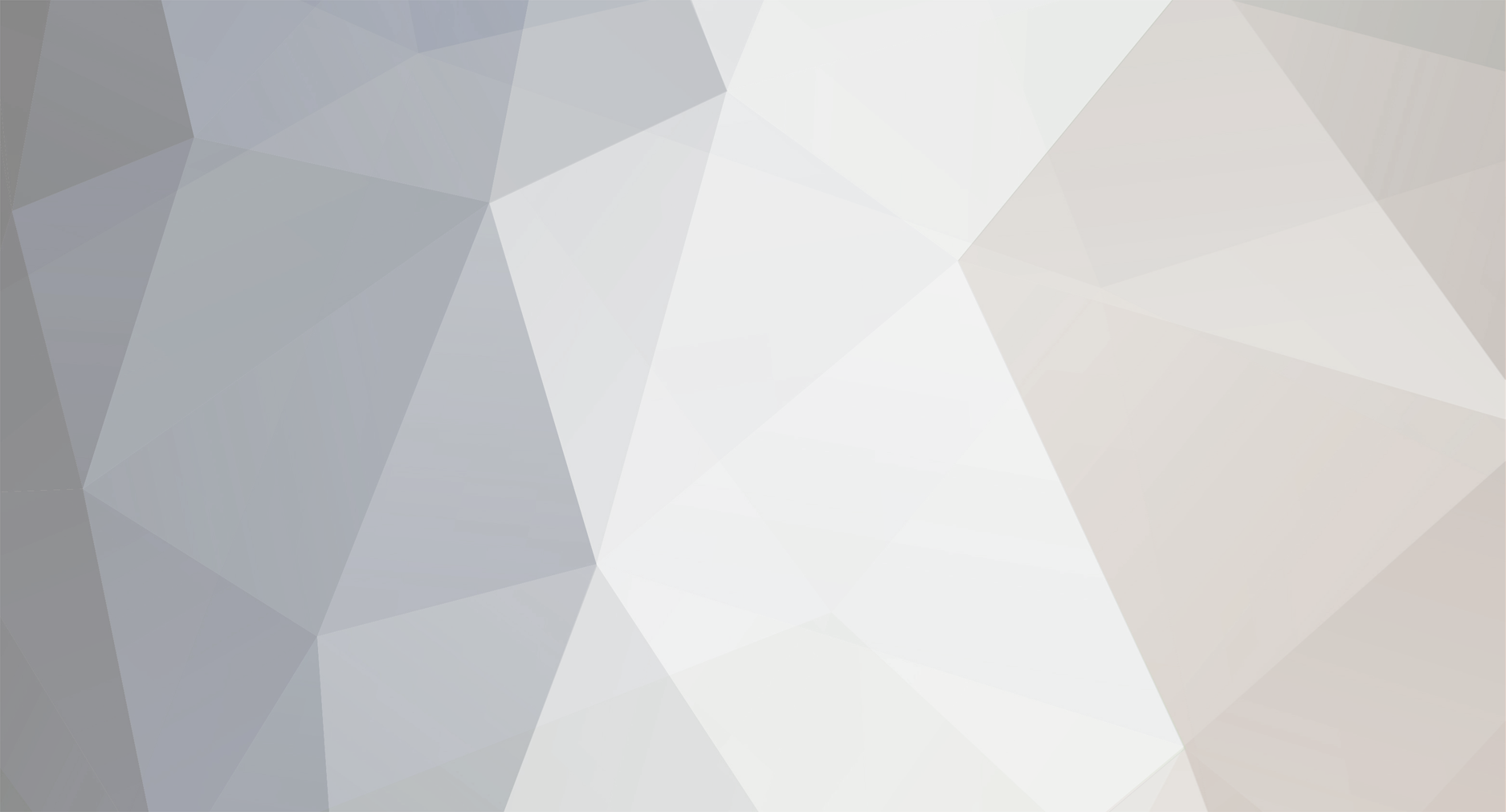
scootstah
-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Posts posted by scootstah
-
-
1) If you've never used a framework, then you can't go wrong with CodeIgniter. It has the best documentation by far, and is generally pretty beginner friendly - plus it's awesome.
2) Yes, generally SQL injection isn't an issue.
3) Most frameworks have lots of functionality that make security a lot easier and safer. Remember that frameworks are community driven, so security holes are usually found and patched quickly.
