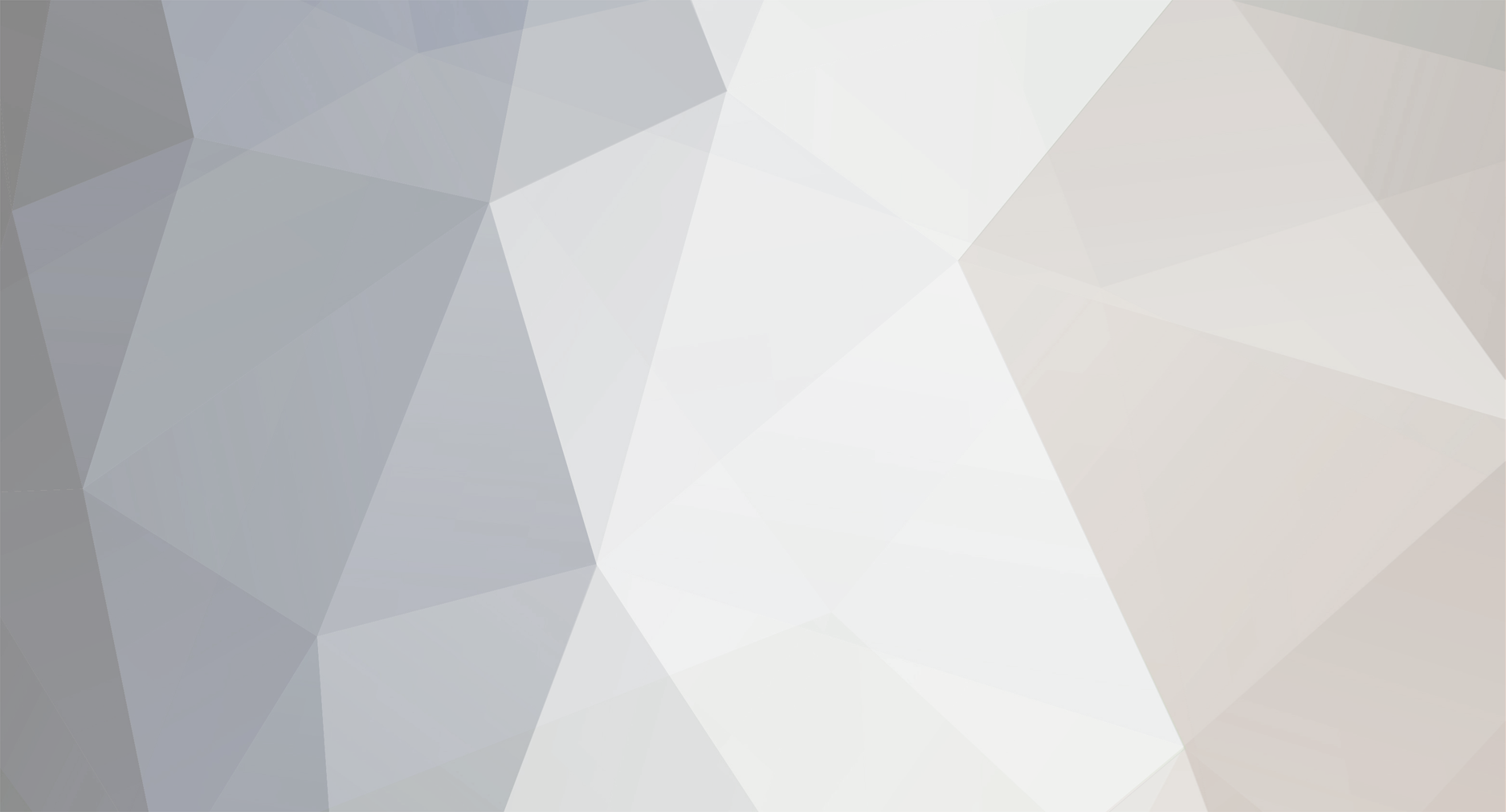
TimeBomb
Members-
Posts
51 -
Joined
-
Last visited
Never
Profile Information
-
Gender
Not Telling
TimeBomb's Achievements

Newbie (1/5)
0
Reputation
-
Is it doable? Of course. Will it have to be more than a simple array function? Probably. Here's some code I roughed together; it could probably use some optimizing. $newArray = array(); foreach($array as $value) { // $value is the nested array $key = $value[1]; // This holds the value #_disabled $disabledInt = intval(preg_replace('(/*_disabled)', '', $key)); // ex. Turns the string 5_disabled to the int 5. $newArray[$disabledInt] = $value; // We'll set the key of the array to the disabled integer. } // Now we can properly sort the array via disabled integer. // Per asort, will be sorted lowest to highest integer. asort($newArray); // This line is optional. // It will slow things down a bit (most array procedural functions are pretty slow), but it will essentially reset the keys to 0 to #, incrementing by one for each array element, as one would normally expect from an array. $newArray = array_values($newArray); The array I used to test with it: $array = array( 0 => array ( 0 => 'test', 1 => '5_disabled', ), 1 => array ( 0 => 'test', 1 => '8_disabled', ), 2 => array ( 0 => 'test', 1 => '2_disabled', ), 3 => array ( 0 => 'test', 1 => '1_disabled', ), 4 => array ( 0 => 'test', 1 => '7_disabled', ), );
-
Ah, this has to do with what makes a GET request, specifically the symbols used in the URI. First off, when we var_dump $_GET with the aforementioned string, we get: array(2) { ["tmp"]=> string(11) "This Thing " ["That_Thing"]=> string(0) "" } The URI I used was /?tmp=This Thing & That Thing. Or, in other words... /?key1=value&key2. The ampersand is a GET variable seperator. The first GET var is designated by the question mark symbol, but all GET variables following that are separated by the ampersand. The Solution An ampersand intended for visual purposes is escaped in a URL as %26.
