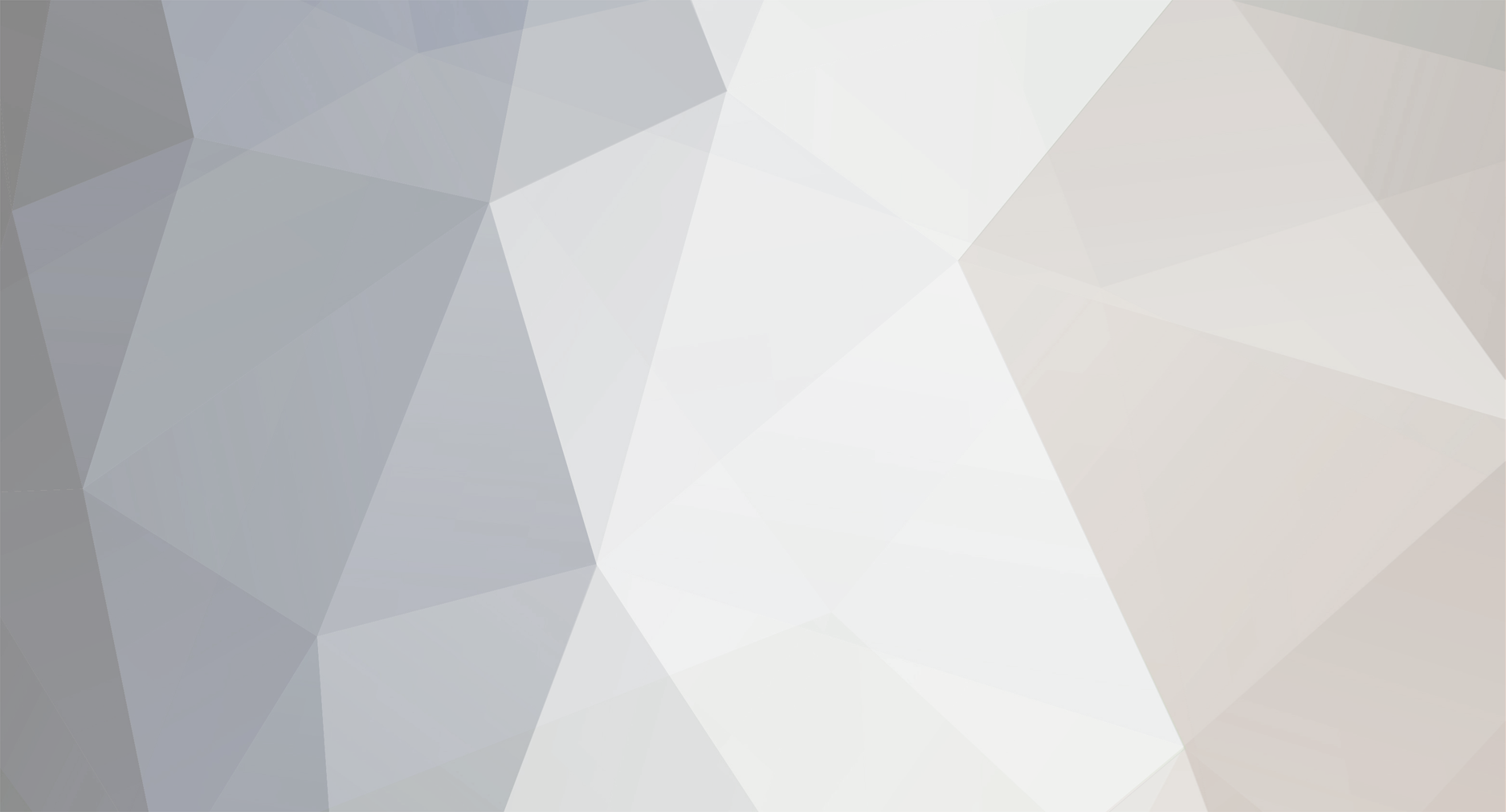
jcbones
Staff Alumni-
Posts
2,653 -
Joined
-
Last visited
-
Days Won
8
Everything posted by jcbones
-
You shouldn't need to create separate pages for the galleries. Creating separate directories is fine, but you should be able to handle everything else on one page. Whether you use a flat file, or a database. So, say you make your gallery directories in a folder named gallery, which resides in the web_root directory, and your gallery.php page resides in the same directory. <?php define('GALLERY_DIRECTORY',str_replace('.php','/',$_SERVER['SCRIPT_FILENAME'])); //since this filename is gallery.php, and our gallery folder is named gallery, we strip the .php off the end of the file, and append a directory separator. $galleryName = strip_tags($_GET['gallery']); $gallery = GALLERY_DIRECTORY . $galleryName; if(!is_dir($gallery)) { die('Your gallery does not appear to exist!'); } echo 'You have found a photo gallery named: ' . $galleryName;
-
Is your id column set as an integer? If the column is set as a string type, then it orders by the first character. So, all 1's (1,10,11,12, etc.) will come before the 2's (2,20,21,etc). To test this out, make you a test table, with the following id's. 1,10,2,200,50,9,18. Set the column type as varchar and run a query to order by the id desc, and asc. Now change the column type to integer.
