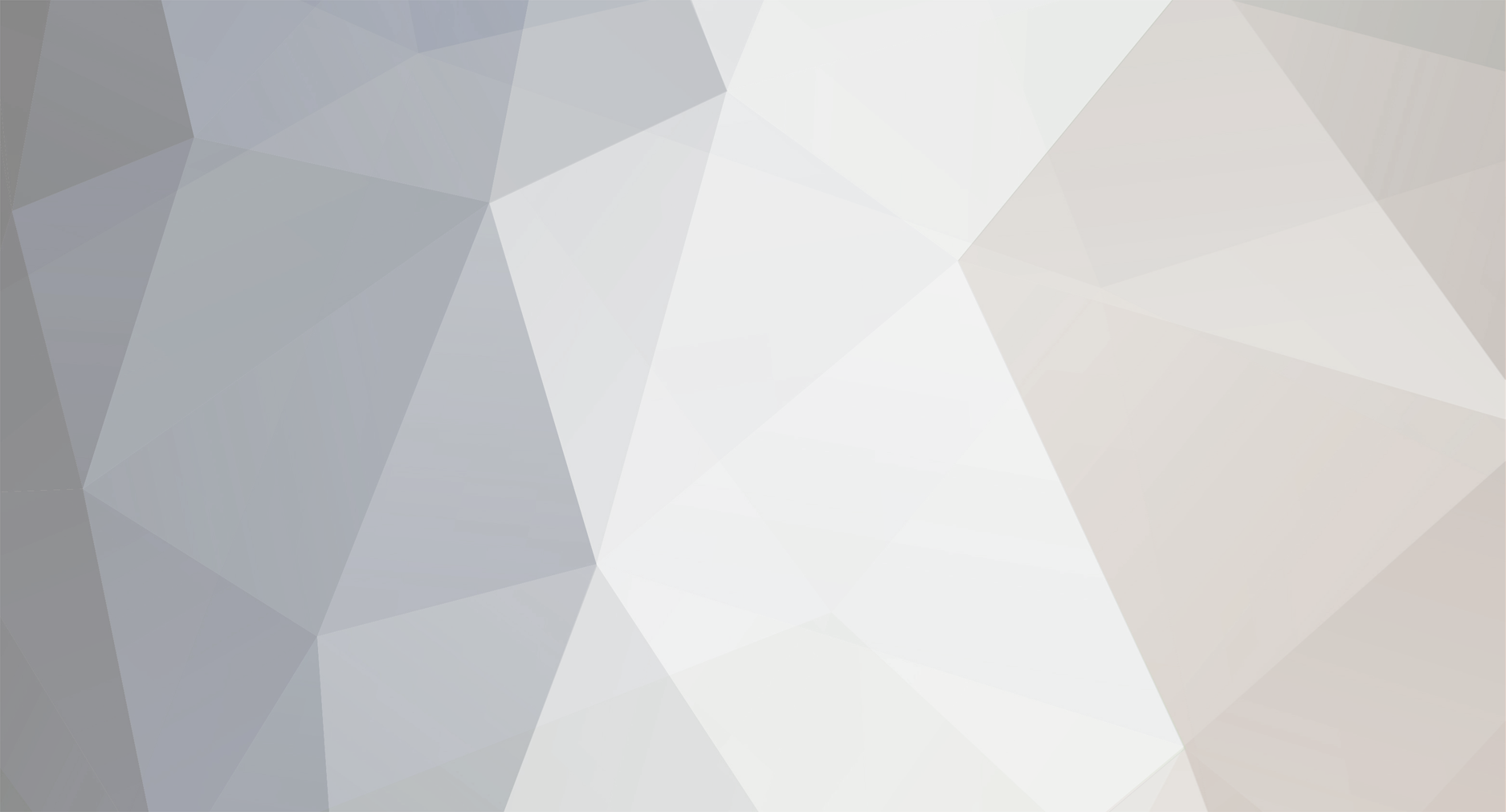
scootstah
-
Posts
3,858 -
Joined
-
Last visited
-
Days Won
29
Posts posted by scootstah
-
-
Are you calling session_start() in connect.php?
