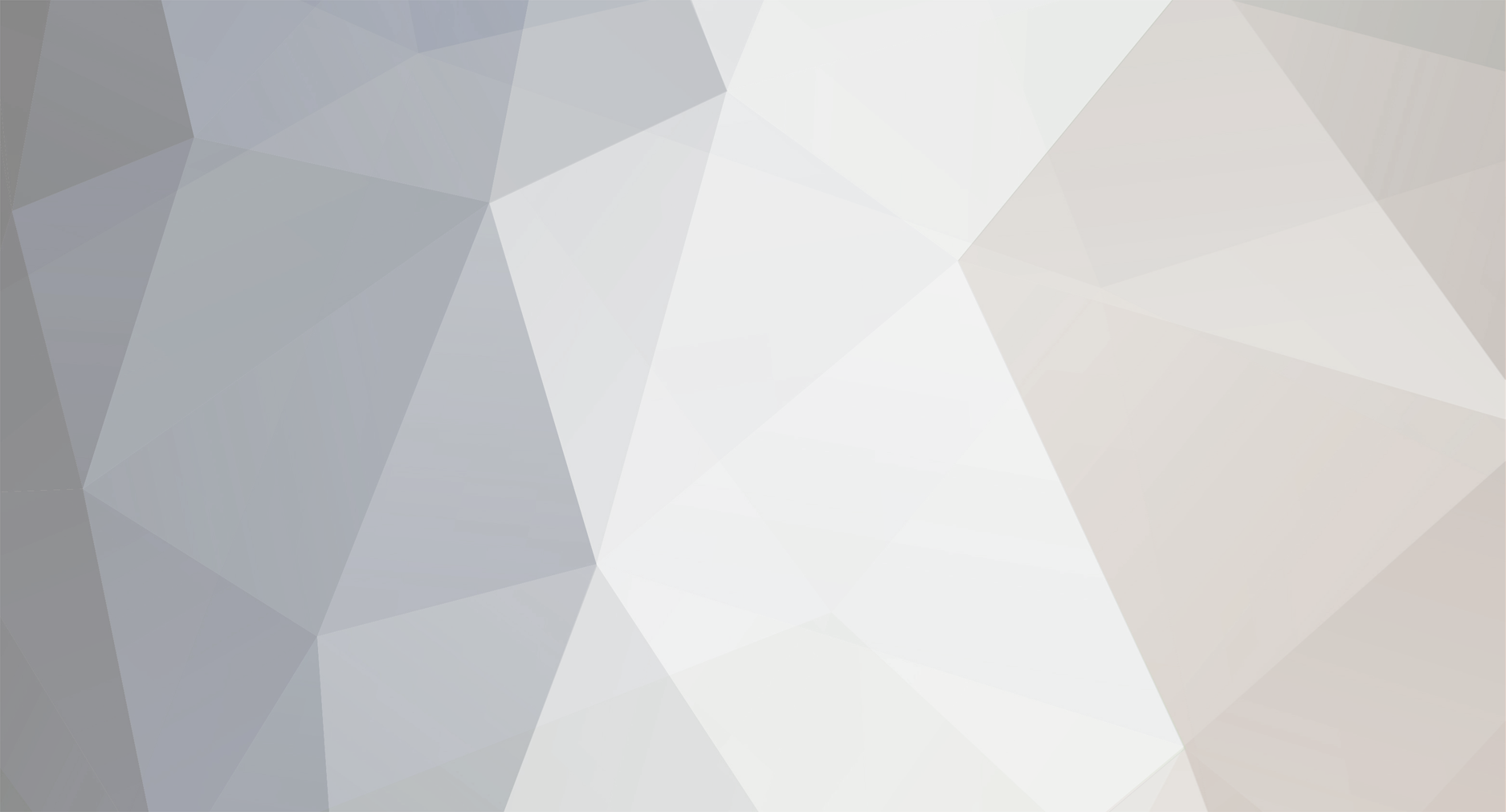
Philip
Staff Alumni-
Posts
4,665 -
Joined
-
Last visited
-
Days Won
20
Everything posted by Philip
-
And I could say I'm a veterinarian because I have a dog.
