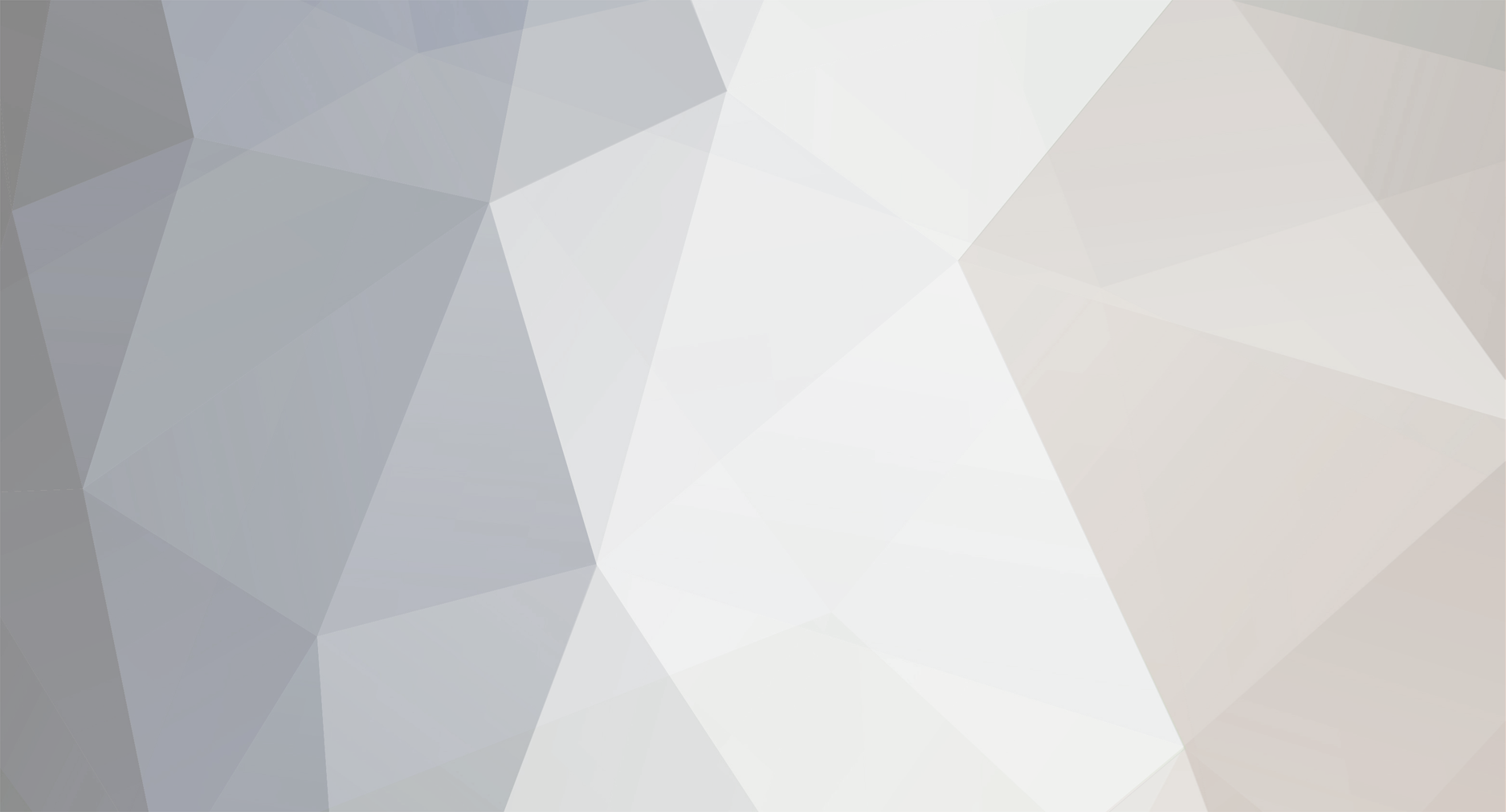
scootstah
Staff Alumni-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Everything posted by scootstah
-
The query is probably failing due to an error, run this and post back the output: $query = 'SELECT * FROM items WHERE ' . $where; $result = mysql_query($query) or die ('Query: ' . $query . '<br />Error: ' . mysql_error());
-
In that case, you would only need one form. You could do something like if (!empty($_POST)) { $price = $_POST['price']; $street = $_POST['street']; $area = $_POST['area']; $where = array(); if (!empty($price)) { $where[] = "price LIKE '%$price%'"; } if (!empty($street)) { $where[] = "street LIKE '%$street%'"; } if (!empty($area)) { $where[] = "area LIKE '%$area%'"; } $where = implode(' OR ', $where); $result = mysql_query('SELECT * FROM houses WHERE ' . $where); if (mysql_num_rows($result) > 0) { while($row = mysql_fetch_assoc($result)) { echo 'results...<br />'; } } else { echo 'no matches!'; } } ?> <form action="" method="post"> Price: <input type="text" name="price" /><br /> Street: <input type="text" name="street" /><br /> Area: <input type="text" name="area" /><br /> <input type="submit" name="submit" value="Search" /> </form>
-
Without knowing more I'd say you'd probably want 3 forms.
