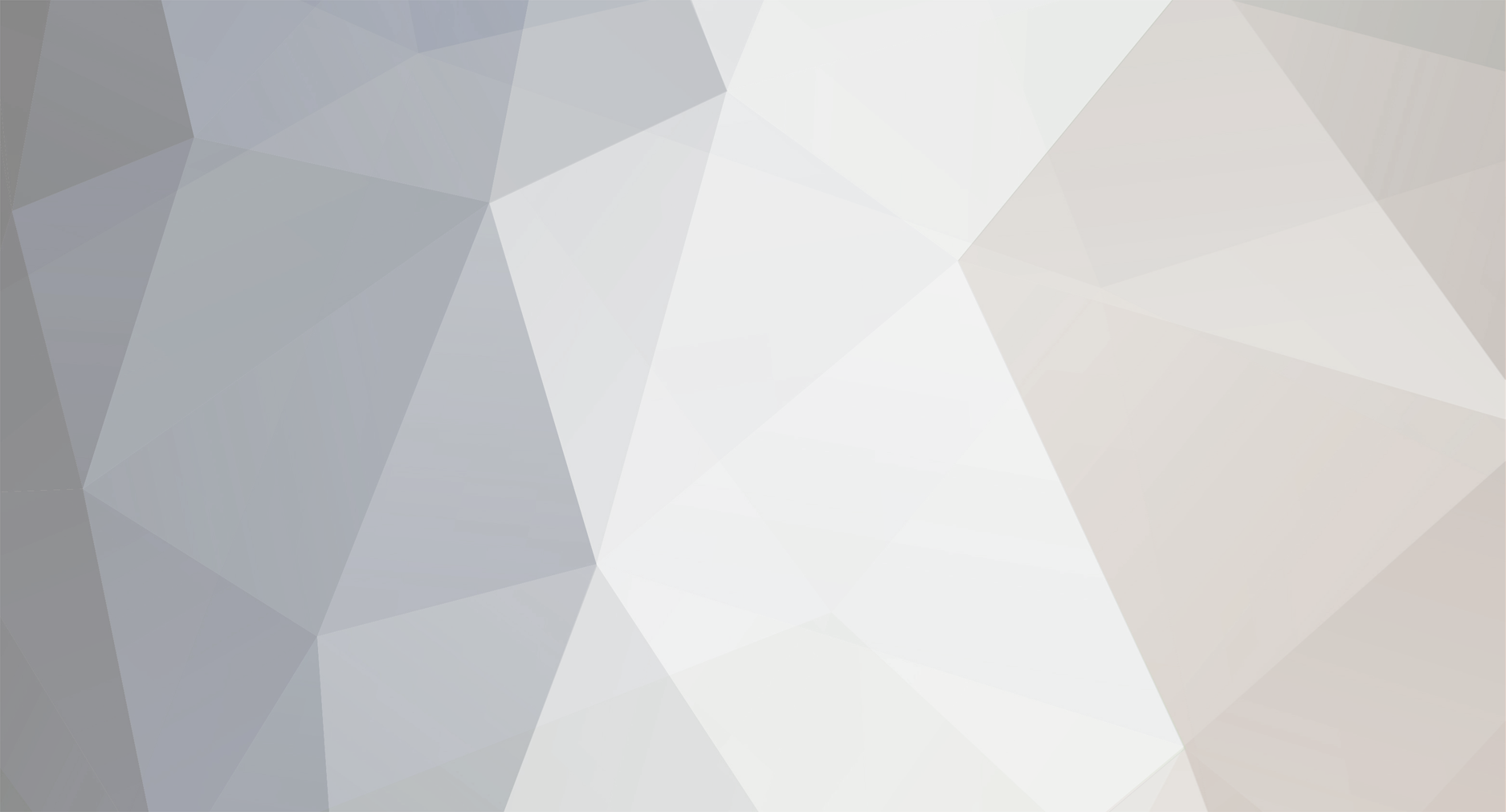
c_pattle
-
Posts
295 -
Joined
-
Last visited
Posts posted by c_pattle
-
-
I have a global variable called options that I have defined which is an array.
var options = { series: [{ type: 'pie', name: 'Title 2 Rating Distribution', data: [] }] };
How do I then insert something into this array from another function? For example I want to perform an ajax request and then copy the returned data into the variable called "data". However if I try to do the follow it says that "options.series.data is undefined".
$.ajax({ url: 'ajax/pie_chart_data.php', success: function(point) { options.series.data.push(point); }, });
Thanks for any help.
-
Great! Thanks for your help.
-
Thanks, I don't think I'm escaping the fields twice. I've copied below my code. Am I escaping it twice?
$submit_sql = sprintf("insert into reviews (review_name, review_content, review_summary) values (\"%s\", \"%s\", \"%s\")", mysql_real_escape_string($_GET['submit_film_name']), mysql_real_escape_string($_GET['submit_film_content']), mysql_real_escape_string($_GET['submit_film_summary']));
-
I have a form that allows users to submit to a database and for security reasons I am using mysql_real_scape_string on all of their input values. However this means that if the user puts something in speech marks such as
"hello"
It will then show up in the database as
\"hello\"
This means that whenever I fetch anything from the database it will have slashes in which doesn't look good. How do other people get round this problem. When I fetch something from my database should I do a string replace and just delete these slashes or is there a better method?
Thanks for any help.
-
btw I know how to do this for one of the criteria such as 0-20 by doing "COUNT(*) from reviews where review_rating between 0 and 20" but I don't know how to do this for all of the criteria I want in the same query.
