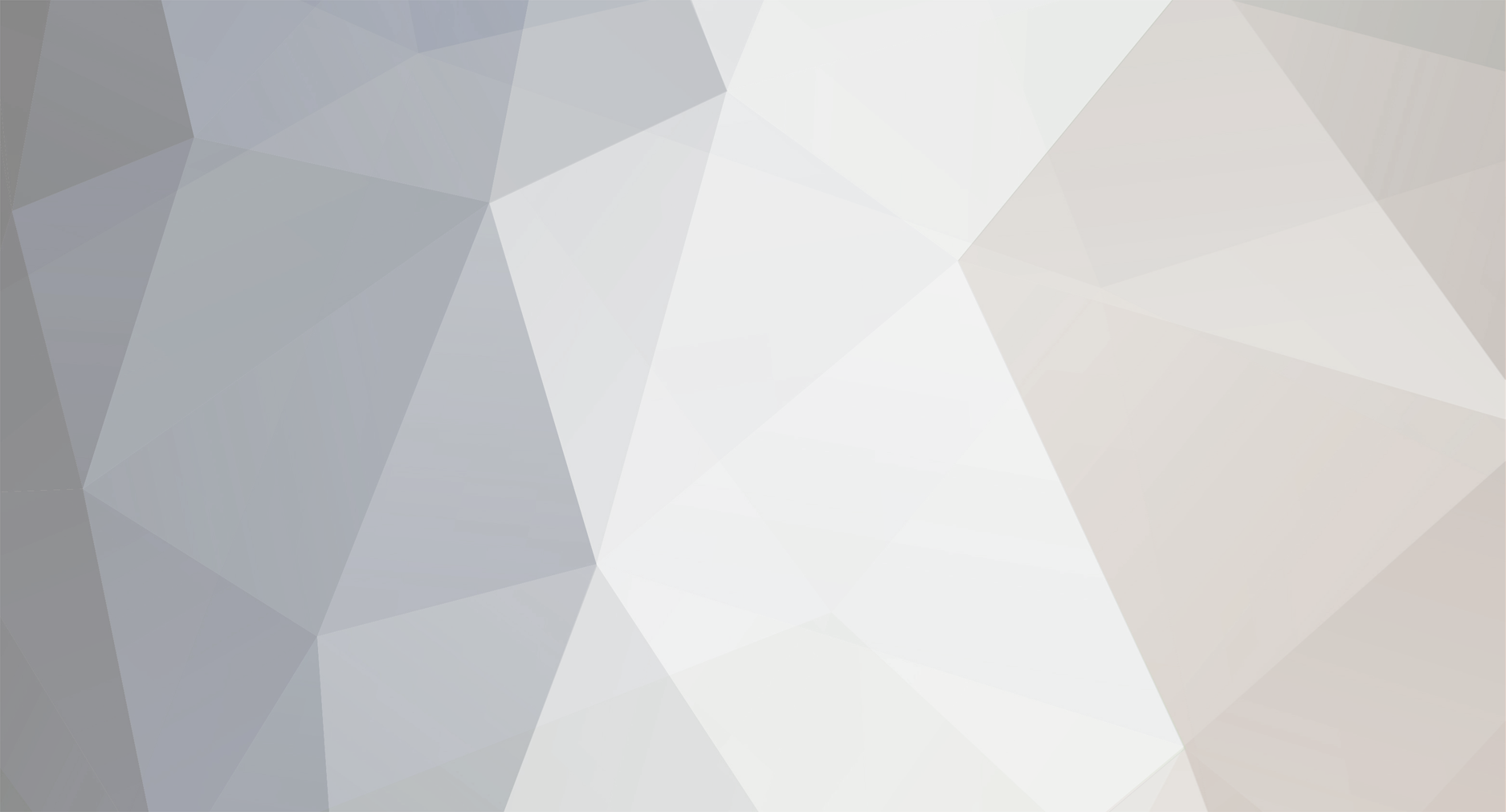
keeB
Staff Alumni-
Posts
1,075 -
Joined
-
Last visited
Everything posted by keeB
-
Debugging with XDebug within Eclipse is pretty amazing with projects of any relative complexity. That, and project management has been unmatched in the many IDE's I have tried (Netbeans PHP Plugin, Geany, Kate, Vim (Love vim, just no project support (that i know of)) I have seen some pretty amazing things that Emacs can do, but their control scheme is way too crazy for me.
