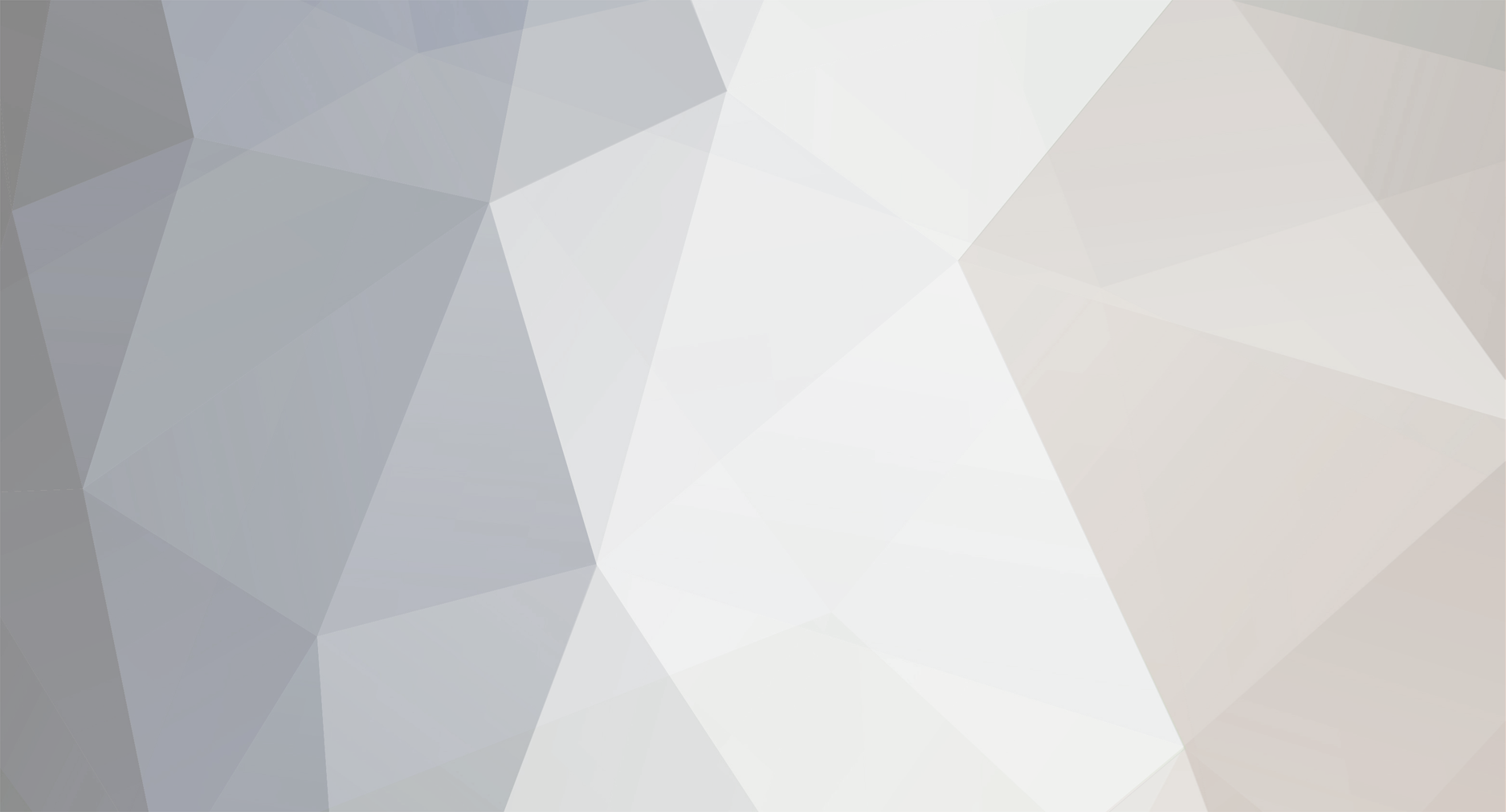
siric
-
Posts
116 -
Joined
-
Last visited
Posts posted by siric
-
-
Would you mind posting the code, so that we can get a better idea of what you are attempting?
-
Good that you got it sorted. Can you mark this thread solved? Thanks
-
Why would you know create a an entry in the table for each product code and store the amount ordered there?
