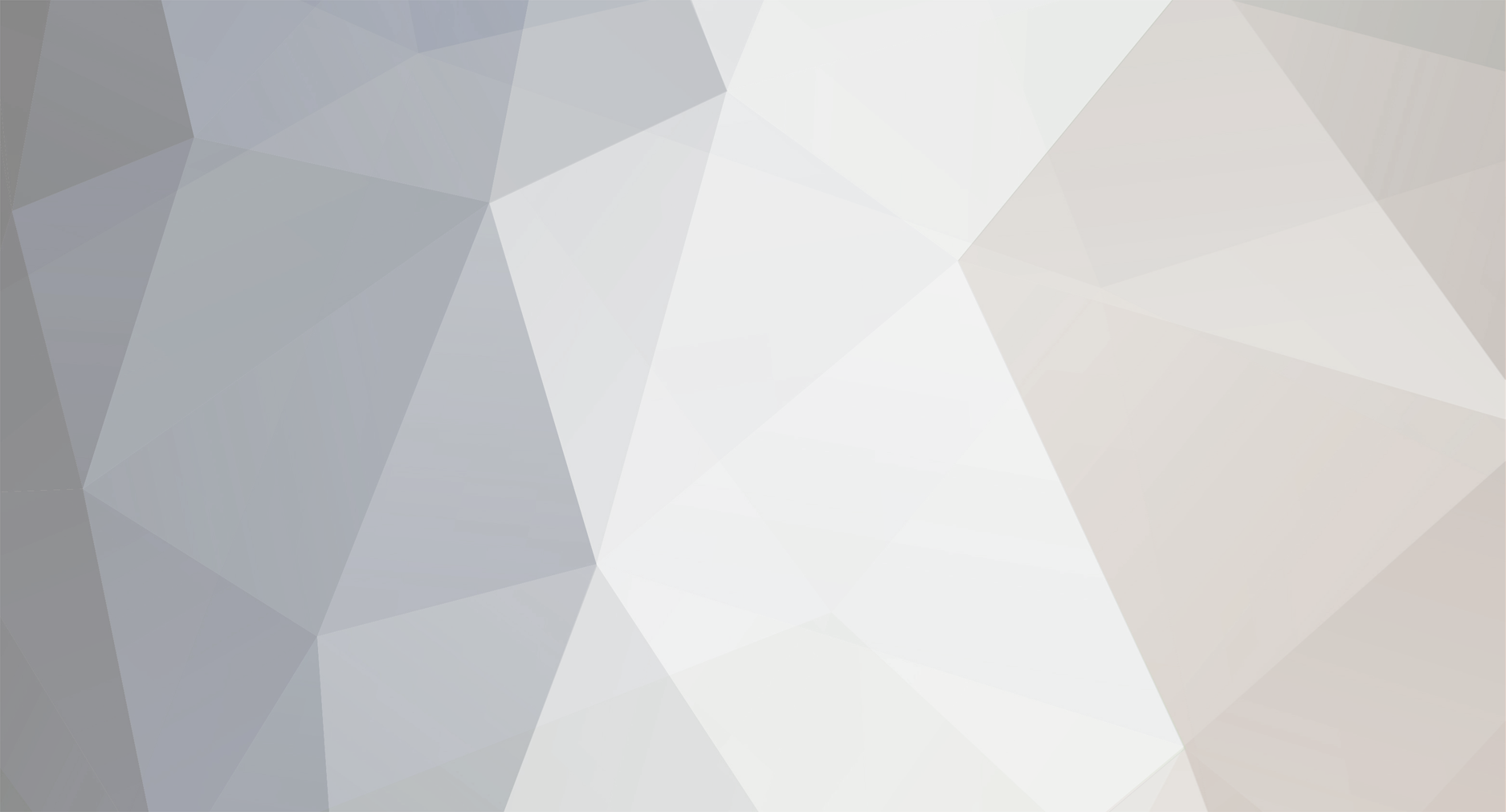
cobusbo
Members-
Posts
224 -
Joined
-
Last visited
Everything posted by cobusbo
-
Hi, I im using an API script to retrieve info, but im getting the error Can anyone please help me out what could be the cause of this error and how to resolve it? <?php /** * MXit API PHP Wrapper - version 1.4.0 * * Written by: Ashley Kleynhans <[email protected]> * * Copyright 2013 DigiGoblin (Pty) Ltd. * * Licensed under the Apache License, Version 2.0 (the "License"); * you may not use this file except in compliance with the License. * You may obtain a copy of the License at * * http://www.apache.org/licenses/LICENSE-2.0 * * Unless required by applicable law or agreed to in writing, software * distributed under the License is distributed on an "AS IS" BASIS, * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. * See the License for the specific language governing permissions and * limitations under the License. * */ /** * Ensure that CURL and JSON PHP extensions are present */ if (!function_exists('curl_init')) { throw new Exception('The Mxit PHP class is unable to find the CURL PHP extension.'); } if (!function_exists('json_decode')) { throw new Exception('The Mxit PHP class is unable to find the JSON PHP extension.'); } if (!function_exists('base64url_decode')) { function base64url_decode($base64url) { $base64 = strtr($base64url, '-_', '+/'); $plainText = base64_decode($base64); return ($plainText); } } if (!function_exists('is_json')) { function is_json($json) { json_decode($json); return (json_last_error() == JSON_ERROR_NONE); } } if (!function_exists('is_base64')) { function is_base64($encoded_string) { $length = strlen($encoded_string); for ($i = 0; $i < $length; ++$i) { $c = $encoded_string[$i]; if ( ($c < '0' || $c > '9') && ($c < 'a' || $c > 'z') && ($c < 'A' || $c > 'Z') && ($c != '+') && ($c != '/') && ($c != '=') ) { return false; } } return true; } } class MxitAPI { private $_version; private $_base_outh_url; private $_base_user_api_url; private $_base_messaging_api_url; private $_app_key; private $_app_secret; private $_token_type; private $_access_token; private $_expires_in; private $_scope; private $_id_token; private $_headers; public $http_status; public $content_type; public $result; public $error; public function __construct($key, $secret) { $this->_version = '1.4.0'; $this->_base_outh_url = 'https://auth.mxit.com/'; $this->_base_user_api_url = 'http://api.mxit.com/user/'; $this->_base_messaging_api_url = 'http://api.mxit.com/message/'; $this->_app_key = $key; $this->_app_secret = $secret; $this->error = FALSE; } private function _call_api($url, $method='POST', $params='', $decode=TRUE) { $this->http_status = NULL; $this->content_type = NULL; $this->result = NULL; $this->error = FALSE; $fields = ''; if (($method == 'POST' || $method == 'PUT' || $method == 'DELETE') && $params != '') { $fields = (is_array($params)) ? http_build_query($params) : $params; } if ($method == 'PUT' || $method == 'POST' || $method == 'DELETE') { $this->_headers[] = 'Content-Length: '. strlen($fields); } $opts = array( CURLOPT_CONNECTTIMEOUT => 10, CURLOPT_RETURNTRANSFER => true, CURLOPT_VERBOSE => false, CURLOPT_SSL_VERIFYPEER => false, CURLOPT_TIMEOUT => 60, CURLOPT_USERAGENT => 'mxit-php-'. $this->_version, CURLOPT_URL => $url, CURLOPT_HTTPHEADER => $this->_headers ); if (($method == 'POST' || $method == 'PUT' || $method == 'DELETE') && $params != '') { $opts[CURLOPT_POSTFIELDS] = $fields; } if ($method == 'POST' && is_array($params)) { $opts[CURLOPT_POST] = count($params); } elseif ($method == 'PUT') { $opts[CURLOPT_CUSTOMREQUEST] = 'PUT'; } elseif ($method == 'DELETE') { $opts[CURLOPT_CUSTOMREQUEST] = 'DELETE'; } elseif ($method == 'POST') { $opts[CURLOPT_POST] = TRUE; } $ch = curl_init(); curl_setopt_array($ch, $opts); $result = curl_exec($ch); $this->http_status = curl_getinfo($ch, CURLINFO_HTTP_CODE); $this->content_type = curl_getinfo($ch, CURLINFO_CONTENT_TYPE); curl_close($ch); if ($this->http_status != 200) { // Problem with API call, we received an HTTP status code other than 200 $this->error = TRUE; } $this->result = (($decode === TRUE) && (is_json($result) === TRUE)) ? json_decode($result) : $result; } private function _api_headers($format='application/json') { $this->_headers = array(); $this->_headers[] = 'Content-type: '. $format; // Don't specify the accept format if data is being sent as an octet-stream if ($format == 'application/json' || $format == 'application/xml') $this->_headers[] = 'Accept: '. $format; $this->_headers[] = 'Authorization: '. ucfirst($this->_token_type) .' '. $this->_access_token; } private function _check_scope($method, $scope) { if (strstr($this->_scope, $scope) === FALSE) throw new Exception('Invalid scope specified for '. $method .'