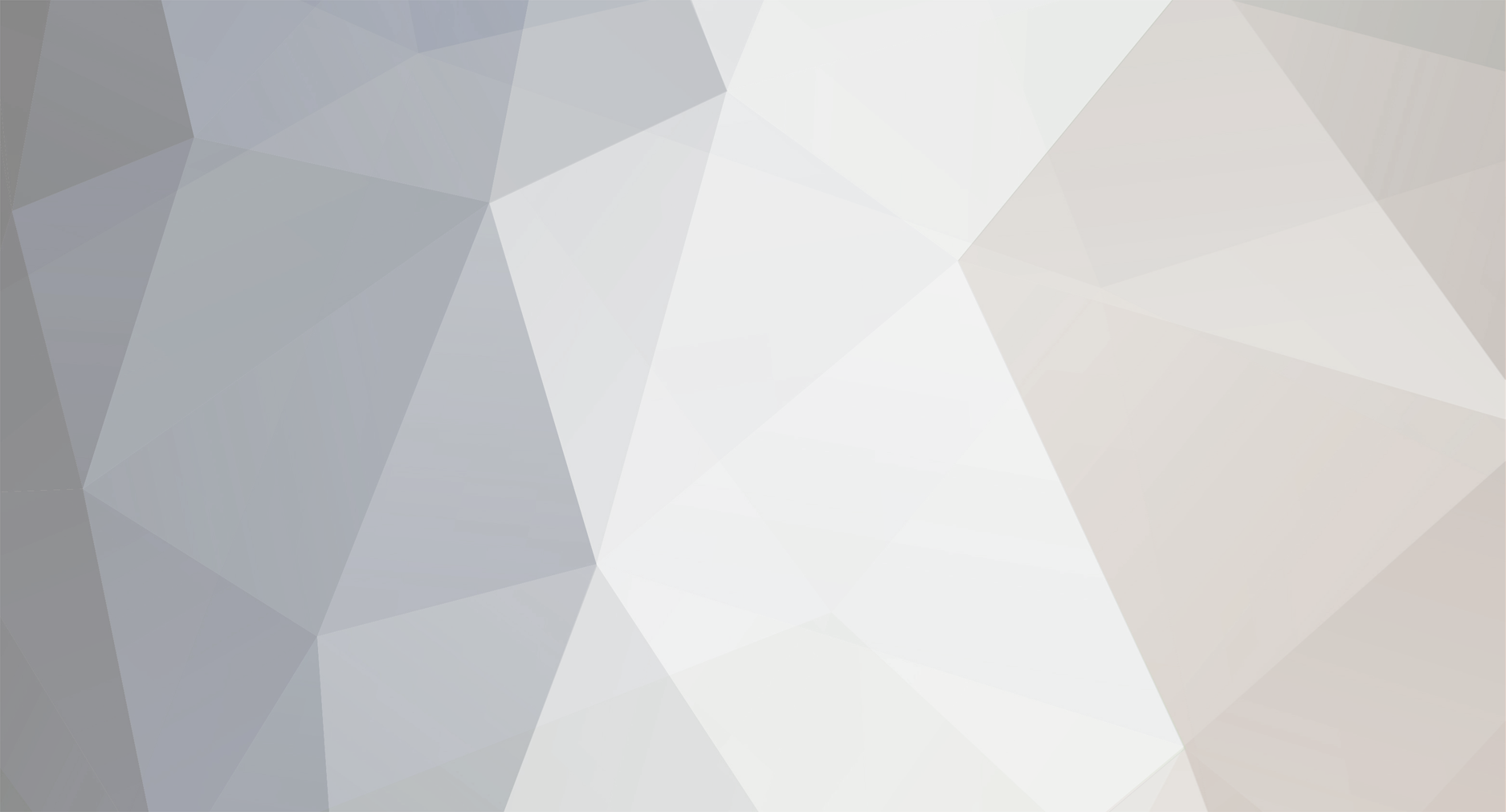
jazzman1
Staff Alumni-
Posts
2,713 -
Joined
-
Last visited
-
Days Won
12
Everything posted by jazzman1
-
Interesting. You want to upload files into an ftp server but using an html form instead an ftp client ??? That sounds me like you want to watch a movie using a code editor
-
Here's a pretty simple example how to insert and retrieve a password hashing string using md5() alg. 1. My database looks like this: 2. then, the php script should be similar like this <?php $_POST['password'] = 'myPassWord'; $salt = md5(uniqid(rand(), true)); $pass = md5($_POST['password'].$salt,true); // insert statement $query = "INSERT INTO users(password, salt_password) VALUES ('$pass','$salt')"; // retrieve the data and comparing the salted pass $query = "SELECT password, salt_password FROM users WHERE user_id = $userID"; $result = mysql_query($query); $row = mysql_fetch_assoc($result); if($row['password'] == md5($_POST['password'].$row['salt'])) { echo "passwords match"; } else { echo "passwords failed"; }
-
$sql = mysqli_query($con, "SELECT password, salt FROM user WHERE id ='".$user_id."'"); while($row = mysqli_fetch_array($sql)){ $salt = $row['salt']; $password = $password1; $hash = md5($salt . $password); The logic should be: if ($row['password'] == md5($data['password'].$row['salt']) where "$data['password']" is the user password input field! How did you salt the password? Is it something like that: $salt = 'salt_password'; $pass = md5($data['password']. $salt); I don't see how to insert the hashing data into a database in your examples. Can you show us the script, please?
-
Good for you.Your script looks good for me Anyway......now, you've got a good example of separating the application logic - business from presentation. You should get into the habit of using php error handling functions while developing a project.
-
Yeap, you got it. This is a pretty simple structure without any security, styling, javascript, etc.. but it would work. Get the image data (path,size,type, etc...) from a db table and display the image using an image tag. That's all you have to do. Try. It wouldn't hurt you
-
take the closing </table> and <span class="re"> out of the while loop. what's the point of using the span element? styling the errors?
-
then remove all commented lines by me
-
No errors?
-
