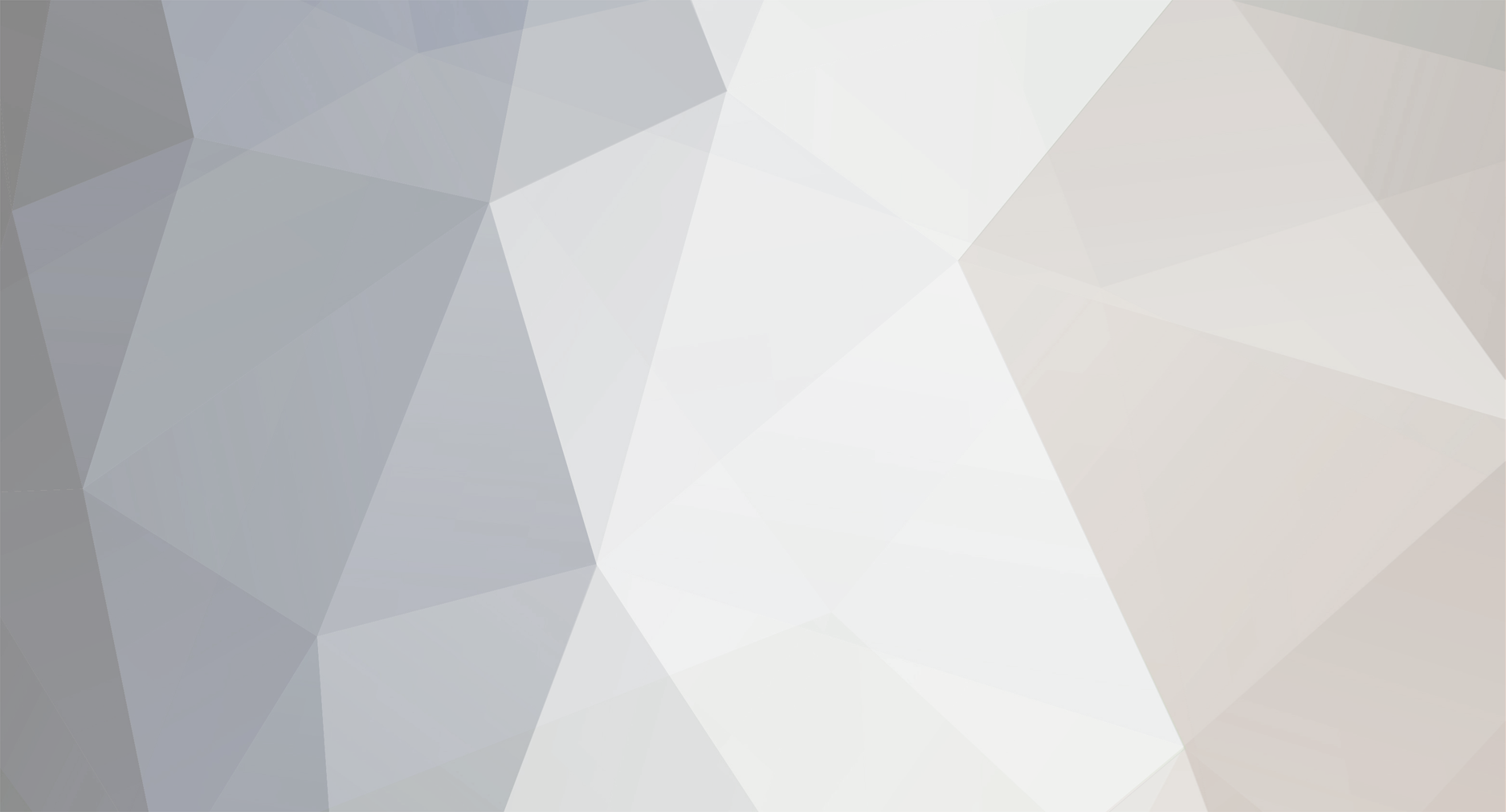
boompa
Members-
Posts
305 -
Joined
-
Last visited
-
Days Won
1
Everything posted by boompa
-
Is there any particular reason you're not using a database for this?
-
In other words, create an empty array to hold errors $errors = array(); your failed validations should add to this array like this $errors[] = 'This is an error'; and you check for errors with if (!empty($errors)) {
-
If you don't see how the array is empty, then start debugging by printing stuff out! <?php $cart_check = '123+0,145+1,134+2,'; $cart_check = trim($cart_check, ','); if ($cart_check == "") { print("Empty cart_check\n"); $cart_check_row_id = ''; } else { $cart_check = explode(',', $cart_check); print_r($cart_check); $key = array_search(158, $cart_check); print("Key: $key\n"); if ($key != "") { $cart_check_row_id = explode("+", $cart_check[$key]); print("Non-empty key -- cart_check_row_id: " . print_r($cart_check_row_id, true)); } else { $cart_check_row_id = array( "0" => 0, "1" => 0 ); print("Empty key -- cart_check_row_id: " . print_r($cart_check_row_id, true)); } $cart_check_row_id = $cart_check_row_id[1]; } print("Final cart_check_row_id: $cart_check_row_id\n"); php -f cartcheck.php Array ( [0] => 123+0 [1] => 145+1 [2] => 134+2 ) Key: Empty key -- cart_check_row_id: Array ( [0] => 0 [1] => 0 ) Final cart_check_row_id: 0
-
What is the content of $_POST['upload'][0]?
-
$image_upload1 = $_POST['upload'[0]; Do you see a problem there? Or is this not your actual code, and you added a typo? One of the first things to learn is how to debug your code. You can do this here by printing the value of the $_POST array with var_dump($_POST); Does it display what you expect?
