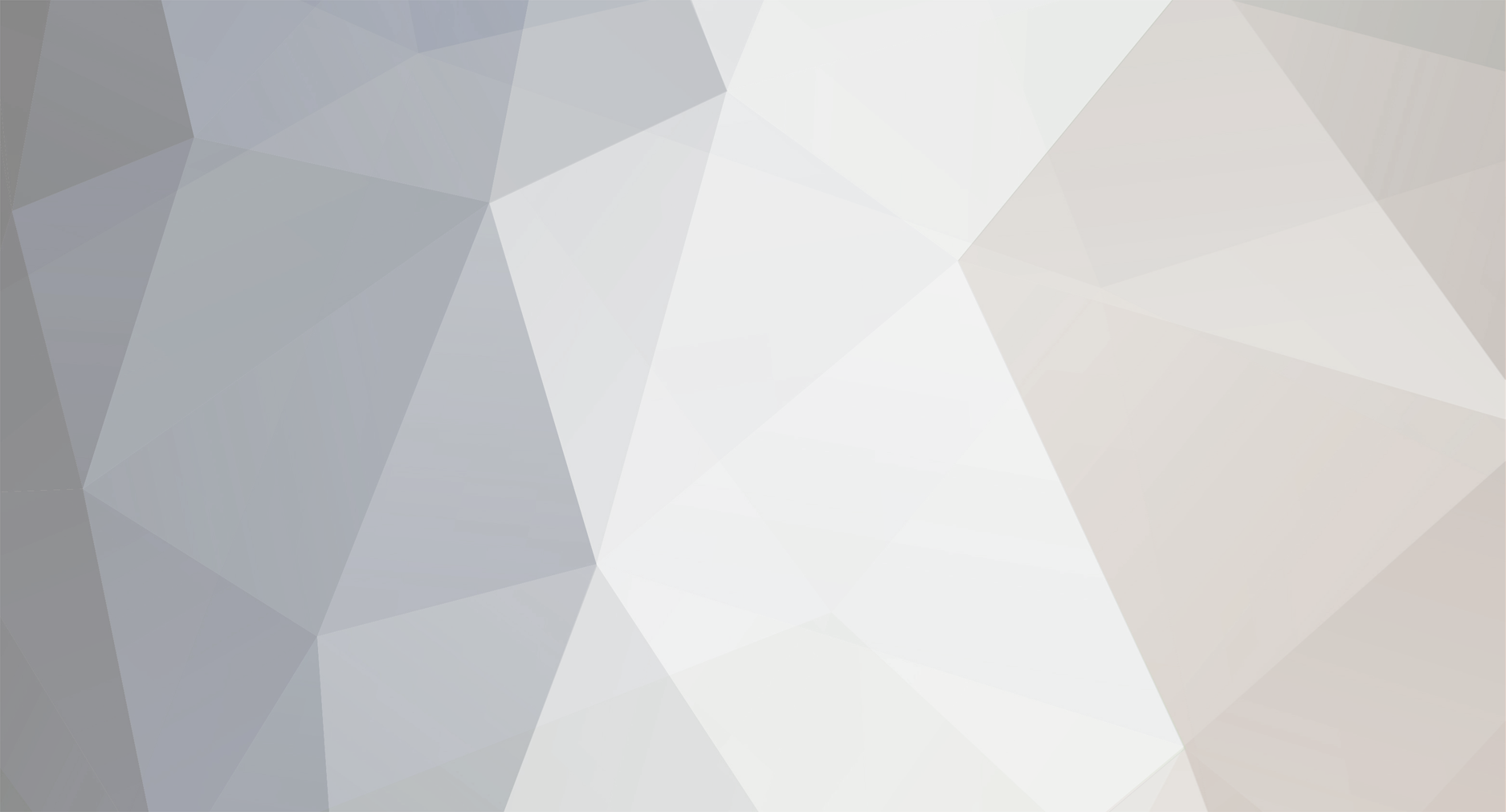
Hall of Famer
-
Posts
314 -
Joined
-
Last visited
Posts posted by Hall of Famer
-
-
Well PHP5 has a function __autoload() which makes it possible to load classes when needed, thereby eliminating excessive lines of include() or require(). Now I have a question about this feature, lets assume we have a class file like this:
interface Shop{ public function getshop(); public function displayshop(); public function purchase($name); public function sell($name); public function rent($name); } class Foodshop implements Shop{ // codes here } class Bookshop implements Shop{ // codes here } class Electronicshop implements Shop{ // codes here } class Petshop implements Shop{ // codes here } class Auction implements Shop{ // codes here, note the class name auction does not contain shop substring... }
If I instantiate an object from one of these classes that implement (or extend) an interface(or an abstract class), how should I design my __autoload() function so that this class file will be loaded properly? Please help.
-
You could try passing an array as a parameter and make the array with associative keys.
Thats a good suggestion, the problem is that some of these parameters are already arrays...
-
Changed createtable() to buildheader(), guess this way it wont create any confusion. I tried to bring everything from the original createtable() method to constructor, but the constructor ended up having way too many arguments and becomes ugly. Is there a way anyone can suggest such that I can reduce the number of arguments to no greater than five?
-
It seems a bit overwrought for something that could simply be created in a loop in a view. Overhead without much utility.
That said, I have some other issues with it, too. Why are there both a createTable and buildTable method? From a usability POV, those methods tell anyone using the class the same thing. Build and create are synonyms. All you're doing is making a muddled API.
Also, from a usability POV, I don't see why both table data and table formatting options can't be passed into the constructor. You have three methods doing what one should handle.
Pass options in as either an array or object, and handle them all in one place. Right now, your methods handle arbitrary things (createTable and buildTable both handle alignment and CSS, while the constructor handles the background). There's no clear design intent with that.
Remember DRY - Don't Repeat Yourself. Code repetition is a symptom of bad design. Simplify.
I see what you mean, thanks for the comment. And yeah, I could as well just have passed table formatting to the constructor. This way I will get rid of the createtable() method.
The reason why the buildtable() method is designed this way is that the alignments may change from each row, so are with the styles and maybe even background colors/images. The default option has all rows adopting the same alignment and style, but I try to make it flexible enough so that this can be changed at anywhere, anytime.
-
Well this is just a rough example, you can use it in different ways. For instance, retrieving database information and pass it to the table class.
