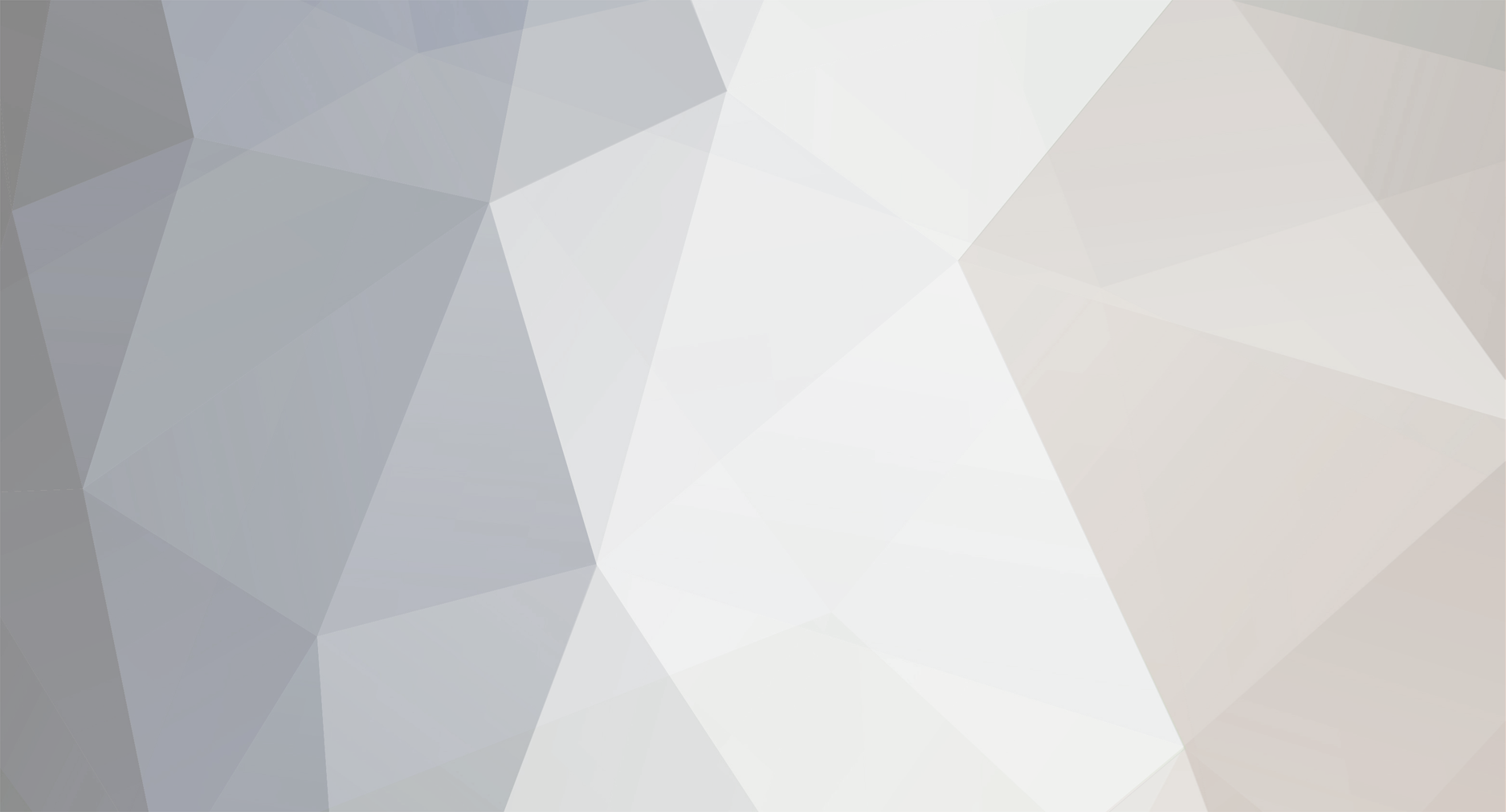
AyKay47
-
Posts
3,281 -
Joined
-
Last visited
-
Days Won
1
Posts posted by AyKay47
-
-
this should be done in the query itself for optimization, you should either use my suggestion or format the date correctly like PFM suggested.
-
Thanks very much guys. In the end, I did not even have to use the JOIN function of MySQL. I simply combined a WHERE and IS NOT NULL with RAND() and LIMIT. I'm going to have to look into this join stuff, though, because it seems like a very popular function.
There are two ways to grab values from multiple tables; a subquery or a join.
Can you post the final solution query please.
-
-
This is how yours should look:
select t1.user_email, t2.img_thumb from table_1 as t1 left join table_2 as t2 on t1.user_id = t2.img_id_fk having t2.img_thumb is not null order by rand() limit 5
Yours does NOT check NULLs after the result set is built, if a member doesn't have a row in table 2 you will get a NULL in your result set when using a left join and no HAVING.