() method, should be: '. $scope); if (!isset($this->_access_token) || !isset($this->_token_type) || !isset($this->_expires_in)) throw new Exception('Access token is not set, obtain an access token using get_token()'); } /** * Requests the user to allow your application access to specific MXit information * * Redirects back to redirect_uri/?code=f00df00df00df00df00df00df00df00d * * Where the value of code (f00df00df00df00df00df00df00df00d in this example) * is used to obtain the access token. */ public function request_access($redirect_uri, $scope, $state='') { $url = $this->_base_outh_url ."authorize?response_type=code&client_id=". $this->_app_key; $url .= "&redirect_uri=". urlencode($redirect_uri); $url .= "&scope=". urlencode($scope); if ($state != '') $url .= '&state='. urlencode($state); header('Location: '. $url); exit; } /** * Authenticates the user against their MXit credentials * * SUCCESS: * Redirects back to redirect_uri/?code=f00df00df00df00df00df00df00df00d * * FAILURE: * Redirects back to redirect_uri/?error=access_denied * * Where the value of code (f00df00df00df00df00df00df00df00d in this example) * is used to obtain the access token. */ public function authenticate($redirect_uri, $state='') { $this->request_access($redirect_uri, 'openid', $state); } /** * Checks the authentication status from the authentication server callback */ public function authentication_status() { if (isset($_GET['code'])) { $authenticated = TRUE; } else { $authenticated = FALSE; $this->error = TRUE; } return $authenticated; } public function refresh_token() { $url = $this->_base_outh_url . "token"; $params = array( 'grant_type' => 'refresh_token', 'refresh_token' => $this->_refresh_token, ); $this->_headers = array(); $this->_headers[] = 'Authorization: Basic '. base64_encode($this->_app_key .':'. $this->_app_secret); $this->_headers[] = "Content-Type: application/x-www-form-urlencoded"; $this->_call_api($url, 'POST', $params); if ($this->error === FALSE) { $this->_access_token = $this->result->access_token; $this->_token_type = $this->result->token_type; $this->_expires_in = $this->result->expires_in; $this->_refresh_token = isset($this->result->refresh_token) ? $this->result->refresh_token : null; $this->_scope = $this->result->scope; // Only applicable to OpenID token requests if (isset($this->result->id_token)) $this->_id_token = $this->result->id_token; } } /** * Request the actual token from the OAuth2 server */ public function get_user_token($code, $redirect_uri) { $url = $this->_base_outh_url ."token"; $params = array('grant_type' => 'authorization_code', 'code' => $code, 'redirect_uri' => $redirect_uri); $this->_headers = array(); $this->_headers[] = 'Authorization: Basic '. base64_encode($this->_app_key .':'. $this->_app_secret); $this->_headers[] = "Content-Type: application/x-www-form-urlencoded"; $this->_call_api($url, 'POST', $params); if ($this->error === FALSE) { $this->_access_token = $this->result->access_token; $this->_token_type = $this->result->token_type; $this->_expires_in = $this->result->expires_in; $this->_refresh_token = isset($this->result->refresh_token) ? $this->result->refresh_token : null; $this->_scope = $this->result->scope; // Only applicable to OpenID token requests if (isset($this->result->id_token)) $this->_id_token = $this->result->id_token; } } /** * Get an access token for an application, to perform an API Request */ public function get_app_token($scope, $grant_type='client_credentials', $username='', $password='') { $url = $this->_base_outh_url ."token"; $this->_headers = array(); $this->_headers[] = 'Authorization: Basic '. base64_encode($this->_app_key .':'. $this->_app_secret); $this->_headers[] = "Content-Type: application/x-www-form-urlencoded"; $params = array('grant_type' => $grant_type, 'scope' => $scope); if ($grant_type == 'password') { $params['username'] = $username; $params['password'] = $password; } $this->_scope = $scope; $this->_call_api($url, 'POST', $params); if ($this->error === FALSE) { $this->_access_token = $this->result->access_token; $this->_token_type = $this->result->token_type; $this->_expires_in = $this->result->expires_in; $this->_refresh_token = isset($this->result->refresh_token) ? $this->result->refresh_token : null; } } /** * Get access token detail */ public function get_token() { $detail = array('access_token' => $this->_access_token, 'token_type' => $this->_token_type, 'expires_in' => $this->_expires_in, 'refresh_token' => $this->_refresh_token, 'scope' => $this->_scope); return $detail; } /** * Set access token detail */ public function set_token($detail) { $this->_access_token = $detail['access_token']; $this->_token_type = $detail['token_type']; $this->_expires_in = $detail['expires_in']; $this->_refresh_token = $detail['refresh_token']; $this->_scope = $detail['scope']; } /** * Validate OpenID token */ public function validate_token() { if (isset($this->_id_token)) { $token_parts = explode('.', $this->_id_token); $header = base64url_decode($token_parts[0]); $payload = base64url_decode($token_parts[1]); $signature = base64url_decode($token_parts[2]); if (is_json($header) && is_json($payload)) { $header = json_decode($header); $payload = json_decode($payload); if ($payload->aud != $this->_app_key) return FALSE; // Time zone differences could potentially pose a problem, so disabling for now /*if ($payload->exp < time()) return FALSE;*/ return $payload->user_id; } else { return FALSE; } } else { return FALSE; } } /** * ------------------------------------------------------------------------------ * The following methods are publicly available (no user authentication) required * so you should use the get_app_token() method when working with them. * ------------------------------------------------------------------------------- */ /** * Get the users internally unique UserId for the provided MxitId or LoginName. * * Url: http://api.mxit.com/user/lookup/{MXITID} * * Application Token Required * * Required scope: profile/public */ public function get_user_id($login) { $this->_check_scope('get_user_id', 'profile/public'); $url = $this->_base_user_api_url ."lookup/". $login; $this->_api_headers(); $this->_call_api($url, 'GET'); return $this->result; } /** * Get the status message for the given MXitId. * * Url: http://api.mxit.com/user/public/statusmessage/{MXITID} * * Application Token Required * * Required scope: profile/public */ public function get_status($login) { $this->_check_scope('get_status', 'profile/public'); $url = $this->_base_user_api_url ."public/statusmessage/". $login; $this->_api_headers(); $this->_call_api($url, 'GET'); return $this->result; } /** * Get the nickname of a MXit user with the given MXitId. * * Url: http://api.mxit.com/user/public/displayname/{MXITID} * * Application Token Required * * Required scope: profile/public */ public function get_display_name($login) { $this->_check_scope('get_display_name', 'profile/public'); $url = $this->_base_user_api_url ."public/displayname/". $login; $this->_api_headers(); $this->_call_api($url, 'GET'); return $this->result; } /** * Download the user avatar image for a MXit user with the given MXitId. * * Url: http://api.mxit.com/user/public/avatar/{MXITID} * * Application Token Required * * Required scope: profile/public */ public function get_avatar($login) { $this->_check_scope('get_avatar', 'profile/public'); $url = $this->_base_user_api_url ."public/avatar/". $login; $this->_api_headers(); $this->_call_api($url, 'GET', '', FALSE); return $this->result; } /** * Get the basic profile information for a MXit user with the given UserId. * * Url: http://api.mxit.com/user/profile/{USERID} * * Application Token Required * * Required scope: profile/public * * NOTE: This method requires the user's mxitid and NOT their login */ public function get_basic_profile($mxitid) { $this->_check_scope('get_basic_profile', 'profile/public'); $url = $this->_base_user_api_url ."profile/". $mxitid; $this->_api_headers(); $this->_call_api($url, 'GET'); return $this->result; } /** * Send a message to one or more MXit users. * Url: http://api.mxit.com/message/send/ * Application Token Required * Required scope: message/send or message/user * * @param string $from * @param string $to * @param string $message * @param boolean $contains_markup * @param array $links array of associative arrays * http://dev.mxit.com/docs/api/messaging/post-message-send * * <code> * $links = array( * array( * 'CreateTemporaryContact':true, * 'TargetService':'Service name', * 'Text':'Link text' * ); * ); * </code> * * @param boolean $spool * @param int $spool_timeout * * @return int error code see http://dev.mxit.com/docs/common-error-codes * for details */ public function send_message($from, $to, $message, $contains_markup=true, $links=array(), $spool=true, $spoolTimeOut=3600) { try { $this->_check_scope('send_message', 'message/send'); } catch(Exception $e) { $this->_check_scope('send_message', 'message/user'); } $params = array( 'Body' => $message, 'ContainsMarkup' => $contains_markup, 'From' => $from, 'To' => $to, 'Spool' => $spool, 'SpoolTimeOut' => $spoolTimeOut, ); if(count($links) > 0) { $params['Links'] = $links; } $url = $this->_base_messaging_api_url ."send/"; $this->_api_headers(); $this->_call_api($url, 'POST', json_encode($params)); } /** * ---------------------------------------------------------------------------------- * The following methods require authentication with the user's username and password * ----------------------------------------------------------------------------------- */ /** * Retrieves the full profile including the cellphone number and email address of the userid in the access token. * * User Token Required * * Required scope: profile/private */ public function get_full_profile($bypass_scope_check=FALSE) { if ($bypass_scope_check === FALSE) $this->_check_scope('get_full_profile', 'profile/private'); $url = $this->_base_user_api_url ."profile"; $this->_api_headers(); $this->_call_api($url, 'GET'); return $this->result; } /** * Update the profile information for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/profile * * User Token Required * * Required scope: profile/write */ public function update_profile($data) { $this->_check_scope('update_profile', 'profile/write'); $url = $this->_base_user_api_url ."profile"; $this->_api_headers(); $this->_call_api($url, 'PUT', json_encode($data)); return $this->result; } /** * Subscribes the MXit user with the given UserId, contained in the access token, to the * service or sends an invite to another user. If {contact} is a Service, then the service * is added and accepted. If {contact} is a MXitId, then an invite is sent to the other user. * * User Token Required * * Required scope: contact/invite * * NOTE: This method requires the user's mxitid and NOT their login */ public function add_contact($mxitid) { $this->_check_scope('add_contact', 'contact/invite'); $url = $this->_base_user_api_url ."socialgraph/contact/". $mxitid; $this->_api_headers(); $this->_call_api($url, 'PUT'); } /** * Get the social graph information for a MXit user with the given UserId contained in the * access token. * * Filters: @All, @Friends, @Apps, @Invites, @Connections (Default), @Rejected, @Pending, @Deleted, @Blocked * * Url: http://api.mxit.com/user/socialgraph/contactlist?filter={FILTER}&skip={SKIP}&count={COUNT} * * User Token Required * * Required scope: graph/read * * NOTE: You might expect count to return 2 items if you specify a value of 2, but it will return 3 items * So you should treat the value similar to an array value, ie specify 1 if you want 2 results */ public function get_contact_list($filter='', $skip=0, $count=0) { $this->_check_scope('get_contact_list', 'graph/read'); $url = $this->_base_user_api_url ."socialgraph/contactlist"; if ($filter != '') $url .= '?filter='. $filter; if ($skip != 0) { $url .= ($filter == '') ? '?' : '&'; $url .= 'skip='. $skip; } if ($count != 0) { $url .= ($filter == '' && $skip == 0) ? '?' : '&'; $url .= 'count='. $count; } $this->_api_headers(); $this->_call_api($url, 'GET'); return $this->result; } /** * Get a list of friends the user might know for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/socialgraph/suggestions * * User Token Required * * Required scope: graph/read * * FIXME: HTTP status 400 (Bad Request) */ public function get_friend_suggestions() { $this->_check_scope('get_friend_suggestions', 'graph/read'); $url = $this->_base_user_api_url ."socialgraph/suggestions"; $this->_api_headers('application/xml'); $this->_call_api($url, 'GET'); return $this->result; } /** * Set the status message for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/statusmessage * * User Token Required * * Required scope: status/write */ public function set_status($message) { $this->_check_scope('set_status', 'status/write'); $url = $this->_base_user_api_url ."statusmessage"; $this->_api_headers(); $this->_call_api($url, 'PUT', json_encode($message)); } /** * Upload or create an avatar image for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/avatar * * User Token Required * * Required scope: avatar/write */ public function set_avatar($data, $mime_type='application/octet-stream') { $this->_check_scope('set_avatar', 'avatar/write'); // Base64 encoding is deprecated if (is_base64($data)) { throw new Exception('Base64 encoding of avatar data is deprecated'); } else { $url = $this->_base_user_api_url ."avatar"; $this->_api_headers($mime_type); $this->_call_api($url, 'POST', $data); } } /** * Delete a avatar image for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/avatar * * User Token Required * * Required scope: avatar/write */ public function delete_avatar() { $this->_check_scope('delete_avatar', 'avatar/write'); $url = $this->_base_user_api_url ."avatar"; $this->_api_headers(); $this->_call_api($url, 'DELETE'); } /** * Get the gallery root information for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/media/ * * User Token Required * * Required scope: content/read */ public function list_gallery_folders() { $this->_check_scope('list_gallery_folders', 'content/read'); $url = $this->_base_user_api_url ."media/"; $this->_api_headers(); $this->_call_api($url, 'GET'); return $this->result; } /** * Get the list of content in the gallery folder for a user with the given UserId contained in the access token and FolderId. * * Url: http://api.mxit.com/user/media/list/{FOLDERNAME}?skip={SKIP}&count={COUNT} * * User Token Required * * Required scope: content/read */ public function list_gallery_items($folder, $skip=0, $count=0) { $this->_check_scope('list_gallery_items', 'content/read'); $url = $this->_base_user_api_url ."media/list/". urlencode($folder); if ($skip != 0) { $url .