Try, <?php ini_set('display_startup_errors', 1); ini_set('display_errors', 1); error_reporting(-1); ?> <?php session_start(true); ?> <?php require_once('/Connections/new.php'); ?> <?php /* if (!function_exists("GetSQLValueString")) { function GetSQLValueString($theValue, $theType, $theDefinedValue = "", $theNotDefinedValue = "") { if (PHP_VERSION < 6) { $theValue = get_magic_quotes_gpc() ? stripslashes($theValue) : $theValue; } $theValue = function_exists("mysql_real_escape_string") ? mysql_real_escape_string($theValue) : mysql_escape_string($theValue); switch ($theType) { case "text": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "long": case "int": $theValue = ($theValue != "") ? intval($theValue) : "NULL"; break; case "double": $theValue = ($theValue != "") ? doubleval($theValue) : "NULL"; break; case "date": $theValue = ($theValue != "") ? "'" . $theValue . "'" : "NULL"; break; case "defined": $theValue = ($theValue != "") ? $theDefinedValue : $theNotDefinedValue; break; } return $theValue; } } $colname_Recordset1 = "-1"; if (isset($_SESSION['MM_Username'])) { $colname_Recordset1 = $_SESSION['MM_Username']; } mysql_select_db($database_new, $new); $query_Recordset1 = sprintf("SELECT * FROM appointments WHERE username = %s", GetSQLValueString($colname_Recordset1, "text")); $Recordset1 = mysql_query($query_Recordset1, $new) or die(mysql_error()); $row_Recordset1 = mysql_fetch_assoc($Recordset1); $totalRows_Recordset1 = mysql_num_rows($Recordset1); */ ?> <title>Appointments</title> <style type="text/css"> body p { text-align: center; } body { background-image: url(slate.jpg); } .new { color: #FFF; } .new { font-weight: bold; } .new .new { text-align: left; } .new { color: #FFF; } .re { text-align: center; } .table .re { color: #FFF; } .table .re { color: #FFF; } </style> <link href="SpryAssets/SpryMenuBarHorizontal.css" rel="stylesheet" type="text/css" align="center"/> <script src="SpryAssets/SpryMenuBar.js" type="text/javascript"></script> </p> <link href="SpryAssets/SpryMenuBarHorizontal.css" rel="stylesheet" type="text/css" align="center"/> <script src="SpryAssets/SpryMenuBar.js" type="text/javascript"></script> </p> <ul id="MenuBar1" class="MenuBarHorizontal"> <li><a class="MenuBarItemSubmenu" href="success.php">Home</a> <li><a href="#">Gallery</a></li> <li><a class="MenuBarItemSubmenu" href="appointments.php">Visits</a> <li><a class="MenuBarItemSubmenu" href="#">Contact</a> </ul> <p align="center"><br> <br> </p> <p align="center"><img src="/images.jpg" width="323" height="156"><br /> <p align="center"> </p> <div align="center"> <span class="new"> <?php echo '<h2>Customer Appointments</h2>'; ?