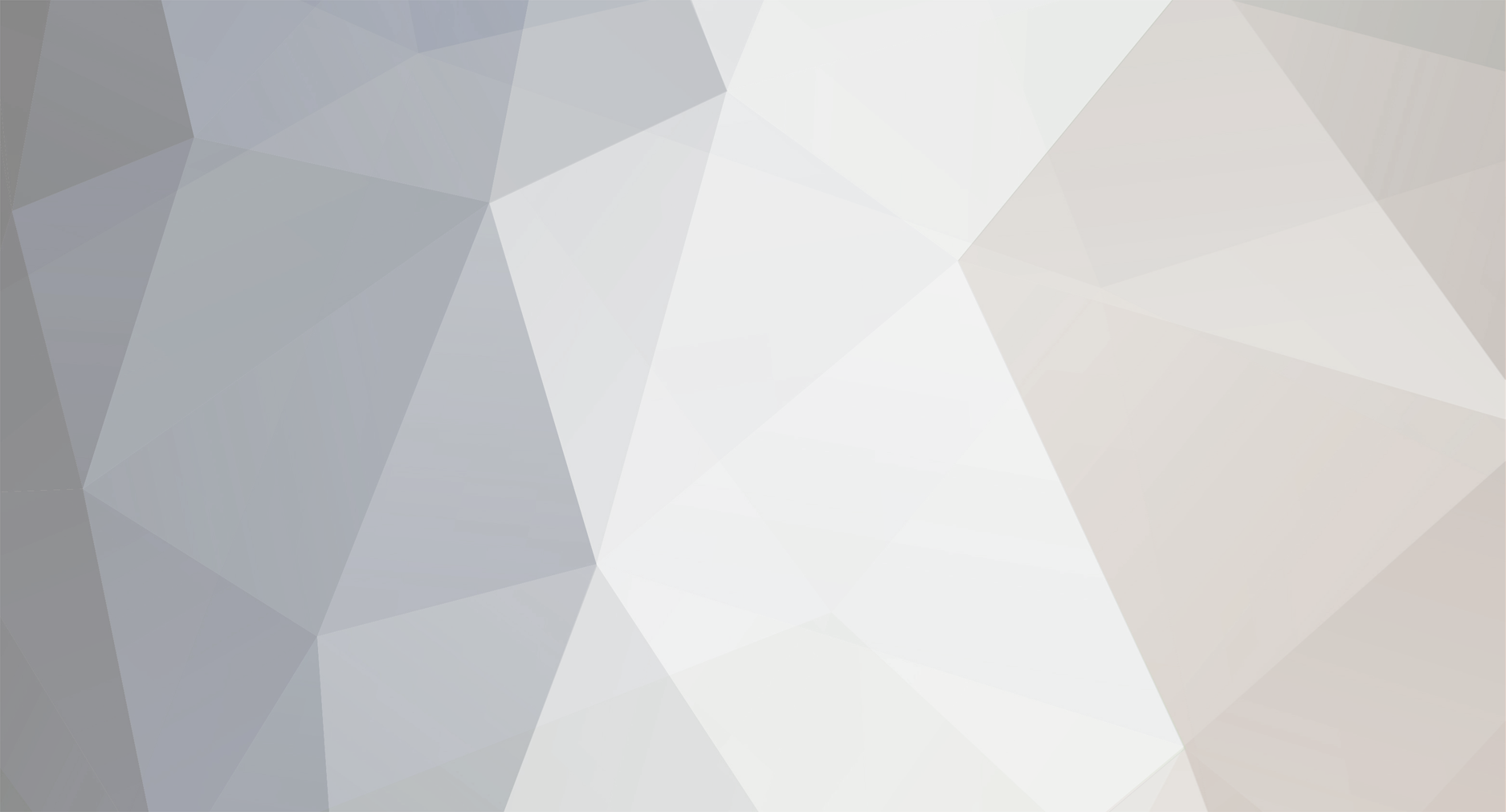
OldWest
Members-
Posts
296 -
Joined
-
Last visited
Never
Everything posted by OldWest
-
turn off form after set number of submissions
OldWest replied to zombie1234's topic in PHP Coding Help
I think you're on the right track. I would have a value as you suggested incremented in a table, and when that number was equal to the limit, a script could be included that was a formless page.. Pretty simple I think. A simple if else call to the database incremented value.. -
why width and height of image is not able to get in php
OldWest replied to rahulmehta's topic in PHP Coding Help
Check both values in your php.ini ; Maximum allowed size for uploaded files. upload_max_filesize = 50M ; Maximum size of POST data that PHP will accept. post_max_size = 50M -
why width and height of image is not able to get in php
OldWest replied to rahulmehta's topic in PHP Coding Help
If I read your question correctly, You might be running up against your php.ini setting for upload_max_filesize. I think 2mb is the limit by default. You can probably override this if your host allows it (again if I am reading your question correctly). -
If your hosting provider is running a php version 5.0 or later, sqllite (lite) should be installed. sqlite is essentially a flat file basic database system that uses commands similar to MySQL.. That would probably be your best bet. sqlite also has its own set of specialized commands (and uses some of standard SQL as well).. http://us.php.net/manual/en/book.sqlite.php
-
Hey Brother! Man. You really need to get a basic book or read some fundamental tutorials on php and MySQL... I am not saying this to be anal, but to try to get your some real help... Just at a glance, without even looking at your attached files, I am wondering why your <input /> fields have the same name. And although type="varchar" might exist in html (i've never used it and not aware of it ever existing), I think you're better of with type="text". I think you would be better off having your hosting company install a script like Joomla, and you can manage everything from that CMS without getting involved in the programming.. - just my 2cents.
-
IGNORE my last post!
-
Here is some example code I wrote using an array to do this job for you. You can process your strings in various ways, but this will produce the desired result: <?php $search = array(' '); $replace = array('-'); $subject = 'This is a string with spaces between each word.'; echo str_replace($search, $replace, $subject); ?>
