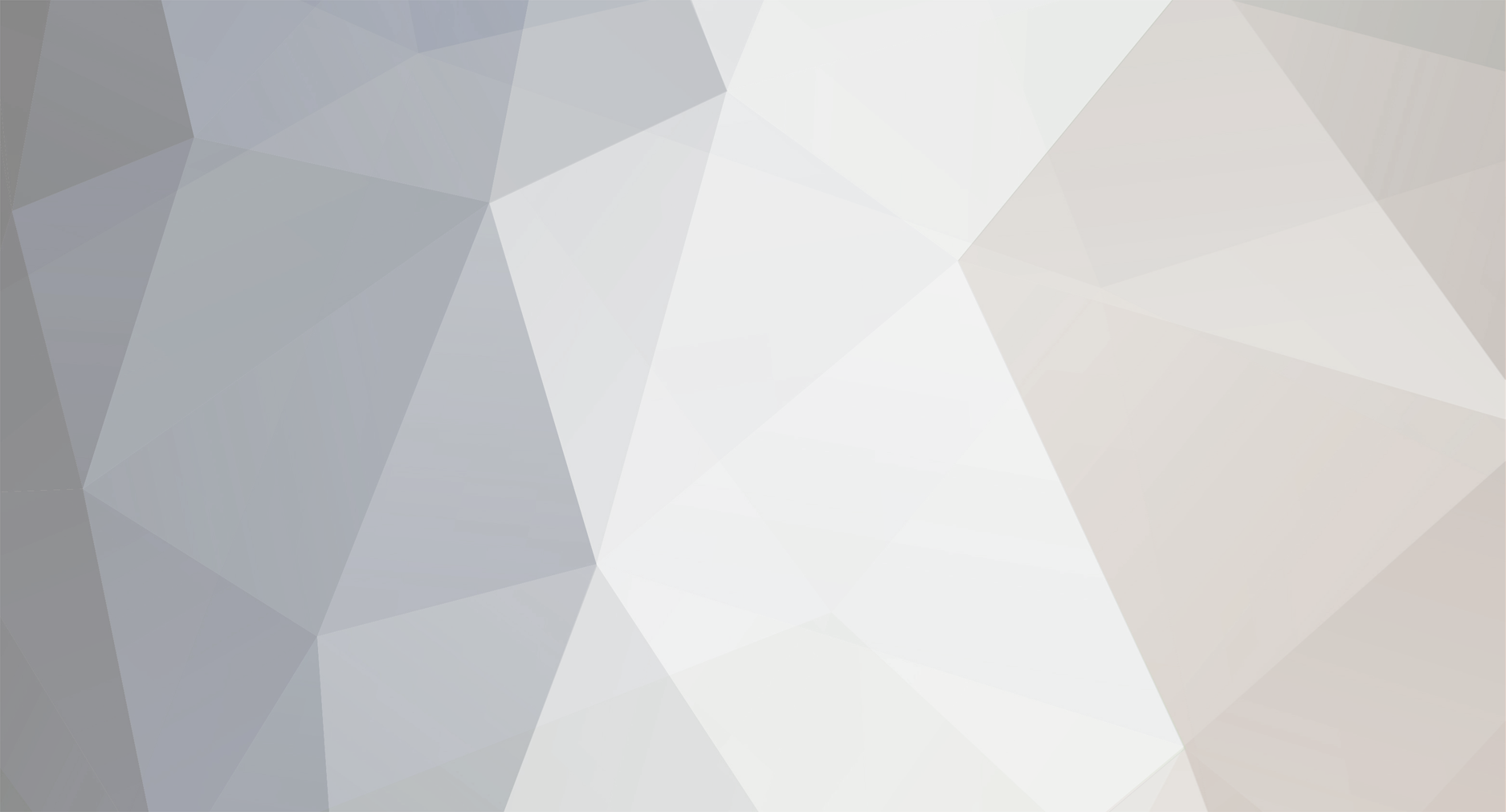
aesthetics1
Members-
Posts
18 -
Joined
-
Last visited
Everything posted by aesthetics1
-
Thank you very much requinix/lemmin. The updated function fixed the problem as far as I can tell.