-
Place the variable clearing lines at the top of the script
<?php //Adding a new user into the database //Clearing variables $register_firstname = $register_lastname = $register_username = $register_email = $register_password_1 = $register_password_2 = ''; $register_firstname = $_POST['register_firstname']; $register_lastname = $_POST['register_lastname']; $register_username = $_POST['register_username']; $register_email = $_POST['register_email']; $register_password_1 = $_POST['register_password_1']; $register_password_2 = $_POST['register_password_2']; if( $register_firstname and $register_lastname and $register_username and $register_email and $register_password_1 and $register_password_2) { if( $register_password_1 == $register_password_2) { $sql="insert into users (first_name, last_name, username, email, password) values (\"$register_firstname\", \"$register_lastname\", \"$register_username\", \"$register_email\", \"$register_password_1\")"; $rs = mysql_query( $sql, $link ); if($rs) { echo("congratulations you have successfully registered"); } else { echo ('error' . mysql_error()); } //Clearing variables $register_firstname = ''; $register_lastname = ''; $register_username = ''; $register_email = ''; $register_password_1 = ''; $register_password_2 = ''; $_POST['register_firstname'] = ''; $_POST['register_lastname'] = ''; $_POST['register_username'] = ''; $_POST['register_email'] = ''; $_POST['register_password_1'] = ''; $_POST['register_password_2'] = ''; } else { echo ("The two passwords do not match. Please retype them"); } } else { echo ("You have not completed all the fields. Please fill in the blank fields"); } ?>
-
Please explain yourself better.
-
Hi,
I ran the script and it worked perfectly.
$url = "http://www.link.com This is a test and hello my name is bob"; $result = xcleaner($url); print $result; function xcleaner($url) { $U = explode(' ', $url); $W =array(); foreach ($U as $k => $u) { $W = explode('.', $u); if (stristr($u,'http') || (count($W) > 1 && $W[1] != "") || (count($W) > 2)) { unset($U[$k]); return implode(' ',$U); } } return implode(' ',$U); }
[attachment deleted by admin]
-
You need to explain yourself a whole lot better than this is you want to get assistance.
-
$result4 = mysql_query("SELECT petname FROM user_pets WHERE username = '$username' AND breeding = 'yes'"); echo "<b><u>Pets breeding:</u></b><br>"; echo "$result4<br>"; <----------------- WRONG
You can't do this. When the data is SELECTED from a table, you need to extract what you want.
$result4 = mysql_query("SELECT petname FROM user_pets WHERE username = '$username' AND breeding = 'yes'"); echo "<b><u>Pets breeding:</u></b><br>"; while ($myrow = mysql_fetch_array($result4)) { echo "$myrow['petname']<br/>; }
-
You need a new table while will include the pic id and a DATETIME field. Once someone downloads a pic, you insert an entry into this table. You can then query this table anytime you want to see pics for a particular period.
-
Post your code so we can have a look at it.
-
Ok,
Ran the code and found a few errors and once corrected, the code worked.
The change from string to integer should be run against the variables that are set at the top of the script and not the $_POST variables
Change
$H = (int)$_POST('hits'); $W = (int)$_POST('walks'); .....
to
$H = (int)$H; $W = (int)$W; .....
and you do not need to assign the $tournID and $playID variables again in this section as you have already done it in the first section.
-
try this
$qry = "INSERT INTO favorites (username, tutorialid, tutorialname, tutorialauthor) VALUES ('$loiginusername', '$row->id', '$row->name', '$row->fullname')";
Might I also assume that you have spelt $loiginusername incorrectly?
-
ah ok, so its more efficient to have a database with sat 100 fields, each containing 1 or 0 than to have one field with a string of 100 chars.
Ok, in that case its I dont need to concatenate the form results.
Thanks!!
Remember, I said that it depends on how many fields you have. You can try either way to see if it makes a difference in performance.
Simply, you 2 choices for a select are
With 1 field
- Select field from table.
- run string function to locate and extract one of multiples values
With multiple fields
- Select fields from table
-
anyone else know how to insert it properly?
echo "<FORM name='emai'" action=$_SERVER['PHP_SELF'] method='post' >";
-
The question of using one or many fields depends on the number of inputs you have. Also remember that if you use one field, then for every query against the database you have to run some extra processing to get the result extracted.
Personally, i would use separate fields for each one.
