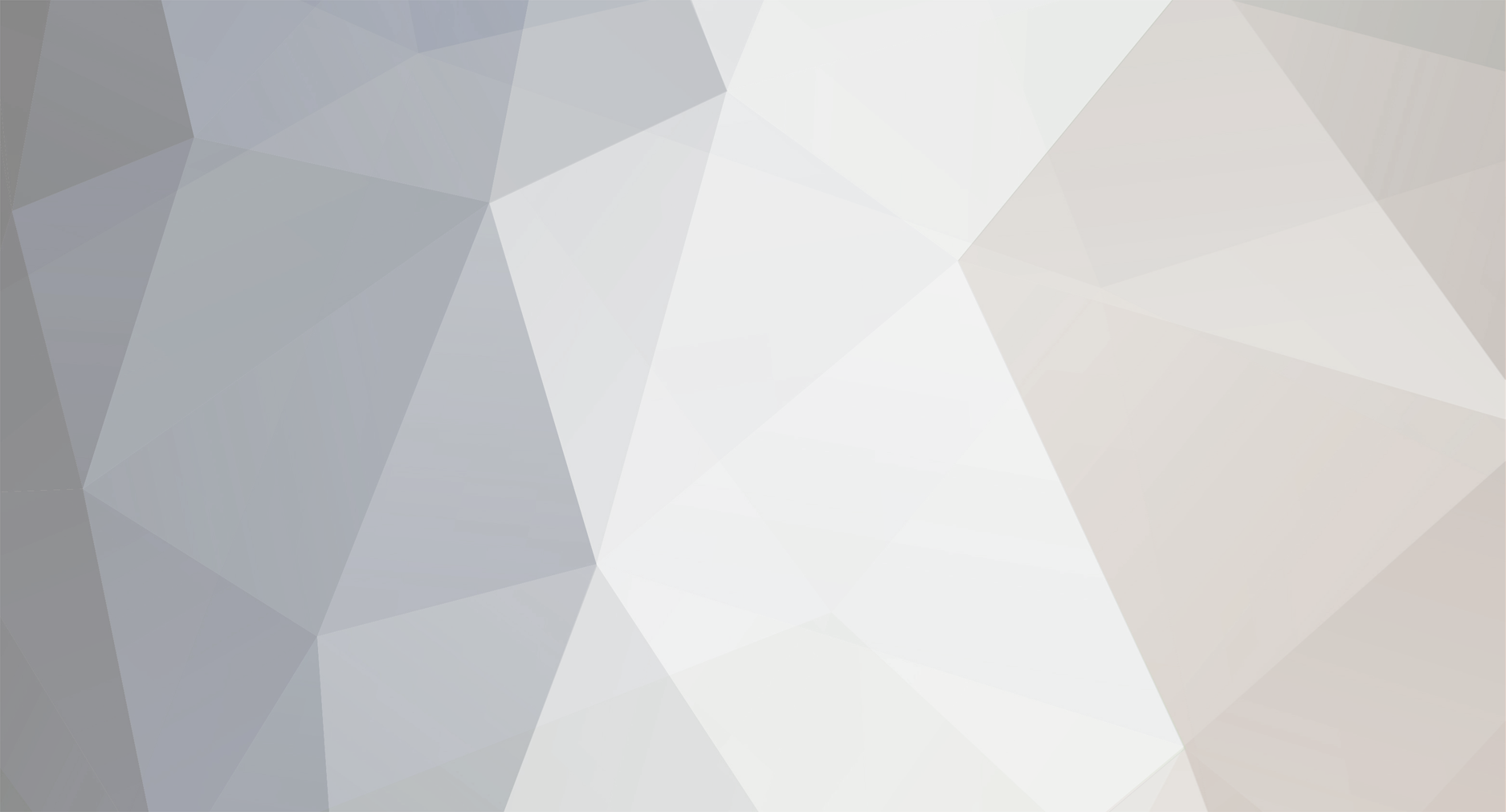
ManiacDan
Staff Alumni-
Posts
2,604 -
Joined
-
Last visited
-
Days Won
10
Everything posted by ManiacDan
-
Writing the web app is going to be your biggest problem. Once you have it written, moving the data isn't all that difficult. You just need to either write a script which outputs your Access data in valid MySQL queries, or use a pre-existing piece of software. This one was the first result in google.
-
9 is IX and 4 is IV, but 99 is not IC, it's XCIX. They favor the X over the L. Similarly, 499 is CDXCIX. The rule is consistent for NINES but not for "one less than the next largest digit." It's difficult to do programmatically. Not impossible, just not fun.
-
Ok, for real, read this: In order to perform an action without refreshing the page, you must use javascript. If said action interacts with the server, you must use AJAX. Go read it again to be sure you understand it. Now that you get that: You can use named anchor tags and hash marks in your URLs so that, when you refresh the page, the page automatically scrolls back down to a specific target. This solves what you're complaining about ("page scrolls up") without forcing you to learn and implement ajax.
-
Roman numeral converters are annoying because the rules aren't set. Though dec2roman is easier than roman2dec.
-
Dude...why do you even know that?
-
We can base64 them all, make it kind of a game. Who has the most posts? TRY TO FIND OUT!
-
According to the Chicago Manual of Style, yes. Lucky for you this script is open source. Get to it.
-
I wrote that years ago, just copied and pasted it here.
