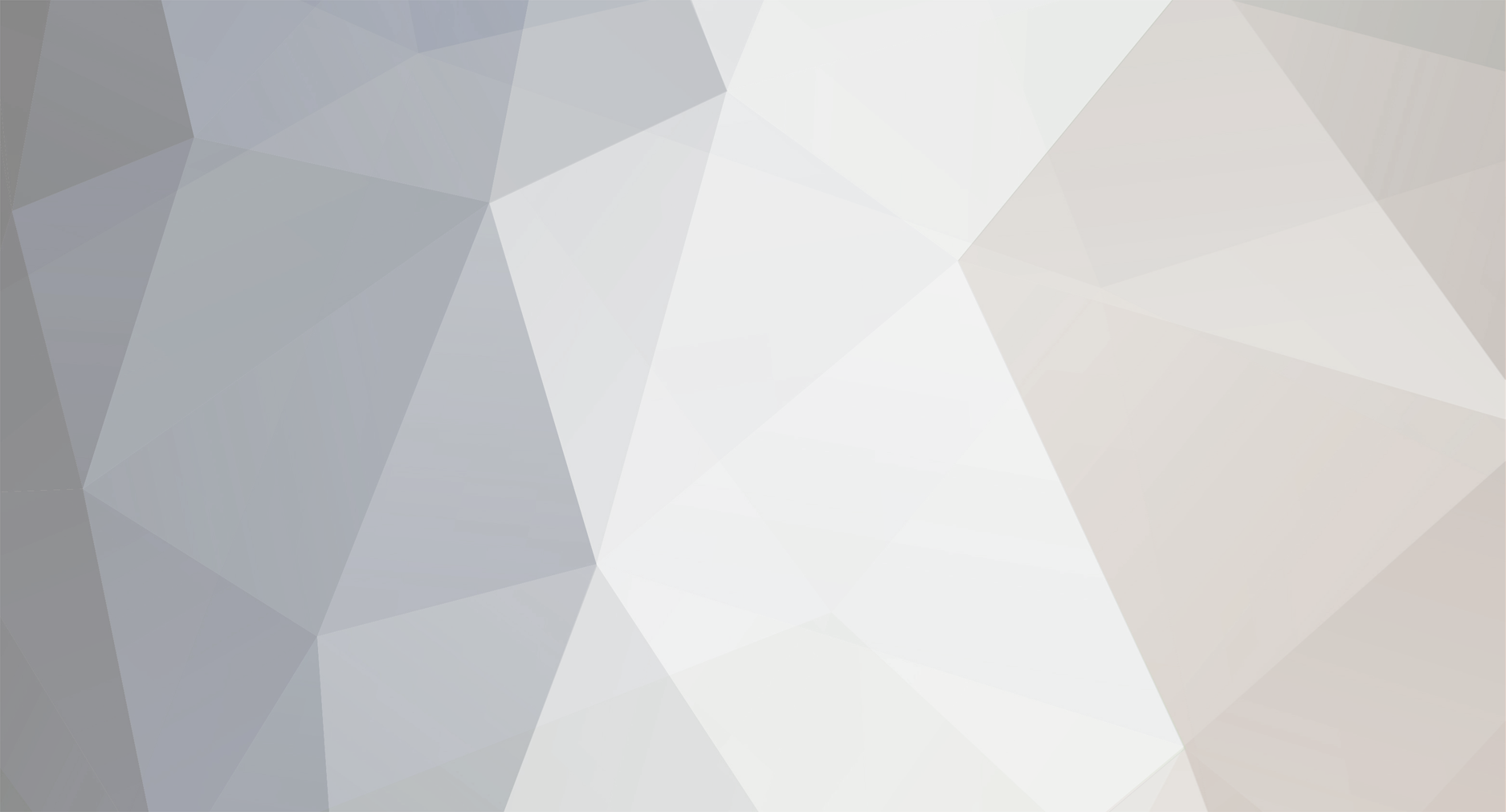
akphidelt2007
Members-
Posts
174 -
Joined
-
Last visited
-
Days Won
2
Everything posted by akphidelt2007
-
I actually had this problem one time and found the answer through google. But, the correct answer is the one above where you should change the data type to an integer field. But if you don't want to you can add an ABS() to the order by column and it should order it as integers... $query1 = "SELECT DISTINCT RMA FROM rmatable where RMA like 'RMAD%' ORDER BY ABS(RMA) DESC";
-
Why do you need a class for this? But, it could look something like this... class randomName { private $vowels = array("a", "e", "o", "u"); private $consonants = array("b", "c", "d", "v", "g", "t"); private function randVowel(){ return $this->vowels[array_rand($this->vowels, 1)]; } private function randConsonant() { return $this->consonants[array_rand($this->consonants, 1)]; } public function generate() { return ucfirst(self::randConsonant().self::randVowel().self::randConsonant().self::randVowel().self::randVowel()); } } $newName = new randomName(); echo $newName->generate();
-
Question about using array_shift with OOP
akphidelt2007 replied to eldan88's topic in PHP Coding Help
You have this question... Why does it take the first element out of the array ?? That is what array_shift does. It takes the first element out of the array. $array = Array(1,2,3,4); $firstEl = array_shift($array); print_r($array); //equals Array(2,3,4) //And $firstEl = 1; For what you are doing you can use isset instead of array_shift... return isset($result_array[0]) ? $result_array[0] : false; This way you retain the $result_array. -
if($action=0) is setting $action to 0 and interpreting the condition as false... which is why it skips that block of code. Use "=="... if($action==0) or in this case you can use "===".
