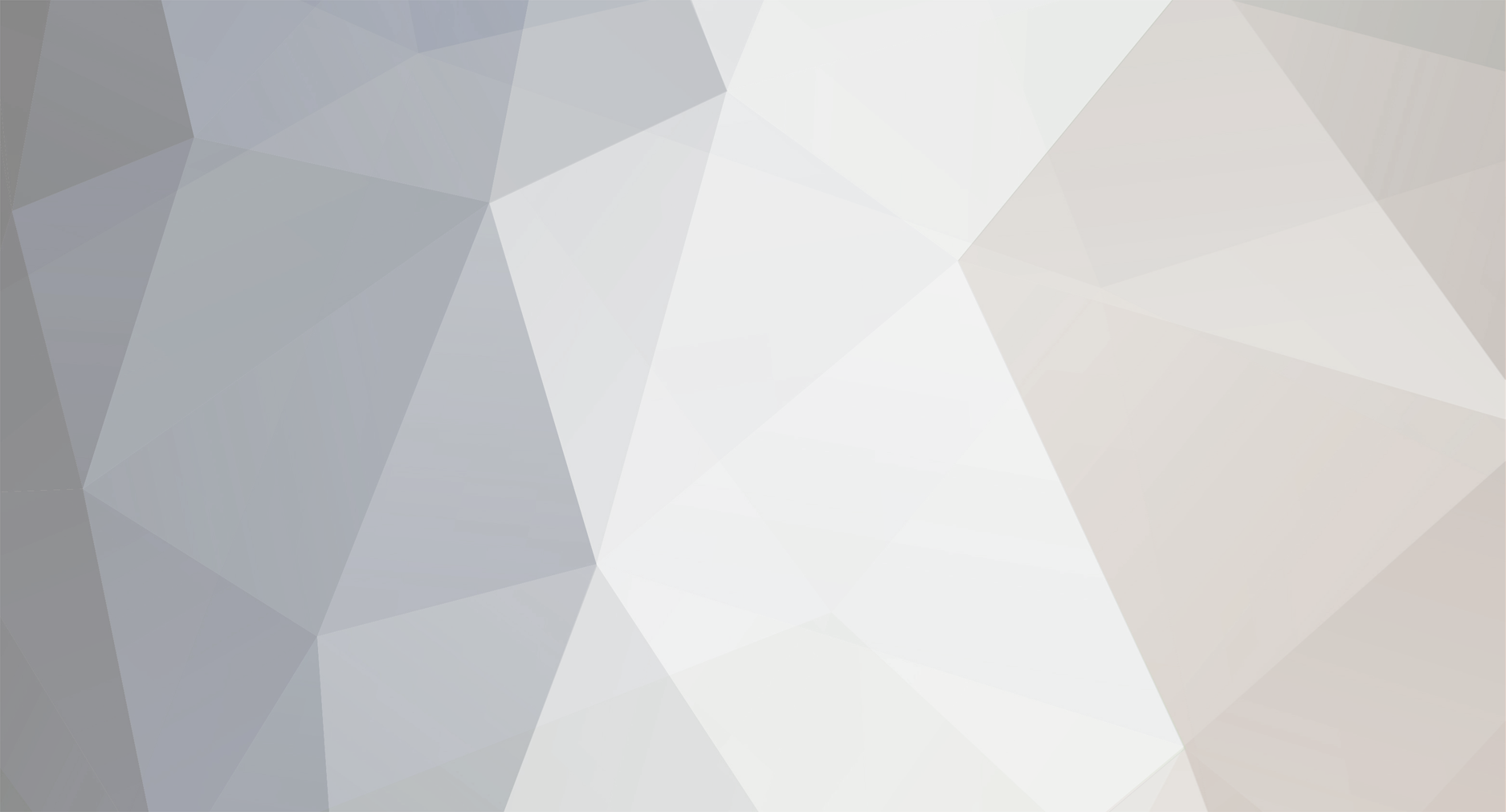
AyKay47
-
Posts
3,281 -
Joined
-
Last visited
-
Days Won
1
Posts posted by AyKay47
-
-
Something that I like to do is wrap array indices in curly braces when using double quotes.
echo "<option value=\"{$row['state']}\">{$row['state']}</option>";
I'm too lazy to concatenate all of the time, improves readability though.
-
So the form is sending the data to the executing page?
If so, simply action='' is fine in the form.
You'll want something like this..
if(isset($_POST['submit'])) //make sure submit button has been clicked { $gender = $_POST['gender']; //radio button value $first_age = $_POST['first_age']; //dd value $second_age = $_POST['second_age']; //dd value $sql = "select fields from table where something = '$gender' and something_else = '$first_age' and something_else = '$second_age'"; //etc... }
keep in mind that this is pseudo code providing a very basic example without the proper error checks etc..
-
By grabbing the values the form sends to "selectdata.php" via $_POST.
Where is the code that handles this form?
-
Also on that note, AyKay47, would you mind telling me the ideal alternative to passing the errors through the session? They have to be carried from one PHP file to another; would the alternative be logging IP session keys with error messages in the database then simply removing them once they are displayed?