-
I have a table that has a field called rating which can be from 0-100. I want to create a mysql query that will count the number of ratings between 0-20 then 21-40 then 41-60 etc. However I'm not really sure how to do this so any help would be great.
-
Thanks, yeah I am sure the variable exists. I think the problem is the fact that it's located in an external file. When I copied the same code into my main index page it works fine but not when it's in another file.
-
I'm having trouble setting a cookie in php. When a user logs in I send an ajax request to an file which contains this code to set the cookie
setcookie("userCookie", $_SESSION['username'], time()+60*60*24*30);
However when I include the following code to check to see if the cookie has been set is says is hasn't
if(isset($_COOKIE['userCookie'])) { echo("cookie set"); } else { echo("cookie not set"); }
I check to see that my browser is accepting cookies and it definitely is so I'm not sure what causing the problem.
Thanks for any help.
-
Thanks but what about if I overwrite "$myclass->variable" several times on one page. How can I then make sure that I can access this variable on another page?
-
So basically what would I do if the two pages I am working with HAVE to be separate?
-
Thanks, but what about if page1.php is a completely seperate page with html and php. If I were to includes I'd end up with two html pages in 1.
-
If I have defined an instance of a class in one script how do I then use this same instance and the same variable in other scripts? For example say I have this code in a file called "index.php"
include("myclass.class.php"); $myclass = new myClass; $myclass->$variable = "hello world";
However if I then have this code in a file called "page1.php" when I try to access the same variable it is empty.
include("myclass.class.php"); echo($myclass->$variable)
How can I use the same instance of this class across all files keep the variable values the same?
Thanks for any help.
-
Whenever I want to use AJAX (using jQuery) I store the PHP code in a seperate file and then send an retrieve data from that file using AJAX. However I was wondering if you can just call a PHP function. Then it would save me having to put each function in a seperate file?
Thanks for any help.
-
I have just start using PHP classes and was wondering how you continue an instance of a class in another file. For example I have a folder called "includes" where I includes files that I send data to when I perform ajax request. However how do I continue an instance of class in these files? Hope that makes sense.
Thanks for any help.
-
Ah right, bit of a silly mistake to make but thanks!
-
I'm having a problem with make my links work. I have a link saved in a session variable but when I print it to the screen it doesn't seem to work. What happens is instead of the link taking you to "www.google.com" it will but the currently directory first so for example if would be "www.example.com/folder/www.google.com".
I have checked the page source and it appears to be fine so I'm not sure what's happening. I've copied my code below.
echo("<h3 class=\"left\"><a href=\"" . $_SESSION['submit_content_link'] . "\" class=\"linker\" title=\"1\" target=\"_blank\">" . $_SESSION['submit_content_title'] . "</a></h3>");
Thanks for any help.
-
I have a table with a field called "tags" and below is an example of how this field might look like.
pensions, employee benefits, group benefits, defined contribution, auto-enrollment
I want to perform a query to get this field for all of the rows in my table. However is there a way to stop mysql from returning duplicates. For example if another field read,
pensions, employee benefits, group benefits, defined contribution, auto-enrollment, tax
Then it would only return "tax" from this field as it's already return pensions, employee benefits etc.
Thanks for any help.
-
Thanks but the problem I have with union is that it will but the records from each tables on different lines and I want it all on one line
-
Here are the two tables that I have. With both tables I have included an examples of the latest row that could have been entered. The first is a table called "articles".
The second is called "announcements".
-
Yeah I am open to suggestions on how to make it better.
Basically I have two tables that are completely unrelated. I want to have a query that will get the most recent 10 rows from both tables but will merge the two tables into one. I want to do this because it will be a lot easier when I come to actually doing something with the data.
For example if I do..
while ($array = mysql_fetch_array($rs)) {
It will be a lot lot easier if all the data I need is on the same row.
-
Thanks, I need to do it because I need to join 2 tables together.
I tried using a left join but because there are no common fields it means that I just got "null" for all of the values from the right table. However if I have a common field then the join will work. Hope that makes sense.
-
I was wondering if anyone know's how to create a field in a mysql query. For example if I have a query such as "select * from names" is there a way to add a field that automatically increment for search row. So for example the query would then return a list of names each with a unique number (e.g 1-10) even though this field is not in the database.
I want to do this because I want to join two query and I want to create a common field which both queries will have.
Thanks for any help.
-
I'm trying to get a good full text search working but am having some problems. Below is the sql that I am using to perform the search. One of the problems I am having is that the search only seems to be working on full words. Also if I misspell a word such as "seacrh" rather than "search" then no matches are found. Is there a way to return results even for misspelt words?
match(author) against('search_phrase ')
Thanks for any help.
-
Is there a way to create a temporary field which will assign an number to each record on both tables, then I could simply compare those two numbers...?
PHP Search Engine
in PHP Coding Help
Posted
I thinking about building a search engine just as a fun project and a way to develop my skills. However I'm not sure where to start...
I want it to be a comparison search engine for computer parts so say the user type in "RAM" it would display results from PC World, Ebuyer, etc. I was thinking of using cURL but how do I know where the search results are on each page. For example say I get a html page of results from PC World and store them in a variable how do I then know what to strip out to get the results I want? Would I just have to look at the source code? Also would I then have to work this out for each site I wanted to search from and have a different method of getting the results from search site?
If anyone has done anything like this in the past and could give me some advice that would be great.
Thanks