-
Login System and Adding data into a Database
TimeBomb replied to timmykins02's topic in PHP Coding Help
I see. I found where the if conditional starts. Can you show me where it ends? -
Login System and Adding data into a Database
TimeBomb replied to timmykins02's topic in PHP Coding Help
Hmm. I see a possible issue with this line of code: if (isset($_POST['login'])) { Why don't you tell us what code that if conditional is supposed to contain? -
The very first validation example for JQuery does just as you described. http://docs.jquery.com/Plugins/Validation
-
Well, since you insist... 1) Create a function for filtering SQL data instead of copy pasting the code. 2) Or, better yet, use prepared statements with mysqli or PDO. See: mysqli prepared statements and PDO prepared statements. This will help further secure user input being sent to the database. Also, the PHP mysql library is not recommended for production use anymore. 3) Use the password() function in MySQL to further hash the password. You probably also want to salt the password as well. 4) Use sessions instead of cookies for storing the user's data. Though, for a 'remember me' function, you will need to use a cookie. Just make sure the data is heavily secured before it goes into the cookie.
-
Simplicity is king. It looks nice and works without issue. Nicely done. My only suggestions would be an optional preview option for users and perhaps custom aliases. These are a couple major reasons why I prefer tinyurl.com over most of the competition. /2cents
-
404 error page for database driven pages
TimeBomb replied to rahulvicky00's topic in PHP Coding Help
A 'database driven page' could be/mean a lot of things. I assume that information is being outputted from the database to the user (based on information found in the URL, i.e. via $_GET). You will need to modify the code somewhere along the line. Perhaps either where the data(ex. HTML) is outputted to the user, or where the database checks if information exists. You will want to check for 'bad' information, ex. empty information or an error, and tell it to either redirect to or load(i.e. through include) the error page instead of showing a blank/wrong page. For a more detailed answer, we will need more information. -
In this scenario, I highly suggest you use single quotes around strings instead of double quotes. Then you don't have to escape the double quotes. Why is the function htmlentities in quotes? You do not want to treat it as a string.
-
I came up with the following: function organizeFileArray($array) { $organizedArr = array(); $tmpFunc = function($value, $key, $element) use (&$organizedArr) { if (! isset($organizedArr[$key])) { $organizedArr[$key] = array(); } $organizedArr[$key][$element] = $value; }; foreach (array_keys($array) as $key) { $element = $array[$key]; array_walk($element, $tmpFunc, $key); } return $organizedArr; } It'll produce the same result as your function, but is a lot more dynamic. The downfall is that, after a bit of benchmarking, your function is around 400% faster than mine. So there is indeed room for optimization. I hope it helps, gl. Edit: I just noticed that the function array_walk is completely unnecessary in this scenario. I modified the function to reflect this: function organizeFileArray($array) { $organizedArr = array(); foreach (array_keys($array) as $key) { $element = $array[$key]; $currElement = $key; foreach($element as $key => $value) { if (! isset($organizedArr[$key])) { $organizedArr[$key] = array(); } $organizedArr[$key][$currElement] = $value; } } return $organizedArr; } Your function is only 80% faster than this - much less appalling than 400% faster.
-
I disagree with the underlined statement. The singleton pattern is an antipattern for a reason. They are unnecessary unless your application is designed poorly. If you effectively use dependency injection, you will never need to use singletons.
-
While static classes are gray area in some scenarios, and just plain poor design in others, singletons are very much so antipatterns. I would suggest making it a normal object, and using dependency injection.
-
How do I count values out of a multi-dimensional array
TimeBomb replied to vincej's topic in PHP Coding Help
You could use PHP's array_walk_recursive. $array = array() // sql data in here $types = array('advertisement' => 0, 'friend' => 0, 'other' => 0); function processArray($value, $key) { if ($value == 'bla') { $types['advertisement']++; } elseif ($value == 'blabla') { $types['friend']++; } else { $types['other']++; } } array_walk_recursive($array, 'processArray'); -
But in PHP, procedural functions are used on a regular basis, even in OO applications. Some functions do not have OO alternatives, and thus must be used procedurally. This is because of PHP's history prior to PHP 5, wherein it was not at all object oriented. A lot of it's base still is procedural. Yes, I know. What I'm saying is that user defined functionality should attempt to fit one paradigm or the other. Making your own classes and function libraries to use in the same project is, IMO, not a good way to go. At the very least, to me, it's confusing. And, indeed, this is why static classes exist. They scratch that "I need a bit of generalized utility functionality without an object" itch while still being OO. It's not ideal, but it fills in an edge case like this better than a group of functions. While I will agree that creating a function library that is not directly related to your OO application is not the best practice, I am concerned that forcing simple, non-OO functions into static classes for the purpose of attempting to keep your application completely OO is poorer practice than simply including the functions. While using both procedural code and OO code in an application is somewhat poor design (although arguably less poor in PHP, due to a majority of its 'base' being procedural), and should be avoided at all costs... forcing non-OO code into an OO application is arguably illogical design. It's like forcing a cube into a circular hole.