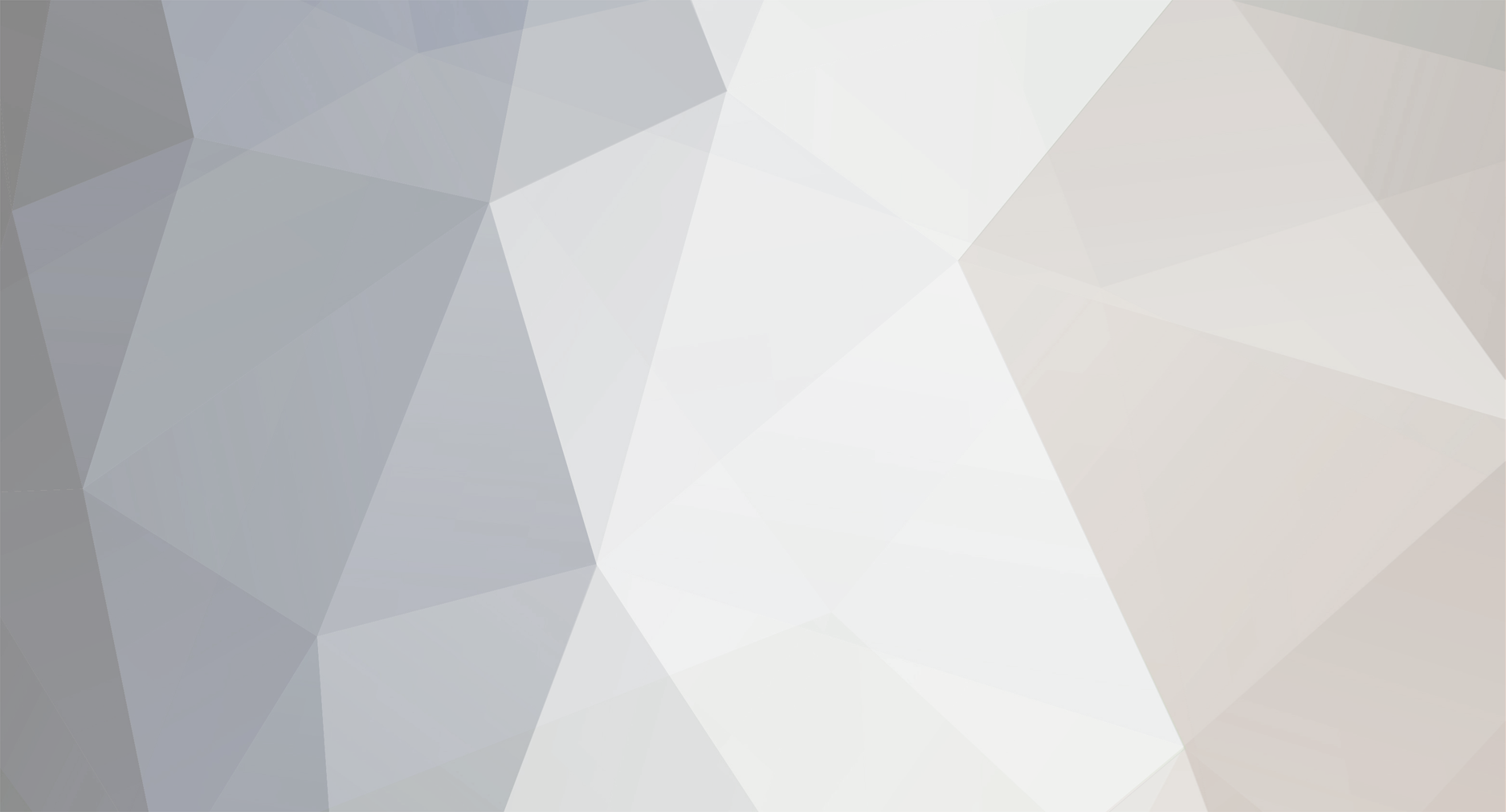
Hall of Famer
-
Posts
314 -
Joined
-
Last visited
Posts posted by Hall of Famer
-
-
Well if you have two dates in string format(Y-m-d), you can always use PHP's datetime extension. You simply cannot just compare two dates by calling the function date() on them, PHP cant compare the two strings properly. However, as of PHP 5.2.2 and later, its possible to compare two DateTime objects. Here's the best way to compare two dates retrieved from an external source(such as user input or sql database) in string format:
$today = new DateTime; $srdate = new DateTime($row['srdate']); if($today >= $srdate){ echo "Need New Date"; } else{ echo $srdate->format('F jS Y'); }
-
Well for the first question, I am not that sure. The reason to use factory method is that it is standardized and organized, it defines a way to approach a problem. Whether you find it useful or not is really up for your own experience and interpretation. In some applications it helps, in other circumstances it can stink. The wisest way to look at it is to get the patterns to work for you, not to force yourself to use a pattern just because it makes your script look professional.
