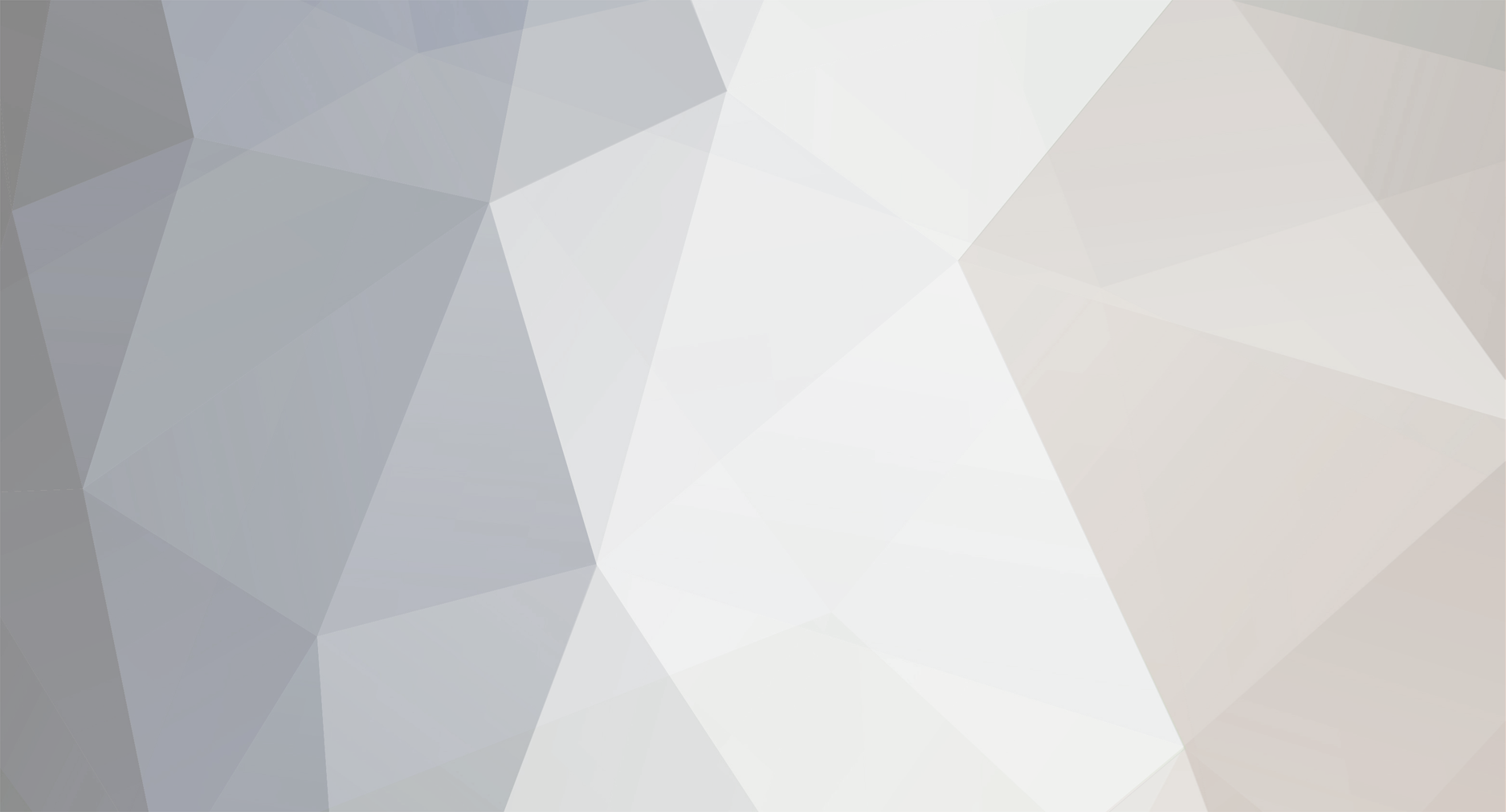
c_pattle
-
Posts
295 -
Joined
-
Last visited
Posts posted by c_pattle
-
-
Problem solved though. Thanks PFMaBiSmAd. I had a new line character after ?>. I didn't realise this would make difference though.
