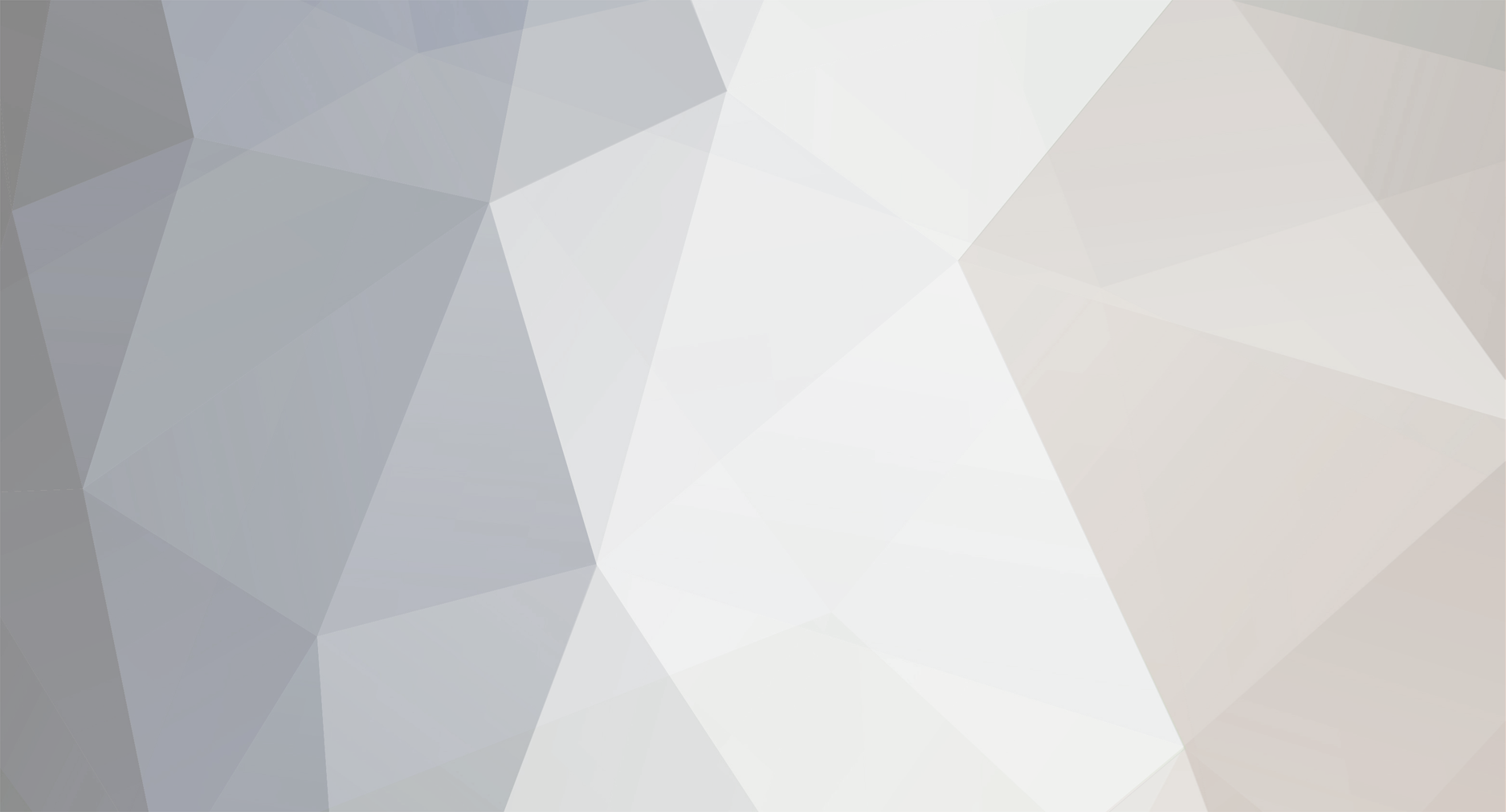
keeB
Staff Alumni-
Posts
1,075 -
Joined
-
Last visited
Everything posted by keeB
-
Your class should look like this (or similar): Notice how I broke up the commonality in to common methods, so that they can be reused and changed in a single place. <?php class Mysql { private $host = 'localhost'; private $user = 'user'; private $pass = 'pass'; private $result = ''; private $db = ''; public function __construct() { } public function set_db($name) { $filename = dirname(__FILE__); if (preg_match('/wwwroot/', $filename)) { $this->db = $name; } } public function get_db_connection() { $conn = @mysql_connect($this->host, $this->user, $this->pass); $this->result = @mysql_select_db($this->db); if(!$conn || !$this->result) { die('Please check the Cabal connection.'); } return $this->result; } } ?>
-
class referenced from with another class not working in IE
keeB replied to calast's topic in PHP Coding Help
I couldn't care less what you do for a living. Your lack of knowledge about the subject was laughable. So, I laughed. I'll bet that the page IS being access via Ajax, and the disparity functionality from browser to browser has to do with JavaScript and not PHP. -
PHP CLI Also preserves the spacing without <pre> tags, which is why it's not there by default
-
class referenced from with another class not working in IE
keeB replied to calast's topic in PHP Coding Help
I seriously lol'd.. A lot. People are staring. -
I made a recipe database for myself, this is what mine looks like... create table food_category ( category_id int not null primary key, name text not null ) go create table food_recipe ( recipe_id int not null primary key, name text not null, category_id int not null REFERENCES food_category (category_id) ) go create table food_ingredient ( ingredient_id int not null primary key, ingredient_name text not null ) go create table food_quantity_type ( quantity_type_id int not null, quantity_name text not null ) go create table food_recipe_ingredient ( recipe_id int not null REFERENCES food_recipe(recipe_id), ingredient_id int not null REFERENCES food_ingredient(ingredient_id), quantity int not null, quantity_type_id int not null REFERENCES food_quantity_type(quantity_type_id) ) go create table food_recipe_tags ( recipe_id int not null REFERENCES food_recipe(recipe_id), tag text not null )
-
What you'll notice, is that as you program more your style will develop naturally. There are a billion different ways to do things. The proper way to do what you're asking would be to break it up in to multiple classes. Encapsulation is about delegating responsibility and keeping things relatively simple. Only by creating a combination of simple objects should you achieve complexity. Reason being, if any part of your requirements change, the changes should be isolated to a small portion of your code base. This phase is called Maintenance. Extending your application should also not impact the rest of your code base.
