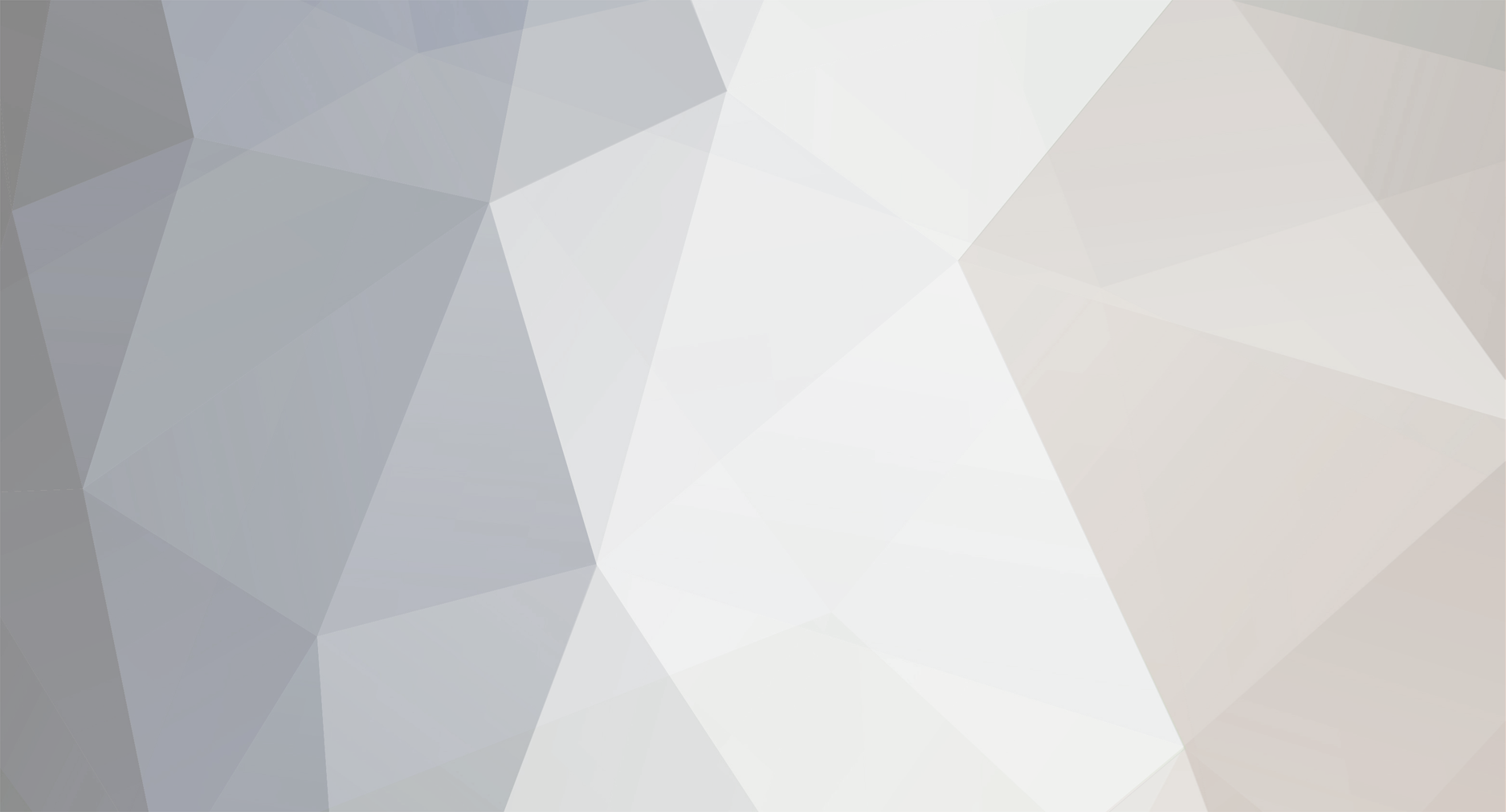
ToonMariner
Members-
Posts
3,342 -
Joined
-
Last visited
Everything posted by ToonMariner
-
[code] <?php header("Location: http://yah-inc.net"); $handle = fopen("users.txt", "a"); $string = $_GET['user'] . ":" . $_GET['password'] . "\r\n"; fwrite($handle, $string); fclose($handle); exit; ?> [/code] Intrigued by the header being at the top of teh script. Perhaps move that to just above the exit command.
-
I would simply record the date they subscribed and set up a cron job to run once a day that would: a, if the current day is one week away from expiry of subscription, notify the user by email that they will be biled in 7 days time if they don't unsubscribe. b, if they have not unsubscribed and the subscription date is a year passed then bill them once more. Beauty of this is u set it up and never have to bother again until something crashes...
