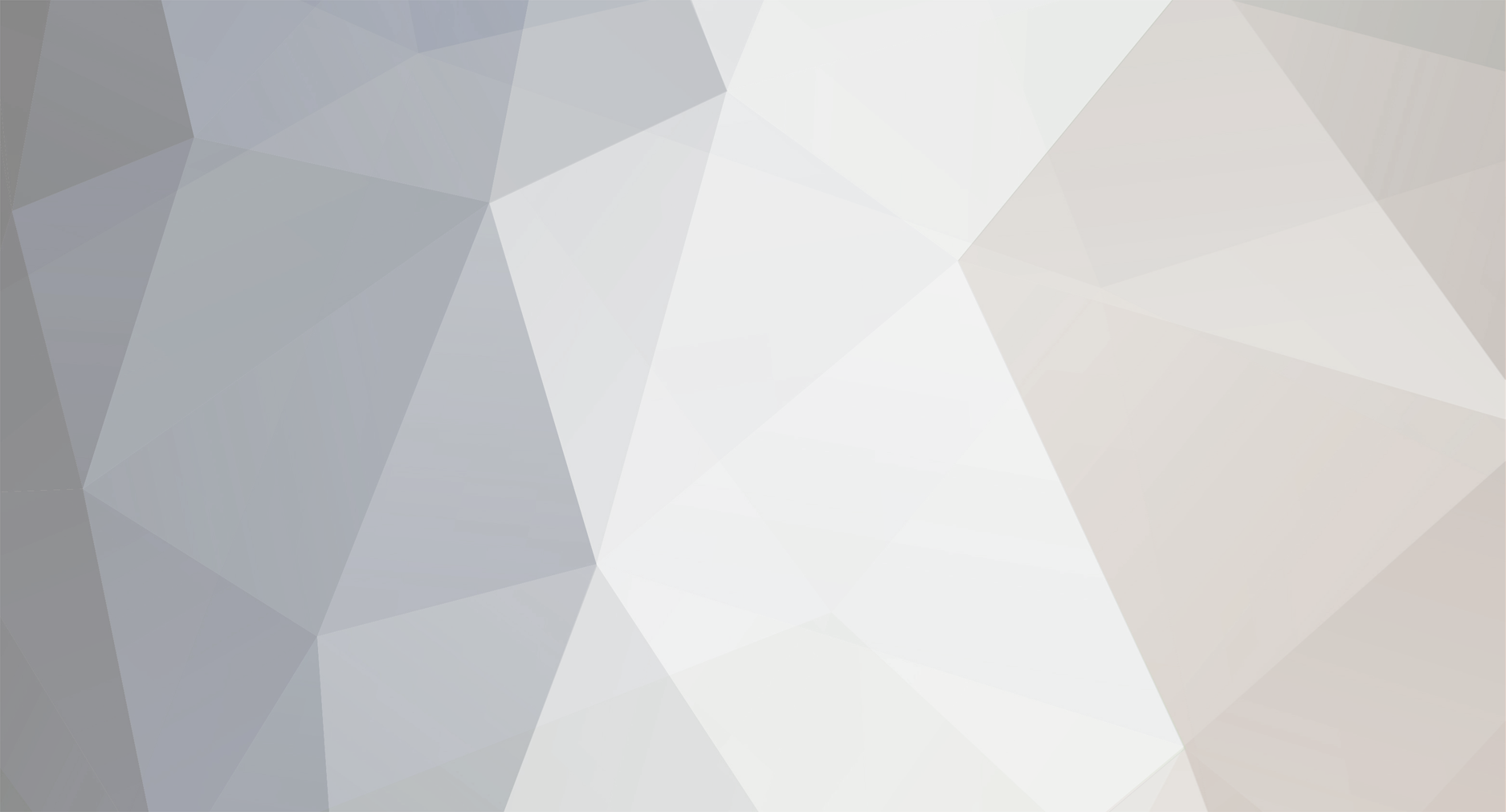
AyKay47
-
Posts
3,281 -
Joined
-
Last visited
-
Days Won
1
Posts posted by AyKay47
-
-
using the code from reply #2 that you posted, I have adding overflow: hidden to .container so it will encase floating items (bit of a hack). This is similar to using clear: both but I prefer this method.