-
Just insert the MySQL function NOW(). It will populate the timestamp column with the current time. INSERT INTO table (timestamp_column) VALUES (NOW())
-
To fix your stringData2 without having PHP 5.3.0, then you will have to enclose it in single quotes, and escape EVERY single quote in the string. $stringData2 = ' <?php $name_first = $_POST[\'name_first\']; $name_last = $_POST[\'name_second\']; $phone = $_POST[\'phone\']; $email = $_POST[\'email\']; $message2 = "First Name: $name_first \n Last Name: $name_last \n Phone: $phone \n Email: $email . "; $to = $receive_email; $subject = \'Registration to \' . $company_name; $message = $message2; $from = $receive_email; $headers = \'From:\' . $from; mail($to,$subject,$message,$headers); echo \'Mail Sent. Click <a href="register.php">here</a> to go back!\'; ?> '; Try it and see if that is what you want, as you haven't really stated what you are trying to do. It looks as if you are using PHP to write PHP, so that is the solution I gave you.
-
how do I display values from another mysql table?
jcbones replied to Darkwoods's topic in PHP Coding Help
What mysql field? How can a property be in two different categories? Perhaps you need a table to tie multiple categories to the one property. Has your database been normalized? If not, I would suggest reviewing some short video's. This will go along way in helping you to get data in an ordered, and dependable fashion. <-Watch all 9, they are short, shouldn't take you to long. -
mysql query select all but or except?
jcbones replied to YoungNate_Black_coder's topic in PHP Coding Help
SELECT * FROM table WHERE id != 1; -
What are you trying to do, because the code flow just doesn't make any sense. Are you writing the PHP code to stringData2 (1), or do you want PHP to parse the code in stringData2 (2)? Assuming it is (1). ONLY the PHP <?php if (isset($_POST['submit'])){ $company_name = $_POST['company_name']; $receive_email = $_POST['receive_email']; //using a nowdoc, nothing inside this nowdoc will be parsed. $stringData2 = <<<'EOF' <?php $name_first = $_POST['name_first']; $name_last = $_POST['name_second']; $phone = $_POST['phone']; $email = $_POST['email']; $message2 = 'First Name: " . $name_first . " \n Last Name: " . $name_last . " \n Phone: " . $phone . " \n Email: " . $email . "'; $to = '" . $receive_email . "'; $subject = 'Registration to " . $company_name . "'; $message = $message2; $from = '" . $receive_email . "'; $headers = 'From:' . $from; mail($to,$subject,$message,$headers); echo 'Mail Sent. Click <a href='register.php'>here</a> to go back!'; ?> EOF; $ourFileName = "register_ac.php"; $ourFileHandle = fopen($ourFileName, 'w') or die("can't open file"); fwrite($ourFileHandle, stripslashes($stringData2)); fclose($ourFileHandle); //using a heredoc, variables inside this heredoc will be parsed. $stringData = <<<ROF <html> <head> <title>" . $company_name . " Registration</title> <script type='text/javascript'> function validateForm() { var name_first=document.forms['registration_form']['name_first'].value var name_last=document.forms['registration_form']['name_last'].value var phone=document.forms['registration_form']['phone'].value var email=document.forms['registration_form']['email'].value if (name_first==null || name_first=='' || name_last==null || name_last=='' || phone==null || phone=='' || email==null || email=='') { alert('Please fill in all information!'); return false; } } </script> </head> <body> <center> <!-- This is the table that contains the registration form! --> <form name='registration_form' method='post' action='register_ac.php' onsubmit='return validateForm()'> <table bgcolor='#cccccc'> <tr> <td> <table border='0' cellspacing='0' cellpadding='5' width='320'> <tr> <td colspan='2' align='center' bgcolor='white'><h2>" . $company_name . " Registration Page</h2></td> </tr> <tr> <td colspan='2' align='center' bgcolor='white'><font size='2'>All fields are required to submit this form.