For the 2nd one, yes you are getting it almost correct. Lets consider the example of an abstract factory VehicleFactory, which have concrete subclasses such as BMW, Benz, Ford, Volvo and Toyota. Each factory is responsible of generating vehicle component objects for various types of vehicles such as getEngine(), GetSteeringWheel(), getBrake(), getTyre() and so on. These methods generate various components objects such as BMWEngine, BenzEngine, FordBrake, VolvoBrake and ToyotaTyre, you can easily manage the creation of such objects using abstract factory pattern.
-
Yeah its possible, but the way you are using classes is somewhat weird. I dont think you grasp the concept of OOP nicely. Heres what you are supposed to do in class 2:
class Class1{ public function a(); public function c(){ $this->a(); } } class Class2{ public function b(){ $class1 = new Class1(); $class1->a(); } }
Or you can declare all methods to be static so you do not need to instantiate an object. It seems to me that you have no idea what a class does and how class methods(member functions) differ from procedural functions.
-
Of course I am not expecting people from this forum to engage in PHP's development process. Its a board where everyone can freely speak his opinion, and I have a point to discuss about PHP's lacking features that need to be improved. Its a matter of point, not a request to make something happen.
-
[/font][/color]
It illustrates a general lack of understanding.
Nope, not at all. It is the shallowness of your comment that triggered my reply. Yes, lets just stop developing PHP and make PHP 5.5 its final version already. Why keep requesting new features? Whatever we have now is part of the language, lets get used to it and get on with things.
-
I think autoloading makes this feature redundant, take kicken's example, is it really that much of a burden to have to type 5 more characters to make your code far more self-explanatory and easier to debug? Not to mention that you're only loading the classes that you actually use...
As for Exception and ArrayObject, couldn't you just:
use \Exception as Exception; use \ArrayObject as ArrayObject;
I can't test this as I'm on my Dad's laptop which doesn't have PHP installed, but it should work?
Yes it does work, but PHP has more than just Exception and ArrayObject in their built-in class library. Do you want to import them all manually? If so, write 20-30 lines of code that look completely the same in every script file.
It is comments like these that make your opinions less and less respectable.
How so? I see no difference between your post and mine. 'Its part of the language, PHP isnt Java/C#, you have to get used to it'. Gotta love this kind of quote.
-
It's just a non-issue IMO. Who really cares? It's one character and is part of the language. Learn to use it, and get on with things.
So we should get used to PHP being purely procedural and stop requesting for object oriented features back in the 90s, 'cause its 'part of the language'?
-
Thank for the support then. XD
Yeah, lets take a break from the general auto-import feature. Anyone here actually disagree that built-in classes should be auto-imported? I mean, dont you get tired of having to remind yourself of writing a backslash in front of the class names such as ArrayObject and Exception? Even if you dont think its a problem that many programmers end up getting errors for forgetting to put this backslash, its just way too inconvenient and ugly.
