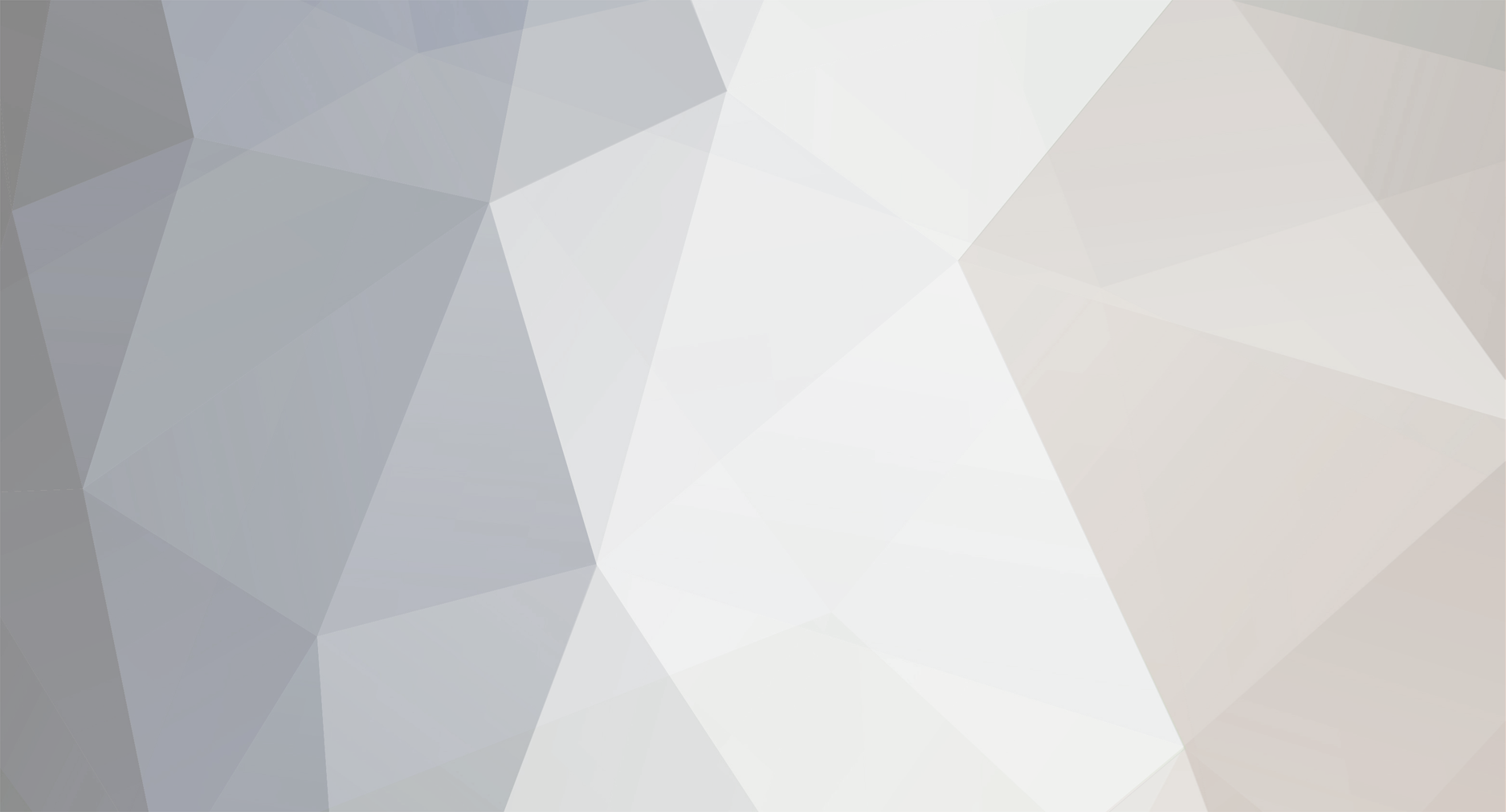
keeB
Staff Alumni-
Posts
1,075 -
Joined
-
Last visited
Everything posted by keeB
-
Database or PHP wise?