-
Can you post the rest of the code?
-
Try $result1 = mysql_query("delete FROM `users` WHERE militia=$id") or die(mysql_error());
-
This str_replace for an external file isnt working!
scootstah replied to Allenph9's topic in PHP Coding Help
Starting a variable with _ is completely valid. The only reason it didn't work in this case is because he set the handle to $fh1, and instead used $_fh1. -
Variables created inside a function will only ever exist inside that function. Likewise, variables created outside of a function can not exist inside the function. When you call the function multiple times, you are making new instances of it. Nothing is being replaced. Using global will allow you to bypass function variable scope. However, it is very bad practice and should be avoided.
-
Essentially, you would need to have a mousedown event, and then a mousemove event. When you fire the mousedown event, you save the mouse coordinates. You can then compare these coordinates to the new coordinates while dragging.
-
You shouldn't parse it before it goes into the database. If anybody ever edits it you are going to have to convert all the HTML back into bbcode, or else it will just be turned to entities.
-
Remove the "&"s.
-
Use htmlspecialchars before you parse the bbcode.
-
Is there a way to not call each file in index.php?
scootstah replied to Mal1's topic in PHP Coding Help
He said they are .tpl files, so I'm thinking he has some sort of template parser. -
Is there a way to not call each file in index.php?
scootstah replied to Mal1's topic in PHP Coding Help
This is actually the preferred approach. This approach has several advantages. You can have pretty URL's, you won't have duplicated code, bugs can be easier to track down, it can prove a little more secure, etc. Here is a diagram of how CodeIgniter (and many other applications) route the application. -
Sessions
-
You still haven't changed the comparison value. echo "<option value='0.00' " . ($array['roastturkey'] == '0.00' ? 'selected="selected"' : '') . ">0</option>"; echo "<option value='1.00' " . ($array['roastturkey'] == '1.00' ? 'selected="selected"' : '') . ">1</option>"; echo "<option value='2.00'> " . ($array['roastturkey'] == '2.00' ? 'selected="selected"' : '') . ">2</option>"; echo "<option value='3.00'> " . ($array['roastturkey'] == '3.00' ? 'selected="selected"' : '') . ">3</option>"; echo "<option value='4.00'> " . ($array['roastturkey'] == '4.00' ? 'selected="selected"' : '') . ">4</option>"; echo "<option value='5.00'> " . ($array['roastturkey'] == '5.00' ? 'selected="selected"' : '') . ">5</option>"; echo "<option value='6.00'> " . ($array['roastturkey'] == '6.00' ? 'selected="selected"' : '') . ">6</option>"; echo "<option value='7.00'> " . ($array['roastturkey'] == '7.00' ? 'selected="selected"' : '') . ">7</option>"; echo "<option value='8.00'> " . ($array['roastturkey'] == '8.00' ? 'selected="selected"' : '') . ">8</option>"; echo "<option value='9.00'> " . ($array['roastturkey'] == '9.00' ? 'selected="selected"' : '') . ">9</option>"; echo "<option value='10.00'> " . ($array['roastturkey'] == '10.00' ? 'selected="selected"' : '') . ">10</option>";
-
Video uploading script with conversion to multiple file types?
scootstah replied to jbonnett's topic in PHP Coding Help
Keep in mind that doing this is pretty resource intensive. So if you are trying to encode multiple files at once you are going to hog a lot of resources. If you're on a shared host, they are going to get mad very quickly. For anything that has anywhere near decent traffic and lots of uploaded videos, you're going to need some serious resources to keep up with demand. -
You'll have to hard refresh the page, so hit ctrl+f5. Or, you could look at the source to see if the HTML is correct.
-
You need to check that the value matches the value of the option tags. echo "<option value='0.00' " . ($array['roastturkey'] == '0.00' ? 'selected="selected"' : '') . ">0</option>"; echo "<option value='1.00' " . ($array['roastturkey'] == '1.00' ? 'selected="selected"' : '') . ">1</option>"; echo "<option value='2.00'> " . ($array['roastturkey'] == '2.00' ? 'selected="selected"' : '') . ">2</option>"; echo "<option value='3.00'> " . ($array['roastturkey'] == '3.00' ? 'selected="selected"' : '') . ">3</option>";
-
Remove the whole if statement.
-
Because that was pseudo test code, you need to put the real value from the database into the ternary statement. So replace $array['wings'] with whatever the column is from your database.
-
You would need to concatenate the ternary statement. echo "<option value='1.00' " . ($array['roastturkey'] == 0 ? 'selected="selected"' : '') . ">0</option>";
-
You're going to need to store the user id in a session or something.
-
Then by modifying my first response, this should work: $result = mysql_query("SELECT * FROM pass WHERE id = 4 "); $row = mysql_fetch_assoc($result); $selected = $row['cutlets']; $options = array( '0.00' => '---', '1.00' => '1', '2.00' => '2', '3.00' => '3' ); foreach($options as $key => $val) { echo '<option value="' . $key . '" ' . ($key == $selected ? 'selected="selected"' : '') . '>' . $val . '</option>'; }
-
What is the output when you echo the query? echo $edit_query_insert;
-
What is the column in your table called that you wish to use as the dropdown selection?