-
It's almost as bad as the real thing. *Philip runs
-
The following scenarios are what I consider code completion (all of which, I hate): Start typing "$foo" and it finishes it with "bar", resulting in "$foobar" Type a quote or start of like an html attribute and it puts in the closing quote e.g. type "<a href" and it finishes it to "<a href=''>"- this by far is the most annoying one IMO Type "<div>" and it includes a "</div>"
-
I must be the only one that hates code completion.
-
mysql* expects parameter 1 to be resource, boolean given This means your query failed, for some reason or another. Remove any error suppressing (@), make sure you have error displaying enabled, and output mysqli_error to see specific information about your error!
-
I use MySQL workbench, mainly on windows. It's not the best that's for sure. I'm interested in seeing other options as well.
-
PHP kept crashing on preg_match* on large strings, why? This is actually one I kept running into recently... There is a limit on the amount of recursions that PHP will do. You're likely running into this limit and should either up the limit or found a different way to go about your problem.
-
When shouldn't I use regex? It may be cool and hip to be a "regex guru" (it is!). And regex may be powerful and sexy (it is!). But more often than not, you don't really need regex to solve your coding dilemma. In reality, regex is almost always either too powerful or not powerful enough for the job. It has an extremely small set of circumstances where it's the best tool for the job. So, before you try to use regex, ask yourself if you really need it. A good rule of thumb is to assume you don't need regex, and turn to it when you are certain that no other option exists. Too powerful There are many string and substring functions that will handle things much more efficiently than regex. For example: Want to know if the value has only numbers or letters or alphanumeric etc? Use one of the ctype functions Want to know if "foobar" is in "this is the foobar moment in time!" Use strpos or stripos Want to split up a string into an array by a known delimiter, e.g. a comma or pipe? Use explode Want to trim whitespace or other known chars from beginning and/or end? Use trim or ltrim or rtrim Want to replace or strip a known value from a string? Use str_replace Not powerful enough On the other end of that spectrum, regex sometimes isn't powerful enough for the job. The most common example of when regex falls short is that a lot of people turn to regex as a solution for trying to parse HTML/XML content. Sometimes regex may work out for you, depending on what you are trying to accomplish. But most of the time, regex is not what you should be using for HTML/XML manipulation. Regex has come a long way over the years, but at its core, regex is for parsing regular grammar, but html/xml is context-free grammar. If you are looking to parse html/xml content, you should use a DOM parser. There are several libraries that are by default included with php, such as DOM or SimpleXML, as well as a lot of great 3rd party libraries, such as Simple HTML DOM or phpQuery. Also, if you are using a php framework, there's a good chance it already has one or more of these (or its own) included and wrapped in your framework syntax.
-
I need help with some regex, how should I post? Regex can be a tricky subject, especially when you need some help with it. Here is the bare minimum on what you should include with your post: Describe your problem Give lots of sample input data Give the matching expected data for the input data you gave (this is important!) If you already have some code (which you should!), show us the code and tell us what you think is going wrong with it We assume its a PHP regex related as this is PHP Freaks, but you still need to specify (if not obvious) if you are talking about POSIX (ereg) or PCRE (preg) flavor.
-
Should I salt my user's passwords, and why? YES! Salting makes it much harder to crack if an attacker is using a rainbow table.
-
What is the point of MD5 / SHA1 / SHA256 / etc.? You should always, always, be hashing your users passwords. Note the word hashing and not encrypting (difference: hashing is one way and cannot be reversed.) These functions will hash a string with varying levels of security. The more processing power it takes (SHA512 will take more than MD5), the more secure it is likely to be. It should be noted that MD5 really shouldn't be used for password hashing, and instead you should be using the crypt function with a more secure algorthym (like Blowfish).