-
Need help selecting the right PHP/MySQL web applications
keeB replied to mclamais's topic in Application Design
Looks a lot like Drupal material to me. -
<?php class CMS { private $var1; private $var2; public function header() { $this->var1 = "i was set in header"; } public function body() { echo $this->var1; } } $c = new CMS(); $c->header(); $c->body();
-
A question about design: user and photo classes
keeB replied to MelodyMaker's topic in PHP Coding Help
I have developed something similar, let me share with you how I designed a simple gallery site. Instead of a 'PhotoManager' I used a 'Gallery,' which is kind of the same in principal. A Gallery contains a list of Albums. Albums contain a list of photos. For me, you can choose a Photo to be your Album 'Cover' on display (not really modeled here, but you can see where it would go.) <?php class Photo { private $width; private $height; public function draw(); public function resize($width, $height); //wrapper for imagemagick, returns a new Photo object. public function getDimensions(); // returns width/height public function load($path); public function save($path); } class Album { private $photoList = array(); //contains a list of photos to iterate over in draw(); public function draw(); // draws the collection of photos public function addPhoto(Photo $photo); //add a photo public function clear($this->photoList = null;) } class Gallery { private $album_list = array(); private $galleryDao; public function load(); //use dao to public function draw(); //draws public setDao(GalleryDao $dao) {$this->galleryDao = $dao;} } class GalleryDao { //database logic is here public function findGalleriesByUser(User $user); public function getRandomGallery(); } class User { #model object private $userId; . . . #some other info, doesn't matter public function getId(); public function getName(); } ?> If you have any specific questions, let me know! -
Snip from night: <?php class Parent { private $other; public function __construct() { $this->other = new Other(); } public function doSomething() { $this->other->do(); } } class Child extends Parent { public function newFunction() { parent::__construct(); # added, works. parent::doSomething(); } } ?>
-
If you'd like to run the design by me, I'd be happy to give you some pointers.
-
The thing about pagination is, you need to store a local cache if you want it to be effective/if you're working with large data sets. As for that function posted, it doesn't really explain itself. Where does the data come from? Where's the result set? Looks like this function just displays the pages, but doesn't display the content of the page. If this were implemented this way in OOP, it'd be a mess.
-
Pass it as a parameter function EpicTMAdminOptions($EpicTMOptions) { $epic_t_m_admin_options = array( 'epictmcolumns' => 'true', 'epictmmidtextask' => 'true', 'epictmmidtext' => 'This is some sample text to put in the middle of your post', 'epictmmidcss' => '<style type="text/css"></style>'); $EpicTMOptions = get_option($this->epic_t_m_admin_options_name); if (!empty($EpicTMOptions)) { foreach ($EpicTMOptions as $key => $option) $epic_t_m_admin_options[$key] = $option; } update_option($this->epic_t_m_admin_options_name, $epic_t_m_admin_options); return $epic_t_m_admin_options; }
-
Serialize help! How to make changes to the serialized class!
keeB replied to pendraggon87's topic in PHP Coding Help
Not true. If you want to set up a proper permission scheme you would go with a Role based schema like ACL[1]. Edit: Here's another useful entry[2] [1]: http://en.wikipedia.org/wiki/Access_control_list [2]: http://en.wikipedia.org/wiki/Role-based_access_control -
Serialize help! How to make changes to the serialized class!
keeB replied to pendraggon87's topic in PHP Coding Help
corbin, he said he didnt want to do this because of the amount of columns or whatever. I'm guessing he doesn't know about relational database schema [1]. I think you should look in to a ORM [2]. Propel [3] is a good example. [1]: http://en.wikipedia.org/wiki/Relational_database [2]: http://en.wikipedia.org/wiki/Object-relational_mapping [3]: http://propel.phpdb.org/trac/ -
How to call a pre-existing object from within a class
keeB replied to Naez's topic in PHP Coding Help
No. In that case you use an interface. http://en.wikipedia.org/wiki/Interface_%28computer_science%29 <?php interface Database { function query($q); } public class MySQLDatabaseImpl implements Database { //must define $query; } class foo { public function doSomethingWithADatabase(Database $db) { //enforce usage of Database class return $db->query("select lol from mymom"); // you know $db will have a query method, and your object doesn't actually care what it does. } } ?> -
Take a look at the MVC Pattern [1] In this case, your View would be the page itself, the Controller would be the logic behind login/authentication/etc, and Model would be the User (and it's supporting/related objects) [1]: http://en.wikipedia.org/wiki/Model-view-controller
-
How Can I Create Animated Dynamic Breadcrumbs - Character by character
keeB replied to carrot's topic in PHP Coding Help
Agree with above. -
I'm of the belief that if the problem is too complex to explain, you haven't boiled down exactly what you want to do yet. Asking for advice on the domain will be more fruitful than trying to assess your pseudo situation.