So you will have:
.container /*ONLY MAIN BOX that holds contentBox(that holds content, sidebar) and needs margin-left and margin-right set to auto in order to be positioned in center of page*/ { width:1180px;/*1180px;*/ margin-left:auto; margin-right:auto; margin-top:10px;/*CSS Reminder:the main div 'container' is inside body so it will move 10px from the top of whatever box precedes it (here it's header div)*/ padding:10px 10px 900px 10px; background-color:grey; overflow: hidden; }
Keep in mind that this will only work if you have specified a width for the div, and overflow can be set to either auto or hidden.
-
Rolling Rock or Blue Moon.
Growing up in Pennsylvania, neither Rolling Rock or Yuengling are particularly appealing to me. I vary from Asahi Super dry, to Guiness Stout, to Sam Adams Lager, to Fat Tire.
I have lived in PA my entire life. Yuengling seems to be very popular where I'm at in PA (south east).
Sam Adams is another popular one around here. Particularly the Octoberfest (my personal favorite).
-
$pattern = '~<td class="word fixed_text">([^<]*)</td>~i';
-
something was happening, but the page was refreshing because the form was being submitted.
To stop this, you can return false on the submit event.
<form id='some_form'><input id='some_field' type='text' /><input type='submit' /></form>
javascript:
$("#some_form").submit(function(e) { var input_val = $("#some_field").val(); //ajax request $.ajax({ url: "some_file.php", data: "field_val=" + input_val, success: function(d) { alert(d); } }); return false; //stops the forms default behavior });
or you can do as you have done, and get rid of the form altogether.
Although typically you should have a back end form handler as well.
-
You'll need to tell me more than "it doesn't work".
What exactly happens?
If you received the correct value when you added alert(tags), then the event is working just fine, and the problem lies either in the ajax call itself, or the handling php page.
-
if $_POST['m'] contains an array of integers, the only sanitation you need to do is to intval the values to ensure they are integers. If you do that, there is no need to escape them.
-
Well, I'm going to give the same answer. Ajax is what you are looking for.
I recommend you look at the Jquery AJAX API
-
Oops, meant name. thanks for noticing that.
-
parse_url doesn't allow or disallow anything. It simply allows you to do it more easily by breaking the url into separate components.
says it right in the descrip, my mistake.
I have not fiddled around with the components argument, so I am not exactly sure what it can return.
What would the advantage be of using parse_url() over a regex in this case? Seems like using parse_url() would require much more code.
-
Thanks, works fine when I pass the variables to the function...
I was convinced variables declared outside the function would have a "global" scope !?#
Apparently not...
No they do not, and do not get into the habit of using the global keyword.
Using it should always be avoided.
-
AyKay, he wants to find URLs, not email addresses.
Monkuar, please try this code:
<?php $text = 'abc example.com2 abc'; if (preg_match('/(?<=[a-z0-9])\.(com|org|net|mil|edu|de|us|uk|au|info)/i', $text)) { echo "You cannot post links or urls unless you have made 10 Posts"; } ?>
I was a bit off key with this one, disregard my original post.
Looking at the solutions here, parse_url() will still allow invalid url's through like test@test.com2
If you want the filter to be very tight, which it appears that you do, something along the lines of one of the regex patterns provided is what you want.
-
this is an issue dealing with variable scope
You will either need to introduce the variables inside of the functions local scope, or pass them in as function parameters.
-
fopen does not return true, ever. It returns either a file handler resource or false. According to my chart here, objects of type resource will never be equal to an integer (which is what you're using instead of true/false).
I almost have that chart memorized now Dan..heh
But Dan is absolutely right, and I overlooked it in my OP.
When using a function that returns a resource or false upon failure, I would use negation to check the return value against the boolean FALSE return.
if(fopen("file.txt","r") !== false)
or check for it returning false in an if else statement.
if(fopen("file.txt","r") === false) { //returned false } else { //returned resource }
Anyway, using file_exists is what I would go with.
Bookmark Dan's chart, it will come in handy in the future.
-
If these are two submit buttons, you can check for the value being set to determine which button was clicked and act accordingly.
