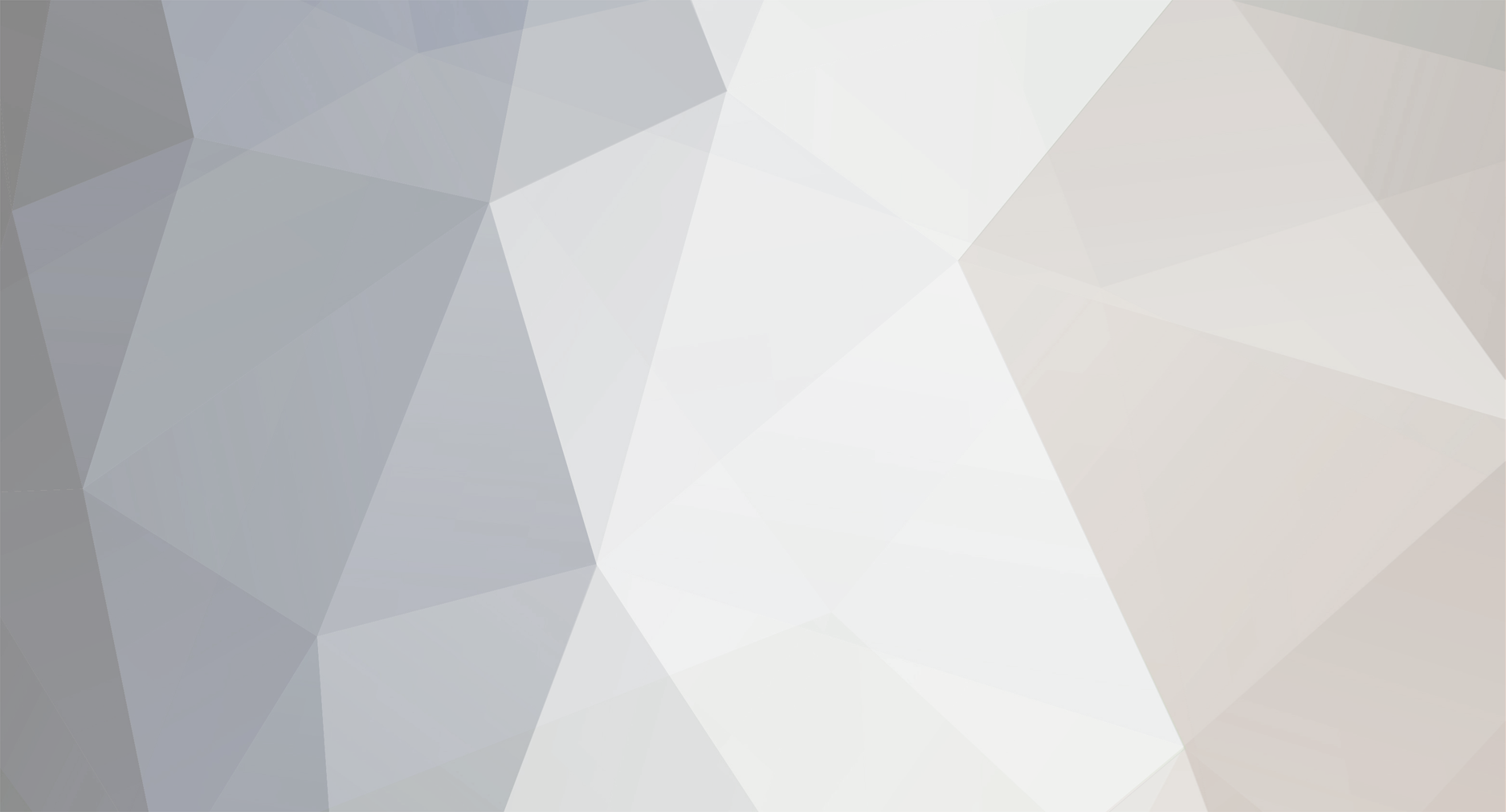
Steveo31
Members-
Posts
25 -
Joined
-
Last visited
Never
Everything posted by Steveo31
-
medic's right. Single quotes won't work, but it's generally a good idea to use double quotes when dealing with variables inside arrays. If the value of the variable is a number, it could be either parsed as a key location, or a value. But as for what you need to do, make sure you are defining "$user" as an array before pushing somethine into it. Also, wouldn't you need to do [code] $user = array(); $username = $_POST['assoc']; $user[] = $username; $name = $user["$username"]; echo $name; [/code] What is the value of $_POST['assoc']? What kind of array are you trying to make?