-
This looks 10,000 times better. The bar fading has GOT TO GO, though. Seriously. Make it a solid color like dark blue or something.
-
[SOLVED] Problem with PHP 4 and 5 class difference
keeB replied to Murlyn's topic in PHP Coding Help
Problem is elsewhere. Please post the error message you're receiving from the interpreter. Edit: Btw, this belongs in 3rd party scripts, but since it's semi OOP related I won't tell you to go post it there. -
Bastardization of a design pattern is a Good Thing. Keeping it simple and understandable is the key. That's the 'beauty' of programming (to me!) At the core, a purely abstract class is the same as an interface. That is to say: <?php abstract class A { abstract function foo() {} } Is the same as <?php interface A { public function foo() {} } The difference is (aside from the fact that an interface MUST NOT contain function definitions,) PHP doesn't allow you to have a class which extends multiple base classes, while you can implement as many interfaces as you'd like. Here's an example <?php interface A { public function foo() {} } interface B { public function bar() {} } //works class C implements A,B { //must define foo(), bar() } //doesn't work class C extends A,B { //error } ?>
-
You can do multiple? Cool. edit: If he doesn't I do
-
Gonna have to use 2 queries. I'd do it inside of the same transaction.
-
Plugin calls to functions or Class Methods? Which is better?
keeB replied to Xeoncross's topic in Application Design
Having not written a plugin system recently which implements callbacks, I cannot accurately engage in what would be considered a fruitful conversation. What I can say is this. If you've found a method which plays to your style, then by all means it's the proper method for you. Is there anything you see as a downside to the route you've chosen to go? I'm guessing so, since you came to the board asking about alternatives. So, what's up? What's working? What isn't? -
I expanded my first gallery script in to something quite impressive. It has album support, slideshow support, movie support (with thumbnails ever <x> seconds) This came out of necessity. Whenever we do something as a family extended family wants PICTURES. More pictures! So, I set up a family intranet for family to view all of the wonderful photos my wife takes of us (especially our daughter) on all of our events. It's become quite a hit. Imagine, we'll be visiting something similar years down the road when then grandkids come over. They're going to be like.. wow, this is lame, using such an ancient technology
-
In my opinion, it's better to stick to a defined structure. By a significant margin.. First, OOP is how I choose to work and organize myself. I see a Form as any other Composite[1] object. I could also see developing a Form using the Decorator pattern [2]. This is instinctual, because I don't first think in terms of language features, I think in terms of overall design. What are the benefits? Well, really, to be truthful... none. I think an input tag will always have the same attributes it has now. You'll still have to come up with a way to actually feed the data to your object to call the ->draw() method. You could do it with the __construct() method, but, if a new attribute you want to support is added in this case you have to update your __construct() definition, which really kills downstream apps. You could set a default value on to it (and all other attributes for that matter) to mitigate the damage, but it's kind of ugly. You see, application design a list of trade offs. If you're not flexible and playing to the strengths of your application and instead allow yourself to be unflexible, you really end up with crap. I think that's why a lot of people fail when it comes to conceptualizing OOP. It takes a lot of work and a lot of planning to implement it properly, but I think the payoff is absolutely huge in the end. Last I'll say is my choice in design has been heavily influenced by the man who wrote the following article[3]. I've linked it quite a few times in the past. He wrote an amazing book called Holub On Patterns [4] which brings new meaning every time I read it. [1] http://en.wikipedia.org/wiki/Composite_pattern [2] http://en.wikipedia.org/wiki/Decorator_pattern [3] http://www.javaworld.com/javaworld/jw-09-2003/jw-0905-toolbox.html [4] http://www.amazon.com/Holub-Patterns-Learning-Design-Looking/dp/159059388X
-
Just for completeness, Travis and I just had a conversation about this issue over AIM. I explained, __get() and __set() not being able to access private methods would be practically useless. Since all class attributes should be declared PRIVATE by convention. Tha'ts the reason you create $x->getAttr(); instead of $x->attr = "lol"; Private methods can be exceptionally fragile. They weren't created to be exposed to the user for a reason. If you wanted a user to be able to call them, you'd make them Public!
-
Symphony includes propel, and is based on command line interaction like PEAR is for generating model/database layer code.