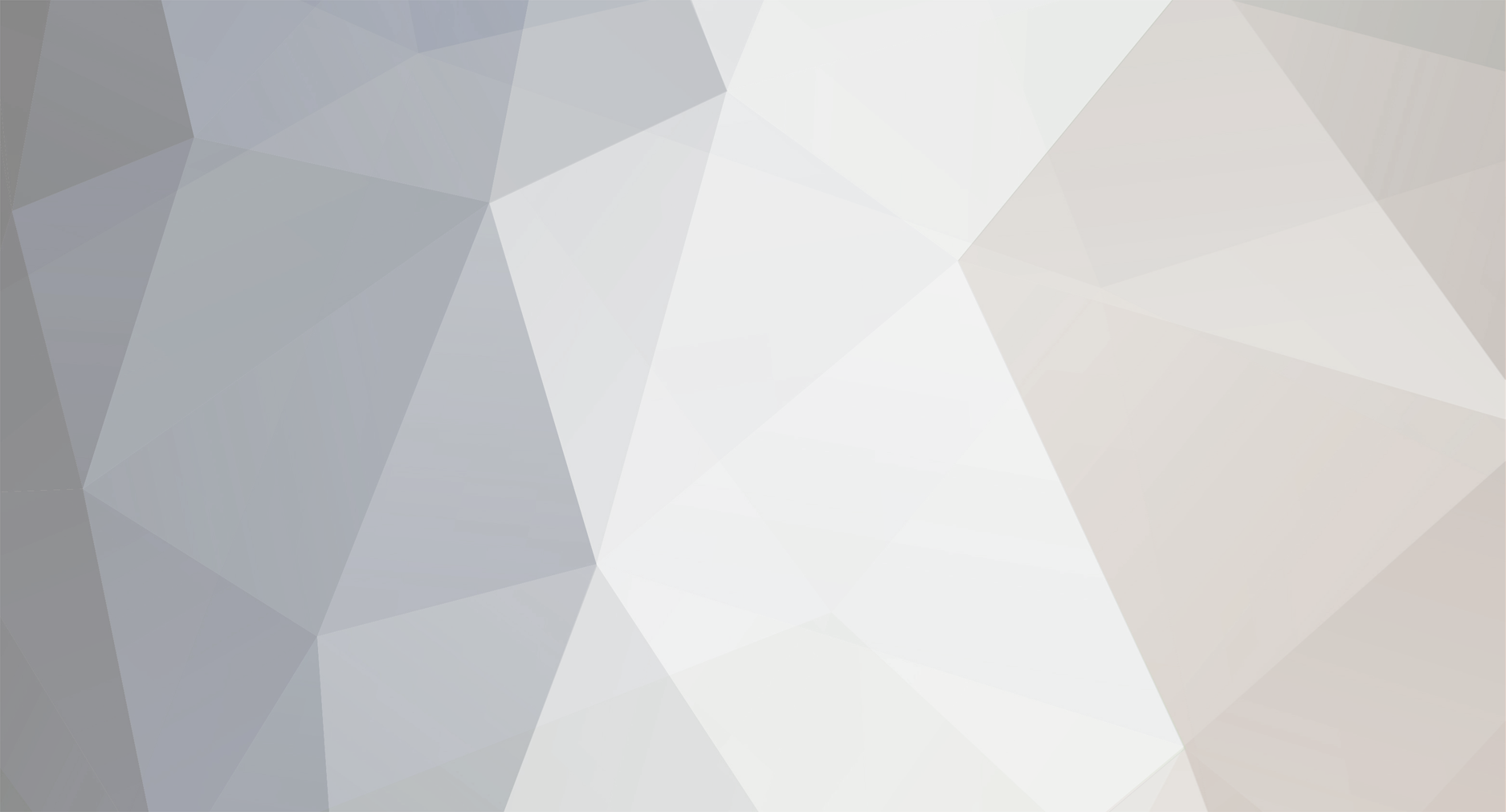
scootstah
-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Posts posted by scootstah
-
-
Not really. What I am talking about is on your index.php page, you would have code such as:
<?php define('CURRENT_PAGE', 'home'); //...
Then in your file where you need to test if your on the home page or not, use
<?php if (defined('CURRENT_PAGE') && CURRENT_PAGE=='home'){ // on home page } else { //not on home page }
Nah, that seems too manual and too crude to me.
Debbie
Too manual? Well generally configuration options are...
This is the best way to make sure your URL's are correct. Things may vary in server configurations and such, and if you need to dynamically create URL's for example then you have a way to control them.