-
Or you could use the MySQL DATE_FORMAT function. Less PHP work. [a href=\"http://dev.mysql.com/doc/refman/5.0/en/date-and-time-functions.html\" target=\"_blank\"]http://dev.mysql.com/doc/refman/5.0/en/dat...-functions.html[/a] [code] SELECT DATE_FORMAT(lastDate, '%M %D') AS formatted_date [/code]
-
Personally I think case/switches are easier than do/while.
-
Generally you can't take a script and expect it to work. Sadly, you need to learn the code and it will take a few months of work to understand it and get it to the point that you speak of. You can also hire someone to do it if you need it done NOW. That, or if you can isolate the problem you can post it here.
-
Like... [code] if(..){ if(...){ } } [/code] Yea sure.
-
If the from header is set correctly, you don't need to set the sendmail_from in the .ini. You'll notice that when the From: header isn't set, then PHP yells at you saying you need one or the other. In the case of sending mail out from a variable address, then you need to format the From: header the right way. It's been a hit or miss for me, but what seems to work is From:email@domain.com Notice there are no spaces. Sometimes a space will work, sometimes I need the < and > brackets, seems to depend on the server I am working on.
-
It's a very roundabout way of doing it... little confusing on this end. Can you write a psuedo-code query of what you are after? I get what you want, but it's just... not clicking :)
-
What is "date is this date", how are you getting those dates, etc?
-
Only way I know is in the query it would be similar to [code] SELECT db1.products.color, db2.members.color FROM db1.products, db2.members [/code]
-
now() function used to INSERT datetime into database column?
Steveo31 replied to Malkavbug's topic in PHP Coding Help
NOW() is a function, not a translated value, so remove the single quotes. INSERT INTO table VALUES('something', 'other value', NOW(), 'other values); -
$open = f open("ipsforperson.txt", 'w'); f write($open, implode("\n",$file)); f close($open); Wouldn't work. The spaces between "f" and "write", etc are synatx errors.
-
Hmm... maybe something like this: [code] session_start(); if(empty($_SESSION['this_page_unique'])){ $_SESSION['this_page_unique'] = rand(0, 10000000); //show the rest of the page here }else{ echo "Go back. You cannot refresh the page."; } [/code] As far as I know there's no Javascript that allows this. I could be wrong though.
-
MySQL would be a better choice here, but if you dont have access, look into fopen(), fwrite(), fclose(), fread(). All functions you need. www.php.net/fwrite
-
The IP thing will have to come from either a mysql DB or a text doc. MySQL would be the better choice as making a counter is very simple. Do you have access to a mysql database?
-
Try adding "or die(mysql_error())" to the query it is referring to. If the rows arguement is throwing a fit, it's usually becuase the query isn't correct.
-
Yea there's a little trick to that: [code] <?php session_start(); // Start Header function page_header(&$pagename) { echo "$pagename"; } [/code] The & sign means its passing by reference or something like that. Check the manual.
-
Not too clear on your post... can you give more examples, etc?
-
I had this prob a while ago. The project I was working on it wasn't that important, so I didn't really look into a solution but I know its something in the Apache configuration, not necessarily the PHP side.
-
Right. The gist of it would be to check to see if the cookie exists. If so, then the necessary info will be in there, like usernames or whatnot. If not, then set it: [code] <?php setcookie('login_info', 'username', time()+60*60*24*30); ?> [/code] i.e. [code] <?php if(isset($_COOKIE['login_info'])){ $_SESSION['username'] = $_COOKIE['login_info']; }else{ //show login form or something } ?> [/code]
-
Can you post an example of an array and what values you want to put in the db? This sounds simple, but won't something like this work: [code] foreach($arr as $val){ if(!empty($val)){ //... etc } } [/code] I'm sure I'm missing something though.
-
Issues with eval() bringing PHP from mysql
Steveo31 replied to scrupul0us's topic in PHP Coding Help
What kind of PHP code are you storing? If it's being ignored, then its becuase there's nothing to eval(). -
What exactly are you trying to do? From what the code puts out, looks like a countdown to Christmas in months, but whats the image?
