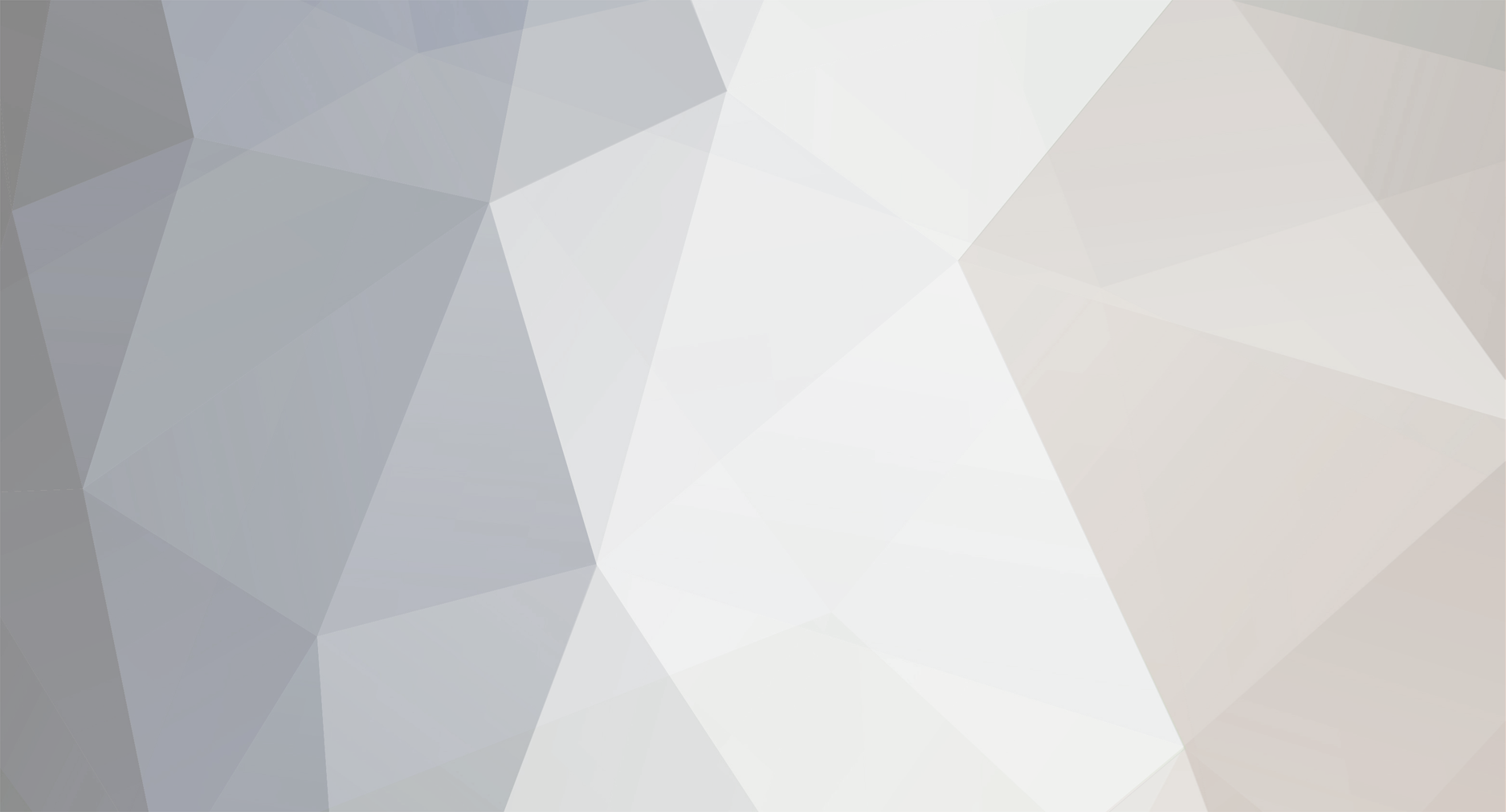
rythemton
-
Posts
107 -
Joined
-
Last visited
Posts posted by rythemton
-
-
You may not be getting any responses because as far as I can tell, it should be working. What do you get if you 'print_r( $dns )' before the second foreach loop? What do you get if you remove the sort?