= '?skip='. $skip; } if ($count != 0) { $url .= ($filter == '' && $skip == 0) ? '?' : '&'; $url .= 'count='. $count; } $this->_api_headers(); $this->_call_api($url, 'GET'); return $this->result; } /** * Creates a gallery folder for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/media/{FOLDERNAME} * * User Token Required * * Required scope: content/write */ public function create_gallery_folder($folder) { $this->_check_scope('create_gallery_folder', 'content/write'); $url = $this->_base_user_api_url ."media/". urlencode($folder); $this->_api_headers(); $this->_call_api($url, 'POST'); } /** * Rename a gallery folder for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/media/{FOLDERNAME} * * User Token Required * * Required scope: content/write */ public function rename_gallery_folder($source, $destination) { $this->_check_scope('rename_gallery_folder', 'content/write'); $url = $this->_base_user_api_url ."media/". urlencode($source); $this->_api_headers(); $this->_call_api($url, 'PUT', json_encode($destination)); } /** * Delete a gallery folder for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/media/{FOLDERNAME} * * User Token Required * * Required scope: content/write */ public function delete_gallery_folder($folder) { $this->_check_scope('delete_gallery_folder', 'content/write'); $url = $this->_base_user_api_url ."media/". urlencode($folder); $this->_api_headers(); $this->_call_api($url, 'DELETE'); } /** * Download the content item in the user's gallery for a MXit user with the given UserId contained in the access token and given FileId. * * Url: http://api.mxit.com/user/media/content/{FILEID} * * User Token Required * * Required scope: content/read */ public function download_gallery_image($file_id) { $this->_check_scope('download_gallery_image', 'content/read'); $url = $this->_base_user_api_url ."media/content/". $file_id; $this->_api_headers(); $this->_call_api($url, 'GET', '', FALSE); return $this->result; } /** * Upload a gallery file for a MXit user with the given UserId contained in the access token. * * CURRENT: * Url: http://api.mxit.com/user/media/file/{FOLDERNAME}?fileName={FILENAME} * * DEPRECATED: * Url: http://api.mxit.com/user/media/file/{FOLDERNAME}?fileName={FILENAME}&mimeType={MIMETYPE} * * User Token Required * * Required scope: content/write */ public function upload_gallery_image($folder, $filename, $mime_type, $data) { $this->_check_scope('upload_gallery_image', 'content/write'); // Base64 encoding is deprecated if (is_base64($data)) { throw new Exception('Base64 encoding of gallery data is deprecated'); } else { $url = $this->_base_user_api_url ."media/file/". urlencode($folder) .'?fileName='. urlencode($filename); $this->_api_headers($mime_type); $this->_call_api($url, 'POST', $data); } } /** * Rename a gallery file for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/media/file/{FILEID} * * User Token Required * * Required scope: content/write */ public function rename_gallery_image($file_id, $destination) { $this->_check_scope('rename_gallery_image', 'content/write'); $url = $this->_base_user_api_url ."media/file/". urlencode($file_id); $this->_api_headers(); $this->_call_api($url, 'PUT', json_encode($destination)); } /** * Rename a gallery file for a MXit user with the given UserId contained in the access token. * * Url: http://api.mxit.com/user/media/file/{FILEID} * * User Token Required * * Required scope: content/write */ public function delete_gallery_image($file_id) { $this->_check_scope('delete_gallery_image', 'content/write'); $url = $this->_base_user_api_url ."media/file/". urlencode($file_id); $this->_api_headers(); $this->_call_api($url, 'DELETE'); } /** * Upload a file of any type to store and return a FileId once file offer has been sent * * CURRENT: * Url: http://api.mxit.com/user/media/file/send?fileName={FILENAME}&userId={USERID} * * DEPRECATED: * Url: http://api.mxit.com/user/media/file/send?fileName={FILENAME}&mimeType={MIMETYPE}&userId={USERID} * * User Token Required * * Required scope: content/send */ public function send_file($user_id, $filename, $mime_type, $data) { $this->_check_scope('send_file', 'content/send'); // Base64 encoding is deprecated if (is_base64($data)) { throw new Exception('Base64 encoding of gallery data is deprecated'); } else { $url = $this->_base_user_api_url ."media/file/send?fileName=". urlencode($filename) .'&userId='. urlencode($user_id); $this->_api_headers($mime_type); $this->_call_api($url, 'POST', $data); } } } ?>
-
Hi I'm currently using a header to retrieve some info $prof = $_SERVER["HTTP_X_MXIT_PROFILE"]; It output the value as en,ZA,1991-01-30,Male,1 I'm trying to use the 1991-01-30 from the above output to calculate if the person is 18 years of age or older Any suggestions on how to do this I tried to put it into an array but was unsuccessful...