-
Okay so I have got my login script to work after two days of me wondering why it was not working, only to find out that it was because the fields in my database were not big enough to hold the password or the salt. So I am now wondering how I can check if people are logged in without using cookies? Currently I have it so if the username and password in the cookie match the username and password in the database, then they are showed the data, but how can I do it without a cookie for a password? Would the idea I put in the last post be sufficient?
You need to create an auto login key and store it in the database. The key needs to be random and unique to the user. Store this key in a cookie, and then use it to look up the user table and log them in. In this scenario you ignore the username/password scheme.
The advantage to this is that even if the cookie is compromised and an attacker is able to log in as that user, they wouldn't have potentially compromised that user's password. Since a lot of people use the same password on multiple websites, this would mean that that user's accounts on other websites are still safe.
Additional security would be to match the user-agent of the request with the one associated with that auto login key. You could also check for IP matches, but some people's IP address changes frequently.
-
You need to add a header to the createchart.php file.
header('Content-Disposition: attachment; filename=image.png');
Change "image.png" to whatever you want it to be.
-
This is the problem:
list ($check, $data) = check_login($dbc, $_POST['user_name'], $_POST['user_pass']); if ($check) { // OK! // Set the session data:. session_start();
You called session_start() AFTER you set session data. session_start() should always be at the top of a PHP file.
-
So you need to use a JOIN then.
$sqlCommand = "SELECT listing_id, listing_title, listing_date, showing, categories.cat_id, categories.cat_name FROM listings JOIN categories ON categories.cat_id = listings.cat_id WHERE showing='0' AND user_id='$user_id' ORDER BY listing_id ASC";
Then you can just do
while ($row = mysql_fetch_array($query)) { echo $row['cat_name']; }
-
You should spend a day or two and read the PHP manual. For the most part, it has very good documentation for all of PHP.
http://www.php.net/manual/en/language.operators.assignment.php Maybe this will help you understand.
When you use the assignment operator "=" only, the value to the right overwrites the previous value in a variable.
$var = 'foo'; $var = 'bar'; // $var is now "bar", "foo was overwritten"
Now if you wanted to append "bar" to "foo", you would use the assignment operator ".=".
$var = 'foo'; $var .= 'bar'; // $var is "foobar"
This is the utmost basic fundamentals of programming. I'm not sure what book you are reading, but it may be more of an advanced book rather than a beginner book that doesn't spend much time on these sorts of things. Because they are very easy and with a proper book you should be able to pick them up right away.
My advice is to learn all of the (or most of the) PHP operators: what they do, how to use them, when to use X over Y, etc. The PHP manual has a wealth of knowledge, and you can find more by Googleing if you need to. Also, don't be afraid to make a .php file and just try stuff out. In my opinion, that is the best way to learn. Once you know a handful of the operators just try stuff out and see if it works in the way you think it should work. If not, look it up again and figure out what went wrong.
-
Here's some information: http://www.faqs.org/faqs/jpeg-faq/part1/section-11.html
It's sort of an old thing, I'm not sure it's really used anymore. Though I could be wrong.
-
Off the top of my head:
Array ( [0] => Array ( [display] => Username [params] => Array ( [type] => text [name] => username [id] => username ) ) )
-
I am using mysquli... sorry...
Ahh, my mistake. Good then, you are already halfway there.
I do have a question though I see people use -> in their code all the time, what does that actually do or mean?
The "->" is called the object operator. It is used in OOP (object orientated programing). I'm probably not going to explain this very well, so I apologize for that. However I'm sure you could Google better explanations of OOP programming.
Basically, this is how it is used.
class MyClass { public $a_variable = 'im a variable'; public function doSomething() { echo 'im doing something'; } } $MyClass = new MyClass; // instantiate the class // now MyClass is an object. You can see this by doing: // var_dump($MyClass); // So to use the object we use the object operator, -> // If we want to use the doSomething() method, we do this $MyClass->doSomething(); // basically this says "use the doSomething method (function) inside the MyClass object // We can also access (public) variables inside the class echo $MyClass->a_variable;
So in other words you are talking about sanitizing upon posting?
Yes, by output I mean sanitize while posting. HOWEVER, while that may be the case for sanitizing HTML - which is only an issue on output (when people view it) - sanitizing after input may not always be the right thing to do. It depends entirely on what you are doing with your data, and what the data is.
