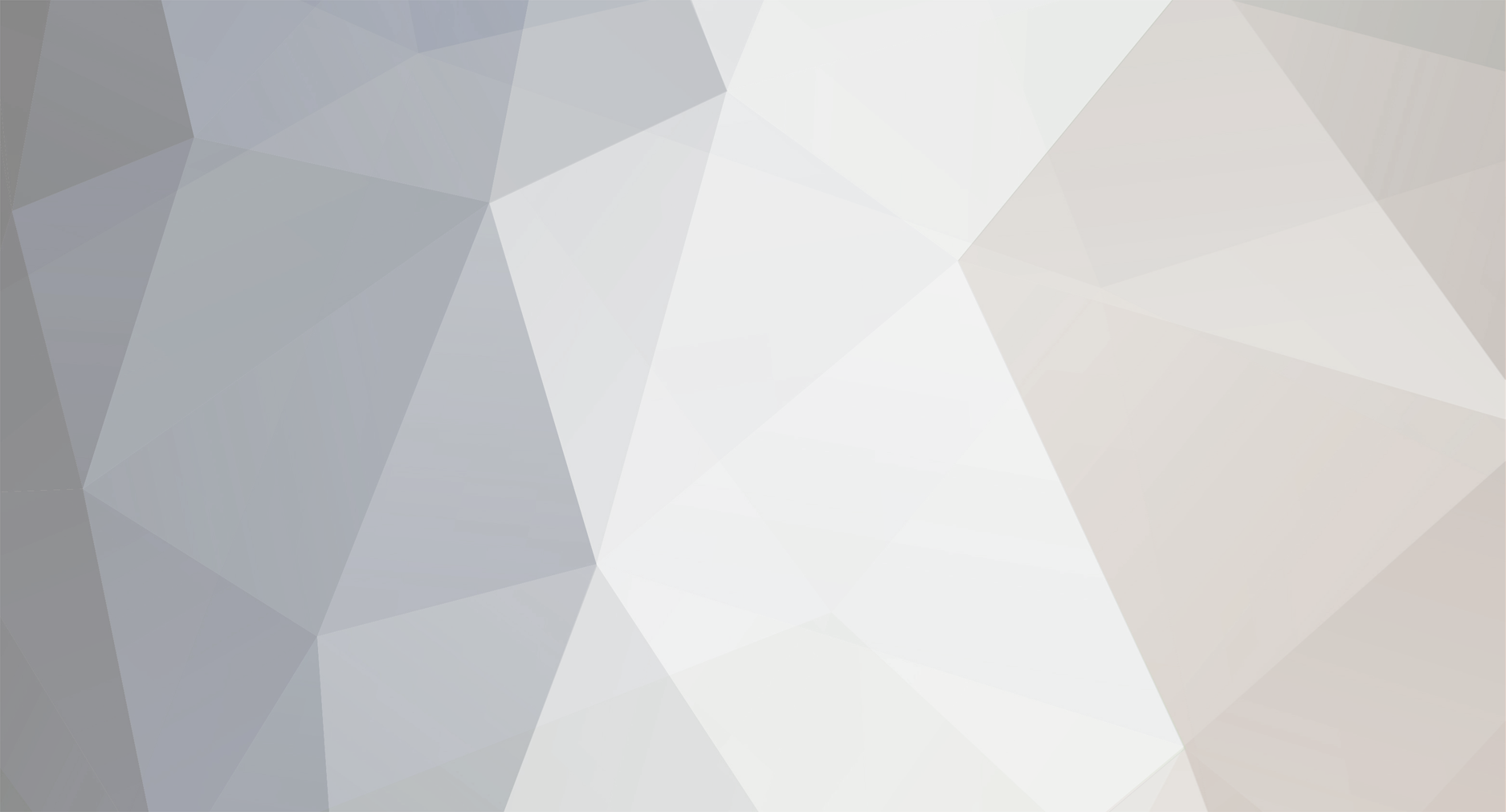
rythemton
-
Posts
107 -
Joined
-
Last visited
Posts posted by rythemton
-
-
Create a function that acts upon 'onsubmit'. This function could cover the page with a full size (width and height 100%) fix position DIV that has the image you want displayed.
I wouldn't use the function to clear or change anything within the form itself, or you might loose the data from the form. Just covering the page would prevent the user from clicking submit again, and would let the user know that something was happening.