-
Hi there. I'm having trouble with a holiday calculation script. I picked it up online and have simply added/removed a few holidays to fit my needs. It seems to calculate everything properly, except for Cesar Chavez day (March 31st). The script basically looks for the holiday, and then determines what day of the week it falls on. If it's a weekday, great. If it's on a Saturday, then Friday is the observed day, so it should return Friday. If it's Sunday, it should return Monday. As I said above, it works for the most part, but Cesar Chavez day won't compute (returns Jan 1 1970 date) in 2013, 2019, etc. Looking at my code, can anyone determine what's going on? Thanks in advance. <?php /* US Holiday Calculations in PHP * Version 1.02 * by Dan Kaplan <design@abledesign.com> * Last Modified: April 15, 2001 * ------------------------------------------------------------------------ * The holiday calculations on this page were assembled for * use in MyCalendar: http://abledesign.com/programs/MyCalendar/ * * USE THIS LIBRARY AT YOUR OWN RISK; no warranties are expressed or * implied. You may modify the file however you see fit, so long as * you retain this header information and any credits to other sources * throughout the file. If you make any modifications or improvements, * please send them via email to Dan Kaplan <design@abledesign.com>. * ------------------------------------------------------------------------ */ if(isset($_POST['year'])) { $current_year = $_POST['year']; } else { $current_year = date('Y'); } // Gregorian Calendar = 1583 or later if (!$current_year || ($current_year < 1583) || ($current_year > 4099)) { $current_year = date("Y",time()); // use the current year if nothing is specified } function format_date($year, $month, $day) { // pad single digit months/days with a leading zero for consistency (aesthetics) // and format the date as desired: YYYY-MM-DD by default if (strlen($month) == 1) { $month = "0". $month; } if (strlen($day) == 1) { $day = "0". $day; } $date = $year ."-". $month ."-". $day; return $date; } // the following function get_holiday() is based on the work done by // Marcos J. Montes: http://www.smart.net/~mmontes/ushols.html // // if $week is not passed in, then we are checking for the last week of the month function get_holiday($year, $month, $day_of_week, $week="") { if ( (($week != "") && (($week > 5) || ($week < 1))) || ($day_of_week > 6) || ($day_of_week < 0) ) { // $day_of_week must be between 0 and 6 (Sun=0, ... Sat=6); $week must be between 1 and 5 return FALSE; } else { if (!$week || ($week == "")) { $lastday = date("t", mktime(0,0,0,$month,1,$year)); $temp = (date("w",mktime(0,0,0,$month,$lastday,$year)) - $day_of_week) % 7; } else { $temp = ($day_of_week - date("w",mktime(0,0,0,$month,1,$year))) % 7; } if ($temp < 0) { $temp += 7; } if (!$week || ($week == "")) { $day = $lastday - $temp; } else { $day = (7 * $week) - 6 + $temp; } return format_date($year, $month, $day); } } function observed_day($year, $month, $day) { // sat -> fri & sun -> mon, any exceptions? // // should check $lastday for bumping forward and $firstday for bumping back, // although New Year's & Easter look to be the only holidays that potentially // move to a different month, and both are accounted for. $dow = date("w", mktime(0, 0, 0, $month, $day, $year)); if ($dow == 0) { $dow = $day + 1; } elseif ($dow == 6) { if (($month == 1) && ($day == 1)) { // New Year's on a Saturday $year--; $month = 12; $dow = 31; } else { $dow = $day - 1; } } else { $dow = $day; } return format_date($year, $month, $dow); } function calculate_easter($y) { // In the text below, 'intval($var1/$var2)' represents an integer division neglecting // the remainder, while % is division keeping only the remainder. So 30/7=4, and 30%7=2 // // This algorithm is from Practical Astronomy With Your Calculator, 2nd Edition by Peter // Duffett-Smith. It was originally from Butcher's Ecclesiastical Calendar, published in // 1876. This algorithm has also been published in the 1922 book General Astronomy by // Spencer Jones; in The Journal of the British Astronomical Association (Vol.88, page // 91, December 1977); and in Astronomical Algorithms (1991) by Jean Meeus. $a = $y%19; $b = intval($y/100); $c = $y%100; $d = intval($b/4); $e = $b%4; $f = intval(($b+8)/25); $g = intval(($b-$f+1)/3); $h = (19*$a+$b-$d-$g+15)%30; $i = intval($c/4); $k = $c%4; $l = (32+2*$e+2*$i-$h-$k)%7; $m = intval(($a+11*$h+22*$l)/451); $p = ($h+$l-7*$m+114)%31; $EasterMonth = intval(($h+$l-7*$m+114)/31); // [3 = March, 4 = April] $EasterDay = $p+1; // (day in Easter Month) return format_date($y, $EasterMonth, $EasterDay); } ///////////////////////////////////////////////////////////////////////////// // end of calculation functions; place the dates you wish to calculate below ///////////////////////////////////////////////////////////////////////////// ?