Typically errors are triggered within the executing script, so they don't need to be saved.
However, if this is necessary, use $_SESSION's
-
then $_SESSION['GORB']['message'] has been defined as a string, not an array.
You can't "push" values onto a string.
Posting the relevant code would help.
Also, I wouldn't use the $_SESSION superglobal array to store simple errors, complete waste of resources.
-
There was no reason to change $path from the original post.
-
If the SQL is a static string that has absolutely no user data effecting it at all, then SQL injection is not possible.
Say that you are building a query to use in PDO, but you are using a variable to determine which table to select from, and that variable comes from user data. This means that if you do not escape this user data, then the SQL query can be polluted and SQL injection is still possible even though you are using PDO.
If the SQL query is clean, then you are good to go.
-
Without seeing the environment that this code is in, it will be hard to help.
However, if you can expand on what exactly "doesn't work", maybe we can help further.
-
Since you haven't really provided everything that I need to answer this accurately, I will assume that the `dateposted` field is type DATE.
SELECT newsID, headline, dateposted, image, article FROM news WHERE dateposted BETWEEN '2012-02-01' AND '2012-02-29' ORDER BY newsID DESC
-
The dropdown appears to be working as you have coded it in jsfiddle, unless this is not the functionality that you are looking for?
-
Sorry to jump in on this one. But on the subject of PDO - is it right that it automatically escapes all your data so you don't need to mysql_real_escape_string ?
To expand this a little further, the basic logic is to escape all user data before inserting into a db using your RDBMS escape string function, and to use htmlentities() upon grabbing data from a db. This converts things like quotes etc into their HTML entities before being executed. IMO, the safest way to work with databases is to use PDO, which separates the SQL and the user data.
no, it executes the SQL and the PHP data separately, so escaping isn't an issue.
If you need a further explanation, read here
-
To expand this a little further, the basic logic is to escape all user data before inserting into a db using your RDBMS escape string function, and to use htmlentities() upon grabbing data from a db. This converts things like quotes etc into their HTML entities before being executed. IMO, the safest way to work with databases is to use PDO, which separates the SQL and the user data.
-
Well I wish I could help further, but Im having trouble wrapping my head around what it is exactly that you are trying to do.
The code I provided sets var dataOut to the Ajax response string. You can do whatever you want with this string.
So I'm not really sure why you are having further issues.
-
Well, I know that the code I posted wont trigger a 500 error by itself, because I have used this exact code on a live server.
Something else is causing the error.
Just add the "no indexing" code to the .htaccess file.
-
its called the ternary operator
In your example, if $_GET['a'] is set, then $controllerAction will be set to the value of $_GET['a'], else it will be set to "portal/home".
-
It used to default to the HTML view, then was changed to the Source view. Now with the new design it is back to the HTML view.
I have always just forced a plain text output from within the code, with things like header("Content-Type: text/plain") or ini_set("default_mimetype", "text/plain").
yeah, I have started to do the same now. thanks Sal
-
it does this because in the pattern it is looking for a forward slash, which will only occur in pages inside sub-folders.
RewriteEngine On RewriteBase / RewriteCond %{REQUEST_FILENAME} !-d RewriteCond %{REQUEST_FILENAME} \.php -f RewriteRule ^(.*)$ $1.php
-
select columns from table where date <= DATE_ADD( NOW(), INTERVAL 7 DAY )
this is assuming that you do not have dates in the past.
-
well, the code I posted is the "proper" way to handle an ajax request.
var dataOut is set to the ajax response string, so you can use that variable in whatever way you want, be it function calls etc.
Which really brings us full circle, you can do something like what you were in the OP, minus the success: param, which doesn't exist inside of a normal function.
-
what does writeOut do?
this is what I'm thinking, you can tinker with it to suit your code:
var xmlHttp; try { // Firefox, Opera 8.0+, Safari xmlHttp=new XMLHttpRequest(); } catch (e) { // Internet Explorer try { xmlHttp=new ActiveXObject("Msxml2.XMLHTTP"); } catch (e) { try { xmlHttp=new ActiveXObject("Microsoft.XMLHTTP"); } catch (e) { alert("Your browser does not support AJAX!"); } } } xmlHttp.onreadystatechange = function(){ if(xmlHttp.readyState == 4){ dataOut = xmlHttp.responseText; var ajaxDisplay = document.getElementById("dispDiv"); ajaxDisplay.innerHTML = dataOut; } }; xmlHttp.open("GET", "data.php", true); xmlHttp.send(null);
Of course, I always recommend you use jquery's AJAX API..
-
wait, you are using the success: parameter as if this is jquery Ajax.. it's not..
-
dateOut = xmlHttp.responseText; success: function writeOut(dataOut){
simple variable naming mistake.
dateOut != dataOut
-
1. Var name is not defined in the functions scope and therefore cannot be used.
2. The code you posted has nothing to do with checkboxes.
-
That explanation makes no sense to me at all.
Maybe the relevant code will.
PHP form no longer working
in PHP Coding Help
Posted
then the form that this page is getting it's data from is not sending the data correctly anymore.
something has changed, anything that you know of?