-
Sorry I forgot to mention that my inc_theme.php is not outputting anything to the screen. Even when I comment out that file include I still get the error.
-
Sorry. If I change my index.php file to this
require_once("includes/inc_theme.php"); include("classes/autoloadClass.class.php"); session_start();
Then I get these error messages.
Warning: session_start() [function.session-start]: Cannot send session cookie - headers already sent by (output started at /var/www/webemail/classes/autoloadClass.class.php:9) in /var/www/webemail/index.php on line 4
Warning: session_start() [function.session-start]: Cannot send session cache limiter - headers already sent (output started at /var/www/webemail/classes/autoloadClass.class.php:9) in /var/www/webemail/index.php on line 4
It's saying that the error is appearing on line 9 of my autoload class put that line doesn't exist. It only goes up to line 8. This is my autoload class again.
<?php function __autoload($class_name) { include("classes/" . $class_name . ".class.php"); } ?>
-
Sure. Here is my index page
<?php require_once("includes/inc_theme.php"); session_start(); include("classes/autoloadClass.class.php");
By starting the session before I include the autoload class I don't get the error anymore but I still get the error when I try to use the header function late down the page.
-
I'm trying to use the autoload class but am having some trouble. Here is my autoload class,
function __autoload($class_name) { include("classes/" . $class_name . ".class.php"); }
The problem I am having is that when I include this class at the top of this page I then keep getting this error - "Warning: Cannot modify header information - headers already sent by" whenever I try to start a session or use the "header" function but I'm not sure why that's happening because surely I haven't echoed anything yet.
Thanks for any help.
-
I understand it now, thanks for such a detailed response.
-
This could be a silly question and it may not be very relevant here, forgive me if that is the case. When you have a domain like http://example.com and you then have a sub domain like http://something.example.com how is that achieved? Is it to do with the way the site is coded or structured?
-
Thanks
I tried to copy my class instance into a session variable but I am having some problems. On my index page I have this copied my class to a session variable and then on my other page I am doing this
$db = new dbClass(); $contentclass = new contentClass($db); session_start(); $contentclass = $_SESSION['contentclass'];
When I create the class I am passing a database connection using dependency injection. However when I copy my from session variable it effectively clears the connection meaning I can use any of the method in my class. Does anyone know what I should do?
Thanks for any help.
-
I was looking for a way to use to same instance of a class over multiple pages (e.g with the same property values) and I came across serialization. However the only articles I could find on it where quite a few years old. Is this still a good practise? Also if it is how to you recommend I do it? I have experimented serializing objects to session variables, is this a good method or should I use text files or databases instead?
Thanks for any help.
-
I'm having a bit of trouble using composition. I have a function in a class that uses composition. Here is that function.
function emailDetails() { $this->to = "hello@example.com"; $this->subject = "Login Details"; $this->message = "Here are your login details"; return new emailClass($this); }
Then in the "emailClass" the __construct function looks like this.
function __construct(&$user){ $this->user=&$user; $this->to = $this->user->to; $this->from = "someone@example.com"; $this->subject = $this->user->subject; $this->message = $this->user->message; }
This all works fine but my problem is that I then can't use the "emailClass" on it's own because it requires an instance of a class to be passed to it in it's construct. Is this a poor case of design on my part?
-
Thanks for all your help. I'll post some code to make it easier to understand what I want to achieve. This is my base class which connects to a database (it will contain more methods in time).
class baseClass { public $mysql_conn; function __construct($db) { $this->mysql_conn = $db; $rs = mysql_select_db("webdev_db", $this->mysql_conn->db) or die ('Could not connect: ' . mysql_error()); } }
Then I have a class called userClass and in that class is a method that is going to be used to email login details to a user. However because the userClass is already extending the baseClass I can't extend the userClass to inherit the methods in emailClass (my class for handling emails). The emailClass and the userClass aren't related I just need the functionality in that class. Hope that makes sense.
class userClass extends baseClass { function __construct($db) { parent::__construct($db); } function emailDetails() { //Code to send email }
-
I have a class that extends my base class as the base class has functions that all classes will need to use. However I have a function in my class that requires an email to be sent and I have a separate class for sending emails. The logical thing to do would be for my class to be an extension of both of these classes. However from what I've read you can do that in PHP so could any recommend as good way to solve this problem?