-
Yeah, there are ups and downs with wildcard import, I agree. But you have to understand that there are circumstances that you wont run into naming conflict while its more convenient to import an entire namespace. For instance, with PHP's built-in classes you want to use ArrayObject and Exception without having to add that backslash infront of their names. Another example is utility namespace package, in which all classes are used in the script. Like I pointed out before, there are always programmers who make mistakes, if they mistakenly import two namespaces that have naming conflict its their own problem. Just like newbies missing semicolons, there are always people who make this kind of errors, it cant be helped.
-
I use devshed a lot, but it seems that the admin hasnt been posting a lot of PHP articles lately.
-
If he answers my questions, then yes I will respond to his.
-
Merry Christmas, hopefully this is not way too late.
-
" mind elaborating why 'goto' is added in PHP 5.3 when its been argued as a misfeature by many experienced coders? You've gotta give people choices, let them make their own decision on how to write their scripts."
Good job answering your own question!
umm whats your point? PHP right now does not give us a choice for wildcard import, thats what I've been arguing about.
By the way, here's a question. Given the pseudo-code
use Fruit\*; use Colors\*; $o = new Orange();
Where is PHP supposed to find the Orange class?
Well ask Java's developers how they handle situations like this with their wildcard import.
Sure there are programmers who make mistakes like that, but it cant be helped. You see people getting errors of missing semicolons everyday, so PHP should allow users to conclude a line without semicolon? Oh yeah, all variables should be constants since some amateur programmers end up overwriting them in an unexpected fashion?
At least, PHP needs to provide auto-importing built-in classes such as ArrayObject and Exception.
-
How much have you actually used namespaces Hall of Famer? Most of your arguments refer to issues that I myself have not found to be an actual problem. Sure, there may be some annoyances, but no language can avoid those (annoyances).
Well many people found it not a problem for PHP to be a purely procedural language back in the 90s, so its redundant to bring in OOP? The point is that there's not a way a programming language can meet every coder's need, but a real mature programming language offers a great deal of flexibility to various programmers. The fact that you do not see it a problem not not dictates that the others feel the same way as you do. If you still dont understand, mind elaborating why 'goto' is added in PHP 5.3 when its been argued as a misfeature by many experienced coders? You've gotta give people choices, let them make their own decision on how to write their scripts. Yes no language can avoid certain annoyances, but it's not an excuse to sit here and refuse to improve. You cant be perfect, yet you can always get better.
-
Whats most annoying about PHP's namespace is that you have to use \ in front of PHP's builtin-class names such as Exception, ArrayObject and even stdclass. Not mentioning how ugly it is to write something like \ArrayObject, this sure makes it much easier to run into bugs in which the evil backslash \ is missing. I know PHP did this for a reason since you may end up defining your own classes that share the same name with PHP's built-in classes, but in most circumstances its not the case. If PHP provides a wildcard import mechanism, at least we can import all classes from PHP's global space so that we dont have to remind ourselves to use backslash \ sign in front of these built-in class names.
-
Well you can try out Mysidia Adoptables, its a script I am developing. The current version is still not that good, since I am in the middle of the process of rewriting the code in OOP/MVC. But still, it comes with many features such as leveling, breeding, trade, and itemshop.
Link: www.mysidiaadoptables.com
-
I personally do believe PHP needs to add support for wildcard import of an entire subnamespace. Again like I pointed out before, it is a choice given to developers who want to use it. Sure there are people who contend that its not a good practice, but Id say its a matter of choice and opinion. Without wildcard import there are times I have to write 10+ or even 15+ use statement in a script file. Not sure if it does increase server load, hopefully not since it seems that I have to live with this for a few months or even years.
-
Well PHP 5.5 Alpha is already released, and the new features for PHP 5.5 is pretty much set in stone and not subject to major changes anymore. Tbh it does not seem to be as much of an upgrade over PHP 5.4 compared to what an impact PHP 5.3 and 5.4 have been over older versions. But this is comprehensible considering it has not even been a year since PHP 5.4.0 came out, PHP dev team probably is trying to make more frequent releases but less new additions each time.