-
I'm getting a "headers already sent error". What does that mean? When you get this error, it means that you've already started to send content to the user and you're trying to send other header information that should be at the beginning of the request. Common situations include outputting content and trying to redirect the user later, or outputting content and then trying to set cookies. This works in javascript, because it is clientside. This doesn't work with php or other server-side languages, because cookies and redirects are sent in the header of a response, and the header goes at the beginning of the response, before content. It's like trying to dial a number to call your friend when you already called them and are in the middle of talking to them. The only thing that happens is your friend starts wondering why you're pressing random numbers. IOW the browser will not do anything with headers sent after content has already started being sent. So for example, if you try and set a cookie after outputting content, it's not going to get set. So php outputs a warning to inform you of this. So, how do you fix it? There are 2 ways; the first and preferred method, you can reorder your script so that you send the vital information (cookies, header redirects, etc.) before you send any content. The second would be to use output buffering. You can also read more about this in one of older, yet still relevant, resource topic.
-
Call to undefined function "mysql_connect" This means your MySQL PHP library is not loaded. You should check to see if it is loaded by running phpinfo();. If it is not included, you need to check your php.ini configuration or contact your host for more information. It should be noted that mysql_ functions should be replaced with PDO library or the mysqli library.
-
Can you do / write _________ for me? Sure, we could do it for you. But it is going to cost you, and better suited for the freelance forum. Instead, show us some code that you've tried or ask for guidance on what is the best way to go about something. Otherwise, don't expect anything except for snarky replies! Remember, we are volunteering our time to help you. Help us help you by at least attempting to solve the problem on your own!
-
faq README: Everything you'd want to know about PHP Freaks
Philip replied to Philip's topic in PHPFreaks.com Website Feedback
What do those user badges mean? How do I get one?!!? PHP Freaks Staff This is the "Staff" of the PHP Freaks community, the members who actively help out and make decisions around here. There is no formal application process for becoming a Staff member of PHP Freaks. Nominees are picked by current PHP Freak Staff. We actively look for people to join our ranks and we do have some criteria that must be met before considering someone, but in order to keep people from trying to "cheat the system," we refrain from making our methods public. If you wish to be promoted to one of these groups, our best advice to you is to be an active member of the community, be a ninja, help others, etc... and in time you will be noticed. Guru The Gurus are a group of people who have been around for a while, are active, and have shown to be very knowledgeable in one or more areas of interest of PHP Freaks. Gurus take part in making decisions to shape the community, have limited moderator permissions, and have permissions to post blog posts and tutorials on the main site. Moderator In addition to Guru responsibilities and abilities, the moderators can modify, lock, and delete any post or topic. They are responsible for enforcing the rules and may issue warnings or ban any member. Questions regarding a particular moderation action or warning should always be made to whomever did it. If a resolution cannot be found then you may appeal to an admin. The mods have a great deal of influence in the decision making on PHP Freaks. Administrator In addition to Moderator responsibilities and abilities, the admins are responsible for running the server, and they have root access to our servers. They are the highest authority on the forum, and are those who ultimately have the final say in any matter. Questions regarding the operation of the website, questions/help with your account, etc. should be made to an administrator. Other Groups Staff Alumni This group is composed of people who were former staff members of the PHP Freaks community. They no longer possess any authority or special privileges on PHP Freaks. -
faq README: Everything you'd want to know about PHP Freaks
Philip replied to Philip's topic in PHPFreaks.com Website Feedback
Why can't I edit my profile? In order to prevent spam, we've disabled the ability to edit your profile. This includes changing your avatar, your signature, etc. This will be lifted once you hit 10 posts. You should not spam your way to those 10 posts though! If you are in need of editing your profile right away, contact an administrator and we may help you out, depending on how nice you ask. -
faq README: Everything you'd want to know about PHP Freaks
Philip replied to Philip's topic in PHPFreaks.com Website Feedback
I don't like my username, can I change it? We used to not allow username changes, then we started to do them manually. With our switch of forum software we allow you to change your display name on your own. To do so, you need to have 100 posts and can only change your username once per year. You will not be able to change it to a name that is already in use (even the user is not using that account anymore.) No exceptions. Your new name should reflect professionalism and if deemed inappropriate, it will be reverted. You can make the change in the User Control Panel "Display Name" tab. It will show you more information about if you've used your 1 time or not, or if you cannot see that page, you likely do not have enough posts. -