Thanks for any help.
-
Ah right, I understand it now. Thanks for your help.
-
I'm trying to use dependency injection to pass a database connection to an object but I'm not sure why it's not working. I have my "dbClass" below that connects to a MySQL database.
class dbClass { public $db; function __construct() { $this->db = mysql_connect("localhost","username","password") or die ('Could not connect: ' . mysql_error()); return $this->db; } }
Then I have my "baseClass". This is the class that I want to feed to connection too.
class baseClass { public $mysql_conn; function __construct($db) { $this->mysql_conn = $db; $rs = mysql_select_db("webdev_db", $this->mysql_conn) or die ('Could not connect: ' . mysql_error()); } }
And this is my index.php file. The error I'm getting is "supplied argument is not a valid MySQL-Link resource". However I tripled checked and my db connection details are definately correct.
$db = new dbClass(); $baseclass = new baseClass($db);
Thanks for any help.
-
I have a class and a lot of the function in the class require a connection to a MySQL database. At the moment I am using the "__construct()" to connect to the database each time a new instance of the classes is created. Is this the best way to do it or should I have a separate class for connection to the database? Just wanted to get an idea of what other people do.
Thanks for any help.
-
Just a quick question. I'm using the Live HTTP Headers add-on for Firefox that display all the HTTP headers etc. I'm writing an article on it and want to include a screenshot of it in action. Are there any headers that I should hide from the reader for security reasons?
Thanks for any help.
-
Hi everyone. Just wondering what we all use in terms of developers toolbars. I've downloaded Firebug for Firefox but wondering if anyone can recommend anything else?
-
Hi everyone
I've been learning PHP for quite a while now and I think of I got a good understanding of a lots of aspect of PHP programming. I know how to use functions, classes, includes, use javascript for ajax, regular expression and things like cURL. I'm now at the point where I'm not sure what to learn next. I want some advanced stuff to get my teeth into. Any suggestions?
Thanks for any help.
-
I wasn't sure where the best place to put this was but I'm sure someone here will know the answer. I have a website and I want to create a resources page that will provide links to resources that website designer and developers will find useful. My question is are you allowed to link to other sites without there permission? My list is going to be quite big, around 50 url's so having to get permission from all of these will be a hassle.
Thanks for any help.
-
I'm wanting to make a sitemap for my website but as it's quite big there are a lot of url's I need to include. I was wondering if anyone know's of a way this can be done using PHP. e.g. using PHP to generate a list of URL's. I had a think myself but wasn't sure how to do it.
Thanks for any help.
-
-
Thanks for your help. In that case should I just have one example for example "search.php?type=all&film=a+potential+value"?
-
I'm wanting to write a script that will automatically generate a sitemap for my website but I'm wondering how to find out when each page was last modified. Is there any easy way to do this using PHP?
Thanks for any help.
-
I'm wanting to make a sitemap for my website but should I includes ALL possible url's? For example I have a search page (where you can search a film) where the url syntax is something like "search.php?type=all&film=a+film". Should I make sure that all possible urls are included in my sitemap e.g all the films in my database? Hope that makes sense.
Thanks for any help.
php/mysql help
in PHP Coding Help
Posted
I'm having trouble working out a mysql query. I have two tables called "content" and "providers".
Content Table
Providers Table
What I want to do is to select all of the information in the "providers" table but also to perform a count on the "content" table to get the number of content for each provider. So in this case it would show all of the information for provider 1 and also show that provider 1 has 2 pieces of content because they have 2 entries in the "content" table.
I am able to get these results as two separate queries but I was wondering if it was possible to do it in one query.
Thanks for any help.