-
Should I copy that code into the log in page and into all the pages that I want the log in to continue active?
Yes - you must have session_start() at the top of every page in which you wish to use session functionality.
Also if you are going by my example, make sure you set $_SESSION['logged_in'] = true when you login.
-
Isn't that what you wanted? To not show rows that meet those conditions?
-
Something like this?
$sql = mysql_query("SELECT * FROM slideshow"); if(mysql_num_rows($sql) > 0) { $images = array(); $captions = array(); while($row = mysql_fetch_assoc($sql)) { $images[] = array( 'slideID' => $row['slideID'], 'image' => $row['image'] ); if ($row['caption'] == 1) { $captions[] = array( 'slideID' => $row['slideID'], 'caption_h3' => $row['caption_h3'], 'caption_content' => $row['caption_content'], 'caption_link' => $row['caption_link'], 'caption_link_text' => $row['caption_link_text'] ); } } echo '<div id="slider">'; foreach($images as $i) { echo '<img src="' . $i['image'] . '" alt="" title="#htmlcaption{' . $i['slideID'] . '}" />'; } echo '</div>'; if (!empty($captions)) { foreach($captions as $c) { echo '<div id="htmlcaption{' . $c['slideID'] . '} class="nivo-html-caption"> <h3>' . $c['caption_h3'] . '</h3> <p>' . $c['caption_content'] . '</p> ' . ($c['caption_link'] != '' ? '<p><a href="' . $c['caption_link'] . '">' . $caption_link_text . '</a>' : ''); } } }
-
Try something like this:
SELECT config FROM inettuts WHERE user_id = '5' AND config NOT LIKE '%Twitter%' AND config NOT LIKE '%color-grey%' AND config NOT LIKE '%not-collapsed%'
-
First off, $numOfWingsCount will never be greater than 2 because you set it to 0 every iteration. You need to assign it to 0 before the for loop.
If you were using an array this would be easier. You could do:
$match = array('W', 'W', 'L'); // inside for loop if ($match[$matchWcounter] == 'W'
Otherwise you have to do something like this:
// inside for loop if (${'match' . $matchWcounter} == 'W'
I can't test this right now so, try it and see if it works.
-
Then it is a sessions issue, or an issue with how you are implementing them.
Do something like this on each page:
session_start(); if ($_SESSION['logged_in'] === true) { // logged in }
Make sure you set $_SESSION['logged_in'] = true when you log in.
Also note that session_start() has to be on every page (where you want this functionality) or this won't work.
-
Can you post what this array might look like? I'm a little confused.
-
Almost!
Change
echo $meeys[$matchWcounter];
To
echo $meeys . $matchWcounter;
-
1. I copied the code from a tutorial…What should I do instead of a cookie?
You can use a cookie, just don't store a password in it. You should store as little as you possibly can. To auto login users after their session has expired, generally you would make an autologin cookie. This cookie will contain a unique auto login key assigned to the user when they first logged in. It will be stored in a database table and used to login the user.
The database table should have these columns : user_id, autologin_key, ip_address, user_agent, time
The user_id is, obviously, the user's id and is the PRIMARY KEY. This is how you will look up the user after matching the autologin key.
The autologin_key is the unique key assigned to the user when they first login. It will probably be an MD5 or SHA1 hash, so 32 or 40 characters in length.
The ip-address and user_agent will be optionally used to verify the cookie wasn't stolen. If it has a different IP and user agent, then it may be a safe bet to destroy that autologin and make them login again.
The time will be the time at which the autologin was created. This is so you can expire them after so long and force them to relogin.
The code is pretty simple. When they first login, just create a unique key and insert it into the autologin table.
$autologin_key = sha1(uniqid(mt_rand(), true);
Now, store this key in a cookie. When the user's session expires you can check if an autologin cookie exists. If it does, see if that autologin key was found in the database. If it was, you can (optionally) compare the ip address's, user agents, and see if it has expired. If all of these check out, you can login the user.
This is a better approach than storing a password in a cookie, because in the event the cookie gets comprised, while they can potentially hijack that user's account they won't be able to steal their password (where they could then compromise accounts on other websites).
4. I'm not sure what you mean here.
if(!$_POST['username'] | !$_POST['userpassword']) {
You have a single | between these two items. What you wanted was ||, which is a logical operator meaning "or".