So its about time to take a step further and look at what PHP 5.6 can implement. I am bringing up a wishlist here, and I will be realistic this time. PHP sure is better off going pure OO like C# and Ruby, but I personally do not see it happening at least until PHP 6, the next major release. The fact that PHP has to compensate for a good number of newbie programmers makes it even harder goal to achieve. Still, for minor releases and maintenance releases they can at least add more OO functionalities that are missing from the current PHP core, lets see what are possible candidates to be added to PHP 5.6:
1. C# Property Accessor Syntax:
Well this has actually been proposed a while ago, many people were eager to see it implemented into PHP 5.5 but it never did. Its kinda pity, but for now we have to live with what we have. For PHP 5.6 though, I'm really looking forward to seeing this new feature being part of PHP core. The proposal on PHP RFC can be found here:
https://wiki.php.net...-as-implemented
There aint much to explain at this point, I like most of the recommendations made in that page. It not only simplifies the creation of getter and setter methods, but also provides a clean and flexible way of accessing properties. The final property and final property methods proposal is pretty neat too, its usefulness will come in handy.
2. Inner Class/Interface and Annoymous Class:
This may sound a bit ambitious for PHP 5.6, while I do believe there is a chance it will happen. Inner classes are rather useful in composite pattern design, it works best for a clean one to one composite relationship such as a user and a userprofile. Anonymous classes can help too, they can achieve the same effect as inner class and some prefer this style of programming. It should also be possible to define inner interfaces, which can be implemented by inner classes. It may even be a good idea to have inner trait inside an outer trait, perhaps thats already way too complicated? An example is shown below:
class User{ public $uid; public $username; private $password; private $email; interface UserInterface{ // declaration of public methods inside. } class UserProfile implements UserInterface{ // codes for user profile inside. } class UserLog implements UserInterface{ // codes for user log inside. } }
Oh yeah, I just realized this has not even been posted on PHP RFC at this point, which does not make any sense. Perhaps it is a difficult feature to implement? Hopefully someday it will become possible.
3. Revision of property/method/class visibility system:
Umm at this point PHP has public, private and protected visibility for properties and methods, just like what it used to be since PHP 5.0. With namespace emerging in PHP 5.3 however, the system may need to be modified slightly. At this point there is no difference between the keyword 'var' and 'public', although the former may as well become deprecated in future. Instead of having 'var' as obsolete, it can prove to be actually useful.
In Java the visibility public means the property/method/class can be accessed anywhere in the program, while without the public keyword it can only be used by classes in the same package. PHP sure can borrow this idea. I mean, the keyword 'var' may as well become property accessible by classes in the same namespace. Similarly for methods, if a method is not declared to be public, private or protected, it can only be used by classes in their very namespace. A public property/method, on the other hand, is globally accessible. An example of this revision is shown below:
namespace N1{ class C1{ public $p1 = "public property"; var $p2 = "namespace restricted property"; public function m1(){ return "This is a method accessible globally"; } function m2(){ return "This is a method only accessible in the namespace N1"; } } } namespace N2{ $class = new \N1\C1; echo $class->p1; echo $class->m1(); // This will work, and return the appropriate result. echo $class->p2; echo $class->m2(); // Fatal error: Cannot access nonpublic property/method p2, m2 in a different namespace! }
How does that look? Well PHP may as well consider adding support for class visibility when Inner class/interface becomes available, this way you can declare an inner class/interface as private or protected. Right now a class can only be declared abstract or final, not even static. Perhaps its about time for PHP to make a push?