-
Before I begin, I would recommend reading the manual for help with functions. The very cool way that php.net is set up is you can type in "http://www.php.net/" and then the name of the function you want to look up, e.g. "http://www.php.net/mysql_num_rows" will bring up the appropriate page. List of Current Topics: URL's like page.php?id=something Session Errors MySQL Data Retrieval Trans-page variables Slashes/line breaks in blocks of text Decrypting MD5 MySQL Date/Time Formatting Force Download What does this error mean? A lot of the same questions are circulating, and come in every few days. That's great, I don't want you to NOT post in the forums, but they are easily answered and quite common as I've said. $_GET Variables Q: How do you get those dynamic URL's like www.domain.com/page.php?id=home? A: Those are $_GET superglobals. First off, do you know why you are using them? A lot of people want to know how, but don't realize the importance of it. Now, I was guilty of this when I started out, but after a bit of coding experience, it's clear why they are necessary. After all, you wouldn't buy a Ford Pinto just because you like horses. If you have a Pinto, then I apologize... their reputation precedes them As an example, I'll use the website I have built on my computer. There are tables and whatnot, and all I would like to change is the content in the main center table, and the title. Based on that, you can use GET variables to change them. Everyone has their own coding styles, so I am not trying to convert you into my style. This is just a working example of how to use these efficiently. Inside the main table it looks something like this: <table width="100%" style="etc..."> <tr> <td><?php include 'caseswitch.php'; ?></td> </tr> </table> That simply includes the .php file called "caseswitch.php" into that table data row. Inside that file are case/switch statements that show content based on the GET variable in the url. For example, index.php?cat=articles has this statement: switch($_GET['cat']){ case 'articles': include 'articles.php'; break; } Yes, I realize there are a lot of includes here, but it helps keep the code clean and seperated for ease of use. articles.php is just HTML/PHP/MySQL code that displays what I need it to inside the table previously discussed. Further Reading: http://www.phpfreaks.com/forums/index.php?...topic=32746&hl= --------------- Session Error Q: I keep getting this error: Warning: Cannot send session cookie - headers already sent by (output started at session_header_error/session_error.php:2) in session_header_error/session_error.php on line _. What can I do to fix it? A: This usually means that the code for starting the session, "session_start();" is not where it should be. If your page looks like this: <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN"> <html> <head> <title>Untitled</title> </head> <body> Hello World! </body> </html> Then your session_start() code needs to go here: <?php session_start(); //other header commands here ?> <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.0 Transitional//EN"> <html> <head> <title>Untitled</title> </head> <body> Hello World! </body> </html> After session_start, you can have cookie headers, etc. --------------- MySQL Data Retrieval Q: I have inserted the data into a MySQL database, but how do I show it? (I sometimes get "Resource id#") A: To do this, you should use mysql_fetch_array, or in many cases, mysql_fetch_assoc. The first one obtains an array where you can either use the column name, or a row number. Since I don't think in numbers, I use the second one, which only obtains an array of the column names. More info: http://www.php.net/mysql_fetch_array http://www.php.net/mysql_fetch_assoc To do this, let's assume you have a query set up. In this case, an example would be: $sql = "SELECT name, email FROM people_table"; $query = mysql_query($sql); while($row = mysql_fetch_assoc($query)){ echo $row['name'].'<br />'; echo $row['email'].'<br />'; } The while() loop will go through all the data in the table, and when it reaches the end (when there's no more data to go through), it will stop. Notice how the declaration of the $row variable is not used with the equality operator, ==. --------------- Variables Q: My variables don't show up in the next page! A: If you are using PHP version 4.2 or higher, register_globals is off, meaning you have to refer to the variables by their types. I would recommend keeping them off. If you POSTed the variables, you have to refer to them by $_POST['varname']. Same goes for $_GET, $_FILES, $_SERVER, $_COOKIE, and $_SESSION variables. --------------- Slashes, quotes, and line breaks, oh my! Q: Why are there slashes/no line breaks in my MySQL result? They weren't/were there before...!? A: This question is broken up into two, in case it didn't make much sense here. The first is that a query for a bunch of text, like a post, or an article or something has backslashes next to apostrophes '. A built in function in MySQL can add slashes if enabled. This is because the way the MySQL, and I'm sure other databases, handle information that you insert with single quotes. Confused? Take a look: <?php //set up the info to insert: $string = "Hey man, what's up? How've you been?"; //make a string query for simplicity's sake: $sql = "INSERT INTO tablename (`field`) VALUES('$string')"; //make the query: $query = mysql_query($sql) or die(mysql_error()); //Retreival of the data: while($row = mysql_fetch_array($query)){ echo $row['field']; } ?