-
You can create a function that returns the select string back to you and all you do is feed the array and array key. Here is an untested example function buildSelect($name,$query,$valueKey,$textKey=false) { //if the value key = the text key then just keep them the same $textKey = $textKey ? $textKey : $valueKey; $html = "<select name='$name'>"; $result = mysql_query($query) or die(mysql_error()); while ($row = mysql_fetch_array($result)) { $html .= "<option value='{$row[$valueKey]}'>{$row[$textKey]}</option>"; } $html .= "</select>"; return $html; } So then for your code you would do something like this... echo "Location:"; echo buildSelect('location',"SELECT Location FROM admin",'Location');
-
You can have multiple submit buttons in one form. Just got to give them a different name or value and check which one was pushed.
-
Just play around with it. Always debug your queries and your request variables... do a lot of print_r($_POST), print_r($_GET) to figure out what the actual values are. And then if you can't figure it out, come back and ask questions.
-
Change echo $query to echo $sql
-
Why do you have two forms?
-
Post what you are doing that is preventing you from echoing out a variable.
-
What I always do when there is no results is to echo out the query to see exactly what you are generating... $query = "SELECT * FROM $db_tb_name WHERE `$db_tb_atr_name` LIKE '%$query%' AND `dbdate1` > '$mydate1' AND `dbdate2` < '$mydate2'"; echo $query; Post what that says.
-
Most like isset($_POST['Member_ID']) isn't getting set. echo $query right before the if(mysql_query($query)){} and see what it says.
-
Well one thing, you forgot the parenthesis ending the mysql_query()... $query_for_result=mysql_query("SELECT * FROM $db_tb_name WHERE `$db_tb_atr_name` LIKE '%$query%' AND `dbdate1` > '$mydate1' AND `dbdate2` < '$mydate2'";
-
You are putting this in the wrong spot... or die("Could Not Formulate the Query"); //try this and see what it tells you $query = "UPDATE Points_Rewards Set Bank = '$bank', Reward_1 = '$reward1', Reward_2 = '$reard2', Reward_3 = '$reward3' WHERE Member_ID = '$memid'"; $result = mysql_query($query) or die(mysql_error());
-
A variable in PHP holding two different values
akphidelt2007 replied to justin7410's topic in PHP Coding Help
You are declaring the variable, then running the query, then re-declaring the variable erasing the previous value. if you did this... it would only take the 2nd $sqlCommand. $sqlCommand = "CREATE TABLE pages ( id INT UNSIGNED AUTO_INCREMENT NOT NULL PRIMARY KEY, page_title VARCHAR(255), page_body TEXT, page_views INT NOT NULL default '0', FULLTEXT (page_title,page_body) ) ENGINE=MyISAM"; // Create the table 2 for storing Blog entries $sqlCommand = "CREATE TABLE blog ( id INT UNSIGNED AUTO_INCREMENT NOT NULL PRIMARY KEY, blog_title VARCHAR(255), blog_body TEXT, blog_views INT NOT NULL default '0', FULLTEXT (blog_title,blog_body) ) ENGINE=MyISAM"; $query = mysql_query($sqlCommand) or die(mysql_error()); -
Without going in to too many details of what you are doing since there are a tons of sites online that describe how to login with hashes and salts. The bottom line is you have to create a string that matches the password string you store in the database. So if you are creating a random salt, then you have to store that salt value in the database along with the user so you can retrieve it when the user logs in. So just like creating the password you would go $plainTextPassword = $_POST['password']; $salt = "Query to get salt from user based on username" $password = crypt($plainTextPassword,'$2y$12$'.$salt); //then you check this password with the password stored in the database.
-
It means $customPosts or $posts is not an array. You have to provide an array even if it's an empty array. If the array is not explicitly set, than before you run the foreach statement... do an if(isset($customPosts)) { foreach() }
-
You are getting the column count error because you have not specified any columns. $query = "INSERT INTO audit_data (column1,column2,column3,etc,etc,etc,etc) VALUES"; The way you have it set up will require a very tedious amount of typing. Not including the fact that you are submitting data that isn't secure. There's way too much to advise on with this. Gonna have to break it down piece by piece to get a hold of what exactly you are doing and make it work.
-
If you know the year... why don't you just do $date = $year.'0101';
-
I don't get what you mean by those variables are "html forms". You mean you are saving the html code of a form to that variable within your script? Or are those just names of forms? Just go through each variable at a time and check to see what value you are getting. Most likely none of them are set.
-
Yes. Just try it yourself. Try to echo out one of those variables and see what you get. Make sure error reporting is turned on
-
Those values have to be explicitly created in the script itself, not from the form. The second the form is submitted you have to create the values. Only the $_POST values are maintained after the form is submitted. So if $query is not echoing than one or a multiple of those values are not set. Unless you skipped most of your code just to show us a short version, those values aren't being created anywhere within the script. Try to echo out $value... guarantee you will get an undefined variable error.
-
Where are these being set? if(isset($value, $iso40, $iso42, $iso50, $iso54, $iso55, $iso56, $iso60, $iso70, $iso73, $iso74, $iso75, $iso76, $iso80, $iso84, $iso85)) {
-
Yes... does "MyPassword" = "3209salksd83220sd98sla320skalk"? The password has to match the text in the database. So whatever method you are salting and hashing your password to create it, you have to do that to the submitted plain text password to create that same string and then compare those two.
-
That's a property in a class. To create something like that you can do it like... //say you have a user class class user { public $username = ''; function user($username) { $this->username = $username; } } $user = new user('YourName'); echo $user->username;