-
I dare you: <?php //new and improved recursive version of my last attempt: function dec2english($number) { if ( !is_string($number) && !is_float($number) && !is_int($number) ) { return false; } if ( is_string($number) ) { //we know it's a string. see if there's a negative: if ( substr($number, 0, 1) == '-' ) { $number = substr($number, 1); $number = $number * -1; } } $number = strval($number); if ( $number == '0' ) { return "Zero"; } $negative = $number < 0 ? "Negative" : ""; $number = trim($number, '-'); $split_by_decimal = explode(".", $number); $decimal_string = ''; if ( count($split_by_decimal) > 1 ) { $decimal_string = process_decimal($split_by_decimal[1]); } return trim(preg_replace("#\s+#", " ", $negative . " " . process_number($split_by_decimal[0]) . " " . $decimal_string)); } function process_number($number, $depth = 0) { $group_designators = array( "", "Thousand", "Million", "Billion", "Trillion", "Quadrillion", "Quintillion", "Sextillion", "Septillion", /*that's enough for now*/); $numbers = array( '1'=>"One", '2'=>"Two", '3'=>"Three", '4'=>"Four", '5'=>"Five", '6'=>"Six", '7'=>"Seven", '8'=>"Eight", '9'=>"Nine", '10'=>"Ten", '11'=>"Eleven", '12'=>"Twelve", '13'=>"Thirteen", '14'=>"Fourteen", '15'=>"Fifteen", '16'=>"Sixteen", '17'=>"Seventeen", '18'=>"Eighteen", '19'=>"Nineteen", '20'=>"Twenty", '30'=>"Thirty", '40'=>"Forty", '50'=>"Fifty", '60'=>"Sixty", '70'=>"Seventy", '80'=>"Eighty", '90'=>"Ninety", ); //we already know that we have a numeric string. Process it in groups of three characters while ( strlen($number) > 0 ) { if ( strlen($number) <= 3 ) { $number_to_process = $number; $number = ''; } else { $number_to_process = substr($number, strlen($number) - 3); $number = substr($number, 0, strlen($number) - 3); } if ( strlen($number_to_process) == 3 ) { $output[] = $numbers[substr($number_to_process, 0, 1)]; $output[] = "Hundred"; $number_to_process = substr($number_to_process, 1); } if ( isset($numbers[$number_to_process]) ) { $output[] = $numbers[$number_to_process]; } else { //we're dealing with a number greater than 20 and not divisible by 10: $tens = substr($number_to_process, 0, 1) . "0"; $ones = substr($number_to_process, 1, 1); $output[] = isset($numbers[$tens]) ? $numbers[$tens] : ''; $output[] = $numbers[$ones]; } return process_number($number, $depth+1) . " " . implode(" ", $output) . " " . $group_designators[$depth]; } } function process_decimal($number) { $suffix = array( "Tenths", "Hundreths", "Thousandths", "Ten Thousandths", "Hundred Thousandths", "Millionths", //enough ); return " and " . process_number($number) . $suffix[strlen($number) - 1]; } echo dec2english("-19832498347.34") . "\n"; echo dec2english(14.12) . "\n"; echo dec2english(1432489723485) . "\n"; echo dec2english(10234.45645) . "\n"; echo dec2english(-10.2) . "\n"; echo dec2english("1298721498732.111111") . "\n";
-
You ARE refreshing the site. You're going to a whole new page. That's the problem: it's doing what you tell it to do. If you'd like to perform actions WITHOUT refreshing the page, use javascript.
-
You could run through the array once: $left = array(); $right = array(); $l = true; foreach ( $arr as $val ) { if ( $l ) { $left[] = $val; } else { $right[] = $val; } $l = !$l; }
-
The <a> tag itself probably has an href="#". That will scroll to the top of the page unless the onclick of that <a> tag has return false at the end.
-
Regular expressions or the DOMDocument will do this for you.
-
How to debug this code: 1) Find line 11 2) What's wrong with it? hint: $enteredpass = md5( $_POST[ 'password' ] ;
-
yes, obviously XSLT was used to transform into HTML, that's how web pages are made. We used XML so that we could build pages using SimpleXML and so all our documents would be well-formed. XHTML would have been just as good, really, but there were other side benefits, like firefox opening up our source documents as traversable trees instead of pages.
-
Why are you stopping me from using ~, ^, and * in my passwords? What is with people and their ridiculous password limitations recently? Just make sure it's 6 characters or more, and be done with it.
-
I believe you mean 567.890 and not 234.567.890
-
Eh, looks ok to me. Once you start using thousands separators you run into internationalization issues and other stuff.
-
ON DUPLICATE KEY solves the question you seem to be asking in the thread TITLE, but has nothing to do with the thread's actual CONTENTS. A trigger is the proper solution to this.
-
Php On A Seperate Business Logic Server From Web Serve...how?
ManiacDan replied to bluesclues9's topic in Apache HTTP Server
Did you not read this entire thread? -
You're distributing your source code to multiple people? You cannot secure it, you're sending them your source code. There are applications that obfuscate PHP code, but they're not foolproof, and they're expensive. Look into a product like Ioncube if you really must distribute your source code.
-
So when you echoed the content and it was correct...how did that happen? Curl can be set to follow redirects with the same setopt you're already using.
-
strpos is case sensitive, is the target string /Images/?
-
If you're building a json string (for javascript) then you're doing it the long, complicated, and incorrect way. Christian showed you json_encode, you'll probably want to switch to that at some point.
-
Sure...Don't show it to anyone. You really need to be more specific here.