And if you have any ideas on how to make a strictly non-hard coded table class, please lemme know and Id love to hear.
-
Well I will add attributes supports next. The reason to use this other than plain HTML is that, well, I have too many hard coded html tables in the script and the script files just look ugly.
-
This is a table class I created, the purpose of doing this is that the software I am working on has too many hard coded php tables and forms. It definitely needs some improvement, but I am guessing it is quite useful to construct simple tables, especially for tables used to show database results.
The class file:
<?php class Table { public $tablename; public $columns = array(); public $columnwidth; public $tablewidth; public $style; public $alignment; public $cells = array(); public $background = array(); public $content; public function __construct($tablename, $columns, $columnwidth = "", $tablewidth = "", $background = ""){ // Create the table $this->tablename = $tablename; if(!is_array($columns)) die("Cannot create a table with only one column..."); if(is_array($columnwidth) and count($columns) != count($columnwidth)) die("Specification of column width is incorrect, please report this to administrator."); $this->columns = $columns; if(is_array($columnwidth)){ foreach($columnwidth as $width){ $this->columnwidth[] = "width='{$width}'"; } } elseif(!empty($columnwidth) and !is_array($columnwidth)) $this->columnwidth = " width='{$columnwidth}'"; else $this->columnwidth = ""; $this->tablewidth = $tablewidth; $this->background = $background; $this->content = "<br>"; } public function createtable($alignment = "", $style = "", $border = "yes"){ // This method builds the interface of the table, it must be chained with showtable() or buildtable() $this->alignment = $alignment; $this->style = $style; $this->columns = $this->formattable($this->columns); // Construct the basic table structure $this->content .= "</br><table"; $this->content .= (!empty($this->tablewidth))?" width='{$this->width}'":""; $this->content .= ($border == "yes")?" border='1'":""; $this->content .= (!empty($this->background))?" {$this->background[0]}='{$this->background[1]}'>":">"; // Create the very first row of table $i = 0; $this->content .= "<tr>"; foreach($this->columns as $column){ $this->content .= "<td {$this->columnwidth[$i]}>{$column}</td>"; $i++; } $this->content .= "</tr>"; return $this; } public function buildtable($cells, $alignment = "", $style = ""){ // This method will build the body of our table, it must be chained with showtable() or endtable() $this->content .= "<tr>"; $this->alignment = (!empty($alignment))?$alignment:$this->alignment; $this->style = (!empty($style))?$style:$this->style; $this->cells = $this->formattable($cells); // Now it is time to construct a row of our table $i = 0; foreach($this->cells as $cell){ $this->content .= "<td>{$cell}</td>"; $i++; } $this->content .= "</tr>"; return $this; } private function formattable($text){ // Thie method formats the content of tables with alignment or style, can only be called witin the table class if(!is_array($text)) die("Cannot format the table content."); if(!empty($this->style)){ if(is_array($this->style) and count($this->style) != count($text)) die("Cannot specify the style of table columns..."); for($i = 0; $i < count($text); $i++){ $text[$i] = (is_array($this->style))?"<{$this->style[$i]}>{$text[$i]}</{$this->style[$i]}>":"<{$this->style}>{$text[$i]}</{$this->style}>"; } } if(!empty($this->alignment)){ if(is_array($this->alignment) and count($this->alignment) != count($text)) die("Cannot specify alignment of table columns..."); for($i = 0; $i < count($text); $i++){ $text[$i] = (is_array($this->alignment))?"<{$this->alignment[$i]}>{$text[$i]}</{$this->alignment[$i]}>":"<{$this->alignment}>{$text[$i]}</{$this->alignment}>"; } } return $text; } public function showtable(){ // This method allows the page to show an unfinished table, incase control blocks, loops or forms need to be used $content = $this->content; unset($this->content); return $content; } public function endtable(){ // This method terminates the construction of a table $this->content .= "</table>"; return $this->content; } } ?>
And here is an example, the output is also shown below:
<?php include("classes/class_tables.php"); $content = ""; $table = new Table("Mytable", array("Column1", "Column2", "Column3", "Column4"), 100, 400, array("bgcolor", "#FFA500")); $content .= $table->createtable("left")->showtable(); $content .= $table->buildtable(array("r1c1","r1c2","r1c3","r1c4"), "center", "strong")->showtable(); $content .= $table->buildtable(array("r2c1", "r2c2", "r2c3", "r2c4"), "left", "u")->showtable(); $content .= $table->buildtable(array("r3c1", "r3c2", "r3c3", "r3c4"), "center", "li")->showtable(); $content .= $table->buildtable(array("r4c1", "r4c2", "r4c3", "r4c4"), "left", "strike")->endtable(); echo $content; ?>
So what do you think? Please do not hesitate to point out any problems you see from the codes, Id like to improve it as much as I can before using it in the applications of mine. Comments please?
-
Umm I suppose this should suffice. You can also expand the class Rand to include other methods in future.
class Rand{ static $Roman = array("I", "II", "III", "IV", "V", "VI", "VII", "VIII", "IX", "X"); public static function getrand($num){ if($num < 1) die("Cannot generate less than one number..."); for($i =0; $i<$num; $i++){ $rand = mt_rand(0,9); $randnum[$i] = self::$Roman[$rand]; } return $randnum; } } // To output 15 Roman characters generated from the above method, simply do this $result = Rand::getrand(15); print_r($result);
-
Well lets say I have a class called Private_Item with a property like $owner. The class itself and constructor will look like the way below: (Note the Private_Item class extends from a parent class called Item, this is not to be worried here)
class Private_Item extends Item{ private $iid; // The itemname and category properties already exist for parent class private $owner; private $quantity; private $status = "Available"; public function __construct($name, $owner, $quantity){ parent::__construct($name); $this->owner = new User($owner); $this->quantity = $quantity; } }
Whenever I define a variable like $item = new Private_Item($itemname, $itemowner, $quantity), the $itemowner is used as reference to instantiate a User object, and then passed to the property $this->owner. In this way, I have actually instantiated two objects at once. One for the item, the other for the user who owns this item.
I have a question about using PDO's fetch class method though. I'd like to use it to retrieve database info and instantiate a Private_Item object immediately after fetching it from MySQL database. The code looks like below:
$item = $stmt->fetch(PDO::FETCH_CLASS, "Private_Item");
The real question is, can the user object still be instantiated in the meantime? I am not quite sure about this, and I may end up having only one $item object but its property $item->owner is not. For those of you familiar with advanced PDO stuff, please lemme know if I can instantiate a subsequent object with the method fetch(PDO::FETCH_CLASS). Thanks.