faq README: Everything you'd want to know about PHP Freaks
Philip replied to Philip's topic in PHPFreaks.com Website Feedback
Can you delete my post / thread / account? This post is primarily targeted at people who have posted asking for help as these seem to be the people who most frequently ask these questions. All PMs or emails to staff members regarding deletion requests will henceforth be ignored as they are answered in this thread. Q: Will you delete my post/topic? No. Q: Why won't you delete my post/topic? Imagine if everybody requested their posts to be deleted. If everybody did this there would be no community left, which means you couldn't get the help you required. Secondly, at about 700 posts per day, we would be very busy deleting posts if that was the case. Time is not really an issue, however. There are other reasons why we deny your deletion request. You've come to this forum asking for free help. You don't pay anyone any money, neither PHP Freaks nor the volunteer who is spending his/her spare time on helping you. We think that when you are getting such an awesome service for free, it is only fair that the help you have received is publicly archived for anyone to use. We also think that is a reasonable and very small price to pay. There is also another reason. As already mentioned, people have been helping you for free. By deleting your topic we are also deleting their work. That would be unfair. But I'm a citizen of the EU and GDPR gives me the right to demand you delete my post/topic! Actually it does not. It gives you the right to have your personal information scrubbed from our databases and that does not include the content you have posted. If you so request we will delete your account and anonymize your posts/topics, however their contents will otherwise remain intact. Q: But I didn't even get sufficient help, so why can't you just delete it? We are not interested in forming a committee with the purpose of finding out whether someone received adequate help or not each time someone asks. Q: But what if my client finds it? So what if your client finds out? If the topic reveals that you are incompetent at doing the work your client pays you to do, then you should not have taken the job in the first place. At least be adult enough to admit you have received help instead of relying on lies and deception. Remember that anything you post will be publicly available, so think before you post! Q: But what if my teacher finds it? If your teacher finds it and you were supposed to do it yourself, it's your own fault for cheating. Q: Aren't you guys human? While the real identity of all staff members hasn't been verified, we are pretty certain that all our staff members belong to the homo sapiens species (except for @.josh..whatever (s)he/it is, it's not human...). Really though, trying to shift the blame is pretty pointless. Before you registered, you agreed to our Terms of Service stating that you will grant us an irrevocable license to use content you post using our systems. For added convenience we added a big red notice on all post forms informing you that we will not delete the things you post. You posted knowing these terms. Q: But my post makes me look immature/foolish/stupid/unintelligent. Won't you delete it? "Think before you speak" is a good rule to remember that applies both in real life and on the internet. Q: Won't you make an exception just this time? Sure! Like we'll make an exception for everybody else who asks... No. Q: So what should I do? If you're unwilling to accept that your topics here will be archived publicly, you should hire a consultant to help you in private. This rule blows! Maybe it does, but we did not force you to visit this web site. Basically, think of your posts as emails. You cannot alter emails once they have been sent either. Nor can you take them back (delete them). Think of your post like a speech transmitted live on television. You cannot change what you say there either. Consider what you write before you hit the "post" button. Your post is probably not so important that it cannot wait one minute while you do that. -
faq README: Everything you'd want to know about PHP Freaks
Philip replied to Philip's topic in PHPFreaks.com Website Feedback
What is the purpose of this forum? The purpose of this forum is to provide a means of communication between the staff and community. Please consider the following, before posting: You may post in this forum to.... Ask a question, comment or concern about the main site. Examples are: Requesting a feature for the main site. Reporting a bug on the main site. Requesting an article, tutorial or other main site content. Telling us what an awesome/terrible job we are doing! [*]Ask a question, comment or concern about the forums, IF it has to do with something administrative (NOT IPB code related). Examples are: Requesting an xyz forum. Reordering of forums. Reporting broken/outdated links/stickies. Requesting new stickies. [*]Ask a question, comment or concern about site or forum policy. Examples are: Regarding acceptable content. Interpretation of main site or forum rules. Requesting addition/removal of a rule. If your thread conforms to these things, before posting..... Read the general rules and terms of service. These rules cover general conduct, as well as policies concerning your personal