-
/* to make sure that "true" (string) isn't the same as TRUE (boolean) */
PHP considers every non-empty string to be TRUE, except "0", when comparing a STRING to a BOOLEAN.
if( "FALSE" == TRUE ) // This will be considered TRUE because the string is not empty. if( "FALSE" === TRUE ) // This will be considered FALSE because one is a STRING and the other a BOOLEAN
-
To understand the difference between != and !==, you need to understand the difference between == and ===. The triple = will verify type as well as value, so it is stricter:
if( "24" == 24 ) // This will return TRUE if( "24" === 24 ) // This will return FALSE because one is an INT, the other a STRING if( 1 == TRUE ) // This will return TRUE if( 1 === TRUE ) // This will return FALSE because one is an INT, the other a BOOLEAN if( FALSE == "" ) // This will return TRUE if( FALSE === "" ) // This will return FALSE because one is a BOOLEAN, the other a STRING
!= is the opposite of ==, so if == is TRUE, then != is FALSE. !== is the opposite of ===, so if === is TRUE, then !== is FALSE.
if( "24" != 24 ) // This will return FALSE if( "24" !== 24 ) // This will return TRUE because one is an INT, the other a STRING if( 1 != TRUE ) // This will return FALSE if( 1 !== TRUE ) // This will return TRUE because one is an INT, the other a BOOLEAN if( FALSE != "" ) // This will return FALSE if( FALSE !== "" ) // This will return TRUE because one is a BOOLEAN, the other a STRING
Sometimes you should use one over the other, and sometimes it don't matter. It will take experience to learn when it matters and when it doesn't.
I hope this helps.
-
Check out my reply to http://www.phpfreaks.com/forums/index.php?topic=353529.0
The code I put in there is long, so I'm not going to post it twice. I created it 2 years ago, but it still works.
-
Here is code I created. Hope it gives you enough information to create one that does what you need:
#!/usr/bin/php -q <?php /* Parse Mail Script created by Jerry S Hamaker Pooled together from multiple sources Created on April 21, 2010 For each email that comes in, this script creates a private gallery, and places all parts of the email into files with details being placed into the database under the new private gallery. It also generates a medium size image (for very large images) and thumbnail for each image that comes in. */ require( "../admin/dbconnect.php" ); // This file connects to the MySQL server $subject = "None"; $from = ""; $galleryid = 0; //parse the email function getbody( $message ) { global $subject, $from, $galleryid; $header = ""; $type = "text/plain"; $encoding = "none"; $boundary = ""; $body = ""; $filename = "NoName"; $lines = explode("\n", $message); $getheader = TRUE; foreach( $lines as $value ) { if( $getheader ) { $header .= $value . "\n"; if (preg_match("/^Subject: (.*)/", $value, $matches)) { $subject = $matches[1]; } if (preg_match("/^From: (.*)/", $value, $matches)) { $from = $matches[1]; } if (preg_match("/^Content-Type: ([^;]+);/", $value, $matches)) { $type = $matches[1]; } if (preg_match('/boundary="([^"]+)"/', $value, $matches)) { $boundary = $matches[1]; } if (preg_match("/^Content-Transfer-Encoding: (.*)/", $value, $matches)) { $encoding = $matches[1]; } if (preg_match('/filename="([^"]+)"/', $value, $matches)) { $filename = $matches[1]; } } else { $body .= $value . "\n"; } if (trim( $value )=="") { // empty line, header section has ended $getheader = FALSE; } } if ( $type == "multipart/mixed" || $type == "multipart/alternative" ) { getmultiparts( $body, $boundary ); } else { if( $encoding == "base64" ) { $body = base64_decode( $body ); } // store and process part $templocation = '../image/file_temp.tmp'; if( $fh = fopen( $templocation, 'w' ) ) { fwrite( $fh, $body ); fclose( $fh ); $origname = $filename; $type1 = $type; $localname = $templocation; $checkimage = getimagesize( $localname ); $imagewidth = ( isset( $checkimage[ 0 ] ) ? $checkimage[ 0 ] : 0 ); $imageheight = ( isset( $checkimage[ 1 ] ) ? $checkimage[ 1 ] : 0 ); $type2 = ( isset( $checkimage[ 'mime' ] ) ? $checkimage[ 'mime' ] : '' ); $mimetype = ( $type1 == $type2 ? $type1 : ( $imagewidth == 0 || $imageheight == 0 ? $type1 : $type2 ) ); $resizableimage = FALSE; if( $mimetype == "image/gif" || $mimetype == "image/jpeg" || $mimetype == "image/png" ) $resizableimage = TRUE; $query = "INSERT INTO images ( height, width, type, gallid,"; $query .