-
Can you post all of your php code for the form w/ download button? (only the code related to this function).
-
Can you post an example of a string you are referring to?
-
Your question seems incomplete. If you want to make a php script "pretty" for a GUI (meaning output and any function controls), you need to read up on css and html. And integrate that into your script.
-
user login works on my localhost computer but not on my webhost
OldWest replied to viperjts10's topic in PHP Coding Help
be sure to mark thread as solved! -
user login works on my localhost computer but not on my webhost
OldWest replied to viperjts10's topic in PHP Coding Help
If this is the error you are facing as you noted: Then you should should also (in addition to the other recommendations in this thread) consider any white-space or any other html prior to the output of the session_start().. -
Code Critique: Using $_GET. Critique bashing welcome!
OldWest replied to OldWest's topic in PHP Coding Help
Completed with all recommendations.. For note, I want to pull the city_name to echo it on the page so the output is user friendly indicating which city is related to the results: <?php if (isset($_GET['id']) && ctype_digit($_GET['id'])) { $id = (int)$_GET['id']; } else { echo "Nice try! The id passed is not an integer."; } if (isset($_GET['city_name']) && is_string($_GET['city_name'])) { $city_name = (string)$_GET['city_name']; } else { echo "Nice try! The id passed is not a string."; } $posts_by_city_sql = "SELECT id, city_id, title FROM postings WHERE city_id='$id'"; if ($posts_by_city_results = mysqli_query($cxn, $posts_by_city_sql)) { $row_cnt = mysqli_num_rows($posts_by_city_results); if (mysqli_num_rows($posts_by_city_results)) { printf("<br />Congratulations! There are %d postings in: <strong>$city_name</strong>", $row_cnt); echo "<ul>"; while ($posts_by_city_row = mysqli_fetch_array($posts_by_city_results)) { echo "<li><a href='posting_details.php?id=$posts_by_city_row[id]'>$posts_by_city_row[title]</a></li>"; } echo "</ul>"; } else { printf("<br />We're sorry. There are %d postings in: <strong>$city_name</strong>", $row_cnt); } } else { trigger_error('Query failed ' . $posts_by_city_sql); exit(); } mysqli_free_result($posts_by_city_results); mysqli_close($cxn); ?> -
Code Critique: Using $_GET. Critique bashing welcome!