account/content. Read the stickies! There is a very good possibility your question/comment/concern has already been addressed! Look for similar threads! Even if it hasn't made it to sticky status, it may still have been addressed in someone else's post. We don't expect you to scour through pages and pages of posts, looking for something posted who knows how long ago. But at least look on the first page or two... Put some thought into your post! If you want something added or removed, be it feature, content or policy, don't just tell us; tell us why. You may NOT post in this forum to.... Make suggestions about altering the forum's core coding. If there is an IPB mod out there you'd like to see implemented here, you can suggest it. If there is not a mod, we may consider making our own mod, if it sounds cool enough. But note that we are not associated with the IPB development team or community. We simply use their board, just like other people. So if you are looking for a way to have IPB code changed or extended for your own site, you are in the wrong place! Report a forum bug. Note, there is a difference between some setting that could be changed (or is set a certain way because we want it to be), vs. a genuine bug in the forum software. If you are looking for clarification about ability to do something (like edit posts) then refer to stickies/rules, or ask if you do not see your issue addressed there. But for genuine bugs in the forum software...again, we are NOT part of the IPB team/community! Go report it on their site! Make posts that belong in other forums. This includes, but is not limited to, asking for help on your script, where to find xyz script, or arbitrary threads from "So...what's your favorite xyz" to "What do you think about xyz site?" We have a variety of forums that cater to all sorts of things. Use them! If you do not follow these rules.... Your thread may be deleted, closed or moved to its proper location. A warning or other action may be issued to you. Ignorance of the rules is not an excuse! -
This topic is everything you'd want to know about PHP Freaks and some frequently asked questions. Table of Contents - You can find the answers to these questions below in this thread: General Rules and Terms of Service What is the purpose of this forum? Can you delete my post / thread / account? I don't like my username, can I change it? Why can't I edit my profile? What do those user badges mean? How do I get one?!!?
-
This topic is to provide information for those looking for help with regex in PHP. Below are some great resources and FAQs: Table of Contents - You can find the answers to these questions below in this thread: I need help with some regex, how should I post? When shouldn't I use regex? PHP kept crashing on preg_match* on large strings, why? Some Resources Common REGEX patterns Cheat sheet ($2) regular-expressions.info Andy's Regex Tutorial
-
This topic is here for those looking for help on frequently asked questions, or just need some direction in where to find more information. Resources The official PHP website - you can find the manual here PHP Freaks main site - you can find tutorials, articles, and more there Table of Contents for FAQs Can you do / write _________ for me? Call to undefined function "mysql_connect" I'm getting a "headers already sent error". What does that mean? What is the point of MD5 / SHA1 / SHA256 / etc.? Should I salt my user's passwords, and why? mysql* expects parameter 1 to be resource, boolean given
-
This thread should help users with frequently asked questions and links to some resources we've found useful. HTML5, should I use it? In short, YES! HTML5 is great because it keeps the web moving forward with new developments. New attributes & form types are included and are backwards compatible. Other new additions such as new tags (section, article, etc.) are great additions and only require a slight JS & CSS fix to apply to older browsers. A few links that come in handy for HTML5: html5.org, html5.info, and diveintohtml5.info Microdata, what is it? Microdata is great generating rich content markup. It allows crawlers and screen readers to know more about the information being displayed. An example of this would be the "Person" schema, which allows you to define meta-data about a user. This is great for blogs and profiles, and Google will be able to render and display this information in the search results. You can read more from Google or visit schema.org Should my layout be based on tables or CSS? This is an old question that surprisingly still pops up today. Tables are for tabular data, not layouts. You should use CSS for presentation & layouts, and HTML for the structure of the data. (X)HTML, what is it? In short, XML is for structuring data, HTML is for displaying data, thus XHTML allows you to combine both. There are different rules to be applied to XHTML that don't apply to HTML4 documents. You can read a full debate on this on our site, or read more about XHTML on wikipedia.
-
I disagree. Their support is among the best, especially compared to the lowerend boxes (unless you know somebody / are friends with the providers.) They have always been courteous to me, even when I ask a stupid question... and I have done the same thing on lower boxes and gotten rude replies which gave off the vibe "why are you wasting my time?" Plus their uptime is fantastic. I almost always see emails / tweets about how a node on a lower host is going to be rebooted or got DDoS'd so it had to be taken down for a bit, etc. But I've only once had that happen in the 3 years I've had a linode.