-
Help to create a HTML email from a script I have
boompa replied to Dicko_md's topic in PHP Coding Help
Are you using a library like SwiftMailer or PHPMailer to send the mail? If not I'd try one of those first. -
What a horrible idea this is! Letting someone execute arbitrary SQL code against your database? Good gravy!
-
No one is stopping you from using the information that has been provided and massaging it into the format you desire. If your XML file looked like this, your code would work. <?xml version="1.0" encoding="utf-8"?> <reports> <entry> <fname>First</fname> <lname>Last</lname> <location>Mesquite</location> <report>power</report> <description>Storm Report Power Outage</description> </entry> <entry> <fname>First Two</fname> <lname>Last Two</lname> <location>Mesquite</location> <report>lightning strike</report> <description>Lightning strike reported</description> </entry> </reports> $data = "http://www.mesquiteweather.net/xml/mesquite.xml"; $xml = simplexml_load_file($data); foreach($xml->entry as $entry) { $fname = $entry->fname; $lname = $entry->lname; $location = $entry->location; $report = $entry->report; $description = $entry->description; print ("First name: $fname\n"); print ("Last name: $lname\n"); print ("Location: $location\n"); print ("Report: $report\n"); print ("Description: $description\n"); print ("\n"); } Result: $ php -f weather.php First name: First Last name: Last Location: Mesquite Report: power Description: Storm Report Power Outage First name: First Two Last name: Last Two Location: Mesquite Report: lightning strike Description: Lightning strike reported
-
Actually, it *is* perfectly functional, if you print the data. <?php $data = "http://www.mesquiteweather.net/xml/mesquite.xml"; $xml = simplexml_load_file($data); $fname = $xml->fname; $lname = $xml->lname; $location = $xml->location; $report = $xml->report; $description = $xml->description; print ("First name: $fname\n"); print ("Last name: $lname\n"); print ("Location: $location\n"); print ("Report: $report\n"); print ("Description: $description\n"); ?> Result: $ php -f weather.php First name: First Last name: Last Location: Mesquite Report: power Description: Storm Report Power Outage Your code assumes there's a wrapper around a bunch of entry elements, and there is not.
-
insert doesn't work - help please! ...and thank you.
boompa replied to perplexia's topic in PHP Coding Help
Sort of an aside, having not using MS Access for the last 15+ years and never from PHP. I'm a bit perplexed as to why you'd use mysql_real_escape_string on values being passed to an Access database? Does that work? -
Submit to tables based on Criteria, & Cleaner Code.
boompa replied to SalientAnimal's topic in PHP Coding Help
So, did you really mean for all those checks to be bitwise operators as opposed to logical ones? -
Go back to the manual page and re-read the "Note" under the "Description" section.
-
Globals are bad, and you don't show us where you're creating the $mysqli global object you're attempting to use.
-
They're not encrypted, they're hashed. Encryption is a two-way process, hashing is one-way.
-
Consult a lawyer.
-
What exactly is involved with using Authorize.net?
boompa replied to Zane's topic in Application Design
Is this what you want? -
Check your single quotes and concatenation on this line: echo '<a class=\"fancybox fancybox.iframe\" href=".$result->url.?venue_name=$id."</a>';
-
It's got nothing to do with the extension. Show the code you're attempting to use. Example code: ?php $fp = fopen("http://www.national-lottery.co.uk/player/lotto/results/downloadResultsCSV.ftl", "r"); if (!$fp) { die("Failed to open URL"); } else { while(($data = fgetcsv($fp)) !== false) print_r($data); } ?>
-
I'd start by making sure that $this->Item->selectAll() is returning rows by adding a log statement and checking the logs after the call. This: $this->set('todo',$this->Item->query('insert into items (item_name) values (\''.mysql_real_escape_string($todo).'\')')); and this: $this->set('todo',$this->Item->query('delete from items where id = \''.mysql_real_escape_string($id).'\'')); are definitely very wrong. You don't really want to set the view variable to the results of an INSERT or a DELETE query do you?. And I'm quite sure you're doing it wrong by using query() instead of the model's create()/save() and delete() methods.
-
When I search Google for "ebay api", this page is the first one in the list. That pages links to the API documentation, which is where you need to start your research.
-
What about something like what you see here in the docs? A try/catch mechanism?
-
Unless you've done something on the service side, the answer would appear to be btherl's here: Running your test script from the command line gives me stdClass Object ( [TestStringResult] => <information><MainA>ABCDE</MainA><MainB>123456789</MainB><SetA><ValuesA><TextLine1>Test Text 1</TextLine1><TextLine2>Test Text 1</TextLine2></ValuesA></SetA><SetB><ValuesB><NumberLine1>123456</NumberLine1><NumberLine2>123456</NumberLine2></ValuesB></SetB></information> )
-
Are you looking for something like $organogram_is_set = isset($_POST['organogram']) && !empty($_POST['organogram']); or are you saying you're trying to check if there's an email address in the organogram field? It's early, I'm a bit easy to confuse after only one cup of coffee.
-
Can't increment/decrement overloaded objects nor string offsets
boompa replied to Phalanxer's topic in PHP Coding Help
In order to use an increment/decrement, the variable being incremented or decremented must exist and have a value. What if you do if ($_POST['num'] == '3') { print_r($_SESSION['tablea']); $_SESSION['tablea']['number1']++; } Does that demonstrate that the key number1 exists as a member of $_SESSION['tablea']? Is $_SESSION['tablea'] an array?