OldWest replied to OldWest's topic in PHP Coding Help
Completed the implement of thorpes recommendations. Now getting on data decisions and filtering. <?php $id = mysqli_real_escape_string($cxn, $_GET['id']); if (!ctype_digit($id)) { echo "Nice try. $id is not an integer."; exit(); } $city_name = mysqli_real_escape_string($cxn, $_GET['city_name']); if (!is_string($city_name)) { echo "Nice try. $city_name is not a string!"; exit(); } $posts_by_city_sql = "SELECT id, city_id, title FROM postings WHERE city_id='$id'"; if ($posts_by_city_results = mysqli_query($cxn, $posts_by_city_sql)) { $row_cnt = mysqli_num_rows($posts_by_city_results); if (mysqli_num_rows($posts_by_city_results)) { printf("<br />Congratulations! There are %d postings in: <strong>$city_name</strong>", $row_cnt); echo "<ul>"; while ($posts_by_city_row = mysqli_fetch_array($posts_by_city_results)) { echo "<li><a href='posting_details.php?id=$posts_by_city_row[id]'>$posts_by_city_row[title]</a></li>"; } echo "</ul>"; } else { printf("<br />We're sorry. There are %d postings in: <strong>$city_name</strong>", $row_cnt); } } else { trigger_error('Query failed ' . $posts_by_city_sql); exit(); } mysqli_free_result($posts_by_city_results); mysqli_close($cxn); ?> -
Code Critique: Using $_GET. Critique bashing welcome!
OldWest replied to OldWest's topic in PHP Coding Help
You might want to re-read my original reply. Its the argument passed to mysqli_num_rows that needs checking. (Well, both actually) Am I getting you? if (mysqli_num_rows($posts_by_city_results) !=true && $row_cnt != true) -
Code Critique: Using $_GET. Critique bashing welcome!
OldWest replied to OldWest's topic in PHP Coding Help
Interesting point and method. I like the casting thing. checking up on what ctype does.. Will mess around with this idea. Thanks o bunch -
Code Critique: Using $_GET. Critique bashing welcome!
OldWest replied to OldWest's topic in PHP Coding Help
had to use is_numeric() ... was this the correct point? <?php $id = mysqli_real_escape_string($cxn, $_GET['id']); if (!is_numeric($id)) { echo "Nice try. $id is not an integer."; exit(); } $city_name = mysqli_real_escape_string($cxn, $_GET['city_name']); if (!is_string($city_name)) { echo "Nice try. $city_name is not a string!"; exit(); } $posts_by_city_sql = "SELECT id, city_id, title FROM postings WHERE city_id='$id'"; $posts_by_city_results = (mysqli_query($cxn, $posts_by_city_sql)) or die("Was not able to grab the Postings!"); $row_cnt = mysqli_num_rows($posts_by_city_results); if (mysqli_num_rows($posts_by_city_results) != true) { printf("<br />We're sorry. There are %d postings in: <strong>$city_name</strong>", $row_cnt); } else { printf("<br />Congratulations! There are %d postings in: <strong>$city_name</strong>", $row_cnt); echo "<ul>"; while ($posts_by_city_row = mysqli_fetch_array($posts_by_city_results)) { echo "<li><a href='posting_details.php?id=$posts_by_city_row[id]'>$posts_by_city_row[title]</a></li>"; } echo "</ul>"; } mysqli_free_result($posts_by_city_results); mysqli_close($cxn); ?> -
Code Critique: Using $_GET. Critique bashing welcome!
OldWest replied to OldWest's topic in PHP Coding Help
Hmmm interestingly good point. I assume I would want to check this to ensure the data passed is as expected - more of a security check? I think is_int() will work for the id and is_string() would work for city_name.. hope I am getting your point correctly! Back at it... -
Code Critique: Using $_GET. Critique bashing welcome!