So it should read
if(!$_POST['username'] || !$_POST['userpassword']) {
6. I did the session_start() but it didn't do anything for me. but i will put it back inWell, session_start() doesn't "do" anything. It allows you to use sessions and keep them active between pages.
-
You could send a POST from any page to that page and it would still process it. Or just use GET for that matter, since you are using REQUEST.
-
The "from" is just a header and doesn't mean much. You can still see where the email actually came from.
With that said, I don't think you should make the "from" another user's email address. Maybe make the reply-to their email address. Make the from something like "no-reply@domain" or something.
-
Please use code tags.
I don't really know what the question is here.
When you have multiple rows returned you have to loop through them. If you just do $array = mysql_fetch_array() it's only going to return one row. So you have to loop through them.
If you want to see if a specific category exists you can do one of these two things:
1. Select all rows and use in_array:
$query = mysql_query("SELECT * FROM categories"); $categories = array(); while($row = mysql_fetch_assoc($query)) { $categories[] = $row; } if (in_array('specific category', $categories)) { // category exists }
2. Select only the specific row and then see if any rows were returned
$query = mysql_query("SELECT * FROM categories WHERE category='specific category'"); if (mysql_num_rows($query) > 0) { // category exists }
Hope that helps.
-
Please use code tags.
Have you compared the database result beside the serialized data before it went into the database? What is different about it?
I'm guessing you are either exceeding the character length or you have magic_quotes on.
-
No offense, but you seem to be copy and pasting code left and right. You have some very wrong things in that code. How does it not throw a ton of errors?
1. Never store a password in a cookie and use it in that way. Terrible idea. There is never a reason you need to store a password in a cookie.
2. You are missing a quotation and a comma here:
mysql_connect("hose "name", "password") or die(mysql_error());
3. header(" testing_login.php"); - This is not how you redirect. You want
header('Location: testing_login.php');
4. You need another | here:
if(!$_POST['username'] | !$_POST['userpassword']) {
5. You are putting things in while loops that don't need to be there.
6. session_start() should be called at the top of the page.
7. $sessionvar = $this->GetLoginSessionVar(); - I have no idea what you are trying to do with this. You can't use $this in a procedural function.
8. if(empty($_SESSION['$sessionvar'])) - You can't use single quotes with a variable because it will output what is literally contained in the quotes. When dealing with array keys you don't need any quotes if you are passing a variable.
I would seriously recommend you learn some fundamentals of PHP before you go any further. You seem to be missing a lot of key concepts.
Sorry if I seem harsh.
-
Since the hash is created by MD5($salt . $password), that is how you must compare it.
So when a user goes to log in, you need to re-hash the plaintext password the same way and then compare it to the one in the database.
So when they login, have something like this:
$username = $_POST['username']; $password = $_POST['password']; $query = mysql_query("SELECT username, password, salt FROM users WHERE username='" . $username . "'"); if (mysql_num_rows($query) == 1) { $row = mysql_fetch_assoc($query); // hash password using the salt from the database and the plaintext password $hash = md5($row['salt'] . $password); // now compare this hash with the one in the database if ($hash == $row['password']) { // user is logged in } }
A couple things though:
1. Hash the salt - it's not very secure only being numbers.
2. Use mt_rand() instead of rand() - it is more random. Also, pass "true" as a second parameter to uniqid() as it provides more entropy.
3. NEVER EVER store a password in a cookie. There is never a case where this is necessary, and it is just very bad practice. Even if it's hashed - it just doesn't need to be there. And that goes for SESSIONs too.
-
Can we see the rest of the code?
-
It works for me.
Did you check the cookie is being set properly?
-
So this is what I have done:
$salt = "agfuihag4jdhf7"; $_POST['pass'] = md5($salt.$_POST['pass']); if (!get_magic_quotes_gpc()) { $_POST['pass'] = addslashes($salt.$_POST['pass']); $_POST['username'] = addslashes($_POST['username']); }
Should this make it more secure?
No. If you have the same salt for everyone, then you still have the same hashes and thus a rainbow table is still effective.
The salt needs to be random and unique for each user.
Using salts with MD5 will make it more secure than not using salts, but MD5 is still a bad choice.
-
While I use prepared statements myself, there's not much effort added to make standard query calls secure. For basic queries, prepared statements require more code.
