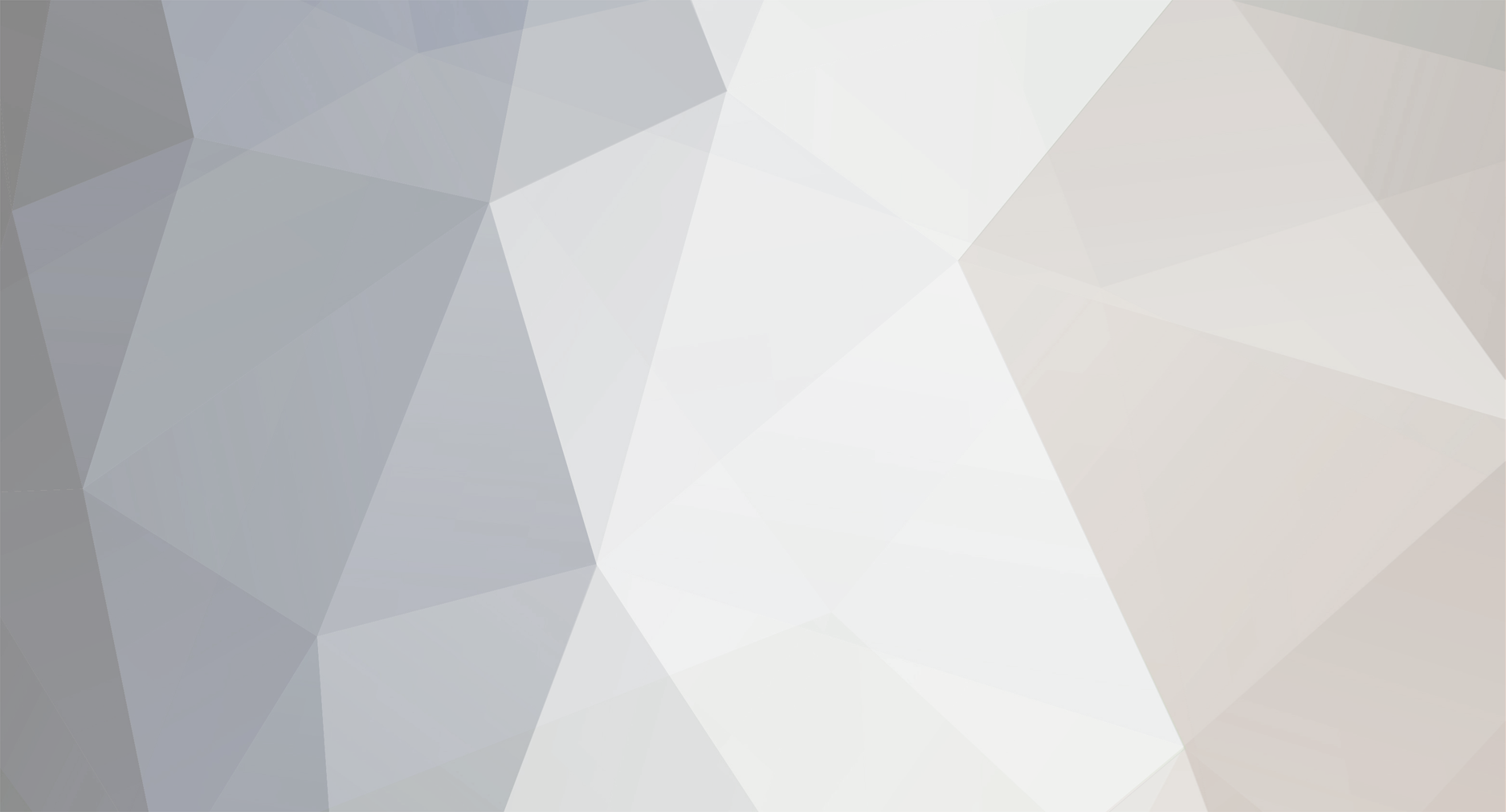
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
Second thought, wrap this in intval() function. $split = (int) $num/2; Should be: $split = intval($num/2); //gets rid of decimals.