-
Ah thank you I see I had the same function on both pages and got redeclare error so I fixed it
-
Hi I got two functions and I want to use the 2nd function within the first function is this possible Function 1 class poll { public $db; public $tbl; public $pollvars; public $poll_view_html; public $poll_result_html; public $options; public $options_text; public $poll_question; public $form_forward; public $template_set; public $ip; public function __construct() { global $POLLTBL, $CLASS; $this->tbl = $POLLTBL; $this->poll_view_html = array(); $this->poll_result_html = array(); $this->options = array(); $this->options_text = array(); $this->poll_question = array(); $this->form_forward = basename($_SERVER['PHP_SELF']); $this->ip = getenv("REMOTE_ADDR"); $this->db = &$CLASS["db"]; $this->pollvars = $this->db->fetch_array($this->db->query("SELECT * FROM ".$this->tbl['poll_config'])); $this->template_set = "default"; if ($this->pollvars['result_order'] == "asc") { $this->pollvars['result_order'] = "ORDER BY votes ASC"; } elseif ($this->pollvars['result_order'] == "desc") { $this->pollvars['result_order'] = "ORDER BY votes DESC"; } else { $this->pollvars['result_order'] = ''; } } Function 2 function getUserIP() { $mxitidun = $_SERVER['HTTP_X_MXIT_DEVICE_ID']; $client = @$_SERVER['HTTP_CLIENT_IP']; $forward = @$_SERVER['HTTP_X_FORWARDED_FOR']; $remote = $_SERVER['REMOTE_ADDR']; if($mxitidun != '') { $ip1 = $mxitidun; } elseif(filter_var($client, FILTER_VALIDATE_IP)) { $ip1 = $client; } elseif(filter_var($forward, FILTER_VALIDATE_IP)) { $ip1 = $forward; } else { $ip1 = $remote; } return $ip1; } I'm trying to Use the line in function 1 $this->ip = getenv("REMOTE_ADDR"); and replace getenv("REMOTE_ADDR"); with the function output value of Function 2
-
Thank you, I figured another way aswell just now. I added another field in my database named rank(numbers) and add a a new value each time into the field each time I create a topic and changed my display loop to work with the rank instead of the topics. But I'm going to use the above code
-
So there isn't a way to sort it by ID? Here Is my current display page... 100%!!! - Edit Topic | Add Descr | DEL * Test description - Edit Descr | Del barand - Edit Topic | Add Descr | DEL * test 2 - Edit Descr | Del * test - Edit Descr | Del Hahaha - Edit Topic | Add Descr | DEL * ???? - Edit Descr | Del jogom - Edit Topic | Add Descr | DEL * naaaaa - Edit Descr | Del moo - Edit Topic | Add Descr | DEL * meee - Edit Descr | Del Test Heading - Edit Topic | Add Descr | DEL * Hmmmm""" - Edit Descr | Del * Test - Edit Descr | Del Database Table Layout ID Topic Description Date 2 Test Heading Test 1442826471 3 100%!!! Test description 1442826546 8 Test Heading Hmmmm""" 1442826530 9 Hahaha ???? 1442827332 11 moo meee 1442842709 12 jogom naaaaa 1442843031 13 barand test 1442845391 14 barand test 2 1442845636 I want it to show like barand - Edit Topic | Add Descr | DEL * test - Edit Descr | Del * test 2 - Edit Descr | Del jogom - Edit Topic | Add Descr | DEL * naaaaa - Edit Descr | Del moo - Edit Topic | Add Descr | DEL * meee - Edit Descr | Del Hahaha - Edit Topic | Add Descr | DEL * ???? - Edit Descr | Del Test Heading - Edit Topic | Add Descr | DEL * Hmmmm""" - Edit Descr | Del * Test - Edit Descr | Del 100%!!! - Edit Topic | Add Descr | DEL * Test description - Edit Descr | Del So basically I want my topics column to be ordered DESC according to ID and my News column ordered ASC
-
The thing is it still order the topics column first, I want to sort the ID column first and then the topics column, but if I change it to ORDER BY id DESC, topic my topics and descriptions isn't grouped no more?
-
Thank you @Barand I got it working $sql = "SELECT * FROM News ORDER BY topic"; if (in_array($testip, $admin)) { echo $addnew . "<br><br>"; $lasttopic = ''; while($myrow = mysql_fetch_array($result1)) { $id = $myrow["id"]; $topic = $myrow["topic"]; if ( $lasttopic != $myrow['topic'] ) { print "<b>" . $myrow['topic'] . "</b> - " . $Edittopic . " | " . $adddescr . " | " . $deletetopic . "<br>"; $lasttopic = $myrow['topic']; } print "<p>* " . $myrow['news'] . " - " . $Editnews . " | " . $delete . "<br></p>"; } Just a question since im ordering it by Topic how will I add Descending order by id to show the latest topic and description at the top?
-
Any assistance please?
-
I used 1 and 2 as the topics and 99 and 100 as the description of 1(topic) and 1000 and 2000 description of 2(topic)
-
I'm getting the output How to show it as 1 * 99 * 100 2 * 1000 * 2000 $sql = "SELECT * FROM News ORDER BY topic"; $result1 = mysql_query($sql, $db)or die($sql."<br/><br/>".mysql_error()); while($myrow = mysql_fetch_array($result1)) { print $myrow['topic'] . " - " . $Editnews . "<br>"; print $myrow['news'] . " - " . $Editnews. "<br>"; }
-
Hi I have the following fields in my database table id - int topic - varchar news - varchar What im trying to do is group the topics ex topic1 - 123 topic2 - 987 topic1 - 456 topic2 - 654 I want to show it as Topic 1 * 123 * 456 Topic 2 * 987 * 654 seems like I'm going wrong somewhere... $sql = "SELECT * FROM News GROUP BY topic"; $result1 = mysql_query($sql, $db)or die($sql."<br/><br/>".mysql_error()); while($myrow = mysql_fetch_array($result1)) { print $myrow['topic'] . " - " . $Editnews . "<br>"; print $myrow['news'] . " - " . $Editnews. "<br>"; }
-
Hi I'm trying to find a script where I can create my own topics and descriptions to use as a news page or notification board with the layout like Heading 1 - Edit | Delete | Down Description 1 - Edit | Delete | Down Description 2 - Edit | Delete | Up | Down etc... Heading 2 - Edit | Delete | Up Description 1 - Edit | Delete | Down Description 2 - Edit | Delete | Up | Down etc... etc... It should be able to add as many headings and descriptions as you wish ad an option next to each one to edit the heading or description Does anyone know of a sortlike script?