</font></td> </tr> <tr> <td colspan='2'><hr></td> </tr> <tr> <td><b>First Name:</b> </td><td align='right'><input type='text' name='name_first' size='30'></td> </tr> <tr> <td><b>Last Name:</b> </td><td align='right'><input type='text' name='name_last' size='30'></td> </tr> <tr> <td><b>Phone Number:</b> </td><td align='right'><input type='text' name='phone' size='30'></td> </tr> <tr> <td><b>Email:</b> </td><td align='right'><input type='text' name='email' size='30'></td> </tr> <tr> <td colspan='2'><hr></td> </tr> <tr> <td> </td><td align='right'><input type='submit' name='submit' value='Register'></td> </tr> </table> </td> </tr> </table> </form> <!-- End of the table containing the Registration form! --> </center> </body> </html> ROF; $ourFileName = "register.php"; $ourFileHandle = fopen($ourFileName, 'w') or die("can't open file"); fwrite($ourFileHandle, $stringData); fclose($ourFileHandle); } else { echo " </head> <body> <center> <form name='myForm' method='post' action='' onsubmit='return validateForm()'> <table> <tr> <td colspan='2' align='center'><h2>Registration Configuration Form</h2></td> </tr> <tr> <td>Company Name: </td><td><input type='text' name='company_name' size='30'></td> </tr> <tr> <td>Email: </td><td><input type='text' name='receive_email' size='30'></td> </tr> </table> </form> </center> </body> </html>"; } ?>
-
how do I display values from another mysql table?
jcbones replied to Darkwoods's topic in PHP Coding Help
Try running: SELECT a.*, b.title FROM properties AS a JOIN categories AS b ON a.pr_category = b.id I would suggest running that query inside your database management software (phpMyAdmin), or the console, to make sure it returns the data you expect. This will save you coding time. -
If I wasn't so lazy today, I would have broken the query out of the function. But, ya caught me...
-
Accessing and processing 3rd level JSON array through php
jcbones replied to frobak's topic in PHP Coding Help
Or, you could use something like: $feeds = $facebook->api('/255500227799817/feed'); foreach($feeds['data'] as $feed => $list) { echo "<h2>$feed</h2><ul>"; foreach($list as $k => $v) { echo "<li>$k - "; if(is_array($v)) { //if $v is an array. echo '<ul>'; //make a sub UL foreach($v as $kk => $vv) { //loop the array. echo "<li>$kk - $vv</li>\n"; //list it. } echo '</ul>' . "\n"; //close it. } else { //if it ISN"T an array. echo $v; //just print it. } echo "</li>\n"; } echo "</ul>"; } -
Have you tried: $ipList = file('./IPS.txt'); //set each line from the file IPS.txt into an array called ipList.
-
Things can be overlooked by even the best of programmers. I would suggest standard de-bugging protocol. error_reporting(E_ALL); ini_set('display_errors','On'); mysql_query("UPDATE contacts SET fname='" . mysql_real_escape_string($_POST['formName']) . "' WHERE ID = '" . intval($rec) . "'") or trigger_error( mysql_error() );
-
So, let me get this straight, because I'm not sure you understood my question. You typed that query into the database console, and it returned the rows you expected. If that is the case, then you will have to look into your db class to see where the error is. Perhaps your database class has an error/de-bug function. I would run that to see if there is an error.
-
Calling the same function twice, which doesn't get the data. if (@mysql_num_rows($query)) { while ($req=@mysql_num_rows($query)) {
-
Have you run your query directly in the database, to make sure it performs as expected?
-
having trouble with this query won't insert in the specified field
jcbones replied to co.ador's topic in PHP Coding Help
What is the question? -
You don't need sprintf() on that string. $sql = 'SELECT car_manufacturer, COUNT(*) as car_count FROM car_pool GROUP BY car_manufacturer'; $result = mysql_query($sql) or trigger_error($sql . ' has an error<br />' . mysql_error()); if(mysql_num_rows($result) > 0) { while($row = mysql_fetch_assoc($result)) { echo $row['car_manufacturer'] . ' | ' . $row['car_count'] . '<br />'; } } else { echo 'There is NO cars selected!'; } Teynon has a typo, this should work.