-
An alternative suggestion would be to array_pop(). <?php while(!empty($list)) { $newsData = file("news/". array_pop($list)); $newsTitle = $newsData[0]; $submitDate = $newsData[1]; unset ($newsData['0']); unset ($newsData['1']); $newsContent = ""; foreach ($newsData as $value) { $newsContent .= $value; } echo "<tr><th align='left'><h2>$newsTitle</h2></th><th align='right'>$submitDate</th></tr>"; echo "<tr><td colspan='2'>".$newsContent."<br/><hr size='1'/></td></tr>"; } ?> You could also sort the array based off of the filemtime(). This would be the best method, any others would possibly allow incorrect listings at times. Doing that, I would leave the foreach block intact, and change the function: <?php function getNewsList(){ $fileList = array(); // Open the actual directory if ($handle = opendir("news")) { // Read all file from the actual directory while (($file = readdir($handle)) !== false) { if (!is_dir($file)) { $fileList[filemtime('news/'.$file)] = $file; } } } krsort($fileList); return $fileList; } ?>
-
This will cause it to fail: elseif ($password == $repassword) Should be: elseif ($password != $repassword) I would suggest using what MJ gave you, it is high quality!
-
<?php // Get categories $sql = "SELECT catid, catname AS catname FROM $t_cats WHERE enabled = '1' $sortcatsql"; // Version 5.0 $res = mysql_query($sql); $num = mysql_num_rows($res); $split = (int) $num/2; $i = 0; while ($row = mysql_fetch_array($res)) { echo '<div style="width:48%;float:left">'; ?> <a href="?view=post&cityid=<?php echo $xcityid; ?>&catid=<?php echo $row['catid']; ?>&shortcutregion=<?php echo $_GET['shortcutregion']; ?>"><?php echo $row['catname']; ?></a><br> <?php echo ($split == ++$i) ? '</div><div style="width:48%;float:left">' : NULL; } echo '</div>'; ?> Let us know!
-
Oh, we will help you understand things better. Sessions store a file on the server that contains all of the data you wish to store for a short period of time. You can access that data in an array. In order for the server to know what data to make available, it will set a cookie in the clients browser that contains a value that matches the file name the data is inside. There is only 2 criteria to using sessions. 1. Start the session: <?php //this line MUST be called before ANY output to the page: session_start(); 2. You must save data to the session: <?php //this line MUST be called before ANY output to the page: session_start(); $_SESSION['started'] = 'Yes, you have started a session.'; Now to use that session, is just as simple. <?php //this line MUST be called before ANY output to the page: session_start(); //call the session: if(!empty($_SESSION['started'])) { echo $_SESSION['started']; //You should get an echo, on your first page refresh. } else { $_SESSION['started'] = 'Yes, you have started a session.'; echo 'Session is not showing up currently, please refresh the page.'; } ?>
-
I would set a session variable, 0 for fight ended, 1 for fight started. So then you could call it: if($_SESSION['fight'] == 0) { //there is no fight currently, lets start one> $_SESSION['fight'] = 1; } if($_SESSION['fight'] == 1) { //Someone started a fight on the last page> //Do fight stuff. if($fight_is_finished) { //Let everyone know that fight is over. $_SESSION['fight'] = 0; } } If that even makes sense to you.
-
So why can you not just send the info to the function? In the above script, the function is called here: $updated_time = strtotime('+' . hoursLeft($database_date,7,16,24) . ' hours',strtotime($database_date)); //Handle the date: Where the function is set, the arguments are very explicit on what arguments it expects. function hoursLeft($time,$work_starts,$work_ends,$time_to_email,$skip_weekends = true) { //this function handles the count of how many hours you need to fulfill your task. The first parameter is the current time, in the form of a timestamp from the database. The second parameter is when the work day starts. The third parameter is when the work day ends. The fourth parameter is how many hours must elapse before the email goes out. The fifth parameter is an optional parameter, true skips weekends, false includes them. Default is true(skipped).
-
website is showing stuff thats not in the .php?
jcbones replied to dominic600's topic in PHP Coding Help
Does your host have a database prefix, and did you include it in your database connection? -
PHP Class - What is it for and when to use it
jcbones replied to paddy100's topic in PHP Coding Help
I also found this tutorial to be very helpful and informative. -
Select mysql & display in an html table using php
jcbones replied to skidmark10's topic in PHP Coding Help
I apologize for that, I'm gonna have to get more sleep, and quit being mean to people! -
Gonna need more clarification. 1. You have special events. 2. Why do you need to make special events skip weekends (should be a hard date). 3. How does the special events interact with this script.