= " filename, imagename ) VALUES ( "; $query .= $imageheight . ", " . $imagewidth . ", \"" . mysql_real_escape_string( $mimetype ) . "\", "; $query .= $galleryid . ", \"" . mysql_real_escape_string( htmlize( $origname ) ) . "\", "; $query .= "\"" . mysql_real_escape_string( htmlize( $origname ) ) . "\" )"; if( mysql_query( $query ) && $lastid = mysql_insert_id() ) { $filedestination = '../image/file_' . str_pad( $lastid, 5, '0', STR_PAD_LEFT ) . '.img'; $thumbdestination = '../image/file_' . str_pad( $lastid, 5, '0', STR_PAD_LEFT ) . '.thm'; $meddestination = '../image/file_' . str_pad( $lastid, 5, '0', STR_PAD_LEFT ) . '.med'; if( rename( $localname, $filedestination ) ) { if( $resizableimage ) { $img_src = ( $mimetype == "image/gif" ? imagecreatefromgif( $filedestination ) : ( $mimetype == "image/png" ? imagecreatefrompng( $filedestination ) : imagecreatefromjpeg( $filedestination ) ) ); if( $imagewidth > 600 ) { $medwidth = 600; $medheight = floor( $medwidth * $imageheight / $imagewidth ); $img_dst = imagecreatetruecolor( $medwidth, $medheight ); imagecopyresampled( $img_dst, $img_src, 0, 0, 0, 0, $medwidth, $medheight, $imagewidth, $imageheight ); if( $mimetype == 'image/gif' ) { imagegif( $img_dst, $meddestination ); } elseif( $mimetype == 'image/png' ) { imagepng( $img_dst, $meddestination ); } else { imagejpeg( $img_dst, $meddestination ); } imagedestroy( $img_dst ); } $thumbheight = ( $imageheight > 100 ? 100 : $imageheight ); $thumbwidth = ( $imagewidth > 100 ? 100 : $imagewidth ); if( $imageheight > $imagewidth ) { $thumbwidth = floor( $thumbheight * $imagewidth / $imageheight ); } else { $thumbheight = floor( $thumbwidth * $imageheight / $imagewidth ); } $img_dst = imagecreatetruecolor( $thumbwidth, $thumbheight ); imagecopyresampled( $img_dst, $img_src, 0, 0, 0, 0, $thumbwidth, $thumbheight, $imagewidth, $imageheight ); imagejpeg( $img_dst, $thumbdestination ); } else { $defaultthumb = '../image/default.thm'; if( file_exists( '../image/' . $mimetype . '_default.thm' ) ) { $defaultthumb = '../image/' . $mimetype . '_default.thm'; } copy( $defaultthumb, $thumbdestination ); } } } } // if( file_exists( $templocation ) ) unlink( $templocation ); } } //parse multi-part function getmultiparts( $message, $seperator ) { $lines = explode("\n", $message); $process = ""; $firstsep = TRUE; foreach( $lines as $value ) { if( strpos( $value, $seperator ) === FALSE ) { $process .= $value . "\n"; } else { if( $firstsep ) { $process = ""; $firstsep = FALSE; } else { getbody( $process ); $process = ""; } } } } //get the email $fd = fopen("php://stdin", "r"); $email = ""; while (!feof($fd)) { $email .= fread($fd, 1024); } fclose($fd); $galleryname = date( 'Ymd His' ) . '-Emailed'; $query = 'INSERT INTO gallery ( gallname ) VALUES ( "' . mysql_real_escape_string( $galleryname ) . '" )'; $result = mysql_query( $query ); if( $result && $newgall = mysql_insert_id() ) { $galleryid = $newgall; getbody( $email ); $query = 'UPDATE gallery SET galldesc="' . mysql_real_escape_string( '--== ' . $subject . ' ==-- Uploaded By: ' . $from ); $query .= '" WHERE gallid=' . $galleryid; mysql_query( $query ); } ?>
I know it's long. I hope it helps you with what you are trying to do. This file uses a function to parse the email, if it finds multi-part boundaries, it use another function to split up the parts, and each part is then passed to the parse function. Once the email has been parsed, it updates the gallery name to include the subject of the email and the email address it came from.