-
Hi I'm trying to create a mute function My Database table layout: ID int AI word varchar 30 I got 260 words in my table now I'm trying to check my $_POST['message'] string to see if any of these words are in the string and if it does it should insert a 10 min mute $resultmute = mysql_query("SELECT word FROM StringyChat_WordBan") or die(mysql_error()); $rowmute = mysql_fetch_assoc($resultmute); if (stristr($message,$rowmute['word']) !== false) { $expire = time() + (60 * 10); $querymute = "UPDATE timeban SET user='$name', bantime='$expire', banby='ADMIN' WHERE mxitid='".$mxitid."'"; mysql_query($querymute); } The current problem I have is I want to check case insensitive characters. For instance if I got the word "badwords" in the database and the word is spelled "Badwords" it should still be considered a bad word. At the moment it seems like only certain words in my database get filtered and the rest not... My second question is regarding the replacing of the word with this function function filterBadWords($str) { $result1 = mysql_query("SELECT word FROM StringyChat_WordBan") or die(mysql_error()); $replacements = "x"; while($row = mysql_fetch_assoc($result1)) { $str = preg_replace('/\b' . $row['word'].'\b/i', ':-x', $str); } return $str; } Is there a way to check if theres another letter next to the word and if so not to filter it. for example assassinate will be filtered to :-x:-xinate but I want to check if there is some character at the end or the beginning of the same word not to filter it...
-
Hi I'm currently having a syntex error with my bad words filter function function filterBadWords($str) { $result1 = mysql_query("SELECT word FROM StringyChat_WordBan") or die(mysql_error()); $replacements = "x"; while($row = mysql_fetch_assoc($result1)) { $str = preg_replace('/\b' . $row['word'].'\b/ie', ':-x', $str); } return $str; } I know its the :-x part causing the problem but how can I fix it? and is there maybe a better way to do the filter because if I got the word "ass" in my list it will replace assassinate to :-x :-xinate ?
-
Ah thank you after making a few changes I got it working $marray = array( '1' => 'apple', '2' => 'orange', '3' => ' ', '4' => ' ', '5' => 'apple'); $tmp = array_filter(array_map('trim', $marray)); // remove blanks $str = ''; foreach ($tmp as $k=>$v) { $str .= "$k. $v, "; } $mrules = "Welcome Moderator please read the following rules. " . $str; ///=> Welcome Moderator please read the following rules. 1. apple, 2. orange, 5. apple,
-
Hi I'm trying to create an array to put it into a string like the following $marray = array( '1' => 'apple,', '2' => 'orange,', '3' => ' ', '4' => ' ', '5' => 'apple'); And the string should look like $mrules = "Welcome Moderator please read the following rules. " . 1. Apple, 2. Orange, 5. Apple. The empty values in the array shouldn't be showed any assistance please
-
Compare tables and update 2nd table with new value
cobusbo replied to cobusbo's topic in PHP Coding Help
Thank you that solved the problem just learned something new with this query -
Compare tables and update 2nd table with new value
cobusbo replied to cobusbo's topic in PHP Coding Help
I tried it $updatename = "UPDATE pm INNER JOIN Users2 u ON pm u.mxitid = pm.ip SET pm.username = u.Username"; mysql_query($updatename) or die("Error: ".mysql_error()); getting error -
Hi I'm trying to compare two tables with each other and update the 2nd table with the new updated name from table 1 via their unique identical ID $resultus = mysql_query("SELECT * FROM Users2"); $rowus = mysql_fetch_array($resultus); $naamus = urldecode($rowus['Username']); $mxitus = $rowus['mxitid']; $resultnup = mysql_query("SELECT * FROM pm"); $rownup = mysql_fetch_array($resultnup); $naamup = $rownup['username']; $ipup = $rownup['ip']; while($ipup == $mxitus){ $updatename = "UPDATE pm SET username= \"$naamus\" WHERE ip = \"$mxitus\""; mysql_query($updatename) or die("Error: ".mysql_error()); } The $mxitus and $ipup is the 2 fields that would be identical in both tables now Im trying to update the pm table username field with the naamus field from Users 2 any assistance please?