> </span></div><table width="1248" border="3" align="center"> <tr class="new"> <td width="116" class="re" style="text-align: center"><strong>Date Booked</strong></td> <td width="96" class="re" style="text-align: center"><strong class="table">Time Booked</strong></td> <td width="823" class="re" style="text-align: center"><strong class="table">Notes</strong></td> </tr> <?php $sql = sprintf("SELECT datebooked, timebooked, notes FROM appointments WHERE username='%s'", mysql_real_escape_string($_SESSION['MM_Username'])); $result = mysql_query($sql); // check query did execute without errors if ($result) { // output each file while ($row = mysql_fetch_assoc($result)): ?> <tr class="table"> <td class="re" style="text-align: center; color: #FFF;"><?php echo $row['datebooked']; ?></td> <td class="re" style="text-align: center"><?php echo $row['timebooked']; ?></td> <td class="re" style="text-align: center"><?php echo $row['notes']; ?></td> </tr> </table> <span class="re"> <?php endwhile; // end the while loop // query did not execute, log or show error message } else { trigger_error('Cannot get users files from database: ' . mysql_error()); } mysql_free_result($result); ?> </span> Let me know if you get an error.
-
I am at work now to get my time looking more deeply into this beautiful script, but you should die here thought $query_Recordset1 = sprintf("SELECT * FROM appointments WHERE username = %s", GetSQLValueString($colname_Recordset1, "text")); $Recordset1 = mysql_query($query_Recordset1, $new) or die(mysql_error()); What is $new? your database credentials?
-
This code should be immediately restructure from scratch You don't have to repeat your css and javascrip code adding them into while loops. Why are you using two identical queries: SELECT * FROM appointments WHERE username = %s" //and SELECT id, username, datebooked, timebooked, notes FROM appointments WHERE username=
-
Wow....it' weird....it's not from the code above I think, that script looks good for me. Disable css and try again.
-
No, it's not really about the Doctype declaration. You can set dynamically the content of the html meta tag by php or just, use a static html: <meta http-equiv="content-type" content="text/html;charset=utf-8" />
-
what about a css link?
-
it wisely
-
No. Don't. Just google - php gallery tutorial, find some decent one and pick it up. I am not trolling or being glib, but you are not a guy who is trying to learn into the good programming habits BTW: thanks for the beer mate
-
You can put the php content surround by <?php ?> tags everywhere you want in the document. Since php is a server-side language, all the fancy work is done and parsed from the php parser before any html content gets a peek by the browser. As for the variable name $u_name you can be free to use any name you want following the rule of a valid php variable name.
