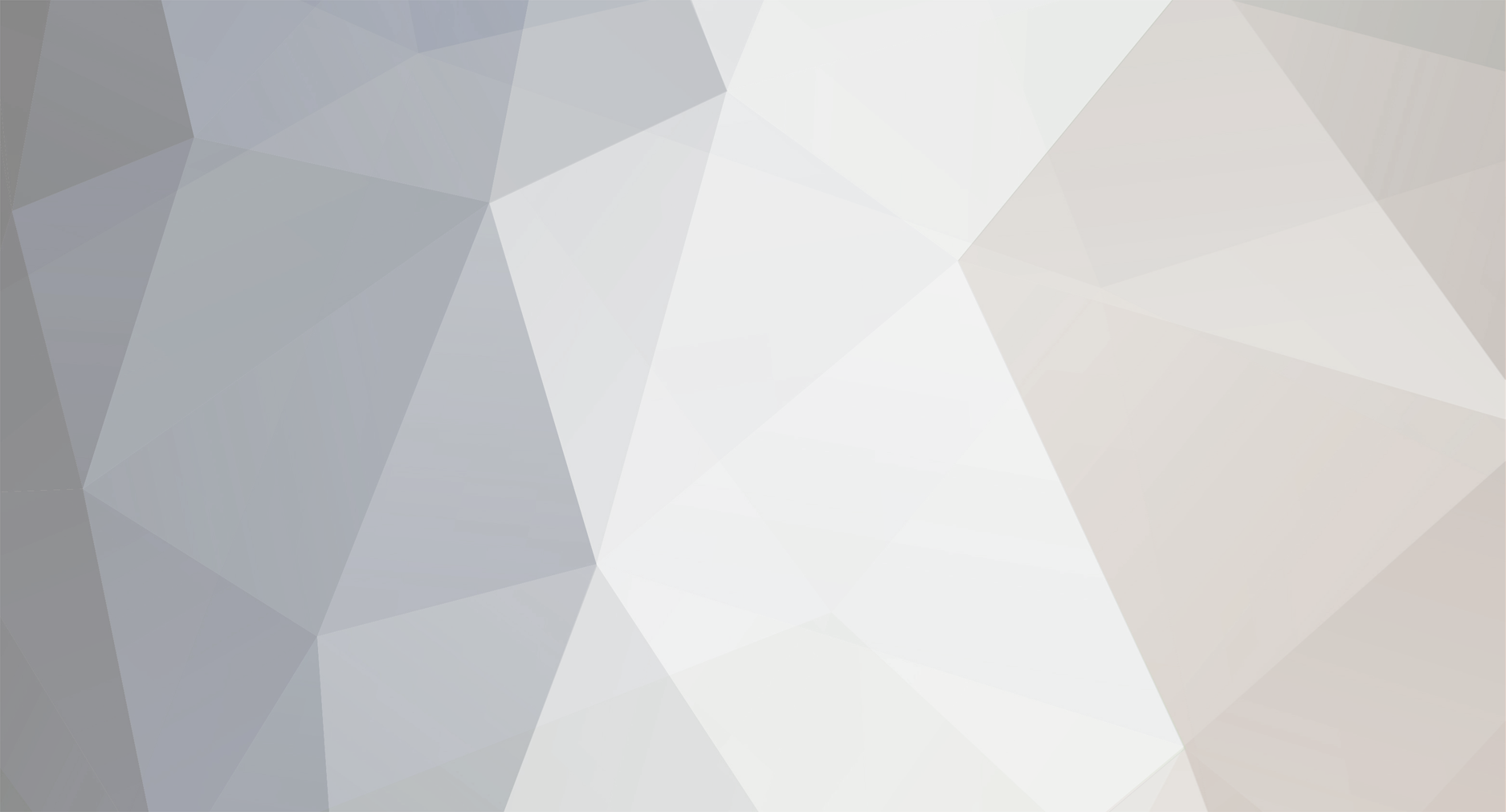
rythemton
-
Posts
107 -
Joined
-
Last visited
Posts posted by rythemton
-
-
Here is code I used to add additional 'Address' fields to a form:
function newaddress() { formvalue = parseInt( document.getElementById( 'additions' ).value ) + 1; formvalue = formvalue.toString(); formdata = document.getElementById( 'fields' ); checkfields = formdata.getElementsByTagName( 'input' ); totalstored = 0; var inputname = new Array(); var inputvalu = new Array(); for( var i=0; i<checkfields.length; i++ ) { var testvalue = checkfields[ i ].value; if( testvalue.length > 0 ) { inputname[ totalstored ] = checkfields[ i ].id; inputvalu[ totalstored ] = testvalue; totalstored++; } } newform = '<fieldset><label for="fname' + formvalue + '">First Name</label><input name="fname'; newform += formvalue + '" id="fname' + formvalue + '" /><label for="lname'; newform += formvalue + '">Last Name</label><input name="lname' + formvalue; newform += '" id="lname' + formvalue + '" /><label for="address' + formvalue; newform += '">Address</label><input name="address' + formvalue + '" id="address'; newform += formvalue + '" /><label for="city' + formvalue + '">City</label><input name="city'; newform += formvalue + '" id="city' + formvalue + '" /><label for="state' + formvalue; newform += '">State</label><input name="state' + formvalue + '" id="state' + formvalue; newform += '" /><label for="zip' + formvalue + '">Zip Code</label><input name="zip'; newform += formvalue + '" id="zip' + formvalue + '" /><label for="phone' + formvalue; newform += '">Phone Number</label><input name="phone' + formvalue + '" id="phone' + formvalue + '" /></fieldset>'; formdata.innerHTML += newform; for( var i=0; i<totalstored; i++ ) { document.getElementById( inputname[ i ] ).value = inputvalu[ i ]; } document.getElementById( 'additions' ).value = formvalue; return false; }
The form field that had the 'additions' Id was a hidden field. I appended numbers on the end of the field names so they had unique names for PHP. I found when I added additional fields, the previous fields reset to their default values, so I added the loops to store, then restore, the field values that were filled in before the additional fields were added. Perhaps you can modify this code to work for you.
-
To include images, there are two methods: included in the email or linked to files on your web server.
Linked to files on your web server is easiest, since you just include the full address to the image on your web server.
Included in the email requires a multi-part content type, and requires encoding the image. The following has some example code:
http://webcheatsheet.com/PHP/send_email_text_html_attachment.php
Good Luck!
-
Is there a way to put the "mysql_real_escape_string" is a loop to cover all the variables, or do they need to be sanitized individually?
I've used variable variables:
$dataentries = array( 'apples', 'donuts' ); foreach( $dataentries AS $value ) { $$value = isset( $_POST[ $value ] ) ? $_POST[ $value ] : ''; $$value = mysql_real_escape_string($$value); }
-
The problem is that you are using the same names over again, so whenever the post is submitted, there is currently no way to distinguish which item is which. As PHP is processing the form, since they have the same name, it overwrites the old value. You need to give them different names and/or use arrays.
<input type="hidden" id="prodId<?php echo $item['id']; ?>" name="prodId[]" value="<?php echo $item['Id']; ?>" /> <input type="text" size="2" name="qty<?php echo $item['id'];" maxlength="2" value="<?php echo $item['Quantity']; ?>" /> <!-- Use the following to get the values: --> <?php foreach( $_POST[ 'prodId' ] AS $product ) { $quantity = $_POST[ 'qty' . $product ]; } ?>
I've use appending the ID to the name successfully. As long as the same product isn't listed multiple times, there shouldn't be any more conflicts.
-
In the Stats table, I wouldn't store a player's lifetime stats. I'd add the Game ID, and store stats for each player for each individual game. You could then add or average stats whenever you need to, and you'd be able to check stats per game, per season, or lifetime as needed.
Make sure to include the game date in the schedules table to help with selecting which games are in a particular season.
In the player's table, I'd include the player's current team id, while in the stats table, I'd include the team id he played on for those stats. That way you can calculate team stats, and if a player changes teams, the stats still point to both him and the team he was playing on for that game, with the added benefit that you could calculate an individual player's stats on a per team basis.
-
foreach($array as $key => $value) { $fields = $key.'='. $value .'&'; } rtrims($felds);
If you copy/pasted directly from your code, you seem to have errors in these two lines of code.
In the first line, the equals (=) should be concatenating (.=), otherwise the variable will only have the last key and value in it. The second line has 'fields' mispelled.
I don't know if that fixes the problem, but it's a place to start.
Split a string - or can you?
in PHP Coding Help
Posted
Since this will put a space in front of every capital letter, I put the trim on the end to remove the space from the beginning.