-
That is the best assumption to make -- You cannot make assumptions
-
That is correct. Generally, you will probably never need to make private methods. But, they are accessible to the class only (as you thought). Here's a code example: <?php class A { private $b = "hi"; } $a = new A() print $a->b; //doesn't work because b is private class C { private $a; public setA($foo) { $this->a = $foo; } } $c = new C(); $c->setA('hello!'); // this does work! /// now lets mix what we know about public and private and make a bit more complex object class D { private $a; public setA($foo) { $this->doSomethingWithA($foo); } private doSomethingWithA($foo) { $this->a = substr($foo, 0, 5); } public function getA() { return $this->a; } } $d = new D(); $d->setA('Something long string...........'); print $d->getA(); // returns 'Somet' ?>
-
How that ever happens is beyond me .. ;x
-
I think it's a great first attempt. My only complaint (and bad habit) is not declaring public, private variables/functions so any client code can access your object directly. This is not a good idea.
-
FTP is not secure so I do not recommend that. I would break it in to 2 (or more) Classes. One would just represent a single file, while the other would represent the Collection of files. If you have any specific questions let me know
-
I don't think it should matter so much, nor should your framework be so tightly coupled with the way the data is coming in. You should have an implement an Interface that translates the Query String in to a data structure that you can understand down stream in your application. <?php class QueryString { //model object to be passed down stream private $controller; private $method; private $args = array(); // .. get methods .. // .. set methods .. } interface QueryStringTranslationService { // document how toQueryString should return a QueryString Model Object public function toQueryString($arr); } class GETQueryStringTranslationServiceImpl implements QueryStringTranslationService { public function toQueryString($arr) { //arr should be passed to here by application because it has determined you've received a $_GET request in some fashion $qs = new QueryString(); $qs->setController($arr['c']); $qs->setMethod($arr['m']); $qs->setArgs($arr['args']); return $qs; } } ?> The benefit of doing it this way is if there is some new trend of the month (which there always is for the web) and the URL is structured differently, you (or anyone using your framework) would be able to quickly swap out the GETQueryStringTranslationServiceImpl () for an implementation that more suits your needs. This gets back to another question of yours I just answered. In my opinion, there are the types of things frameworks need to provide.
