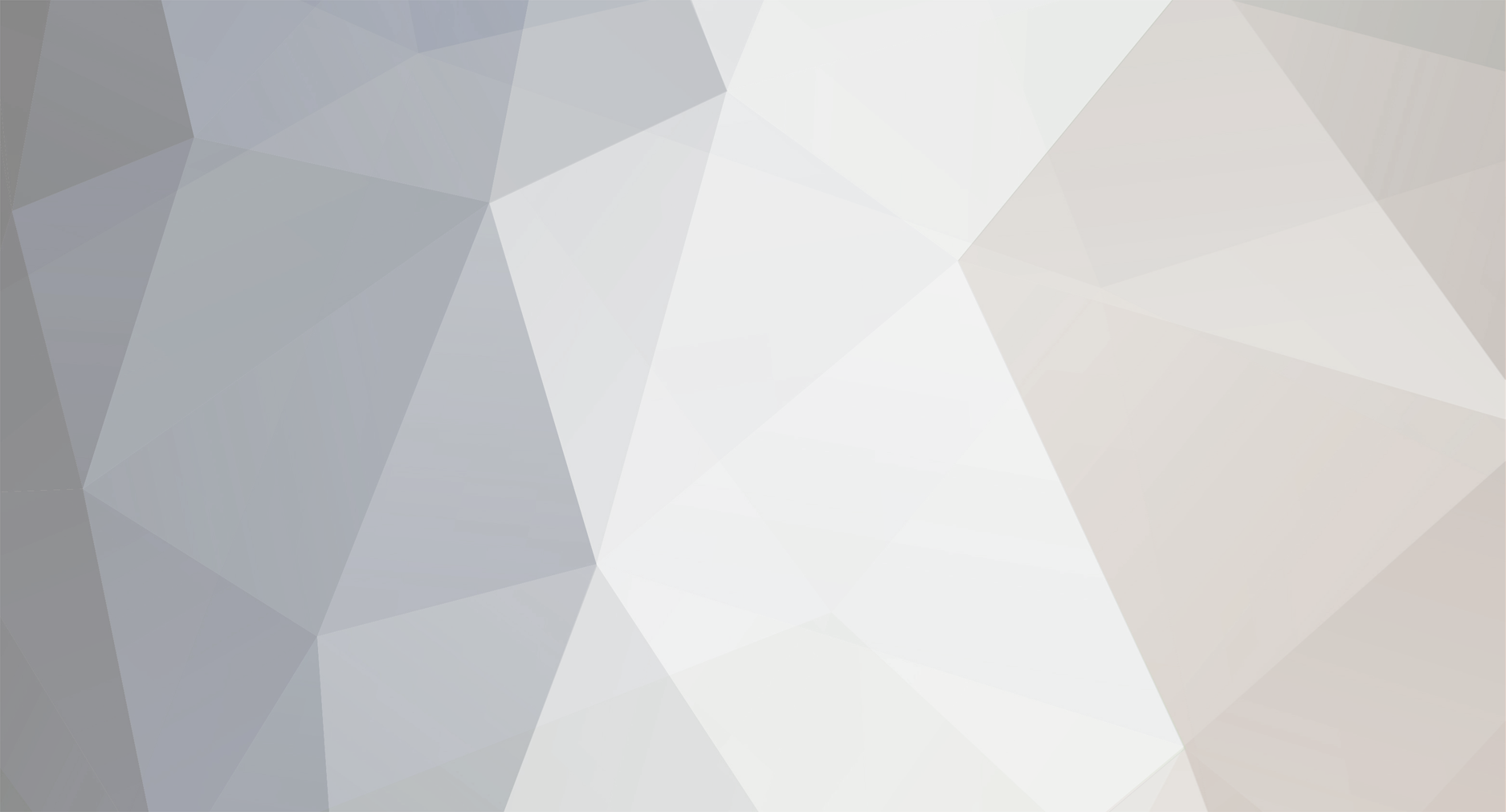
AyKay47
-
Posts
3,281 -
Joined
-
Last visited
-
Days Won
1
Posts posted by AyKay47
-
-
the second argument of implode is expected to be an array.
Is $list2Field an array?
Seeing that it is coming from a $_POST value, i will assume that its not.
-
Simply create a .htaccess files in the htdocs folder (doc root for xampp).
However before you do this, make sure that this directive:
#LoadModule rewrite_module modules/mod_rewrite.so
in the servers http.conf file (normally found in \apache\conf) is not commented so the module is enabled.