-
I think using Error as a case is not a good idea to begin with, because you really should be using Exceptions. Corbin provided you with 2 good examples on how to achieve what you would like.
-
First thought: I'd store the map in a binary format in a single table of the database. Second thought: If you're using a custom list of sprites i'd still go with the first thought, it'd be an extremely compressed format 0 = unfilled 1 = spirte 1 2 = sprite 2 . . . n = sprint n Then I would separate these by some other special character like | So a blank map would look like this: |0x625|0x225|0x400|0x400| Then I'd read it in chunks and assemble. After typing that, I'd just use a different column of the table for each.
-
Sounds like you answered your own question. 1 Email -> 5,000 people should store: The email, The fact that it was sent (successful|failed) to 5,000 people. Table Person ( Id . . ) Table Email ( Id Originator_Id -- Person.Id (index here.) Content Attachments ) Table Sent_Email ( Recipient -- Person.id Email -- Email.Id (index here.) Status -- 0 success, 1 fail, 2 unkown )
-
If it were a (big) project where the type of client really matters, I'd do something like this. Pseudo Database code: create table Person ( userId int, firstname text, . . . ) create table contact_base ( id int, userId int references person(userid), //foreign key to person.userid enabled boolean ) create table msn ( id int references contact_base(id) // foreign key on contact_base.id -- properties , -- unique , -- to , -- msn , } Representation in PHP: <?php class Person { $private $userid = null; . . } class Contact_Base { private $enabled = false; private $id = null; . . . } class MSN extends Contact_Base { # unique msn properties } class PersonDao { function fetchContactInfo($userId) { $q = "SELECT * FROM Contact_Base cb INNER JOIN MSN m on msn.id = Contact_Base.id -- INNER JOIN AIM (etc) where cb.userId = $userId"; } } ?>
-
Easiest method for 'Show new replies to your post'
keeB replied to blackcell's topic in Application Design
I think of a topic on a message board as a container. http://en.wikipedia.org/wiki/Container_%28data_structure%29 If I was implementing my message board with that idea in mind, I might load all (or some, or specific) posts which contain the user, and find the last post. -
I can't think of a reason why you would want to keep an objects state in another class. Can you please elaborate on what you are trying to accomplish?
-
Importing a postgresql generated SQL file using phppgadmin or something else
keeB replied to StreamMe's topic in PostgreSQL
If you have access to the command line: psql -d <database> -f <filename> <username> -
I don't mind that you disagree This isn't my patch, though
-
http://en.wikipedia.org/wiki/Factory_method_pattern
-
http://www.google.com/url?sa=t&ct=res&cd=3&url=http%3A%2F%2Fwww.phpclasses.org%2Fbrowse%2Fpackage%2F3071.html&ei=SwYDSJjoOoOKpwTM0vnQBw&usg=AFQjCNHo0-ymsaeEmIv7Xtg_nD8rhzyPiA&sig2=oVcsOZzVkPbEBQyZgWm28g
-
I think it really only matters when you are developing a library for other people to use. In this case, you really want to ensure the data that is being submitted is the type of data you're looking for. I'd like to be able to typehint basic types of I need to, for some reason. Instead of having: <?php //silly example function add2nums($a, $b) { assert (is_int($a)); assert (is_int($b)); return $a+$b; } //better function add2nums(int $a, int $b) { return $a+$b; } ?> More readable, to me at least
-
Look in to SQL Joins.
-
But, for a CMS, it surely isn't (always submit/post). If you don't want that complexity, make it in the form constructor with default options? Or initialize it with default options? I was just making a clear example so I don't get asked the opposite: "Where would I set action/method?!"