-
Try the following and tell me if you get an error: <?php ini_set('display_startup_errors', 1); ini_set('display_errors', 1); error_reporting(-1); if (isset($_POST['upload']) && $_FILES['userfile']['size'] > 0) { $fileName = $_FILES['userfile']['name']; $tmpName = $_FILES['userfile']['tmp_name']; $fileSize = $_FILES['userfile']['size']; $fileType = $_FILES['userfile']['type']; $u_name = $_POST['username']; $fileType = (get_magic_quotes_gpc() == 0 ? mysql_real_escape_string( $_FILES['userfile']['type']) : mysql_real_escape_string( stripslashes($_FILES['userfile']))); $fp = fopen($tmpName, 'r'); $content = fread($fp, filesize($tmpName)); $content = addslashes($content); fclose($fp); if (!get_magic_quotes_gpc()) { $fileName = addslashes($fileName); } $con = mysql_connect('localhost', 'username', 'password') or die(mysql_error()); $db = mysql_select_db('company', $con); $query = "INSERT INTO upload (`username`, `name`, `size`, `type`, `content`) VALUES ('$u_name','$fileName', '$fileSize', '$fileType', '$content')"; $result = mysql_query($query,$con); if ($result) { echo "<br>File $fileName uploaded<br>"; } else { echo "file upload failed"; exit; } } ?> <html> <head></head> <body> <form method="post" enctype="multipart/form-data"> <table width="350" border="0" cellpadding="1" cellspacing="1" class="box"> <tr> <td>please select a file</td></tr> <tr> <td> <input type="hidden" name="MAX_FILE_SIZE" value="99000000"> <input name="userfile" type="file" id="userfile"> <input name="username" type="text" id="username"> </td> <td width="80"><input name="upload" type="submit" class="box" id="upload" value=" Upload "></td> </tr> </table> </form> </body> </html>
-
what linux distro are you using?
-
This is your script from reply #31. <html> <head></head> <body> <form method="post" enctype="multipart/form-data"> <table width="350" border="0" cellpadding="1" cellspacing="1" class="box"> <tr> <td>please select a file</td></tr> <tr> <td> <input type="hidden" name="MAX_FILE_SIZE" value="99000000"> <input name="userfile" type="file" id="userfile"> <input name="username" type="text" id="username"> ADDED THIS LINE </td> <td width="80"><input name="upload" type="submit" class="box" id="upload" value=" Upload "></td> </tr> </table> </form> </body> </html> <?php if (isset($_POST['upload']) && $_FILES['userfile']['size'] > 0) { $fileName = $_FILES['userfile']['name']; $tmpName = $_FILES['userfile']['tmp_name']; $fileSize = $_FILES['userfile']['size']; $fileType = $_FILES['userfile']['type']; $username = $_FILES['username']['username']; ADDED THIS LINE HERE $fileType = (get_magic_quotes_gpc() == 0 ? mysql_real_escape_string( $_FILES['userfile']['type']) : mysql_real_escape_string( stripslashes($_FILES['userfile']))); $fp = fopen($tmpName, 'r'); $content = fread($fp, filesize($tmpName)); $content = addslashes($content); fclose($fp); if (!get_magic_quotes_gpc()) { $fileName = addslashes($fileName); } $con = mysql_connect('localhost', 'username', 'password') or die(mysql_error()); $db = mysql_select_db('company', $con); if ($db) { $query = "INSERT INTO upload (username name, size, type, content ) " . ADDED USERNAME AT THE START "VALUES ('$fileName', '$fileSize', '$fileType', '$content')"; mysql_query($query) or die('Error, query failed'); mysql_close(); echo "<br>File $fileName uploaded<br>"; } else { echo "file upload failed"; } } ?> Replace $username = $_FILES['username']['username']; to $u_name = $_POST['username']; Your query should be: $query = "INSERT INTO upload (`username`, `name`, `size`, `type`, `content`) VALUES ('$u_name','$fileName', '$fileSize', '$fileType', '$content')";