To create a string from submit, you assign the posted variables to a variable in your action script
$var1 = $_POST['var1']; $var1 = $_POST['var1'];
and to string them together, if you want to use 1 table field
$string = $var1.$var2....
-
There are loads of examples on forms and what the different components do that you can Google. eg http://www.tizag.com/phpT/examples/formvar.php and http://www.phpf1.com/tutorial/php-form.html
I assume that you are not sure what to put in the areas that you have the question marks.
You must give each form field a name and a value which will be captured once you submit the form. In the case of the selects, you don't really need the option name as the select name will be used.
<td><input type="checkbox" name="studied" value="yes" /></td> <td><select name="times"> <option>1</option> <option>2</option> <option>3</option> <option value="4">4</option> </select></td>
If the value being displayed in the dropdown box is the value that you want to pass to the action script, you do not even need the value, so the 3rd and 4th option will do the same thing.
Once the form is submitted, the script that is called will extract the values by using
$studied = $_POST['studies']; $times = $_POST['times']
-
At first glance, looks ok to me. What exact problems are you having?? Have you tried it??
-
Send me a copy of the database structure and I will look into it.
I just sent it in a message to you. Thank you!
The problem jumped out at me once I tried to run it. You cannot do an INSERT...WHERE. That would imply that you already have a row that meets the WHERE criteria in which case you do an UPDATE.
It would be
UPDATE stats set atBats='$abats', hits = '$hits', walks='$walks', RBI= '$RBI', strkOuts='$strkOuts', singles='$singles', doubles='$doubles', triples='$triples', homeRuns='$homeruns', runsScrd='$runsScrd', fieldersCh='$fieldersCh' where FK_playerID='$FK_playerID' and FK_tourneyID='$FK_tourneyID';
I have just assumed that those are the variable names and if not, you get the drfit.
-
Send me a copy of the database structure and I will look into it.
-
Have a look at this thread http://www.phpfreaks.com/forums/index.php/topic,297072.0.html
-
Is this a local database? If so, I would run something a client like SQLyog or Navicat Lite and run the query in there. This will allow you to identify where you error is occurring and to play around with it until it is right.
Even if it is a hosted database, use PhpMyAdmin and do the same thing.
-
If you intend to add the slash \ after the 34 and 18, you need to escape them. So your query should be
INSERT INTO storedata (id, NAME, city, state, phone, zip_code) VALUES ('VZFF-3418-NN??N?', '', 148, '34\\', '', '18\\' );
The first slash lets php know the the next character should be accepted literally and not as a control character.
-
Note that you had a few places where tags where closed but had not been opened
eg
<p>Search by Zip:</font></b>
Also am not sure why you are using a Z-index?? This is usually use when you want one area to appear to be in front of another.
Anyway, give this a try.
<table width="950" height="490" border="0" align="center" cellpadding="0" cellspacing="0"> <tr> <td height="47" bgcolor="efe9e3"><div align="center"><img src="950/top-block2.png" width="161" height="47" /></div> <td width="775" rowspan="2" valign="top" bgcolor="#FFFFFF"><p class="style1"><img src="direct-assets/Sl-tag.jpg" alt="" width="745" height="59" /> </p> <p class="style1"><span class="style5"><strong>Please use the form below to locate a retailer near you.</strong></span></p> <table width="700" border="0" align="center" cellpadding="0" cellspacing="0"> <tr> <td height="371" valign="top" class="PHP-area"> <?php if(!isset($_POST['submit'])){ ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> <label for="zip">Search by Zip:</label><div><input type="text" name="zipCode" size="20" class="input-box" /> <b>(example: 01922)</b></div> <br/> <label for="state">Search by State:</label><div><input type="text" name="state" size="20" class="input-box" /> <b>(example: NY for New York)</b></div><br/> <input type="submit" name="submit" value="Go"> </form> </td></tr></table>
Have a look at this page as well for some guidelines http://css-tricks.com/label-placement-on-forms/
-
Ok, I found some mistakes when I copied the code in there... so now I have it in and it works, but once you get the results it ruins the page layout... is it a Z-Index issue now?
Once formatting is screwed you know that it is a CSS issue. if you want to post that, I can have a look.
PHP Submit Form Timer?
in PHP Coding Help
Posted
Don't know if you use just PHP as a normal redirect will not submit as well. I think that you may have to resort to using JavaScript.