-
preg_match("/^[\.A-z0-9_\-\+]+[@][A-z0-9_\-]+([.][A-z0-9_\-]+)+[A-z]{1,4}$/", $str);
will match an email
http://www.addedbytes.com/cheat-sheets/regular-expressions-cheat-sheet-version-1/
this is a regex cheet sheet that i use explanes alot of stuff...
not to be rude, but that is one of the grossest email regex I have seen in a while.
There are so many things wrong with it that I'm not even sure where to start.
That regex would match an "email" like test@test.com.com.com.com.com.com.org.co
This is one I like to use:
~^[a-z0-9._%+-]+@[a-z0-9.-]+\.[a-z]{2,4}$~i
depending on the context you could wrap it in word boundaries instead of anchors.
-
A couple of things:
1. set the list items to float left not the anchors, you typically want the parent most element to float not a child.
2. having display: block with floating items is pretty useless since the float property takes the element out of position anyway and is typically used to stack elements.
3. What is the total width of these elements?
How large are the photos?
Is there any chance that the elements simply are too large to fit into 940px?
with these things being said, my css would look something like this (note that this is pseudo code since I do not know how large the images are etc..):
.grid_12 { float: left; //should this really be floating? width: 940px; } .grid_1, .grid_2, .grid_3, .grid_4, .grid_5, .grid_6, .grid_7, .grid_8, .grid_9, .grid_10, .grid_11, .grid_12 { margin: 0 10px; } .topbar { height: 60px; background-color: #fff; } .fl { float: left; } .fr { float: right; } .user-menu ul { list-style-type: none; } .user-menu li { //changed to list items float: left; } .round { border-radius: 5px; } .icon { background-color: red; background-repeat: no-repeat; background-position: center; padding: 13px; margin-right: 5px; } .ic-user { background-image: url('../images/icons/ic_user.png'); } .ic-settings { background-image: url('../images/icons/ic_settings.png'); } .ic-messages { background-image: url('../images/icons/ic_mail.png'); } .ic-logout { background-image: url('../images/icons/ic_cancel.png'); } .mini-info { background-color: black; font-size: 12px; color: #fff; padding: 4px; border-radius: 7px 5px 5px 7px; }
I question the floats that you have, but that might just be because I can't see the entire layout.
some minor changes, but will certainly make a difference.
If this still does not work for you, please post a full screen shot of the page so I can see the full layout.
-
well, you won't want them set to display: block anyway.
If this did not solve the issue, you will need to provide all of the relevant code, even if you think it is not causing it. I suspect some kind of width issue. But I cannot be sure without seeing the full css and relevant html.
-
This is because you have set .mini-info to display: block;
This will cause the span to be a block, thus creating a break before and after the element.
You will probably want .mini-info to be display: inline-block instead.
-
what does your query look like atm?
-
Alright, you asked for my help.
So you are going to have to post the relevant updated code along with the issue, as it appears that Zane has pretty much already answered this and Pika helped.
-
In my day, we didn't even have sticks.
How did you make fire?
Scared the fat women so they'd run, and let thigh friction do the rest . . .
oh my..
-
I figured it out! I need a second pair of quotes outside of the first set.
ManiacDan has a good point. If you are not going to take the time to read and understand the responses, why bother posting at all? I have no idea why you feel the need to add slashes to that bit of code, but they are not needed at all, as ManiacDan has already pointed out. Rather frustrating.
-
That pattern will match a standard email. But will also match things like test@test.helooooooooooooooooooooo (which apparently the code in this forum will match)
which clearly is not an email.
Also, there are numerous characters that are valid in email addresses that you have left out of your pattern (dashes, underscores, etc..)
post a sample string and your logic for matching the email address.
-
One thing that I do notice is the regex that you are using to filter usernames.
Really, a regex of this nature is not necessary if you are allowing any character through.
Simply using strlen for this to check the strings length would be a quicker way of doing it.
Also, comments in code should describe why something is being done, not what is being done. Things like this:
// Validate Username. if (empty($trimmed['username'])){ // No Username. $errors['username'] = 'Enter your Username.'; }else{ // Username Exists. if (preg_match('~(?x) # Comments Mode ^ # Beginning of String Anchor (?=.{8,30}$) # Ensure Length is 8-30 Characters .* # Match Anything $ # End of String Anchor ~i', $trimmed['username'])){
Your comments are simply describing what you are doing, which we can already see from the code. What would be more helpful is why it is being done, if anything. This will make it easier for you or someone else to pick up your code at a later date and see why certain code is in place.
Just some things that I noticed.
-
if($_POST[$rows["catID"]] == "on"); //square brackets
-
The highest permissions a file should ever have are 0755.
Why do you need a loop if only one value is being sent to the server?
You do realize that opening a file in "w+" mode will truncate the file every time correct?