-
When I need to be able to change the order, I just use the primary ID as a default sorting, that way the value for order can be the same without causing issues:
$queryorder = 'ORDER BY order ASC, id DESC'; // this defaults to new items first compared to other new items $queryorder = 'ORDER BY order ASC, id ASC'; // this defaults to new items last compared to other new items
This also allows me to use larger numbers for manipulation, in case I want to slip something between two items later on without changing the order value of every item.
Just another option.
-
It is creating a line break. If you view the source, you should see it.
The problem is that HTML ignores line breaks. You'll have to use an HTML break, <br>.
-
We definitely need more code to work with. The problem is most likely somewhere else in the page. Do you have another anchor tag (<a>) that isn't closed?
-
If you're in PHP, you can't go further into php. You'll need to concatenate.
// with the concatenate operator: $sql = "SELECT * FROM mytable WHERE title='" . $title . "' "; // or even shorter (only works in double quotes): $sql = "SELECT * FROM mytable WHERE title='$title' ";
-
In the type list, you have the product name listed as an integer (i) so it's being changed to an integer.
-
This sounds like it's an issue with your hosting.
In all reality, I'd switch it to be all in one domain for SEO friendliness. Google considers each sub-domain as a different domain for the purposes of tracking, so by separating your site into separate sub-domains will affect your placement in searches.
If you don't care about SEO, it sounds like it will take finding someone at your hosting company that knows what they are doing.
-
That error means that your query failed for some reason. If the prepare statement fails, it returns FALSE instead of a MYSQLI_STMT object. Troubleshoot your sql statement.
-
You'll have to add that to the constructor:
class ThisIsMyClass { public $var1 = ''; public $var2 = ''; function __construct( $newvar ) { $this->var1 = $newvar; $this->var2 = 'This is my text being concatinated ' . $this->var1 . ' with other text.'; } }
Hope this sample code helps.
-
I think you are looking for the ternary operator. A Google search found: http://www.tuxradar.com/practicalphp/3/12/4
-
If I had to guess at what is being asked, it seems like he is asking if there is a difference between:
<body onload="myfunction();">
and:
<script type="javascript"> window.onload=myfunction; </script>
I personally haven't experienced any difference. I usually like to use the window.onload method so that I don't have to add anything more than I have to for the HTML code.
-
when I change the code to that:
<body> <script type='text/javascript' src='http://ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js'></script> <script language="javascript" type="text/javascript" src="http://domain.com/r.php?id=154"></script> </body>
the script works fine....
Of course you have to add the first line, your code has jquery in it and won't work unless jquery is loaded by the user. You could include something like this at the beginning of your code:
if (typeof jQuery == 'undefined') { document.write('<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1/jquery.min.js"></scr' + 'ipt>'); }
That will detect if jQuery is already loaded, and load it if it isn't.
-
Your problem is the 'return's. Functions stop once they return something.
The second problem is that you really shouldn't be using globals. Instead:
$t = checkname();
That will capture the return value.
-
It really does come down to personal preference. I usually concatenate out of habit. It's taking enough effort sometimes to remember, should I be using a dot (.) or plus (+) to concatenate (PHP or JavaScript) let alone remember if a can use inline variables. It really comes down to, there is more than one way to do the same thing.
All the following do the same thing, and I've used them all at one time or another depending on mood:
echo 'This is my "text"!'; echo "This is my \"text\"!"; ?>This is my "text"!<?php
-
I made the following, and they work:
function create1array( $iterations ) { if( $iterations <= 1 ) return array( array( 1 ) ); else { $maxarray = maxarray( 99, $iterations - 1 ); foreach( $maxarray AS $key1=>$value1 ) { $cursum = 0; foreach( $value1 AS $value2 ) { $cursum += $value2; } $maxarray[$key1][] = 100 - $cursum; } $onearray = array(); foreach( $maxarray AS $value1 ) { $newarray = array(); foreach( $value1 AS $value2 ) { $newarray[] = $value2 / 100; } $onearray[] = $newarray; } return $onearray; } } function maxarray( $maxvalue, $iterations ) { if( $iterations <= 1 ) { $makearray = array(); for( $i = 1; $i <= $maxvalue; $i++ ) { $makearray[] = array( $i ); } return $makearray; } else { $makearray = array(); $startarray = maxarray( $maxvalue - 1, $iterations -1 ); foreach( $startarray AS $value1 ) { $cursum = 0; foreach( $value1 AS $value2 ) { $cursum += $value2; } for( $i = 1; $i <= $maxvalue - $cursum; $i++ ) { $nextarray = $value1; $nextarray[] = $i; $makearray[] = $nextarray; } } return $makearray; } }
The functions ate memory resources fast - I got out of memory errors when trying more than 3.