EDIT: Wait, no. Posting would refer to input. I mean sanitize on OUTUT which would be when the user views the page and the data is retrieved from the database.
-
Sanitizing is generally inputs-only because one would assume you'd only want to store and/or display clean/valid/verified data. There's nothing stopping you from using sanitizing functions in other instances, it's just an ass backwards way of doing things.
This is not always the case. You should sanitize input so that it is the expected format and safe to store. So, knock off SQL injections and validate UTF8 and that's all you really need.
Then sanitize HTML and such at output. In this way, your data is more flexible. You can use it in different ways. If, in 6 months, you decide you actually did want to allow HTML then all you need to do is modify your outgoing sanitation filters.
Of course this isn't necessarily always the best way to go about it. For example, if you have a ton of content being outputted then sanitation on every page load may slow things down a bit (of course caching is usually an option too).
At any rate, there is no end-all, be-all solution for sanitation. It is absolutely situational.
Ok, so what I'm asking is if sanitation is situational, is there a way to define what type of sanitation works where? As I've said before at the beginning everyone seems to sanitize differently for the same type of code.
Posting, $='1', etc...
There has to be some place beyond http://php.net/, that shows in real world situations how to sanitize... in plain English.
Or is this just stuff that people take for granted and say ok, I put that there, and hope it does what it's meant to... to me this stuff is like salt... I really don't understand why the code works, but being told to put it in there and know that it works really isn't great way of knowing how things work...
Read my edit on that post.
Basically, you sanitize for expected input. When you take input from someone, you always assume it is going to be used maliciously. You never think "oh, someone won't think to do that!". You always think "well that guy is shady, he's going to hack my database" and then you take paranoid steps to prevent it.
You always check that your input is the way you expected it to be. Generally sanitation refers to making input safe. So if you were to sanitize for SQL injections, you would escape the data. If you were sanitizing for XSS attacks, you would remove all HTML. There's lots of solutions for specific sanitation methods such as this, but you just have to know where to use what based on what you want the input to be.
How do you remove the HTML? That seems counterproductive....
By remove the HTML, I mean the actual tags. So that if users are allowed to insert data that is then read by other users, they can't use HTML maliciously (or annoyingly). For example if they post a comment and in the comment they write "<script type="text/javascript">alert('muahaha im malicious');</script>" then anyone who reads this comment is going to get an alert box. While this example is relatively harmless, there are many worse things that can happen.
So to sanitize for HTML you can either convert to entities (so things like < become <, which don't actually parse as HTML). You can do that with either PHP's htmlentities or htmlspecialchars functions. The input would then be this:
<script type="text/javascript">alert('muahaha im malicious');</script>
and thus anyone reading it would literally see "<script type="text/javascript">alert('muahaha im malicious');</script>" and it wouldn't execute as code.
Now, generally I prefer to sanitize HTML on output. This way if I ever change my mind about the data, or if there are multiple output formats, I don't have to worry. To do this you just use the above method but on output instead of input.
-
It should be noted that if you want to completely avoid this problem all together, you should be using prepared statements. You can do this either using mysqli or PDO. I won't confuse you with the details, but basically you don't even need to sanitize against SQL injection because queries are made safe internally. I would recommend you read up on mysqli functions, they are far better than standard mysql.
Hope this helped.
I guess what I am getting stuck at is what are prepared statements, and how do I use them to sanitize correctly.
People show me php.net which is fine, but it really doesn't explain to me how it works because I don't understand what they are really doing...
Okay, this is an example of inserting data with a prepared statement.
<?php $mysqli = new mysqli('localhost', 'root', 'root', 'database'); $username = $_POST['username']; $password = $_POST['password']; $query = "INSERT INTO users (username, password) VALUES (?, ?)"; $stmt = $mysql->prepare($query); $stmt->bind_param('ss', $username, $password); $stmt->execute(); $stmt->close();
So any variable data that needs to be put in the query is replaced by ?'s. Then later, we will bind values to these placeholders. PHP does this safely and internally, so you don't even need to worry about SQL injection.
If you aren't comfortable using OOP code, the entire mysqli library is also procedural (although I find the syntax a little uglier).
-
Sanitizing is generally inputs-only because one would assume you'd only want to store and/or display clean/valid/verified data. There's nothing stopping you from using sanitizing functions in other instances, it's just an ass backwards way of doing things.