-
Nick, 22, Programming for 13 years. Started in Basic.
-
I made a gallery before. From what I remember my core objects were, Gallery, which contained a list of Albums, which contained a list of Images All Images could draw themselves, resize themselves, etc.
-
I would think using the Composite Pattern would be your best choice, here. More info: http://en.wikipedia.org/wiki/Composite_Pattern What this gives is true dynamic rendering based on, say, a List, Map, Hash(array) from any sort of Datasource. I suppose you could add templating on top of that, but I am not experienced with the existing templating engines because I don't like UI work <?php $f = new Form(); $f->setHandler("submit.php"); $f->setAction("POST"); $f->add(new Checkbox("lol")); $f->add(new Input($name="name", $value="Default text")) $f->flush(); ?>
-
Well, there are times when you really want a base class, and that is when you want common functionality across all (derived) objects.
-
Sure and no First off, __construct() can't return any values so it can't be a singleton. Second, I suggest programming to an interface instead of using a base class. My reasoning is here: http://www.javaworld.com/javaworld/jw-08-2003/jw-0801-toolbox.html Following the rules in that guide you end up with something like this: <?php interface Database { public function connect($host, $username, $password, $database); //establishes and selects database public function query($query); //queries the database, returns array public static function getInstance(); . . . etc } class mySQLi implements Database { private $instance = null; //for singleton public function __construct() {} public static function getInstance() { if ($instance == null) //bla bla } public function connect($...) { mysqli_connect($host, $username, $password) or throw new SQLException("Could not connect to database"); mysqli_select_db($db, $con); } } //now, finally, a DAO. class AlbumDao { private $dbconnection; public function getImageByName($dir) { //find an image in a certain directory; } public function getImageById($id) { //select * from images where id = id } public function initialize($albumName) { // select * from album a // inner join images i on i.album = a.id // inner join videos v on v.album = a.id // where album.short_tile = '$albumName' } public function setDbConnection(Database $dbconnection) {$this->dbconnection = $dbconnection;} } class Album { private $name; private $title; private $dir; private $dao; public function __construct($name) { $this->name = $name; $this->init(); } private init() { $this->dao->initialize($this->name); } public function getImageList() { // some helper functions which return a list of Image objects } public function getVideoList() { // some other helper functions } public function setDao(AlbumDao $dao) {$this->dao = $dao} } //client $db = new mySQLi::getInstance(); $db->connect(...); $album = new Album("Family Photos"); $a->setDao($db); // make sure it's using our database $a->init(); foreach($a->getImageList() as $image) // loop through and get some images.. ?>
-
I love my linux desktops. There isn't a commercial game that I want to play that I cannot play in Linux. As for Windows, I am not a fan but goddamn is it convenient. I think MS is off base, once again. I like that they're headed in the direction of embracing choice, but I don't think it will change anyone's mind if you're going to add a price tag to this choice.
-
Can you give me an example where something like this would be useful? Apparently I'm an idiot because I can't think of a single reason why.
-
You've given me a headache. Whenever people have questions like this (and yes, it happens often enough) it usually results in a fatal flaw in design and indicative of poor forethought. Why can't you do the following? <?php class parent_class { public function foo() { // do stuff... // attempting to call the bar method of the run-time bound class... call_user_func( array( get_class(), 'bar' ) ); } // this is the compile-time bound class protected static function bar() { // do something... echo "parent!"; } } class child_class extends parent_class { // this is the run-time bound class protected static function bar() { // do something ELSE... self::foo(); // added. echo "child!"; } } // invoke parent_class::bar() parent_class::foo(); // ideally, invoke child_class::bar() child_class::foo(); ?> Or am I missing the point completely?
-
Whatever is using your object.
-
Surely it's a bit complicated at first, but that's why you have us Go ahead and ask away and I'll be happy to clarify/help
-
Is there an option D, None of the above? Look in to Data Access Objects (http://en.wikipedia.org/wiki/Data_Access_Object) http://www.phpfreaks.com/forums/index.php/topic,187289.msg839562.html#msg839562