-
<?php $month = intval($_POST['month']); $day = intval($_POST['day']); $year = intval($_POST['year']); $store_in_database_as = $year . '-' . $month . '-' . $day; ?> On second thought, there is no need to store as a timestamp or datetime, using a date column would be just fine.
-
In order to sort by a distance within MySQL, you would need to build the function inside MySQL. There are ways to do that, but they can get confusing. This tutorial should get you started, although you will have to make note that he isn't storing his data like you are. So it would have to be modified.
-
I would save it in the user table in a timestamp or datetime column. Changing it to the database timestamp format is easy with the strtotime function.
-
Select mysql & display in an html table using php
jcbones replied to skidmark10's topic in PHP Coding Help
No where in your first post did you say anything about a parse error! Post your ENTIRE script, that is the only way to deal with parse errors. (Include the acutal error also, there should be a line number in it.) -
how to hide Table 'table_name' doesn't exist message?
jcbones replied to $php_mysql$'s topic in PHP Coding Help
just checked php.ini and yes it says on display_errors ; Default Value: On ; Development Value: On ; Production Value: Off so i tried to turn it off display_errors ; Default Value: Off ; Development Value: Off ; Production Value: Off but still it shows? i even restarted my wamp server. but this is on my laptop how will i control it on shared webhosting? You need to scroll further down the php.ini file, display_errors is set in the ERROR HANDLING section. What you are looking at is the Quick Reference section. -
They are aliases of your database tables, just so you don't have to type them out each time you refer to a column. LEFT JOIN (test_fixtures tf, test_teams tth, test_teams tta) //also written as LEFT JOIN (test_fixtures AS tf, test_teams AS tth, test_teams AS tta)
-
Select mysql & display in an html table using php
jcbones replied to skidmark10's topic in PHP Coding Help
mysql_select_db("my_db", $con); $result = mysql_query("SELECT * FROM Persons"); echo "<table border='1'><tr><th>Name</th></tr>"; while($row = mysql_fetch_array($result)) { echo "<tr>"; echo "<td>" . $row['FirstName'] . ' ' . $row['LastName']"</td>"; echo "</tr>"; } echo "</table>"; mysql_close($con); ?> -
Did you open the firefox error console, and see if the javascript is throwing an error?
-
Try: <?php $results = mysql_query("SELECT * FROM $table3 ORDER BY id ASC LIMIT $page, $limit"); while ($data = mysql_fetch_array($results)) $str = $data["content"]; echo (strlen($str) > 15) ? substr($str,0,strpos($str,' ',15)).'...' : $str; } ?>
-
<?php $con = mysql_connect("XXX"); mysql_select_db("product_catalogue") or die( "Unable to select database"); if (!$con) { die('Could not connect: ' . mysql_error()); } $image = $_POST['image']; $link = $_POST['link']; $name = $_POST['name']; $id = $_POST['id']; $cat = $_POST['cat']; $version = $_POST['version']; $description = $_POST['decription']; $price = $_POST['price']; $shipping = $_POST['shipping']; if (!$id ) { die('Product ID is required!'); } else { $query = " UPDATE 'Products' SET 'ProductImage' = '$image', 'ProductLink' = '$link', 'ProductName' = '$name', 'Category' = '$cat', 'ProductVersion' = '$version', 'ProductDescription' = '$description', 'ProductPrice' = '$price', 'ShippingPrice' = '$shipping' WHERE 'ProductId' = '$id' "; echo " Update complete "; mysql_close($con); } ?>
-
Your Welcome!
-
1. Retrieve database info. 2. Link the row's primary key (usually `id`) to another script through a _GET variable. ie. /index.php?get=id 3. Grab the primary key in the second script, and populate a form. 4. Post the form to a script that will update the database.
-
2 ways: 1. Ask the user his/her timezone 2. Use javascript to grab it, and then send it to the server.
-
$keys = array_keys($arr); echo $keys[2]; http://php.net/manual/en/function.array-keys.php