This is not always the case. You should sanitize input so that it is the expected format and safe to store. So, knock off SQL injections and validate UTF8 and that's all you really need.
Then sanitize HTML and such at output. In this way, your data is more flexible. You can use it in different ways. If, in 6 months, you decide you actually did want to allow HTML then all you need to do is modify your outgoing sanitation filters.
Of course this isn't necessarily always the best way to go about it. For example, if you have a ton of content being outputted then sanitation on every page load may slow things down a bit (of course caching is usually an option too).
At any rate, there is no end-all, be-all solution for sanitation. It is absolutely situational.
Ok, so what I'm asking is if sanitation is situational, is there a way to define what type of sanitation works where? As I've said before at the beginning everyone seems to sanitize differently for the same type of code.
Posting, $='1', etc...
There has to be some place beyond http://php.net/, that shows in real world situations how to sanitize... in plain English.
Or is this just stuff that people take for granted and say ok, I put that there, and hope it does what it's meant to... to me this stuff is like salt... I really don't understand why the code works, but being told to put it in there and know that it works really isn't great way of knowing how things work...
Read my edit on that post.
Basically, you sanitize for expected input. When you take input from someone, you always assume it is going to be used maliciously. You never think "oh, someone won't think to do that!". You always think "well that guy is shady, he's going to hack my database" and then you take paranoid steps to prevent it.
You always check that your input is the way you expected it to be. Generally sanitation refers to making input safe. So if you were to sanitize for SQL injections, you would escape the data. If you were sanitizing for XSS attacks, you would remove all HTML. There's lots of solutions for specific sanitation methods such as this, but you just have to know where to use what based on what you want the input to be.
-
Sanitizing is generally inputs-only because one would assume you'd only want to store and/or display clean/valid/verified data. There's nothing stopping you from using sanitizing functions in other instances, it's just an ass backwards way of doing things.
This is not always the case. You should sanitize input so that it is the expected format and safe to store. So, knock off SQL injections and validate UTF8 and that's all you really need.
Then sanitize HTML and such at output. In this way, your data is more flexible. You can use it in different ways. If, in 6 months, you decide you actually did want to allow HTML then all you need to do is modify your outgoing sanitation filters.
Of course this isn't necessarily always the best way to go about it. For example, if you have a ton of content being outputted then sanitation on every page load may slow things down a bit (of course caching is usually an option too).
At any rate, there is no end-all, be-all solution for sanitation. It is absolutely situational.
EDIT:
Matt you don't have to change anything to insert the backslashes.
PHP does that for you and that is what we mean by escaping it.
It disarms the potentially dangerous SQL Code that can be abused to enter your DB.
SQL injection is how hackers get into a lot of sites so it's important to protect yourself.
But you need to add the slashes into the code somewhere to make php utilize them correct?
The slashes are to prevent SQL injection. Let's say your SELECT query looks like this:
SELECT * FROM users WHERE username=$username AND password=$password
Now, let's say $username and $password just came directly from a POST form. So, they may contain ANYTHING that a user might have put in them - having not sanitized them, we really have no idea so we are trusting.
Now let's say that $username actually contains this value: "bob';--" (in MySQL, -- is a comment - so anything after it is dropped from the query in this example)
So now when we execute the query, this is what it actually executes:
SELECT * FROM users WHERE username='bob';--
Now anyone can login as "bob" without a password (substitute "bob" with an admin and you should be able to see why this is problematic).
So to combat this we can escape the input. When the input is escaped, special characters (like quotes) will act as literally that character instead of part of the code. So if we escape our $username and $password with mysql_real_escape_string(), then our actual executing query would then look like this:
SELECT * FROM users WHERE username='bob\';--' AND password='hispassword'
Now, the username is just "bob\';--" and is completely harmless, since it wasn't allowed to alter the actual query.
It should be noted that if you want to completely avoid this problem all together, you should be using prepared statements. You can do this either using mysqli or PDO. I won't confuse you with the details, but basically you don't even need to sanitize against SQL injection because queries are made safe internally. I would recommend you read up on mysqli functions, they are far better than standard mysql.
Hope this helped.
-
Something like this:
$maxColumns = 4; $i = 1; while($tir = mysql_fetch_array($tiquery)) { if ($i == ($maxColumns + 1)) { echo '<br />'; $i = 1; } // echo image $i++ }
-
Make the price field an array and use the product number as the index.