<form> <input type='submit' value='yes' /> <input type='submit' value='no' /> </form>
receiving page
if(isset($_POST['yes'])) { //do stuff } else if(isset($_POST['no'])) { //do stuff }
-
That will work, something else must be going on if that is not working.
Please post the relevant code.
-
I am going to write it a different way since this is what I am use to.
$('.sub').click(function() { var tags = $('.add').val(); $.ajax({ type: 'POST', url: "public/ajax/upload_tags.php", data: "tags_to_add=" + tags, success: function(data) { alert(data); } }); });
then in the public/ajax/upload_tags.php page
if(isset($_POST['tags_to_add']) && !empty($_POST['tags_to_add'])) { echo $_POST['tags_to_add']; }
If this does not return anything, check to make sure that the path of the receiving file is correct.
-
1. $_POST['tags_to_add']!="" and !empty($_POST['tags_to_add'] are redundant, they do the same thing.
2. Since you are not receiving any data from the ajax call, the if condition is not being met, so tags_to_add is not getting passed correctly.
The first step is to add debugging to the ajax call to make sure that the value is getting passed to the php script correctly.
$('.sub').click(function() { var tags = $('.add').val(); alert(tags); //$.post('public/ajax/upload_tags.php', {tags_to_add: tags}, function(data) { // alert(data); }); });
-
you are not accounting for the spaces/newline after the closing <dt> tag and before the opening <dd> tag.
$html = "<dt>Today</dt> <dd>Freezing rain mixed with ice pellets changing to rain and ending near noon then cloudy. Wind becoming northeast 30 km/h gusting to 50 this morning then southwest 50 gusting to 70 near noon. High plus 4.</dd>"; preg_match_all("/<dt>Today<\/dt>\s*<dd>([^<]+)<\/dd>/i", $html, $forecast, PREG_SET_ORDER); echo "<pre>"; print_r($forecast); echo "</pre>";
results:
Array ( [0] => Array ( [0] => Today Freezing rain mixed with ice pellets changing to rain and ending near noon then cloudy. Wind becoming northeast 30 km/h gusting to 50 this morning then southwest 50 gusting to 70 near noon. High plus 4. [1] => Freezing rain mixed with ice pellets changing to rain and ending near noon then cloudy. Wind becoming northeast 30 km/h gusting to 50 this morning then southwest 50 gusting to 70 near noon. High plus 4. ) )
-
no where in this code does would it return anything, what are you expecting this to do?
If you want to return the data from the $.post call, you will add something that actually returns the data, like an alert() call.
$('.sub').click(function() { var tags = $('.add').val(); //use this instead of .attr() method $.post('public/ajax/upload_tags.php', {tags_to_add:tags}, function(data) { alert(data); }); });
-
-
so I mean the black background color for my sidebarItem div should "grow",right?
no, you have already answered this question yourself. The parent div's width is fixed at 240px, so no it will not grow. If you want it to expand don't set a fixed width.
The text does not wrap correctly because it is all on string, and you have not allowed it to be broken.
To allow it to break, you will need to adjust the CSS accordingly.
.sidebarItem/*holds itms inside the sidebar box: e.g. ticker, visual funds tracker*/ { background-color:green; padding:5px; border:1px solid black; word-wrap: break-word; //added this }
-
I meant to edit my post.
but the site wont let me
I got it to work:
if (preg_match("/^[a-zA-Z0-9]*((-|\.)?[a-zA-Z0-9])*\.([a-zA-Z]{2,4})$/", $text)) { message("You cannot post links or urls unless you have made 10 Posts"); }
But, if people enter
".com2" it still let's them, I need to add a wildcard to the .com so if they have any text characters after the .com or .net that it errors out, any idea fellas?
really, this is not an ideal regex as it will allow a lot of unwanted things through.
I recommend you use filter_var
var_dump(filter_var("example@example.com", FILTER_VALIDATE_EMAIL));
-
if you are simply wanting to check for a files existence, you should be using file_exists.
Rewrite the code using that function and provide a little more of the relevant code so we can more accurately attack this issue.
-
I do not know of one, but googling "notepad++ plugin list" gave me many results for plugins. Try to search around.
Form Help
in PHP Coding Help
Posted
He is referencing the input field by it's class, which is fine.
What format is the date being passed in?
To be inserted into a DATE field, it needs to be YYYY-MM-DD