-
how can i replace name of the file with php echo
jcbones replied to Lisa23's topic in PHP Coding Help
You need to escape the double quotes, since it is a double quoted string: echo "<td><a href='#' onClick=\"jwplayer().load({'file': '$FirstName', 'hd.file': 'videos/film.mp4'})\"> </td>"; -
Is there a more efficient way to handle this?
jcbones replied to thebusiness's topic in PHP Coding Help
This may work for you: <?php //function from http://www.php.net/manual/en/function.round.php user given in notes. function roundUpTo($number, $increments) { $increments = 1 / $increments; return (ceil($number * $increments) / $increments); } $rounding = 5; if($row['rating'] >= .1 && $row['rating'] < 5.1) { $parts = explode('.',$row['rating']); //next two lines extract the decimal. $number = $parts[0].$parts[1]; //puts numbers back together. $rate = roundUpTo($number, $rounding); } else { $rate = '00'; } $random_game_mini['rate'] = '<img src="'.$setting['site_url'].'/'.$setting['template_url'].'/images/minirating' . $rate . '.png" height="12" width="61">'; ?> -
Html button delete row from mysql doesn't work
jcbones replied to unknown00's topic in PHP Coding Help
You could also print_r you Post variable to see if the command is coming through. echo '<pre>' . print_r($_POST,true) . '</pre>'; -
I think you are looking for "H:i", Date Function
-
You need to build an image script, then link that image script with the image tag. Here is a great tutorial on the whole process.
-
export php page to a file (excel or pdf)
jcbones replied to houssam_ballout's topic in PHP Coding Help
Well, I guess that was on me, as I thought you knew how to populate an array from the database. Being that I don't know your database structure, I will give you arbitrary code. <?php include 'database_connection.php'; $sql = "SELECT * FROM table"; $result = mysql_query($sql) or trigger_error($sql . ' has an error.<br/>' . mysql_error()); if(mysql_num_rows($result) > 0) { while($row = mysql_fetch_assoc($result)) { $data[] = $row; } } if(!is_array($data)) { exit('There is no data to create an excel file from'); } function cleanData($str) { $str = preg_replace("/\t/", "\\t", $str); $str = preg_replace("/\r?\n/", "\\n", $str); } //header("Content-Type: text/plain"); //<-lose the header function. $filename = 'excel_export.xls'; $output = NULL; //define our holding variable. $flag = false; foreach($data as $row) { if(!$flag) { # display field/column names as first row $output .= implode("\t", array_keys($row)) . "\r\n"; //set this row to our variable. $flag = true; } array_walk($row, 'cleanData'); $output .= implode("\t", array_values($row)) . "\r\n"; //set this row to our variable. } file_put_contents($filename,$output); //write the output to a file; ?> -
export php page to a file (excel or pdf)
jcbones replied to houssam_ballout's topic in PHP Coding Help
Well, you take his code snippet: <?PHP function cleanData($str) { $str = preg_replace("/\t/", "\\t", $str); $str = preg_replace("/\r?\n/", "\\n", $str); } header("Content-Type: text/plain"); $flag = false; foreach($data as $row) { if(!$flag) { # display field/column names as first row echo implode("\t", array_keys($row)) . "\r\n"; $flag = true; } array_walk($row, 'cleanData'); echo implode("\t", array_values($row)) . "\r\n"; } ?> And make some changes: <?PHP function cleanData($str) { $str = preg_replace("/\t/", "\\t", $str); $str = preg_replace("/\r?\n/", "\\n", $str); } //header("Content-Type: text/plain"); //<-lose the header function. $filename = 'excel_export.xls'; $output = NULL; //define our holding variable. $flag = false; foreach($data as $row) { if(!$flag) { # display field/column names as first row $output .= implode("\t", array_keys($row)) . "\r\n"; //set this row to our variable. $flag = true; } array_walk($row, 'cleanData'); $output .= implode("\t", array_values($row)) . "\r\n"; //set this row to our variable. } file_put_contents($filename,$output); //write the output to a file; ?>