> <?php // format to use: // // get_holiday("year", "month", "day_of_week", "week_of_month"); // get_holiday("year", "month", "day_of_week); // no 4th field indicates last week of month check // format_date("year", "month", "day"); ?> <div class="clearfix"> <form action="index.php?section=holidays" method="post"> <p><b>Enter a Year:</b></p> <p class="float-left"><input type="text" name="year" value="<?php echo $current_year; ?>" size="4" maxlength="4"></p> <p class="float-left"><input class="button grey small" type="submit" value="Go"></p> </form> </div> <?php echo "<p><b>".$current_year." Holidays</b></p>"; echo '<ul class="bullets">'; echo "<li>New Year's Day ". date("F d, Y", strtotime(observed_day($current_year, 1, 1))); echo "<li>Lincoln Day ". date("F d, Y", strtotime(format_date($current_year, 2, 12))); echo "<li>Martin Luther King Day Observed (Third Monday in January) = ". date("F d, Y", strtotime(get_holiday($current_year, 1, 1, 3))); echo "<li>President's Day Observed (Third Monday in February) = ". date("F d, Y", strtotime(get_holiday($current_year, 2, 1, 3))); echo "<li>Cesar Chavez Day = ". date("F d, Y", strtotime(observed_day($current_year, 3, 31))); echo "<li>Memorial Day Observed (Last Monday in May) = ". date("F d, Y", strtotime(get_holiday($current_year, 5, 1))); echo "<li>Independence Day Observed = ". date("F d, Y", strtotime(observed_day($current_year, 7, 4))); echo "<li>Labor Day Observed (First Monday in September) = ". date("F d, Y", strtotime(get_holiday($current_year, 9, 1, 1))); echo "<li>Columbus Day Observed (Second Monday in October) = ". date("F d, Y", strtotime(get_holiday($current_year, 10, 1, 2))); // Veteran's Day Observed - November 11th ? echo "<li>Thanksgiving (Fourth Thursday in November) = ". date("F d, Y", strtotime(get_holiday($current_year, 11, 4, 4))); echo "<li>Day After Thanksgiving (Friday after Thanksgiving) = ". date("F d, Y", strtotime("+1 day", strtotime(get_holiday($current_year, 11, 4, 4)))); echo "<li>Christmas Day = ". date("F d, Y", strtotime(observed_day($current_year, 12, 25))); echo "</ul>"; ?>
-
Sounds like this would help you! http://www.gizmola.com/blog/blog/archives/51-Exploring-Mysql-CURDATE-and-NOW.-The-same-but-different..html Check it out. Very helpful.
-
Creating an "Editable" Proposal Generator
aesthetics1 replied to aesthetics1's topic in PHP Coding Help
Imagine that, a dynamic database driven web app!... Thanks for the input. -
Creating an "Editable" Proposal Generator
aesthetics1 replied to aesthetics1's topic in PHP Coding Help
Thanks for the reply. The css media print property is how the editable invoice works as well (it hides the edit buttons etc when you print it.) I'm not so much worried about making it "pretty" as I am with the ability to archive the proposals and pull them up later. I guess I could store it all in mysql and pull them up through a search or "recent proposals" list. I think that would be doable unless there is some other simpler way that I can't grasp. Thanks for the suggestion. -
Hello again, I'm trying to work out the right way to do this, and I'm wondering if some of you could give me some tips or point me into the right direction. Basically what I'm looking to create is an "editable" proposal generator to make things a bit easier around the office. Basically you would have a landing page to select your product, select the options, add pricing, and plug a price into a lease payment generator that would spit out the math for the 12/24/.../60 month payment options. I thought about just creating it all in PHP with html forms for all of the variables (customer name, which product, options, etc) and then using PHP to do the math for the lease payments (easy enough I think,) but the part where I'm having trouble is the actual printing and archiving of the proposal once it has been generated. Ideally I would like it to fit perfect on a page every time, obviously, but there are other problems such as the header/footer parts coming out on the page (which I know is a client side thing that I can't really get around.) Is there a better way to do all of this? Is there another language I should be looking at that would make this easier conceptually? I'm sure a few of you have run across this "Editable Invoice" ( http://css-tricks.com/examples/EditableInvoice/ ...) I imagine it behaving similar to this (written with css, javascript.)
-
Thank you very much for your help! That worked perfectly, I'm getting exactly what I needed.
-
Alright, I have found where the problem lies in converting the tables over. There are actually a few dirty entries among the 2000 or so rows. A few of them lack the 00:00:00, and a few were blank, and not NULL. Is there a method for cleaning off the 00:00:00 from all of the rows so I can just fix the blank ones and convert it to just a date?
