()) { //ARGUMENTS CHANGED!!! $parts = explode(' ',$time); //split the time off of the date. list($hour, $minute, $second) = explode(':',$parts[1]); //get the hours, minutes, seconds. if($minute == 0) { //if the minutes is over 0, then return a full hour for it $hours = $work_ends - $hour; } else { //otherwise, take into account that this isn't a full hour. $hours = ($work_ends - 1) - $hour; } $day_count = 0; //original day count is 0; for($i = $work_ends,$count = $hours; $count <= $time_to_email; $i++) { //Start the increment at 16, the count at the current hours, keep the count below 25, increment on each loop. if($i > 24) { //if the increment is over 24, reset it to 0. $i = 1; ++$day_count; //increment day_count when i goes over 24 hour limit. if(!empty($skip_days)) { //if you want to skip weekends CHANGED FROM BOOLEAN, TO IS NOT EMPTY. $day = date('l',strtotime("+ {$day_count} days",strtotime($time))); //get the full text of the current day the hours are pointing to. if(in_array($day, $skip_days)) { //and current day is in the weekend. CHANGED ARGUMENTS TO in_array() FUNCTION. $hours += 24; //add 24 hours to the count. $i = 24; continue; //restart loop, before any counting takes place. } } } if($i > $work_starts && $i <= $work_ends) { //if the increment is between 7 and 16, add to the count, which will break the loop at 24. $count += 1; } $hours += 1; //add to the hours, the loop breaks at a count of 24, which will give us the total hours to add to the updated_time above. } return $hours; //return hours } ?> How to use This function returns an integer. This integer represents hours based on the information you feed the function. Arguments to the function are as follows. 1. A database styled timestamp. (YYYY-mm-dd H:i:s) 2. Time of day to start counting (integer 24 hour clock) 3. Time of day to stop counting (integer 24 hour clock) 4. Amount of time you wish to count (integer hours) 5. Days you wish to skip (array OPTIONAL) Uses <?php $database_date = '2011-08-12, 07:00:00'; //this comes from your database, //Start counting at 7am, count till 5pm, for 20 hours, no skip days. $updated_time = strtotime('+' . hoursLeft($database_date,7,17,20) . ' hours',strtotime($database_date)); echo date('m-d-Y, H:i:s',$updated_time); //Start counting at 7am, count till 5pm, for 20 hours, skip monday. $updated_time = strtotime('+' . hoursLeft($database_date,7,17,20,array('Monday')) . ' hours',strtotime($database_date)); echo date('m-d-Y, H:i:s',$updated_time); //Start counting at 7am, count till 5pm, for 20 hours, skip monday and wednesday. $updated_time = strtotime('+' . hoursLeft($database_date,7,17,20,array('Monday','Wednesday') . ' hours',strtotime($database_date)); echo date('m-d-Y, H:i:s',$updated_time); ?> Hope this helps.
-
Once data is passed back to the script from a database, you can manipulate that data with any and all functions of the programming language you are using.
-
The easiest way for us to understand the error, is to copy/paste it.
-
There are a few problems that hinder you from getting replies. You haven't provided any functions/templates, nor what is required for that template. Since we don't have access to your template, we aren't real sure how to restructure it, to suit your needs. I can offer some advice though. Get the design of your mobile site finished, but put placeholders in it for where you want your data to show up. If you want an image somewhere, put {{IMAGE}}, or a name somewhere put {{NAME}}. This way you will get to see the design of the site, and get it like you want. Next. Now you can use PHP to get the data where you want the placeholders. In the last script you posted, everything that is returned from the database is contained in these few lines: <h3><?php echo $row['first_name'], " ", $row['last_name'], " has <font style=\"color:#FF0000\"> $row[votes]</font> votes";?></h3> Everything in $message is concerning database interactions, but is contained inside the script.
-
I would suggest MAX() along with GROUP BY, something like: SELECT a.athlete_id, a.athlete_id, MAX(p.date) AS `date`, p.program_link FROM t_athletes AS a JOIN programs_for_athletes AS p ON a.athlete_id = p.athlete_id_prog GROUP BY a.athlete_id
-
If you cannot create a user in the script provided, that script should tell you why.