-
I wouldn't consider that a problem. Scope is a necessary part of programming, especially OOP, where objects are supposed to have hard boundaries. But, even in a procedural environment, scope and encapsulation allow you to create modular code through functions.
The thing to remember is that if your code has an external dependency, like a function that has to use a db connection, you need to pass that dependency to the code that uses it in an explicit way. The way PHP (and other languages) do it is via the argument list.
In OOP it's actually a bit easier, as you only need to inject the dependency in once, either right when you need it, or when the object is created. You can store the dependency as an object member, and use it whenever you want. And, since objects are always passed by reference, the dependency object can be passed to others with little overhead.
umm dependency injection? Mind explaining a bit about that? Thx.
-
No, thorpe is saying to never use globals at all, and to pass your db into the functions via their argument lists, like you did in the code above.
The same general approach would be used in OOP as well.
I see, so the problem still occurs even after the conversion into fully object-oriented codes is complete? Oh man what a pain, but I get what you are saying now.
-
So you are saying that registering globals for database information actually is a good practice? I thought using global variables is always bad unless you work on an extremely small project.
And if not, I have to pass the database info in all my functions like this?
function func1($db, $query){ $stmt = $db->prepare($query); $stmt->execute(); } function func2($db, $query2){ $stmt = $db->exec($query2); } function func3($db, $query3){ $stmt = $db->prepare($query3); $stmt->execute(); $obj = $stmt->fetchObject(); return $obj; }
And there is no other way to improve the script?
-
Well I am encountering this dilemma at the moment. The project I am working on has database instantiated with PDO like this:
$dsn = "mysql:host={$dbhost};dbname={$dbname}"; $db = new PDO($dsn, $dbuser, $dbpass);
But there is a problem with variable scope in functions. Whenever I call a function, the database object $db is not carried over unless it is defined as global variable or in superglobal array like this: (replacing $GLOBALS['db'] with $db will give an error, saying its not an object)
$GLOBALS['db'] = $db; function myfunc($query){ $stmt = $GLOBALS['db']->prepare($query); $stmt->execute(); }
And then I get the problem of global variables. They are not secure and require lots of memories to store, definitely not a good practice for a project that is ever expanding. However, I cannot think of an easier way of rewriting the code without making it more complex and messy to read. Sure I can instantiate the database object in every function call, but this means the object is redefined again and again if I call multiple functions at the same time.
Perhaps I can resolve this problem by completely rewriting the codes to be fully Object oriented, but that will take weeks or even months. Can anyone of you think of a better way of passing database object variable to each individual function? Please help.
-
I see, thanks for your advice. The problem is that the css works with the non-OOP script, but not with the OOP script. Its weird though, since I do not believe I've made any mistakes designing the class Tab. I can post two screenshots later today so you can see the difference of the working script(without class/object) and the not-working script(with class/object).
umm I will give a try by removing the curly bracket, hopefully it will make things a bit better.
-
Well I asked why the tab class I designed will not work, this isnt a question? o_o
-
umm no one knows?
-
Well I have a user profile page with codes written like this:
<?php $filename = "profile"; include("functions/functions.php"); include("functions/functions_users.php"); include("functions/functions_adopts.php"); include("functions/functions_friends.php"); include("inc/lang.php"); include("inc/bbcode.php"); //***************// // START SCRIPT // //***************// // This page handles user profiles and shows the site members... $user = $_GET["user"]; $page = $_GET["page"]; if($user != ""){ // We have specified a specific user who we are showing a profile for... // See if the user exists... $article_title = "{$user}'s Profile"; $query = "SELECT * FROM {$prefix}users, {$prefix}users_contacts, {$prefix}users_options, {$prefix}users_profile, {$prefix}users_status WHERE {$prefix}users.username = '{$user}' AND {$prefix}users_contacts.uid = {$prefix}users.uid AND {$prefix}users_options.uid = {$prefix}users.uid AND {$prefix}users_profile.uid = {$prefix}users.uid AND {$prefix}users_status.uid = {$prefix}users.uid AND {$prefix}users_contacts.username = {$prefix}users.username AND {$prefix}users_options.username = {$prefix}users.username AND {$prefix}users_profile.username = {$prefix}users.username AND {$prefix}users_status.username = {$prefix}users.username"; $result = runquery($query); $row = mysql_fetch_array($result); if($row['username'] == $user){ // First let's initiate tab system! include("inc/tabs.php"); include("css/tabs.css"); $article_content = "<div id='page-wrap'><div id='profile'> <ul class='nav'> <li class='nav0'><a href='#visitormessage'>Visitor Message</a></li> <li class='nav1'><a href='#aboutme' class='current'>About Me</a></li> <li class='nav2'><a href='#adopts'>Adoptables</a></li> <li class='nav3'><a href='#friends'>Friends</a></li> <li class='nav4 last'><a href='#contactinfo' class='last'>Contact Info</a></li> </ul><div class='list-wrap'>"; // Format user profile information... $id = $row['uid']; $ccstat = cancp($row['usergroup']); $website = (empty($row['website']))?"No Website Information Given":"<a href='{$row['website']}' target='_blank'>{$row['website']}</a>"; $facebook = (empty($row['facebook']))?"No Facebook Information Given":"<a href='{$row['facebook']}' target='_blank'>{$row['facebook']}</a>"; $twitter = (empty($row['twitter']))?"No Twitter Information Given":"<a href='{$row['twitter']}' target='_blank'>{$row['twitter']}</a>"; $msn = (empty($row['msn']))?"No MSN Information Given":$row['msn']; $aim = (empty($row['aim']))?"No AIM Information Given":$row['aim']; $yahoo = (empty($row['yahoo']))?"No YIM Information Given":$row['yahoo']; $skype = (empty($row['skype']))?"No YIM Information Given":$row['skype']; $row['username'] = ($ccstat == "yes")?"