Good Luck!
-
If you just want the order to be mixed up, you could use the shuffle function.
-
The dot equals (.=) is a shortcut:
// The following: $something .= $more; // Is the same as the following: $something = $something . $more;
Without the dot, whatever is in the variable will be replaced.
$something="this"; $something = "that"; // now $something is 'that' $something .= "more"; // now $something is 'thatmore'
It depends on what you are trying to do.
-
You could also save yourself some rows of data by only adding rows when the price changes, rather than updating every day. Properly created queries would still pull the same data.
$query = 'SELECT * FROM price_changed WHERE id=25 AND date<="2012-03-22" ORDER BY date DESC LIMIT 1';
That would give you the price on March 22nd, even if the last time the price changed was in January, without having to put a new price in every day.
-
I put this in one of my scripts that I was going to make open source:
<?php $output = strtoupper( trim( isset($_REQUEST['source']) ? $_REQUEST['source'] : "false" ) ); if ( $output == "TRUE" ) { header('Content-type: text/plain'); echo ( file_get_contents( "cool.php" ) ); } else { ?> <html> <head>...
The show_source() function is supposed to do syntax highlighting. I guess it depends on what you are doing.
-
$anarray2 = array(); for( $a = 1; $a <= 97; $a++ ) { for( $b = 1; $a + $b < 100; $b++ ) { for( $c = 1; $a + $b + $c < 100; $c++ ) { $d = 100 - ( $a + $b + $c ); $var_1 = $a / 100; $var_2 = $b / 100; $var_3 = $c / 100; $var_4 = $d / 100; $anarray2[] = array( $var_1, $var_2, $var_3, $var_4 ); } } } print_r( $anarray2 );
$anarray2 = array(); for( $a = 1; $a <= 97; $a++ ) { for( $b = 1; $a + $b <=98 ; $b++ ) { for( $c = 1; $a + $b + $c <= 99; $c++ ) { $d = 100 - ( $a + $b + $c ); $var_1 = $a / 100; $var_2 = $b / 100; $var_3 = $c / 100; $var_4 = $d / 100; $anarray2[] = array( $var_1, $var_2, $var_3, $var_4 ); } } } print_r( $anarray2 );
That's how a 4th variable would look. I would think that a recursive function might be what you need, but I'm not sure how it would be constructed.
-
Right now the code returns:
[0] => Array ( [0] => 0.01 [1] => 0.98 [2] => 0.0099999999999993 ) [1] => Array ( [0] => 0.02 [1] => 0.97 [2] => 0.0099999999999993 ) [2] => Array ( [0] => 0.03 [1] => 0.96 [2] => 0.0099999999999993 )
Those are some strange results. Might have to make them whole numbers and divide:
$anarray = array(); for( $a = 1; $a <= 98; $a++ ) { for( $b = 1; $a + $b < 100; $b++ ) { $c = 100 - ( $a + $b ); $var_1 = $a / 100; $var_2 = $b / 100; $var_3 = $c / 100; $anarray[] = array( $var_1, $var_2, $var_3 ); } } print_r( $anarray );
You can see the results here: http://www.bwdplus.com/test/testloop.php
-
Are you sure there is no other way?
There is almost always more than one way to perform a task. The reason I suggested Cron Jobs is because the use the clock, rather than a timer, to determine when to run a task, allowing for greater consistency.
If you have access enough to the server to allow for scripts to run forever, you could also use the time() function to determine when to act.
$checktime = time(); while( TRUE ) { if( time() > $checktime ) { $checktime += 6; // perform actions } }
-
for( $var_1 = .01; $var_1 <= .98; $var_1 = $var_1 + .01 ) { for( $var_2 = .01; $var_2 + $var_1 < 1; $var_2 = $var_2 + .01 ) { $var_3 = 1 - ( $var_1 + $var_2 ); // do something with the three vars } }
For loops don't always have to increment or decrement by 1.
-
Try using Cron jobs, or some other scheduler. This should work even if your hosting doesn't allow scripts to run continuously, which some do restrict.
how to AUTO_INCREMENT 2 colloums not sure if this php or sql
in PHP Coding Help
Posted