> This should bring up an error. To get around the error, you have to addslashes: <?php //set up the info to insert: $string = addslashes("Hey man, what's up? How've you been?"); //make a string query for simplicity's sake: $sql = "INSERT INTO tablename (`field`) VALUES('$string')"; //make the query: $query = mysql_query($sql) or die(mysql_error()); //Retreival of the data: while($row = mysql_fetch_array($query)){ echo $row['field']; } ?> This will bring up the correct data, but with backslashes: Hey man, what\'s up? How\'ve you been? If you don't addslashes, you will get an error. PHP first gets the value of that variable you are inserting, and then tries inserting it. If there are any apostrophes in there, then PHP/MySQL will see it as the end/beginning of another parameter in the INSERT query. This will, unfortunately, cause the slashes show up if you echo the results without a nifty little funtion called stripslashes. It does just what it's name says. To use it, just pull the variable out and strip it down: <?php $sql = "SELECT fieldname FROM tablename"; $query = mysql_query($sql) or die(mysql_error()); while($row = mysql_fetch_assoc($query)){ echo stripslashes($row['fieldname']); } ?> This will output what you put in, no slashes. The second part of this is the issue with line breaks in a multiline entry, so from a textbox for example. You use the function nl2br to make sure that the line breaks STAY where you put them. Take a look at the following example. Let's say you enter this into a textarea: Hi there! Would you like to see some examples of my work? If so, contact me! Sincerely, Joe Shmoe Now, when you put this into the database, and retrieve it without nl2br, it will look like this: Hi there! Would you like to see some examples of my work? If so, contact me! Sincerely, Joe Shmoe So, you just do this. $comm = $_POST['comments']; $sql = "INSERT INTO tablename (`field`) VALUES ('$comm')"; $query = mysql_query($sql) or die(mysql_error()); If you open PhpMyAdmin (or something similar) you will see it is all one paragraph. That's fine. When you pull it out of the database and display it, that's when you use nl2br: $query = mysql_query("SELECT body FROM articles"); while($row = mysql_fetch_assoc($query)){ echo nl2br($row['body']); } That will have the line breaks you normally had to begin with. Thanks Barand! ---------- Decrypting MD5 Q: How do I decrypt MD5? A. You can't. If you must do data encryption and decryption, take a look at mcrypt functions. Related Topics: http://us2.php.net/md5 http://www.phpfreaks.com/forums/index.php?showtopic=29917 http://www.phpfreaks.com/forums/index.php?showtopic=33071 ---------- Dates and Times in MySQL Q. How do I pull out a date from a MySQL DATETIME, DATE, TIME, or TIMESTAMP field into a readable format? A. Thanks for the idea there James (Websitenoobie). His way: $DATE = date('M jS Y g:ia A', strtotime($MYSQLFORMAT)); And another way: $sql = mysql_query("SELECT DATE_FORMAT(`date_field`, '%c-%e-%y') AS the_date FROM aTable"); $row = mysql_fetch_assoc($sql); echo $row['the_date']; Further Reading: Webclass date() symbols PHP.net date() manual MySQL Date and Time Functions ---------- Download Q. How can I force download of files? A. I found this snip of code and it looks promising. <?php // force to download a file // ex, ( [url=http://localhost/php/download.php?file=C:/Apache]http://localhost/php/download.php?file=C:/Apache[/url] Group/Apache2/hongkong.php ) // hope this can save your time :-) $file = $_REQUEST['file']; header("Pragma: public"); header("Expires: 0"); header("Cache-Control: must-revalidate, post-check=0, pre-check=0"); header("Content-Type: application/force-download"); header( "Content-Disposition: attachment; filename=".basename($file)); header( "Content-Description: File Transfer"); @readfile($file); ?> This hasn't been much of a common question, but it is helpful nontheless. ---------- Annoying Errors Solution: Make sure that the file you are trying to include() is named correctly, and is pointing to the right path, such as include 'includeFolder/file.php'; Solution: Check your SQL syntax and make sure that there is indeed a row (or rows) that match the criteria. For example: <?php $sql = mysql_query("SELECT name, id FROM users WHERE name='Jacko'"); //if there is no field called "name" you'll get an error. ?> Solution: Connect to the Database, and if you have connected, make sure that you have selected which DB you want to use with mysql_select_db(). Solution: Make sure the function exists. More to come.... ---------- I hope this helped, and I'll add more as they come to mind -Steve