<img src='templates/icons/star.gif' /> {$row['username']}":$row['username']; $row['favpet'] = ($row['favpet'] == 0)?"None Selected":"<a href='http://www.{$domain}{$scriptpath}/levelup.php?id={$row['favpet']}' target='_blank'><img src='http://www.{$domain}{$scriptpath}/siggy.php?id={$row['favpet']}' border=0></a>"; // Here we go with the first tab content: Visitor Message $article_content .= "<ul id='visitormessage' class='hide'><strong><u>{$user}'s Profile Comments:</u></strong><br /><br /><table>"; $result = runquery("SELECT * FROM {$prefix}visitor_messages WHERE touser = '{$user}' ORDER BY vid DESC LIMIT 0, 10"); while($vmessage = mysql_fetch_array($result)){ $date = substr_replace($vmessage['datesent']," at ",10,1); $query2 = "SELECT * FROM {$prefix}users, {$prefix}users_profile WHERE {$prefix}users.username = '{$vmessage['fromuser']}' AND {$prefix}users_profile.uid = {$prefix}users.uid AND {$prefix}users_profile.username = {$prefix}users.username"; $result2 = runquery($query2); $sender = mysql_fetch_array($result2); $article_content .= "<tr> <td><img src='{$sender['avatar']}' width='40' height='40'></br></td> <td><a href='profile.php?user={$sender['username']}'>{$vmessage['fromuser']}</a> ({$date}) <a href='vmessage.php?act=view&user1={$user}&user2={$vmessage['fromuser']}'> <img src='templates/icons/status.gif'> </a> </br>{$vmessage['vmtext']} </br></td> <td><a href='vmessage.php?act=edit&vid={$vmessage['vid']}'> <img src='templates/icons/cog.gif'> </a> <a href='vmessage.php?act=delete&vid={$vmessage['vid']}'> <img src='templates/icons/delete.gif'> </a></br></td></tr>"; } if($isloggedin == "yes"){ if(isbanned($loggedinname) == 1){ $article_content .= "</table>It seems that you have been banned from this site and thus cannot send profile comment."; } else{ $article_content .= "</table> To Post a profile comment, please write your message in the text area below: <form action='profile.php?user={$user}' method='post'> <textarea rows='10' cols='50' name='vmtext' wrap='physical' ></textarea><br> <input type='submit' name='Submit' value='Submit'></form>"; $vmcontent = $_POST["vmtext"]; // Now check if the two users are friends... if($vmcontent != ""){ $datesent = date("Y-m-d H:i:s"); // $date = "2010-23-03 21:02:35"; $fromuser = $loggedinname; $touser = $user; runquery("INSERT INTO {$prefix}visitor_messages (vid, fromuser, touser, datesent, vmtext) VALUES ('', '$fromuser', '$touser' , '$datesent', '$vmcontent')"); $article_content .= "<p>Your comment for user has been posted. You may now view your conversation with {$user} from <a href='vmessage.php?act=view&user1={$fromuser}&user2={$touser}'>here</a>.</p>"; } } } else{ $article_content .= "</table>Guests cannot post profile comment, sorry..."; } // Now the second tab: About me... $article_content .= "</ul><ul id='aboutme'><li><strong><u>{$lang_basic_info} {$user}</u></strong><br /><br /> <img src='{$row['avatar']}' border=0 width='100' height=100 /><br /> <strong>Member Since:</strong> {$row['membersince']}<br /><br /> Gender: {$row['gender']}<br /> Favorite Color: {$row['color']}<br /> Nickname: {$row['nickname']}<br /> Bio: <br /> {$row['bio']}<br /></li>"; // The third tab: Adopts... $article_content .= "</ul><ul id='adopts' class='hide'><h2>.:AdoptSpotlight:.</h2><br /> {$row['favpet']}<br />{$row['about']}<br /><br /> <strong><u>{$user}'s Pets:</u></strong><br /><br />"; $query = "SELECT COUNT(*) AS pets FROM {$prefix}owned_adoptables WHERE owner = '{$user}'"; $result = runquery($query); $total = mysql_fetch_array($result); if($total['pets'] > 0){ $rowsperpage = 15; $totalpages = ceil($total['pets'] / $rowsperpage); if(is_numeric($page)) $currentpage = $page; else $currentpage = 1; if($currentpage > $totalpages) $currentpage = $totalpages; if($currentpage < 1) $currentpage = 1; $offset = ($currentpage - 1) * $rowsperpage; $query = "SELECT * FROM {$prefix}owned_adoptables WHERE owner = '{$user}' LIMIT {$offset}, {$rowsperpage}"; $result = runquery($query); while($row = mysql_fetch_array($result)){ $image = getcurrentimage($row['aid']); $article_content .= "<a href='levelup.php?id={$row['aid']}'><img src='{$image}' border='0' /></a>"; } $article_content .= "<br />"; if($currentpage > 1){ $newpage = $currentpage - 1; $article_content .= "<strong><a href='profile.php?user={$user}&page={$newpage}'><img src='templates/icons/prev.gif' border=0> Previous Page</a></strong> "; } else $article_content .= "<strong><img src='templates/icons/prev.gif' border=0> Previous Page</strong> "; if($currentpage < $totalpages){ $newpage = $currentpage + 1; $article_content .= " :: <strong><a href='profile.php?user={$user}&page={$newpage}'>Next Page <img src='templates/icons/next.gif' border=0></a></strong> "; } else $article_content .= " :: <strong>Next Page <img src='templates/icons/next.gif' border=0></strong>"; } else{ $article_content .= "This user currently does not have any pets."; } // The fourth tab: Friends... $friendlist = getfriendid($user); $friendnum = getfriendnum($user); $article_content .= "</ul><ul id='friends' class='hide'>{$user} currently have {$friendnum} friends.