OldWest replied to OldWest's topic in PHP Coding Help
thorpe, no doubt my naming conventions are probably very annoying to an advanced php programmer like you , and yeah, it is a bit cumbersome and annoying to type those out! im only doing that so i can follow my code a bit easier while im grasping what is going on with it all.. thanks for your critique.. Here's what I've got now working as expected checking the TRUE status of ..num_rows.. Any other suggestions are of course welcome (don't need to be easy on me! ) <?php $id = mysqli_real_escape_string($cxn, $_GET['id']); $city_name = mysqli_real_escape_string($cxn, $_GET['city_name']); $posts_by_city_sql = "SELECT id, city_id, title FROM postings WHERE city_id='$id'"; $posts_by_city_results = (mysqli_query($cxn, $posts_by_city_sql)) or die("Was not able to grab the Postings!"); $row_cnt = mysqli_num_rows($posts_by_city_results); if (mysqli_num_rows($posts_by_city_results) != true) { printf("<br />We're sorry. There are %d postings in: <strong>$city_name</strong>", $row_cnt); } else { printf("<br />Congratulations! There are %d postings in: <strong>$city_name</strong>", $row_cnt); echo "<ul>"; while ($posts_by_city_row = mysqli_fetch_array($posts_by_city_results)) { echo "<li><a href='posting_details.php?id=$posts_by_city_row[id]'>$posts_by_city_row[title]</a></li>"; } echo "</ul>"; } mysqli_free_result($posts_by_city_results); mysqli_close($cxn); ?> -
As some of you know, I am still learning the fundamentals of good php code practice and have been working on a custom application for my own practice and personal schooling. My below code IS working as expected, but I wanted any ideas or critique on better, more secure, faster, etc methods of it.. Thanks for any input: <?php $id = mysqli_real_escape_string($cxn, $_GET['id']); $city_name = mysqli_real_escape_string($cxn, $_GET['city_name']); $posts_by_city_sql = "SELECT id, city_id, title FROM postings WHERE city_id='$id'"; $posts_by_city_results = (mysqli_query($cxn, $posts_by_city_sql)) or die("Was not able to grab the Postings!"); $row_cnt = mysqli_num_rows($posts_by_city_results); if ($row_cnt == 0) { printf("We're sorry. There are %d postings in: <strong>$city_name</strong>", $row_cnt); } else { printf("Congratulations! There are %d postings in: <strong>$city_name</strong>", $row_cnt); echo "<ul>"; while ($posts_by_city_row = mysqli_fetch_array($posts_by_city_results)) { echo "<li><a href='posting_details.php?id=$posts_by_city_row[id]'>$posts_by_city_row[title]</a></li>"; } // end while loop echo "</ul>"; } // end row_cnt if mysqli_free_result($posts_by_city_results); mysqli_close($cxn); ?>
-
How to create an associative array within a loop
OldWest replied to applebiz89's topic in PHP Coding Help
OScommerce and Zen Cart are two pretty flexible and well written (from my experience) shopping cart applications. Both are open source and are free to use. -
AbraCadaver, oh man.. i missed that one! just looked back at his dir structure... hope that solves it.
-
That's not an error. Thats array output of the directory! That's good news for you.. The directory is being read.. Now you can 1) either read your directories into an array OR 2) use your original script (just try this to see what happens) and use single quotes for your ourDir var.. see if that changes anything.
-
Hmmm.. could be folder permission issues, but you should be using this (as noted by a previous poster): error_reporting(E_ALL); ini_set('display_errors', '1'); On all testing you do.. These errors will give you correct insight in your problems. You say no errors are being generated? Are you developing locally or on a hosting server? And it's possible your php.ini might need to be tweaked for directory reading.. Could be a lot simpler than that, but just shooting in the dark right now.
-
Would you mind running this script please? What's the output: <?php $dir = '../templates'; $files1 = scandir($dir); print_r($files1); ?>