-
Got this from http://stackoverflow.com/questions/948532/how-do-you-convert-a-javascript-date-to-utc
var now = new Date(); var now_utc = new Date(now.getUTCFullYear(), now.getUTCMonth(), now.getUTCDate(), now.getUTCHours(), now.getUTCMinutes(), now.getUTCSeconds());
-
-
$a = NULL; // i think $a will not keep anything $b; // it assign to the default value --> i think it must be the empty value
Actually, both is_null( $a ) and is_null( $b ) will return TRUE responses. An unset or uninitialized variable is considered to have a NULL value. Setting a value to be NULL will also return a FALSE with the isset function, even though the value is set. Let's expand the chart:
$a = "";
$b = 0;
$c = NULL;
$d = "0";
unset( $e );
function $a $b $c $d $e
is_numeric false true false true false
is_null false false true false true
is_string true false false true false
empty true true true true true
isset true true false true false
=="" true true true false true
==="" true false false false false
-
It all depends on what you are doing:
$a = "";
$b = 0;
$c = NULL;
$d = "0";
function $a $b $c $d
is_numeric false true false true
is_null false false true false
is_string true false false true
empty true true true true
I've used it for multipurpose functions before:
function multiPurpose( $value=NULL ) { if( is_null( $value ) ) { // this means no value was sent } elseif( is_numeric( $value ) ) { // this means a number was sent } elseif( is_array( $value ) ) { // this means an array was sent } else { // if it got here, it is none of the above, probably text } }
And sometimes it doesn't really matter.
-
In web pages, HTML is the content of the page, CSS defines how the content appears, JavaScript modifies and adds interaction to the page.
-
HTML5/CSS3 is the standard for mobile devices, so you need to learn it, even though it is still not complete.
-
Check out http://kuler.adobe.com - you can compare colors and create color schemes.
-
In PHP the concatenate operator is the period(.) so you'd use $_POST[ 'message1' ] . $_POST[ 'message2' ].
-
I thought you could get the value of selected options by just asking for the value, but I guess not. Your function should work, you can call the function by putting it in the quotes for the onchange.
My codes should work if you replace the 'this.value' to 'this.options[ this.selectedIndex ].value', or if you didn't set any values in the options, you can change the 'value' to 'innerHTML' or 'text'.
-
There should be no conflicts with the 'onsubmit' code.
Try your way and/or my way of referencing the text field. There is more than one way to select DOM members.
Yet another way of selecting the text field, but will only work if the input field is the next field, would be this code:
this.nextSibling.value = this.value;
Don't be afraid to try new things.
-
$fieldName = trim($_POST[$fieldName]);
Can someone help me out? Thanks in advanced!
Your problem is in this line of code. You need to use a variable variable here, which means you need a double $:
$$fieldName = trim($_POST[$fieldName]);
-
I do agree with AyKay47, you really shouldn't use globals. The reason classes have scope in the first place is to avoid cross-contamination with other code. I have dealt with cases, however, where using a global saved hours of reprogramming. Just use them with extreme caution.
Your variable has no problem leaving the case, but is trapped within the scope of the class.
AyKay47, the issue turpentyne is having is changing the value of a variable within a class, and not seeing any changes in the variable outside the class. The issue is thought to be because of using switch/case statements, but the real issue is a scope issue within a class. I was able to guess that classes were being used because of the '$this->' pointers.
-
<select onchange="document.getElementById( 'inputbox' ).value = this.value;"> <!-- Options Here --> </select> <input type="text" id="inputbox" name="inputbox" />
Should work. I haven't tested it, though.
-
If JavaScript did not open the window, then JavaScript cannot close the window in most web browsers. This was implemented as a Security Feature. Nothing you can do about it. If JavaScript did open the window, then your first script should work.
-
Subtract works fine because subtract only works on numbers, so JavaScript turns the values into numbers before subtracting. Unfortunately, Addition works as concatenate as well as addition, so if one variable is text (it may only do this if the first variable is text, but I'm not sure), it will convert both to text and concatenate them. Try using the parseInt() function to force both numbers to be Ints before adding them.
-
Is this switch within a class? If so, you are running into a scope issue. You'll have to mark the variable as Global within the class.
-
Hmm. Since you can use array reference to check a letter, I thought it would work, but apparently it doesn't. This should work:
$string = 'ThisText'; $newstring = ''; foreach( str_split( $string ) AS $letter ) { $newstring .= ( ctype_upper( $letter ) ? ' ' : '' ) . $letter; } $string = trim( $newstring );
-
foreach( $array AS $key=>$value ) { for( $i; $i < $value; $i++ ) { echo 'Class: ' . $key; /* Create form here */ echo '<br><br>'; } echo '<br>'; }
-
Your second loop needs to include the first dimension in the array reference:
for ($k=0; $k<count($array_of_mu[ $j ]); $k=$k+1) {
Enabling PHP short_open_tag
in PHP Installation and Configuration
Posted
This is very good advice. Even now, Google is making changes that will change SEO.
http://news.cnet.com/8301-1023_3-57399425-93/google-plans-to-penalize-overly-optimized-sites/