<br /><table>"; if($friendnum != 0){ foreach($friendlist as $friendid){ $query = "SELECT * FROM {$prefix}users, {$prefix}users_profile WHERE {$prefix}users.uid = '{$friendid}' AND {$prefix}users_profile.uid = {$prefix}users.uid AND {$prefix}users_profile.username = {$prefix}users.username"; $result = runquery($query) ; $friendinfo = mysql_fetch_array($result); $uid = $friendinfo['uid']; $username = $friendinfo['username']; $friendgender = getfriendgender($username); $onlinestatus = getonlinestatus($username); $article_content .= "<tr><td style='text-align: left'><img src='{$friendinfo['avatar']}' border=0 width='60' height =60></td> <td><strong><a href='profile.php?user={$username}'>{$username}</a></strong> {$friendgender}<br />{$friendinfo['nickname']}<br />{$onlinestatus} <a href='{$friendinfo['website']}' target='_blank'><img src='templates/icons/web.gif'></a> <a href='messages.php?act=newpm&user={$username}'><img src='templates/icons/title.gif'></a></td>"; $article_content .= ($user == $loggedinname)?"<td style='text-align: right'><br /><br /><br /><a href='friends.php?act=delete&uid={$uid}'>Break Friendship </td></tr>":"</tr>"; } } $article_content .= "</table>"; // The last tab: Contact Info! $article_content .= "</ul><ul id='contactinfo' class='hide'><img src='templates/icons/web.gif' /> {$website}<br /> <img src='templates/icons/facebook.gif' /> {$facebook}<br /> <img src='templates/icons/twitter.gif' /> {$twitter}<br /> <img src='templates/icons/aim.gif' /> {$aim}<br /> <img src='templates/icons/msn.gif' /> {$msn}<br /> <img src='templates/icons/yahoo.gif' /> {$yahoo}<br /> <img src='templates/icons/skype.gif' /> {$skype}<br /> <img src='templates/icons/title.gif' /> <a href='messages.php?act=newpm&user={$user}'>Send {$user} a Private Message</a><br /> <img src='templates/icons/fr.gif' /><a href='friends.php?act=request&uid={$id}'>Send {$user} a Friend Request</a><br /> <br /></div></div>"; } else{ $article_content .= "Sorry, but we could not find a user in the system with the name {$user}. Please make sure you have the username right. The user's account may also have been deleted by the system admin."; } } else{ // We did not specify a user, so show the memberlist $article_title = "Memberlist"; $article_content = "Here are all of the members of this site, sorted by registration date.<br /><br />"; include("classes/class_pagination.php"); include("css/pagination.css"); $query = "SELECT * FROM {$prefix}users ORDER BY uid ASC"; $result = runquery($query); $rowsperpage = 15; $pagination = new Pagination($query, $rowsperpage, "http://www.{$domain}{$scriptpath}/profile.php"); $pagination->setPage($_GET
); $query = "SELECT * FROM {$prefix}users ORDER BY uid ASC LIMIT {$pagination->getLimit()},{$rowsperpage}"; $result = runquery($query); while ($row = mysql_fetch_array($result)){ $status = cancp($row['usergroup']); $star = ($status == "yes")?"<img src='templates/icons/star.gif' border=0' /> ":""; $article_content .= "<strong><a href='profile.php?user={$row['username']}'>{$star}{$row['username']}</a></strong><br />"; } $article_content .= "<br />{$pagination->showPage()}"; } //***************// // OUTPUT PAGE // //***************// echo showpage($article_title, $article_content, $date); ?>
In order to improve re-usability of the tabs, I attempted to create a file called class_tabs.php to make it object oriented. It did not work however, as the css is seriously messed up, and I have absolutely no idea why it did not.
Here is the class file:
<?php class Tab{ private $t_num; private $t_name = array(); private $t_alias = array(); private $t_content; private $t_default; private $t_hide; private $t_index = array(); private $t_last = array(); public function __construct($num, $tabs, $default=1){ $this->t_num = $num; $this->t_default = $default; $i = 0; foreach ($tabs as $key => $val){ $this->t_name[$i] = $key; $this->t_alias[$i] = $val; $this->t_index[$i] = ($i == $default-1)?" class='current":""; $this->t_index[$i] = ($i == $num-1)?" class='last":$this->t_index[$i]; $this->t_last[$i] = ($i == $num-1)?" last":""; $i++; } } public function createtab(){ if($this->t_num < 2 or $this->t_num > 5) throw new Exception("The number of tabs must be restricted between 2 to 5!",272); $this->t_content = "<div id='page-wrap'><div id='tab'><ul class='nav'>"; for($i=0; $i<$this->t_num; $i++){ $this->t_content .= " <li class='nav{$i}{$this->t_last[$i]}'><a href='#{$this->t_alias[$i]}{$this->t_index[$i]}'>{$this->t_name[$i]}</a></li>"; } $this->t_content .= "</ul><div class='list-wrap'>"; return $this->t_content; } public function starttab($index){ $this->t_hide = ($index == $this->t_default)?"":" class='hide'"; $this->t_content .= "<ul id='{$this->t_alias}'{$this->t_hide}>"; return $this->t_content; } public function endtab($index){ $this->t_content .= "</ul>"; if($index == $this->t_num) $this->t_content .= "</div></div>"; return $this->t_content; } } ?>
And this is the modified profile codes with OOP:
<?php $filename = "profile"; include("functions/functions.php"); include("functions/functions_users.php"); include("functions/functions_adopts.php"); include("functions/functions_friends.php"); include("inc/lang.php"); include("inc/bbcode.php"); //***************// // START SCRIPT // //***************// // This page handles user profiles and shows the site members... $user = $_GET["user"]; $page = $_GET["page"]; if($user != ""){ // We have specified a specific user who we are showing a profile for... // See if the user exists... $article_title = "{$user}'s Profile"; $query = "SELECT * FROM {$prefix}users, {$prefix}users_contacts, {$prefix}users_options, {$prefix}users_profile, {$prefix}users_status WHERE {$prefix}users.username = '{$user}' AND {$prefix}users_contacts.uid = {$prefix}users.uid AND {$prefix}users_options.uid = {$prefix}users.uid AND {$prefix}users_profile.uid = {$prefix}users.uid AND {$prefix}users_status.uid = {$prefix}users.uid AND {$prefix}users_contacts.username = {$prefix}users.username AND {$prefix}users_options.username = {$prefix}users.username AND {$prefix}users_profile.username = {$prefix}users.username AND {$prefix}users_status.username = {$prefix}users.username"; $result = runquery($query); $row = mysql_fetch_array($result); if($row['username'] == $user){ // First let's initiate tab system! include("inc/tabs.php"); include("css/tabs.css"); include("classes/class_tabs.css"); $profile_tabs = new Tabs(5, array("Visitor Message" => "visitormessage", "About Me" => "aboutme", "Adoptables" => "adopts", "Friends" => "friends", "Contact Info" => "contactinfo"), 2); $article_content = $profile_tabs -> createtab(); // Format user profile information... $id = $row['uid']; $ccstat = cancp($row['usergroup']); $website = (empty($row['website']))?"No Website Information Given":"<a href='{$row['website']}' target='_blank'>{$row['website']}</a>"; $facebook = (empty($row['facebook']))?"No Facebook Information Given":"<a href='{$row['facebook']}' target='_blank'>{$row['facebook']}</a>"; $twitter = (empty($row['twitter']))?"No Twitter Information Given":"<a href='{$row['twitter']}' target='_blank'>{$row['twitter']}</a>"; $msn = (empty($row['msn']))?"No MSN Information Given":$row['msn']; $aim = (empty($row['aim']))?"No AIM Information Given":$row['aim']; $yahoo = (empty($row['yahoo']))?"No YIM Information Given":$row['yahoo']; $skype = (empty($row['skype']))?"No YIM Information Given":$row['skype']; $row['username'] = ($ccstat == "yes")?"<img src='templates/icons/star.gif' /> {$row['username']}":$row['username']; $row['favpet'] = ($row['favpet'] == 0)?"None Selected":"<a href='http://www.{$domain}{$scriptpath}/levelup.php?id={$row['favpet']}' target='_blank'><img src='http://www.{$domain}{$scriptpath}/siggy.php?id={$row['favpet']}' border=0></a>"; // Here we go with the first tab content: Visitor Message $article_content .= "{$profile_tabs -> starttab(0)}<strong><u>{$user}'s Profile Comments:</u></strong><br /><br /><table>"; $result = runquery("SELECT * FROM {$prefix}visitor_messages WHERE touser = '{$user}' ORDER BY vid DESC LIMIT 0, 10"); while($vmessage = mysql_fetch_array($result)){ $date = substr_replace($vmessage['datesent']," at ",10,1); $query2 = "SELECT * FROM {$prefix}users, {$prefix}users_profile WHERE {$prefix}users.username = '{$vmessage['fromuser']}' AND {$prefix}users_profile.uid = {$prefix}users.uid AND {$prefix}users_profile.username = {$prefix}users.username"; $result2 = runquery($query2); $sender = mysql_fetch_array($result2); $article_content .= "<tr> <td><img src='{$sender['avatar']}' width='40' height='40'></br></td> <td><a href='profile.php?user={$sender['username']}'>{$vmessage['fromuser']}</a> ({$date}) <a href='vmessage.php?act=view&user1={$user}&user2={$vmessage['fromuser']}'> <img src='templates/icons/status.gif'> </a> </br>{$vmessage['vmtext']} </br></td> <td><a href='vmessage.php?act=edit&vid={$vmessage['vid']}'> <img src='templates/icons/cog.gif'> </a> <a href='vmessage.php?act=delete&vid={$vmessage['vid']}'> <img src='templates/icons/delete.gif'> </a></br></td></tr>"; } if($isloggedin == "yes"){ if(isbanned($loggedinname) == 1){ $article_content .= "</table>It seems that you have been banned from this site and thus cannot send profile comment."; } else{ $article_content .= "</table> To Post a profile comment, please write your message in the text area below: <form action='profile.php?user={$user}' method='post'> <textarea rows='10' cols='50' name='vmtext' wrap='physical' ></textarea><br> <input type='submit' name='Submit' value='Submit'></form>"; $vmcontent = $_POST["vmtext"]; // Now check if the two users are friends... if($vmcontent != ""){ $datesent = date("Y-m-d H:i:s"); // $date = "2010-23-03 21:02:35"; $fromuser = $loggedinname; $touser = $user; runquery("INSERT INTO {$prefix}visitor_messages (vid, fromuser, touser, datesent, vmtext) VALUES ('', '$fromuser', '$touser' , '$datesent', '$vmcontent')"); $article_content .= "<p>Your comment for user has been posted. You may now view your conversation with {$user} from <a href='vmessage.php?act=view&user1={$fromuser}&user2={$touser}'>here</a>.</p>"; } } } else{ $article_content .= "</table>Guests cannot post profile comment, sorry..."; } // Now the second tab: About me... $article_content .= "{$profile_tabs -> endtab(0)}{$profile_tabs -> starttab(1)} <li><strong><u>{$lang_basic_info} {$user}</u></strong><br /><br /> <img src='{$row['avatar']}' border=0 width='100' height=100 /><br /> <strong>Member Since:</strong> {$row['membersince']}<br /><br /> Gender: {$row['gender']}<br /> Favorite Color: {$row['color']}<br /> Nickname: {$row['nickname']}<br /> Bio: <br /> {$row['bio']}<br /></li>"; // The third tab: Adopts... $article_content .= {$profile_tabs -> endtab(1)}{$profile_tabs -> starttab(2)}<h2>.:AdoptSpotlight:.</h2><br /> {$row['favpet']}<br />{$row['about']}<br /><br /> <strong><u>{$user}'s Pets:</u></strong><br /><br />"; $query = "SELECT COUNT(*) AS pets FROM {$prefix}owned_adoptables WHERE owner = '{$user}'"; $result = runquery($query); $total = mysql_fetch_array($result); if($total['pets'] > 0){ $rowsperpage = 15; $totalpages = ceil($total['pets'] / $rowsperpage); if(is_numeric($page)) $currentpage = $page; else $currentpage = 1; if($currentpage > $totalpages) $currentpage = $totalpages; if($currentpage < 1) $currentpage = 1; $offset = ($currentpage - 1) * $rowsperpage; $query = "SELECT * FROM {$prefix}owned_adoptables WHERE owner = '{$user}' LIMIT {$offset}, {$rowsperpage}"; $result = runquery($query); while($row = mysql_fetch_array($result)){ $image = getcurrentimage($row['aid']); $article_content .= "<a href='levelup.php?id={$row['aid']}'><img src='{$image}' border='0' /></a>"; } $article_content .= "<br />"; if($currentpage > 1){ $newpage = $currentpage - 1; $article_content .= "<strong><a href='profile.php?user={$user}&page={$newpage}'><img src='templates/icons/prev.gif' border=0> Previous Page</a></strong> "; } else $article_content .= "<strong><img src='templates/icons/prev.gif' border=0> Previous Page</strong> "; if($currentpage < $totalpages){ $newpage = $currentpage + 1; $article_content .