You should have error_reporting set to E_ALL or -1, and Display_errors set to "On", if it is a file permissions error, an error will be triggered.
A couple debugging precautions should be put in place here:
if(isset($_POST['submit'])) { $myFile ="notifications.txt"; $username = trim($_POST['username']); $fh = fopen($myFile, 'w+') or die("can't open file"); $data = 'POST: Key: ' . $k . ' - Value: ' . $v . " \n"; $fw = fwrite($fh, $data); if($fw === false) { echo "failed to write to file."; } fclose($fh); }
-
I'm pretty sure from this quote that its part of a full page of textI only need one little piece of data from this page. And the data that I want is in a span tag with an id.. Like so....<span id="TotalDue">$1,004.28</span>
well, then a few questions would be asked before I created any regex for the OP.
Is there only one page that you want to get a match from?
If no, then these questions would also be asked:
Will the desired match always be in a span?
Will the element always have the ID of "ToatalDue"?
-
Surely that would match the data between all tags on a page, not just the specific data in the span tag that the OP requested?
I'm assuming the string given is only part of the page, not the whole thing
if $str contains an entire page rather then one element, then yeah string functions are useless.
-
a try catch block is in no way shape or form a loop.
It basically is an object oriented approach to error reporting, and really depends on your style of coding.
this:
$number = $_GET['number']; if($number == 0) { echo "number cannot be 0"; } else { //proceed with code }
does the exact same thing as this:
$number = $_GET['number']; try { if($number == 0) { throw new Exception("number cannot be 0"); } //code down here will only be executed if the exception is not thrown } catch(Exception $e) { echo $e->getMessage(); //outputs "number cannot be 0" }
So, IMO if you are not programming in OO, you should stick to the if else blocks.
It makes more sense to use OO error_reporting if you code in OO, and procedural if you code in procedural.
But this is entirely up to you. I prefer to use try catch blocks simply because it allows me to check for multiple errors in one block and then proceed with the rest of the code, instead of having to use multiple elseif statements. Since a try block is broken out of after an Exception has been thrown and any code following the thrown Exception will not be executed, this allows for errors to be "tried" before the following code is executed.
-
Note that in my regex I used ~ ~ as the start/end delimiters, instead of /
That is why you are getting an error. While using the DOM Document is great, it's probably overkill for your example
really, so is using a regex.
Simple string functions will do.
$str = '<span id="TotalDue">$1,004.28</span>'; $start_pos = strpos($str,">") + 1; $end_pos = strpos($str, "<", $start_pos); $string = substr($str, $start_pos, $end_pos);
*untested*
-
Should I restrict what characters a new User can use for his/her "Username" or let them type in anything as long as it is 8-30 characters?
I caught hell here before for trying to restrict things like Name to the characters [A-Z \'.-]
Debbie
what point of view should this be from?
Personal opinion, security, etc...
If this is a user handle, really the allowed character set is up to you.
If this is for an actual name, obviously a validity check should be in place.
-
Is magic_quotes_gpc enabled?
Can you give us some code to work with.
-
. And, if I browse to the page it still shows in the address bar as www.mysite.com/football-team.php?team_id=1
well, because you will need to set up the internal links and such to point to the new rewritten url.
You aren't actually changing the pages location, just pointing certain URL's to it.
-
Is there enough bad words that you think it would make more sense to store them in a database? I would store them in an array and compare each word against the array.
$bad_words = array('shit','bitch','witch'); $name = "Manal Nor"; $comment = "Hello lovely world"; $full_string = strtolower($name . " " . $comment); $full_string_arr = explode(" ", $full_string); $check = 0; foreach($full_string_arr as $word) { if(in_array($word, $bad_words)) { $check = 1; break; } } if($check == 1) { //bad word contained in string }
-
escape the dash in the pattern so it is not treated as a metacharacter.
RewriteEngine On RewriteBase / RewriteRule ^football\-team/([0-9]+)$ football-team.php?team_id=$1
-
Sorry, must of misunderstood the first reply, I have now created both files instead of just bob.html and it works, so how come I can't get my URL's to display correctly. I want the url www.mysite.com/football-team.php?team_id=1 for example to be displayed as www.mysite.com/football-team/1
This is the code of my .htaccess file:
RewriteEngine On
RewriteBase /
RewriteRule ^football-team/([0-9]+)$ football-team.php?team_id=$1
Really, this should work.
What exactly do you mean by, "I can't get my URL's to display correctly"?
Need a little regex help. My first little scrape.
in Regex Help
Posted
You can use a variety of delimiters, / and ~ serve the same purpose when wrapping a regex.