<input type="text" value="'.number_format($price, 2, '.', '').'" name="price[' . $productnumber . ']" size="10" maxlength="10">
$product_no = $_GET['product_no']; $price = $_GET['price']; // loop through checkboxes foreach($product_no as $p) { if (isset($price[$p])) { echo $p . ' - ' . $price[$p] . '<br />'; } }
-
This has nothing to do with PHP. You'd do this with HTML and CSS. Take a look at the CSS word-wrap property.
PHP also has a wordwrap function, you know.
I suppose. HTML/CSS just seems better suited to handle this.
-
This has nothing to do with PHP. You'd do this with HTML and CSS. Take a look at the CSS word-wrap property.
-
You'd need Javascript to do this.
-
This will fix the undefined index
$where = ''; if (isset($_POST['price']) && !empty($_POST['price'])) { $price = $_POST['price']; foreach($price as $p) { if (preg_match('/[0-9]\-[0-9]+/', $p)) { $p = explode('-', $p); $where .= 'price BETWEEN ' . $p[0] . ' AND ' . $p[1] . ' OR '; } else if (preg_match('/[0-9]+\+/', $p) { $where .= 'price >= ' . str_replace('+', '', $p) . ' OR '; } } } if (!empty($where)) $where = 'WHERE ' . trim($where, ' OR '); $query = mysql_query("SELECT price FROM package " . $where);
The screen should be blank. This doesn't output anything, it's just a select query. You need to finish it, like do the stuff in your original post after this line:
$q ="SELECT s.supplier, p.package, p.pack_info, FORMAT(p.price,2) FROM supplier AS s INNER JOIN package AS p USING (sup_id) " . $where . " ORDER BY $order_by LIMIT $start, $display";
-
Okay maybe try something like this:
<form action="myscript.php" method="post"> <input type="checkbox" name="price[]" value="0-500">Price 0-£500 </font><br> <input type="checkbox" name="price[]" value="500-1000"> Price £500-£1000</font><br> <input type="checkbox" name="price[]" value="2000+"> Price £2000+</font><br></form>
$price = $_POST['price']; $where = ''; foreach($price as $p) { if (preg_match('/[0-9]\-[0-9]+/', $p)) { $p = explode('-', $p); $where .= 'price BETWEEN ' . $p[0] . ' AND ' . $p[1] . ' OR '; } else if (preg_match('/[0-9]+\+/', $p) { $where .= 'price >= ' . str_replace('+', '', $p) . ' OR '; } } if (!empty($where)) $where = 'WHERE ' . trim($where, ' OR '); $query = mysql_query("SELECT price FROM package " . $where);
-
Wow, disregard that post. I need to stop theory-developing before the coffee hits. o.O
Let me think of something better.
-
You could use a multidimensional array.
$matches = array(array('match' => $match1, 'result' => $matchresult1), array('match' => $match2, 'result' => $matchresult2), array('match' => $match3, 'result' => $matchresult3), array('match' => $match4, 'result' => $matchresult4) ); if($getawayr='Group A'){ $numWins = 0; for($i=0; $i < count($matches); $i++){ if ($matches[$i]['match'] == 'W' && $numWins < 3) { $matches[$i]['result'] = 'Qualified for Semi Finals'; $numWins++; } } }
-
You'll want to rename your checkbox to something more specific, like price[]. Because if you have more checkboxes that you want to group like this you will need a different name.
Something like this maybe?
$price = $_POST['price']; $range = array(); foreach($price as $p) { $range = array_merge($range, $p); } $range = array_unique($range); $x = $range[0]; $z = $range[count($range) -1]; // now $x is equal to the first number (0) // and $z is equal to the last number (1000) // now you can use MYSQLs BETWEEN operator
-
Well in my example, every page has
if ($_SESSION['logged_in'] === true) { }
to determine if the user is logged in. So, obviously it won't magically become true, so you have to assign it to true when you authenticate the user.
// username is good // password is good // blah blah // user is authenticated $_SESSION['logged_in'] = true;
Show a category using an array
in PHP Coding Help
Posted
That's correct. You can also use aliases on the tables so you don't have to type the whole table name out every time.