= " :: <strong><a href='profile.php?user={$user}&page={$newpage}'>Next Page <img src='templates/icons/next.gif' border=0></a></strong> "; } else $article_content .= " :: <strong>Next Page <img src='templates/icons/next.gif' border=0></strong>"; } else{ $article_content .= "This user currently does not have any pets."; } // The fourth tab: Friends... $friendlist = getfriendid($user); $friendnum = getfriendnum($user); $article_content .= "{$profile_tabs -> endtab(2)}{$profile_tabs -> starttab(3)}{$user} currently have {$friendnum} friends.<br /><table>"; if($friendnum != 0){ foreach($friendlist as $friendid){ $query = "SELECT * FROM {$prefix}users, {$prefix}users_profile WHERE {$prefix}users.uid = '{$friendid}' AND {$prefix}users_profile.uid = {$prefix}users.uid AND {$prefix}users_profile.username = {$prefix}users.username"; $result = runquery($query) ; $friendinfo = mysql_fetch_array($result); $uid = $friendinfo['uid']; $username = $friendinfo['username']; $friendgender = getfriendgender($username); $onlinestatus = getonlinestatus($username); $article_content .= "<tr><td style='text-align: left'><img src='{$friendinfo['avatar']}' border=0 width='60' height =60></td> <td><strong><a href='profile.php?user={$username}'>{$username}</a></strong> {$friendgender}<br />{$friendinfo['nickname']}<br />{$onlinestatus} <a href='{$friendinfo['website']}' target='_blank'><img src='templates/icons/web.gif'></a> <a href='messages.php?act=newpm&user={$username}'><img src='templates/icons/title.gif'></a></td>"; $article_content .= ($user == $loggedinname)?"<td style='text-align: right'><br /><br /><br /><a href='friends.php?act=delete&uid={$uid}'>Break Friendship </td></tr>":"</tr>"; } } $article_content .= "</table>"; // The last tab: Contact Info! $article_content .= "{$profile_tabs -> endtab(3)}{$profile_tabs -> starttab(4)}<img src='templates/icons/web.gif' /> {$website}<br /> <img src='templates/icons/facebook.gif' /> {$facebook}<br /> <img src='templates/icons/twitter.gif' /> {$twitter}<br /> <img src='templates/icons/aim.gif' /> {$aim}<br /> <img src='templates/icons/msn.gif' /> {$msn}<br /> <img src='templates/icons/yahoo.gif' /> {$yahoo}<br /> <img src='templates/icons/skype.gif' /> {$skype}<br /> <img src='templates/icons/title.gif' /> <a href='messages.php?act=newpm&user={$user}'>Send {$user} a Private Message</a><br /> <img src='templates/icons/fr.gif' /><a href='friends.php?act=request&uid={$id}'>Send {$user} a Friend Request</a><br /> <br />{$profile_tabs -> endtab(4)}"; } else{ $article_content .= "Sorry, but we could not find a user in the system with the name {$user}. Please make sure you have the username right. The user's account may also have been deleted by the system admin."; } } else{ // We did not specify a user, so show the memberlist $article_title = "Memberlist"; $article_content = "Here are all of the members of this site, sorted by registration date.<br /><br />"; include("classes/class_pagination.php"); include("css/pagination.css"); $query = "SELECT * FROM {$prefix}users ORDER BY uid ASC"; $result = runquery($query); $rowsperpage = 15; $pagination = new Pagination($query, $rowsperpage, "http://www.{$domain}{$scriptpath}/profile.php"); $pagination->setPage($_GET
); $query = "SELECT * FROM {$prefix}users ORDER BY uid ASC LIMIT {$pagination->getLimit()},{$rowsperpage}"; $result = runquery($query); while ($row = mysql_fetch_array($result)){ $status = cancp($row['usergroup']); $star = ($status == "yes")?"<img src='templates/icons/star.gif' border=0' /> ":""; $article_content .= "<strong><a href='profile.php?user={$row['username']}'>{$star}{$row['username']}</a></strong><br />"; } $article_content .= "<br />{$pagination->showPage()}"; } //***************// // OUTPUT PAGE // //***************// echo showpage($article_title, $article_content, $date); ?>
Incase you find it necessary, the css/tabs.css and inc/tabs.php files are provided below. They should be irrelevant to this problem though, but just incase..
css/tabs.css
<style type="text/css"> * body { font: 12px Georgia, serif; } html { overflow-y: scroll; } a { text-decoration: none; } a:focus { outline: 0; } p { font-size: 15px; margin: 0 0 20px 0; } #page-wrap { width: 640px; margin: 30px;} h1 { font: bold 40px Sans-Serif; margin: 0 0 20px 0; } /* Generic Utility */ .hide { position: absolute; top: -9999px; left: -9999px; } /* Specific to example one */ #profile { background: #eee; padding: 10px; margin: 0 0 20px 0; -moz-box-shadow: 0 0 5px #666; -webkit-box-shadow: 0 0 5px #666; } #profile .nav { overflow: hidden; margin: 0 0 10px 0; } #profile .nav li { width: 97px; float: left; margin: 0 10px 0 0; } #profile .nav li.last { margin-right: 0; } #profile .nav li a { display: block; padding: 5px; background: #959290; color: white; font-size: 10px; text-align: center; border: 0; } #profile .nav li a:hover { background-color: #111; } #profile ul { list-style: none; } #profile ul li a { display: block; border-bottom: 1px solid #666; padding: 4px; color: #666; } #profile ul li a:hover { background: #fe4902; color: white; } #profile ul li:last-child a { border: none; } #profile ul li.nav0 a.current, #profile ul.visitormessage li a:hover { background-color: #0575f4; color: white; } #profile ul li.nav1 a.current, #profile ul.aboutme li a:hover { background-color: #d30000; color: white; } #profile ul li.nav2 a.current, #profile ul.adopts li a:hover { background-color: #8d01b0; color: white; } #profile ul li.nav3 a.current, #profile ul.friends li a:hover { background-color: #FE4902; color: white; } #profile ul li.nav4 a.current, #profile ul.contactinfo li a:hover { background-color: #Eac117; color: white; } </style>
<?php if($filename == "profile"){ ?> <script src='http://ajax.googleapis.com/ajax/libs/jquery/1.6.1/jquery.min.js'></script> <script src="js/tabs.js"></script> <script> $(function() { $("#profile").organicTabs(); }); </script> <? } ?>
Please help...