I agree that the standard mysqli prepared syntax is pretty annoying and requires a lot more code. However, I have a mysqli wrapper library that I always use for small projects which make most queries only a couple lines.
-
The reason MD5 (and SHA1) are insecure is because they are very fast hashing algorithms. They are not made for storing passwords. They are made for ensuring the integrity of data. MD5 and SHA1 hashes can be calculated insanely fast, meaning you can calculate every possible hash (therefore password) in a matter of minutes (depending on your computer hardware).
So what you need is a slower hashing algorithm coupled with some salts. A salt is a unique random string appended to the password so that you can not use rainbow tables. A rainbow table is a pre-compiled list of all possible hashes which are compared against your database. If you don't use salts, then the hashes of two (or more) people with the same password (which is pretty common - most people use stupid passwords) will be identical. What this means is that if your database is compromised and attackers get hold of your password hashes, users passwords may get found. This is because that since they have a huge list of hashes, all they have to do is hash all possible permutations and then match them to the table. So if "password"'s hash is "abcdef", and 5 people have the hash "abcdef", then they will know 5 people have the password "password".
So anyway, here is a decent function for most small-medium scale projects.
function hash_password($password, $salt = null) { // create a salt if not already defined if (is_null($salt)) $salt = substr(sha1(uniqid(mt_rand(), true), 0, 10); // $password will be plaintext at this point // $site_key should be a large random string statically // located in a file with secure permissions $hash = hash_hmac('sha512', $password . $salt, $site_key); return array('hash' => $hash, 'salt' => $salt); } $password = 'abcdef'; $pass = hash_password($password);
When users first sign up a unique salt will be generated and appended to the plaintext password. The hashed password and salt will be returned by the function, so store them in separate database columns. When a user then logs in, you will get the user by username (or whatever) from the database, and send the plaintext password as well as the salt stored in the database to the function. You will get a hash back, compare this hash with the hashed version stored in the database and if they match they are good to go.
This function is slow, so brute forcing will take a lot longer. Also, by using salts, rainbow tables have to be generated for every user - which basically makes them unfeasible.
Hope this helps.
-
This error is because the query failed. If you did
$get_order = mysql_query('SELECT * FROM ordersitimised WHERE orderid=$currentorderid') or die (mysql_error());
You would see that you have invalid SQL.
The reason you have invalid SQL is because you used single quotes and didn't concatenate. In PHP, single quotes mean "exactly this". So if you say 'WHERE orderid=$currentorderid', the SQL will look exactly like that - it doesn't get the value of $currentorderid. So you can either concatenate like this: 'WHERE orderid=' . $currentorderid or you can just use double quotes.
So this:
$get_order = mysql_query('SELECT * FROM ordersitimised WHERE orderid=' . $currentorderid);
Or this:
$get_order = mysql_query("SELECT * FROM ordersitimised WHERE orderid=$currentorderid");
Also, since you are new and learning, this is a good time to stop learning with the mysql functions. Mysql is old, outdated, slow, and insecure. You should be using mysqli functions instead.
http://php.net/manual/en/book.mysqli.php
You can use it almost the same as mysql functions, but it allows you to use prepared statements which means you won't be susceptible to SQL injection attacks.
Here's a quick snippet to select from a database using mysqli:
$conn = mysqli_connect('localhost', 'root', 'root', 'database'); $id = 1; $query = mysqli_query($conn, "SELECT * FROM table WHERE id=$id"); if (mysqli_num_rows($query) > 0) { while ($row = mysqli_fetch_assoc($query)) { echo '<pre>' . print_r($row, true) . '</pre>'; } }
Note that this snippet doesn't use mysqli's prepared statements... so I recommend you go read on that subject for a bit. It's honestly not even worth learning to use regular mysql functions. You will never use them in any respectable program, and they take way too much effort to make it secure.
-
Something like this should work:
$curl = curl_init('http://example.com'); curl_setopt($curl, CURLOPT_NOBODY, true); curl_exec($curl); $http_status = curl_getinfo($curl, CURLINFO_HTTP_CODE); curl_close($curl); if ($http_status == 200) { // good to go }
Framework or not to Framework?
in Frameworks
Posted
Sounds like a framework is a good choice for you. A good framework will take most of the work out of your project.
Check out CodeIgniter, it is pretty beginner friendly and has awesome documentation.