-
I added it, but I made a typo - so here it is:
if ($userGuess<$randNum) { echo "<center>You guessed too low!</center>"; $_SESSION['guesses']++; } if ($userGuess>$randNum) { echo "<center>You guessed too high!</center>"; $_SESSION['guesses']++; } if ($userGuess==$randNum) { echo "<center>Congratulations You're right!!!</center>"; unset($_SESSION["randNum"], $_SESSION['guesses']); }
Note the "$_SESSION['guesses']++" lines. Every time they make a wrong guess, the guesses is increment.
-
Try this:
game.php
<?php session_start(); $_SESSION['randNum'] = isset($_SESSION['randNum']) ? $_SESSION['randNum'] : rand(1, 100); $_SESSION['guesses'] = isset($_SESSION['guesses']) ? $_SESSION['guesses'] : 0; ?> <html> <body> <center><form action="game_check.php" method="POST"> Guess a number 1-100:<input type="text" name="userGuess"/> <input type="submit" value="Guess"/> </form></center> <center><form action="game.php"> <input type="submit" value="Restart"/> </form></center> </body> </html>
game_check.php
<?php session_start(); $randNum = $_SESSION['randNum']; $userGuess=$_POST["userGuess"]; if (isset($randNum)) { if ($userGuess<$randNum) { echo "<center>You guessed too low!</center>"; $_SESSION['randNum']++; } if ($userGuess>$randNum) { echo "<center>You guessed too high!</center>"; $_SESSION['randNum']++; } if ($userGuess==$randNum) { echo "<center>Congratulations You're right!!!</center>"; unset($_SESSION["randNum"], $_SESSION['guesses']); } } else { echo "Uh oh"; } ?>
-
Firstly, you should use isset() instead of == null. In your code, if a field is left out you will get a PHP Notice: undefined index.
Secondly I believe this is your problem:
if ([array count] == 0)
It should be
if (empty($error)) {
-
About frameworks, what would happen if I started using one and it died ? (Discontinued...)
That is pretty unlikely to happen if you use one of the more popular ones (IE: CodeIgniter, Kohana, Symfony, CakePHP, Zend, etc).
-
You could use is_int() to see if it is a number. And you could use strlen() to see if it is a certain amount of characters.
$price = $_POST['price']; if (is_int($price) && strlen($price) == 5) { // good to go }
What do you mean by "run a script"? strip_tags() will remove HTML tags, which could prevent XSS attacks.
-
I really doubt you will have that kind of control over any shared hosting. You'll likely need a VPS for that.
-
I don't see a question anywhere. What is this doing or not doing that isn't right?
-
I guess I am confused. Why don't you just change what is included already to instead use the newer version of jQuery?
-
I could help more if you showed me the markup in which you are using this, but maybe this will get you started: http://jsfiddle.net/kgeHe/1/
-
I think you want something like this: http://jsfiddle.net/kgeHe/
-
I believe the GROUP BY function is what you are looking for.
SELECT * FROM SELECT * FROM uitems WHERE username='$showusername' AND location='2' GROUP BY name
Also, you shouldn't use mysql_fetch_array() unless you for some reason specifically require what it does. mysql_fetch_array() returns both an associative array and a numerical array, so your array holds the contents twice - and this is entirely unnecessary. You should either use mysql_fetch_row() (if you want a numerical array) or mysql_fetch_assoc() (if you want an associative array - which in your example, you do).
-
Like I said, without showing me the markup for your dropdown then I really can't help you any further.
Maybe this example will help you: http://jsfiddle.net/uKpUC/
-
I don't know because I'm not familiar with it.
However you can easily solve this by giving each form field a unique name. So like iks1, iks2, iks3 ... etc.
-
Open your web browser, and go to http://localhost
Any PHP files will need to be in the server document root. This is defined in Apache's virtual host config file.
So if you put "insert_ac.php" in the document root (which appears to be C:\wamp\www in your case) then you would then type http://localhost/insert_ac.php into your web browser.
-
Hmm. Without knowing how you wish to use the information I can't offer a better suggestion.
But if it's available today, it should also be available in the future no?
-
I use Eclipse PDT. I just like the feel of it. Powerful if it needs to be, but simple and elegant if not.
I also use Notepad++ for quick edits and such.
-
That's because (I'm assuming) quickform automatically loads the values back into the form when you press submit. And since they all have the same names, they all get the same value.
-
I don't know - how do you have codeigniter set up?
-
Oh, I see. Is the reserved_to/from fields DATE/DATETIME?
If yes you can try
WHERE reservations.reserved_to < CURDATE()
-
Because they all have the same name.
-
I believe he updated your jsfiddle.
-
In PHP, variables cannot start with a number. Where is this $1 supposed to be coming from?
EDIT: Maybe this is what you wanted?
$postip = $argv[1];
-
So are you getting any Javascript errors?
Tell the script to restart from the beginning if it encounters any error
in PHP Coding Help
Posted
PHP has a default max_execution_time of 30 seconds, so this would terminate before that time limit is even reached.