-
Thanks for all your kind replies, I will give a try later tonight. But still, can anyone of you please show me a screenshot of how it appears on your computer? I still wonder why you said it was okay for you guys but couldnt receive the same result for myself.
-
umm anyone can help?
-
umm what do you mean it is working for you? I tried on both firefox and google chrome and the drop down menu wont show. Can you show me a screenshot of how it appears on your computer?
-
Well I've tried to use a sample drop down on the site I am developing, and then work on a PHP script to allow users to change navlinks/menu as they wish. I am already stuck at the very beginning though, the problem is that the css of drop down wont show, and the way it looks is just weird: (Note: Do not worry about the content of the drop down links so far, I will change them once the css is working...)
I've copy/pasted the template.html file(where the template is defined and included) and style.css file here, please help me find out why it wont work. I did upload the graphics to the correct location, they just wont show...
template.html:
<!DOCTYPE html> <head> <title>:BROWSERTITLE:</title> <link rel="stylesheet" href="templates/simple2-final/media/style.css" type="text/css" /> </head> <body> <div class="menu"> <ul> <li><a href="#" >Home</a></li> <li><a href="#" id="current">Products</a> <ul> <li><a href="#">Drop Down CSS Menus</a></li> <li><a href="#">Horizontal CSS Menus</a></li> <li><a href="#">Vertical CSS Menus</a></li> <li><a href="#">Dreamweaver Menus</a></li> </ul> </li> <li><a href="/faq.php">FAQ</a> <ul> <li><a href="#">Drop Down CSS Menus</a></li> <li><a href="#">Horizontal CSS Menus</a></li> <li><a href="#">Vertical CSS Menus</a></li> <li><a href="#">Dreamweaver Menus</a></li> </ul> </li> <li><a href="/contact/contact.php">Contact</a></li> </ul> </div> <div id="wrapper"> <table cellspacing="0" cellpadding="0"> <tr><td colspan="2" id="image"><span><a href="index.php">Mysidia Adoptables v1.3.0 Alpha</a></span></td></tr> <tr><td id="menu"> :SIDEFEED: </td><td id="content"> <h1>:ARTICLETITLE:</h1> <p>:ARTICLECONTENT:</p> </td></tr> <tr><td colspan="2" id="footer"> <p>Your Site ©Copyright Your Name Year - Year ★ Powered by <a href="http://www.mysidiaadoptables.com">Mysidia Adoptables</a></p> </td></tr> </table> </div> </body> </html>
style.css file:
html { background:#222; font-family:Verdana, Arial, Tahoma, sans-serif; color:#333; font-size:12px; } #wrapper { width:950px; margin:0 auto; } table { width:100%; background:#fff; } /* Here's for the top most links */ th { padding:10px; } th a { font-weight:bold; color:#000; text-decoration:none; display:block; width:25%; height:35px; font-size:14px; padding-top:15px; text-align:center; float:left; } /* Here is where you can change the backgrounds for the top links. */ .one { background:#1693A5; border-bottom:5px solid #222; } .two { background:#7FFF24; border-bottom:5px solid #222; } .three { background:#00cdac; border-bottom:5px solid #222; } .four { background:#c3ff68; border-bottom:5px solid #222; } th a:hover { background:#222; color:#fff; border-bottom:5px solid #aaa; } /* Next is our background for the title box, just save your image to the media folder, and put it's name and type in the box. */ #image { border-left:10px solid #fff; border-right:10px solid #fff; border-bottom:5px solid #fff; background:url("gemstone.jpg"); height:150px; } #image span { position:relative; font-size:25px; padding:10px; font-family:Georgia, Times New Roman, serif; } /* Change the color of the link for your site title here */ #image a{ color:#222; text-decoration:none; font-style:italic; font-weight:bold; text-shadow: 0px 1px 1px #eee; } #image a:hover { color:#7FFF24; text-shadow: 0px 1px 1px #fff; } /* Standard table cell definitions */ td { background:#fff; } .menu{ border:none; border:0px; margin:0px; padding:0px; font: 67.5% "Lucida Sans Unicode", "Bitstream Vera Sans", "Trebuchet Unicode MS", "Lucida Grande", Verdana, Helvetica, sans-serif; font-size:14px; font-weight:bold; } .menu ul{ background:#333333; height:35px; list-style:none; margin:0; padding:0; } .menu li{ float:left; padding:0px; } .menu li a{ background:#333333 url("images/seperator.gif") bottom right no-repeat; color:#cccccc; display:block; font-weight:normal; line-height:35px; margin:0px; padding:0px 25px; text-align:center; text-decoration:none; } .menu li a:hover, .menu ul li:hover a{ background: #2580a2 url("images/hover.gif") bottom center no-repeat; color:#FFFFFF; text-decoration:none; } .menu li ul{ background:#333333; display:none; height:auto; padding:0px; margin:0px; border:0px; position:absolute; width:225px; z-index:200; /*top:1em; /*left:0;*/ } .menu li:hover ul{ display:block; } .menu li li { background:url('images/sub_sep.gif') bottom left no-repeat; display:block; float:none; margin:0px; padding:0px; width:225px; } .menu li:hover li a{ background:none; } .menu li ul a{ display:block; height:35px; font-size:12px; font-style:normal; margin:0px; padding:0px 10px 0px 15px; text-align:left; } .menu li ul a:hover, .menu li ul li:hover a{ background:#2580a2 url('images/hover_sub.gif') center left no-repeat; border:0px; color:#ffffff; text-decoration:none; } .menu p{ clear:left; } #content { vertical-align:top; } #footer { font-size:10px; text-align:center; } #content p { padding:3px; margin:auto 10px; } #content h1 { font-size: 16pt; border-bottom: 1px solid #DDD; margin:10px; color: #000; } a{ text-decoration:none; color:#02AAB0; } a:hover { color:#333; }
-
umm are you saying that it is unnecessary to use the php tags in css file? It actually does not make any difference, the font size/style is still screwed up... Please help.
-
Well it is stored as a variable, not to be output directly to the browser.
-
Well yeah. Like I pointed out before, I use the php include function to import additional css file(the main css is defined through an html page though). In order for the script to work, I have to write in this format:
?> <style type="text/css"> // css content in here </style> <?
So yeah, it is because I cannot write css content directly inside php tag.
About class autoload...
in PHP Coding Help
Posted
umm so theres no way for PHP to check if a class implements an interface, or extends from another class/abstract class?