My method will automatically ignore the NULLs, so I don't need to check for a null during a search and after the search.
Again, the HAVING clause is used in conjunction with a group by clause, it does not replace the where clause, quite simply, the query you just wrote is wrong.
I'm not here to argue, OP this is the query you should use for this, provided by Little Guy
select t1.*, t2.img_thumb from table1 t1 inner join table2 t2 on (t1.user_id = t2.img_id_fk) order by rand() limit 5;
-
This doesn't let me search for a specific word/value
sure it does:
$array = array('blue', 'yellow', 'red', 'blue', 'blue', blue', yellow'); $count_arr = array_count_values($array); $blue_count = $count_arr['blue']; //prints 4
-
But you need to check if they are null after you join.
right, your suggestion of using an inner join instead is the correct solution.
-
-
I would do this in the query:
select day(date_field) as day, monthname(date_field) as month, year(date_field) as year from table
then using php you could do something like this.
$query = mysql_query("select day(date_field) as day, monthname(date_field) as month, year(date_field) as year from table"); while($row = mysql_fetch_assoc($query)) { $day = $row['day']; $month = $row['month']; $year = $row['year']; }
*pseudo code*
-
<a href="delete.php?username=<?php echo $username; ?>">Delete User</a> Delete.php: <?php mysql_connect ("localhost","root","") or die("Cannot connect to Database"); mysql_select_db ("test"); $username = $_GET['username']; $username = mysql_real_escape_string($username); mysql_query ("DELETE FROM members WHERE username='$username'") or die ("Error- user has not been deleted"); echo "User has been deleted"; //header("Location: personaldetails.php"); ?>
-
I forgot the order by rand(),
the is not null would also be in the having, not the where.the having clause is used in conjunction with the group by clause, you can use IS NULL and IS NOT NULL in the where clause.
-
Is $username defined elsewhere?
the query syntax is fine, my guess is that $username is not defined.
what is the error?
Also, it's mysql_error(), not mysql_error
-
if (isset($_POST['Submit'])) { $username=mysql_real_escape_string(trim($_POST['username'])); //this needs to match the nameattr of the input field $sql = "select * from memberdetails WHERE username='$username'"; $result = mysql_query ($sql);
*snippet*
-
post the entire updated code, using code or php tags
-
you are using the same name attribute for the submit button and the text field.
Change the text field name to something that makes sense, like "username"
-
you are trying to compare a resource to an integer, the code should read:
$sqlid = "select * from temp where Email='".$a[8]."'"; $idresult = mysql_query($sqlid) or die(mysql_error()); $res = mysql_fetch_assoc($idresult); if (mysql_num_rows($idresult) > 0) { $tempid = $res['ID']; die('sql id:' . $tempid . 'or' . $res['ID']. 'or ' . $sqlid ); }
also, don't use die()'s to display messages, they are very user unfriendly.
-
select t1.user_email, t2.img_thumb from table_1 as t1 left join table_2 as t2 on t1.user_id = t2.img_id_fk where t2.img_thumb is not null
Typically you want to name fields so they make sense with what they hold and with relationships, a user id is not an image id..
-
Typically you'll return false on the submit button click and call .submit() on the form element in your function.
Really can't help any further given a picture and a form..
-
Marked as null. In the MySQL DB, there's a checkmark to indicate that it is null.
Oh, right, how are these values initially being inserted?
-
about the keyword global: i've seen more people saying it's considered a bad practice. Why exactly?
It completely breaks the encapsulation of a function etc by introducing variables from the global scope..
Wordpress is poorly coded..
-
Question: What exactly is the difference between a blank field and one that is checkmarked as NULL? Is there even anything that I need to worry about, or is it pretty much the same thing?
check marked as null?
But it also needs to be put back to NULL once a value is already in there if the user selects it. A default value in MySQL won't do that if he's doing an update. Will it?I'm trying to wrap my head around the logic here, but I haven't been given enough information, so it's hard to tell.
-
I would simply set the arg $value to null as default:
setlevel($world,$coords,$var,$value = null)
Then, if $b == 0, do not pass a value for that arg:
if($b == 0) setlevel($p,$co,"board");
which will keep $value as NULL in your function.
Depending on your logic for this, the best option would be to change the mysql field definition to default NULL, changing the value when yes is selected.
-
we can't help you with this without seeing all of the relevant code.
-
I would use an input array for this, set the input name to an array format:
<input name="quantity[]" type="text" value="" size="6" maxlength="2" />
Then iterate the field values, adding the value to the previous value each iteration:
$total_quantity = 0; foreach($_POST['quantity'] as $value) { $value = intval($value); //some sanitization to make sure the value is an integer $total_quantity += $value; }
This eliminates the need for a regex and multiple loops.
-
Are you using a date/datetime field to store the date in your db table?
Javascript onclick function?
in Javascript Help
Posted
target='_blank'?