-
What kind of things are you doing? Did you know you can run PHP outside of a web environment and lets your scripts run for much longer? My usual approach is to write native applications to get things done that does not belong on the web. I then implement an interface to these processes via PHP so I can share whatever the native application is doing with anyone who is interested. I feel you should leverage the strengths of all of your tool set. Start to think about.. what things can I offload to something in C#.Net and how can I hook in to that to display it in PHP? Good luck. If you have any questions about this type of thing feel free to ask!
-
What would you like a frameowrk to do automatically
keeB replied to Liquid Fire's topic in Application Design
This is something I would not like the framework to do automatically. I would prefer to be provided an extension point to implement Decorator's. An example of what a Decorator is can be found on Wikipedia here: http://en.wikipedia.org/wiki/Decorator_pattern -
I don't really understand exactly what you're asking. I see you have a feature list, what would you like help with?
-
What should be able to be done within a user management system?
keeB replied to Liquid Fire's topic in Application Design
I think you have a great list started there. I would like to point out, you should probably really expand on the 'Edit user information' for whatever type of application you're developing. -
This line is peculiar for($i = 0; $i < count($this->file_src_name); $i++) { If I am reading it properly, you're getting the count() of $this->file_src_name .. why would it ever be anything other than 1? I bet you if you figure that out the problem will really go away.
-
Localization is normally done with templates an external strings. Here's an example: main_en_Us.properties loginform.username.inputfield='Login' loginform.password.inputfield='Password' main.template.php <html> <form action="some.php" method="post"> <caption><?php load_localized_string('loginform.username.inputfield', $user->getLocale()); ?></caption> <input type="text" name="username" /> <caption><?php load_localized_string('loginform.password.inputfield', $user->getLocale()); ?></caption> <input type="password" name="password" /> </form> </html> wherever load_localized_string is stored: <?php function load_localized_string($resource, $locale) { $resource_file = null; if ($locale == "" || $locale == null) { $resource_file = "main_en_US.properties"; } // map $locale to $resource_file // load resource file // parse resource file // return value in resource file based on key } Very basic example of how I would implement this in PHP. I would probably load the entire file up front and have it cached for easier access.
-
Yeah, I just read about this. So, in my example, It would look like this: <?php class A { public $b; } function set_b($obj) { $obj->b = "lol"; } $a = new A(); $a->b = "before"; $c = clone $a; set_b($a); print $a->b; //i would expect this to show 'before' print $c->b; //i would ESPECIALLY expect this to show 'before' ?> Can I do this? <?php set_b(clone $a) ?> I guess I'll try it out.
-
Wow. I actually never knew that everything was passed by reference in PHP. That's dumb. Here's a proof: <?php class A { public $b; } function set_b($obj) { $obj->b = "lol"; } $a = new A(); $a->b = "before"; $c = $a; set_b($a); print $a->b; //i would expect this to show 'before' print $c->b; //i would ESPECIALLY expect this to show 'before' ?>
-
max_user_connections error - need troubleshooting help
keeB replied to cougkid's topic in MySQL Help
What you're talking about is a mysql_pconnect(), mysql_pclose() -- persistent connections. -
The advantage is separating logic from presentation. In this example, I would wrap that tidbit in a function and return the array. A procedural approach <?php //some-include-file.inc.php function get_option($obj, $value, $display) { return array($obj->$value, $obj->$display); } //some-display-file.php $result = new SomeObject(); $ret = get_option($result, $value, $display); print '<option value="' . $ret[0] . '">' . $ret[1] . '</option>'; An OO approach would be harder to template for you without more detail.
-
Don't mix in HTML this way. It's always a bad idea and makes your code much more confusing to read. <?php class A { public $a = "1"; public $b = "2"; } $result = new A(); $value = a; $display = b; print_r( $ret =array($result->$value, $result->$display)); print '<option value="' . $ret[0] . '">' . $ret[1] . '</option>'; ?>
-
max_user_connections error - need troubleshooting help
keeB replied to cougkid's topic in MySQL Help
This is a Database help question. The problem probably lies here. Your script is probably not closing it's connection when the query is complete. -
Your class name is 'template' not Template.