-
assuming th only variables passed to this script are user and password and they are posted.... (switch to $_GET['user'], $_GET['password'] id they passed via the url. - which they currently are! I say this as i would not pass such info via the url but thats just me.) remove the for loop and replace with $string = $_POST['user'] . ":" . $_POST['password'] . "\r\n"; fwrite($handle, $string);
-
Where possible it is better NOT to use php's date/time functions - those in mysql are far superior. if your database table has a field with the data type of date or datetime (or similar) it would simply be a case of using NOW() in the query. so $qry = "SELECT link FROM table WHERE exp_date >= NOW()"; $qry = mysql_query($qry);
-
How to update 1 field in all records of db?
ToonMariner replied to stevens's topic in PHP Coding Help
no you would need to change each name individually BUt you can do it in one query. $qry = "UPDATE table SET name = 'tom' WHERE id='1'"; $qry = mysql_query($qry); That is your query to update an individual record. Now you could have: $qry = "UPDATE table SET name = 'tom' WHERE id='1';"; $qry .= "UPDATE table SET name = 'dick' WHERE id='2';"; $qry .= "UPDATE table SET name = 'harry' WHERE id='3';"; $qry = mysql_query($qry); so if you generate you query string in a doo loop you could do it all pretty quickly. -
using ajax would mean you did not have to do all that though! ;) you don't want to have to grab all the possible options first - let the user see a nice clean html structure with minimal js. it would still mean they needed js switched on mind!
-
glen: have a look at this [url=http://brainstormsandraves.com/articles/semantics/structure/]http://brainstormsandraves.com/articles/semantics/structure/[/url] you can't get every browser to look exactly the same (and according to Molly Holzschlag thats a good thing - I agree!) BUT you can create good html. tables are for data NOT layout! pointless html simply takes up extra bandwidth and slows down the users experience of yur site. My advice is to purcahse something like (Sir) Jeffrey Zeldman's desiging with web standards or Dan Shafer's Desiging without tables (a sitepoint book)
-
gdlibrary is a library that allows php to do some image magic! depending on your version of php it may be bundled with it - just find the extension in teh php.ini file and uncomment the line - restart and bang your ready. image_create from is indeed a function. [url=http://uk.php.net/manual/en/ref.image.php]http://uk.php.net/manual/en/ref.image.php[/url]
-
You need AJAX kidda... Don't panic - it doesn't take too long to learn W3C's ajax tutorial is a good place to start. If you need any more help you can get me on msn (toonmariner@hotmail.com)
-
dual_alliance: have a read of the pagination tutorial ([url=http://www.phpfreaks.com/tutorials/43/0.php]http://www.phpfreaks.com/tutorials/43/0.php[/url]) Woodburn: you may benefit from the same tutorial but your needs are a little more specific so here goes... say we have 50 entries in a database and they all have a unique id. The page will grab the record that has an id of 18 and this is defined in the url like so script.php?id=18. Now it may ot follow that previous would be 17 and next would be 19 but the code below won't be affected as we will use the order by clause in the query. ok.. <?php [code] <?php // Select all the records $qry = "SELECT * FROM `ur_table` ORDER BY `your_orderd_field`"; $qry = mysql_query($qry); // create an array of all the results. // the keys of this array will be the same as the table fields... $results = array(); while ($row = mysql_fetch_assoc($qry)) { foreach($row as $key => $val) { $results[$key][] = $val; } } // grab the record you are looking for (id=18) $curr = array_keys($results['id'], 18); $curr = $curr[0]; // array_keys returns an array - even if only one result! $prev = $curr - 1; $next = $curr + 1; echo $results['text_to_display']; // echo the result you want. echo "<a href=\"" . $_SERVER['PHP_SELF'] . "?id=" . $prev . "\">previous</a>"; echo "<a href=\"" . $_SERVER['PHP_SELF'] . "?id=" . $next . "\">next</a>"; ?> [/code] Now there is a more efficient way of doing this which would involve two queries BUT there would be a similar computation to do to get the relevant id's. Only go down that route if the amount of data in your table is enormous....
-
if you are passing data via the url then simply have.. <a href="thisscript.php?id=XXX">previous</a> <a href="thisscript.php?id=YYY">next</a>. Now you must grab the correct values of xxx and yyy to make this work. To do so I would (not the most efficient I suspect but never mind) select all the possible result and use data_seek to traverse the array of results.
-
yep. after the move_uploaded_file you could copy the file to another dir. creating a thumbnail properly requires you to use the gdlibrary (have a look at image_create_form_jpeg _gif etc.)