4. Improvement in PHP Namespace:
In my earlier thread about PHP namespacing missing an import feature, I brought up the idea that it should be allowed for client coders to import an entire subnamespace and refer to the classes inside this subnamespace by their short-name only. It becomes quite controversial, some argue it is not a good practice. I can say Static Properties/Methods aint good practices either, nor is with 'goto' statement added in PHP 5.3, and PHP implemented them anyway. The point is to give programmers another choice they can make flexibly, not mentioning how hard it is to actually prove a feature is a mis-feature until it has been used and examined in multiple applications for years.
Consider the situation in which you do not have 5+ layers of subnamespaces, this will simplify coding quite a bit. Here's an example:
namespace N1{ class C1{} class C2{} class C3{} } namespace N2{ use \N1; $class1 = new C1; $class2 = new C2; $class3 = new C3; }
On the other hand, I feel that its a good time for PHP to begin reorganizing their built-in libraries into appropraite namespaces rather than in a global namespace as they are today. The SPL library for instance, may as well become an SPL namespace so that you instantiate classes of ArrayObject and SPLFileInfo as SPL\ArrayObject and SPL\FileInfo. Functions can be grouped into different namespaces too, although as an OOP programmer I do not even think its necessary to have functions not in class scope at all. Its up for them to decide anyway.
5. Enum language structure:
Another feature already proposed in PHP RFC, which makes me feel optimistic that it will be available in not so distant future(maybe a year or two, hopefully not too long). Enum can help with PHP programming just like it has been in many other programming languages, its usefulness needs no more justification. The RFC post can be found below, looks like someone was able to implement it a while ago but it never actually went into voting phase. Kinda strange if you ask me, perhaps demand for it aint that high. Still, it can be a valuable addition to PHP 5.6, and definitely not as overwhelming as inner/anonymous classes:
So what do you think?
-
Yeah, sorta seems that way to me too, what is $row['title']? Perhaps you should use this instead:
$_GET['title']
Not sure if this helps though, it's difficult to help you unless we know what this $row['title'] is supposed to mean.
-
freelancer.com is your friend.
-
Well there are popular ORM products such as Doctrine and Propel, while some frameworks such as Zend and CakePHP have their own ORM method. It is something I did not really quite know of while learning MVC, just grasped a bit of taste of how ORM works very recently. What do you think about ORM? Is it commonly used in most web applications nowadays? Or is it still only minority of web developers apply ORM in their software design?
-
I found it easier to learn PHP though, probably due to my tiny bit of knowledge about C++ and Java before touching this programming language. Python is fine for me too, unlike Ruby which is far more difficult than probably PHP and Python combined.
-
Well in my script I first use md5 on the raw password, then apply sha1 on the combined username and md5'd password. Finally the new string is concatenated with salt and pepper, a sha512 function is then acted on the combined string to give a final result. The difference between pepper and salt is that the former is hard coded for each site/application, while salt is user-specific and alterable. Heres the way I did it lol:
public function encrypt($username, $password, $salt){ $config = Registry::get("config"); $pepper = $config->peppercode; $password = md5($password); $newpassword = sha1($username.$password); $finalpassword = hash('sha512', $pepper.$newpassword.$salt); return $finalpassword; }
Kinda weird isnt it?
-
No, you only have to write one line like this:
use \Model\User;
Then when you use those classes, do:
new User\Member or new User\Admin
Nope, this is not what a well-designed namespace system should work. The fact that you have to refer to the class as User\Member and User\Admin instead of just Member and Admin simply kills the purpose of importing namespace. In your case, why not just write the full class name in the format of \Model\User\Member and \Model\User\Admin? How much does it simplify by getting rid of the \Model part? Unless you have a real complicated namespace like App\Model\User\Member\Profile\ProfileField\EditableProfileField, I dont see how it helps practically when you still have to write User\ as prefix for your classes.
Php Or Python
in Other Programming Languages
Posted
I find it easier to learn PHP, Python is not that bad but I just dont feel the need to learn it. Ruby is definitely by far the hardest to learn among all web programming languages, which is only recommended for programmers with at least 2-3 years of coding experience.