-
I do not want the NULLs included in what is grabbed from the database, so that is fine. I don't think I am going to be able to convert the structure of the field over in its current state though, can't figure out why. All values are either a timestamp format (2010-07-23 00:00:00) or 'NULL...'
-
It is a timestamp column actually. Will it work if all entries are followed by 00:00:00? how about if the field is NULL for some entries? I think that might work for me, going to test it out... Thanks for the speedy reply! EDIT: The fields are actually varchar, go figure.. Trying to convert them over right now but I'm getting an error: #1292 - Incorrect datetime value: '' for column 'columnname' at row 7 looks like it doesn't like an empty value somewhere?..
-
This may sound a little complicated.. I'm fairly new to some of these concepts so please excuse me if there is a much easier way... Basically, I am looking to show all entries from my mysql database that have a timestamp earlier than the current month. The entries are in the following format (where the first number is the year, the second is the month, the third is the day): 2010-07-23 Basically, I want to show all entries that have: 2010-06-XX 2010-05-XX 2010-04-XX 2010-03-XX 2010-02-XX 2010-01-XX What would the syntax of the SQL query for something like that look like? What PHP code would I need to grab this? I already have code grabbing the current month, and searching for anything that looks like: '%-$current_month-%' Like I said, please excuse me if this is a rather strange way of going about this. Thanks for any help. EDIT: I am currently looking at http://php.net/manual/en/function.date.php to see if there is anything helpful here but I haven't found anything quite suited to my situation...
-
correct me if I'm wrong, but I think it's good practice to make sure the dbconnect file isn't in your public html directory. usually 1 directory up, so you would then include it with: <?php include ('../dbconnect.php'); ?>
-
Phone Numbers - PHP is subtracting them.
aesthetics1 replied to aesthetics1's topic in PHP Coding Help
Thanks, the single quotes worked. I thought I tried that, but I got a parse error, must have fat-fingered it the first time. Thanks a lot. Yes, the database isn't a numeric only field. Thanks for that tidbit. -
Hello, This is probably something quick and simple. I have a web form that is entering information into a database, specifically a phone number in 555-555-5555 format. My SQL Query looks like this: $sql = "INSERT INTO $database.`service` (`customer_no`, `serial`, `phone`) VALUES ($customer_no, $serial, $phone)"; which outputs values like this: VALUES (number, number, 555-555-5555) my problem is, when the phone number is entered into the database, it isn't putting "555-555-5555" it's putting in -5555 (The difference of 555 minus 555 minus 5555...) How can I stop this from happening in this instance?
-
Delete Edit Add
-
David, Thanks a lot for your input.. Going to try and run the compact and repair tool this afternoon when everyone's off of the system. Hopefully that will take care of the sluggishness of the system, however I'd still like to rebuild it soon... Anything you recommend reading about database maintenance? Whether it be for MySQL or Access? Sounds like something I should be reading up on. Thanks!
-
Hello. I work for a small family-owned copier sales and service dealership. We have a Customer Service database that is done in Access. It keeps track of all of our customers, all of the copy machines, and also holds all of our open and old (completed) service calls. The problem is that it is beginning to really slow down. I don't know much about access.. I know that the database is extremely old, and not very functional or intuitive and that I want to update it or completely re-design it. I've begun work on a php/mysql based version of the database. I have already copied all of the data using a tool from the access tables into mysql, and restructured the tables to my liking. Some things that I'm having trouble with are: 1.) Keeping everything clean and easy. The database as it sits now has a Customer table, which holds all customer information (customer numbers, contact info, unique ids, etc), a Machine Table which holds serial numbers, copy counts, and links the machine to the customer (it has a field for customer number that ties in to the customer table), and a third table for Service Calls (tied to machines, uses the serial number). Is there an easier way to structure this, or does this seem pretty simple as-is? We move machines around, add new machines or get rid of old ones, create and delete customers, and we have to clear service calls (finish fields in the database and set from open to done.) 2.) I'm writing everything from scratch essentially. I wonder if there is something out there already made that I can pick from or base my code on at the very least. Is there a better language or database that I should be looking into? Or is there a way I can simply speed up the access queries (taking 10-30 seconds now, whereas the php/mysql version is near instant..) I have googled to try and find something that would work, but my google zen didn't pay off this time. I think I just need some guidance from someone who has taken on something like this before. Sorry for the wall of text. Any help or guidance would be greatly appreciated.. My users are growing weary and want something done sooner than later. Thanks, -Jamie