-
if this a case of testing on a different machine than the live serve you should check to see if register_globals is on or off (it should be off!) and i suspect the testing server has it turned on. You should use if (isset($_POST['submit'])) instead of just if ($submit) OR you can define the short name vars before you process any of the post/get data $submit = $_POST['submit']; and so on.... ALSO it is probably better to pass all the data to the function implicitly instead of using global within the function - just gets you used to doing things in a 'strict' manner. but it aint that important here.
-
hmm maybe you can't cut corners like that then! ;) try this $lookfor = array( '/("/([0-9])+(,)([0-9])/i', '/([0-9])+(\.)([0-9])/i' ); $repwith = array( '\\1.\\3' '\\1,\\3' ); $extra_data = preg_replace($lookfor,$repwith,$data)); if (strcomp($extra_data,$data) != 0) { $new_query[] = "AND (beskrivelse LIKE '%$data%' OR beskrivelse LIKE '%$extra_data%')"; } else { $new_query[] = "AND beskrivelse LIKE '%$data%'"; } $query = implode(' ', $new_query); return $query;
-
$lookfor = array( '/("/([0-9])+(,)([0-9])/i', '/([0-9])+(\.)([0-9])/i' ); $repwith = array( '\\1.\\3' '\\1,\\3' ); if (($extra_data = preg_replace($lookfor,$repwith,$data)) === true) { $new_query[] = "AND (beskrivelse LIKE '%$data%' OR beskrivelse LIKE '%$extra_data%')"; } else { $new_query[] = "AND beskrivelse LIKE '%$data%'"; } $query = implode(' ', $new_query); return $query; Hopefully something like that should do it.
-
2 things to try.... 1. pass the value as numeric instead of in the quotes (i.e. make_slide(\\4) - if it returns an error then forget that. 2. On your testing of the returned string change to this.... return $photo . " length: " . strlen($photo) . " value: " . (0 + $photo); note the operands on the 0 + $photo (why is the 0 + in there?) there is an operator precedence problem there... "a string " . 9 + 67 will evaluate as "a string 9" + 67 which you can't do!!!!
-
it should get the LAST time that user added any thing - and he did say he only wanted the last time.... redarrow - what is the out put of that script? (perhaps remove the exit; command as well ;))
-
<?php $query_time="select `time_added` from `member_topics` where `user_id`='$user_id' ORDER BY `time_added` DESC"; $result_time=mysql_query($query_time); $timmed=mysql_fetch_assoc($result_time); $time_check=$timmed['time_added']; ?> That should do it.
-
Not really... Stab in the dark seems as it appears you are using oop stuff - has the php engine been upgraded?
-
$user = mysql_escape_string($_POST['user']); $pwrd= mysql_escape_string ($_POST['pwrd']); $query = "SELECT * FROM `users` WHERE `username` = '$user' AND `password` = '$pwrd''"; $query = mysql_query($qry); if (mysql_num_rows($query) == 0) { echo "Not recognized"; } if (mysql_num_rows($query) > 1) { echo "Duplicate detection"; // write some code to alert aite admin of problem; } if (mysql_num_rows($query) == 1) { header( 'Location: http://www.3d.caucasus.net/test/admin.php' ) ; } you could make that nicer with a switch statement but I couldn't be arsed today!
-
Determining if a Pocket PC/Smartphone is visiting
ToonMariner replied to phil.t's topic in PHP Coding Help
All these are well and good BUT.... You can save yourself a lot of work and hassel by producing a site has semantic markupand then simply use the css methods I described earlier. Not done much with smarty templates but if you can edit them yourself and create a layout that has no in-line styling (such has height width padding etc.) and simply give elements a class or id then this would be a site that would (could) look good in all clients and be 3000000 times easier to manage...... -
Only penneth to add is that creating a connection to a database uses huge amounts of system resources. Purely on a 'be nice to the server' basis I woudl stick to one database. They are built to take a bot of hammer so don't be scared of having 200 tables in a database - its nothing ;)
-
Determining if a Pocket PC/Smartphone is visiting
ToonMariner replied to phil.t's topic in PHP Coding Help
Ths simple answer is to create a style sheet for handheld devices..... This is how some of my bigger sites css is declared..... <link href="/global/css/screen/screen1.css" rel="stylesheet" type="text/css" media="screen"> <link href="/global/css/braille/braille.css" rel="stylesheet" type="text/css" media="braille"> <link href="/global/css/aural/aural.css" rel="stylesheet" type="text/css" media="aural"> <link href="/global/css/handheld/handheld1.css" rel="stylesheet" type="text/css" media="handheld"> <link href="/global/css/print/print1.css" rel="stylesheet" type="text/css" media="print"> <link href="/global/css/tv/tv1.css" rel="stylesheet" type="text/css" media="tv"> There is a style sheet there for every type of device that cn view your site. You just need to add the media attribute to let the client know which sheet it should use.