-
Never mind did some further research and found that read is a reserved word had to change it to `read`
-
$read1 = "unread"; $query = "INSERT INTO pm (username,mxitid,message,time,read,ip) VALUES (\"$naam\",\"$ip1\",\"$message\",\"$date\",\"$read1\",\"$banby2\")"; I'm getting the error This only happened when I added the read and value $read I got the field named read in my database.... any suggestion where im going wrong? If I remove the read field the rest of the query works 100%
-
Upload resize and convert images turn black help
cobusbo replied to cobusbo's topic in PHP Coding Help
I used my file Ext with this function all seems to be working fine now thank you $imageTypeArray = array ( 0=>'UNKNOWN', 1=>'GIF', 2=>'JPEG', 3=>'PNG', 4=>'SWF', 5=>'PSD', 6=>'BMP', 7=>'TIFF_II', 8=>'TIFF_MM', 9=>'JPC', 10=>'JP2', 11=>'JPX', 12=>'JB2', 13=>'SWC', 14=>'IFF', 15=>'WBMP', 16=>'XBM', 17=>'ICO', 18=>'COUNT' ); $size = getimagesize($filename); $size[2] = $imageTypeArray[$size[2]]; My new file looks like <?php include $_SERVER['DOCUMENT_ROOT'] . '/chat2/chat_code_header.php'; $ip = $_SERVER["HTTP_X_MXIT_USERID_R"]; if(!isset($ip)) { $ip = "Debater"; } $result9 = mysql_query("SELECT * FROM Users2 WHERE mxitid = \"$ip\""); $row = mysql_fetch_array($result9); $id = $row['ID']; // Access the $_FILES global variable for this specific file being uploaded // and create local PHP variables from the $_FILES array of information $fileName = $_FILES["uploaded_file"]["name"]; // The file name $fileTmpLoc = $_FILES["uploaded_file"]["tmp_name"]; // File in the PHP tmp folder $fileType = $_FILES["uploaded_file"]["type"]; // The type of file it is $fileSize = $_FILES["uploaded_file"]["size"]; // File size in bytes $fileErrorMsg = $_FILES["uploaded_file"]["error"]; // 0 for false... and 1 for true $fileName = preg_replace('#[^a-z.0-9]#i', '', $fileName); // filter the $filename $kaboom = explode(".", $fileName); // Split file name into an array using the dot $imageTypeArray = array ( 0=>'UNKNOWN', 1=>'GIF', 2=>'JPEG', 3=>'PNG', 4=>'SWF', 5=>'PSD', 6=>'BMP', 7=>'TIFF_II', 8=>'TIFF_MM', 9=>'JPC', 10=>'JP2', 11=>'JPX', 12=>'JB2', 13=>'SWC', 14=>'IFF', 15=>'WBMP', 16=>'XBM', 17=>'ICO', 18=>'COUNT' ); $size = getimagesize($fileTmpLoc); $size[2] = $imageTypeArray[$size[2]]; $fileExt = $size[2]; // Now target the last array element to get the file extension // START PHP Image Upload Error Handling -------------------------------- if (!$fileTmpLoc) { // if file not chosen echo "ERROR: Please browse for a file before clicking the upload button."; exit(); } else if($fileSize > 5242880) { // if file size is larger than 5 Megabytes echo "ERROR: Your file was larger than 5 Megabytes in size."; unlink($fileTmpLoc); // Remove the uploaded file from the PHP temp folder exit(); } else if (!preg_match("/.(gif|jpg|png)$/i", $fileName) ) { // This condition is only if you wish to allow uploading of specific file types echo "ERROR: Your image was not .gif, .jpg, or .png."; unlink($fileTmpLoc); // Remove the uploaded file from the PHP temp folder exit(); } else if ($fileErrorMsg == 1) { // if file upload error key is equal to 1 echo "ERROR: An error occured while processing the file. Try again."; exit(); } // END PHP Image Upload Error Handling ---------------------------------- // Place it into your "uploads" folder mow using the move_uploaded_file() function $moveResult = move_uploaded_file($fileTmpLoc, "images/".$id.".".$fileExt); // Check to make sure the move result is true before continuing if ($moveResult != true) { echo "ERROR: File not uploaded. Try again."; exit(); } // Include the file that houses all of our custom image functions include_once("ak_php_img_lib_1.0.php"); // ---------- Start Universal Image Resizing Function -------- $target_file = "images/".$id.".".$fileExt; $resized_file = "images/resized_".$id.".".$fileExt; $wmax = 320; $hmax = 240; ak_img_resize($target_file, $resized_file, $wmax, $hmax, $fileExt); unlink($target_file); // ----------- End Universal Image Resizing Function ---------- // ---------- Start Convert to JPG Function -------- if (strtolower($fileExt) != "jpg") { $target_file = "images/resized_".$id.".".$fileExt; $new_jpg = "images/resized_".$id.".jpg"; ak_img_convert_to_jpg($target_file, $new_jpg, $fileExt); unlink($target_file); } $new_jpg = "images/resized_".$id.".jpg"; if(!get_magic_quotes_gpc()) { $new_jpg = addslashes($new_jpg); $filePath = addslashes($filePath); } $result = mysql_query("UPDATE Users2 SET pprofilepic='$new_jpg', aprove='requested' WHERE mxitid='$ip'") or die(mysql_error()); // ----------- End Convert to JPG Function ----------- // Display things to the page so you can see what is happening for testing purposes echo "The file named <strong>$fileName</strong> uploaded successfuly.<br /><br />"; echo "It is <strong>$fileSize</strong> bytes in size.<br /><br />"; echo "It is an <strong>$fileType</strong> type of file.<br /><br />"; echo "The file extension is <strong>$fileExt</strong><br /><br />"; echo "The Error Message output for this upload is: $fileErrorMsg"; if($result){ header('Location: ../profile/thankyou.php'); } else { echo "ERROR"; } // close mysql mysql_close(); ?> -
Upload resize and convert images turn black help
cobusbo replied to cobusbo's topic in PHP Coding Help
Isn't there a way to change the file type to the right one before processing it? because doing it manually is a pain? -
Upload resize and convert images turn black help
cobusbo replied to cobusbo's topic in PHP Coding Help
This is the image I tried to upload attached how should I go on fixing the problem because it seems all png files bigger than 100kb end up being black after the resizing aswell