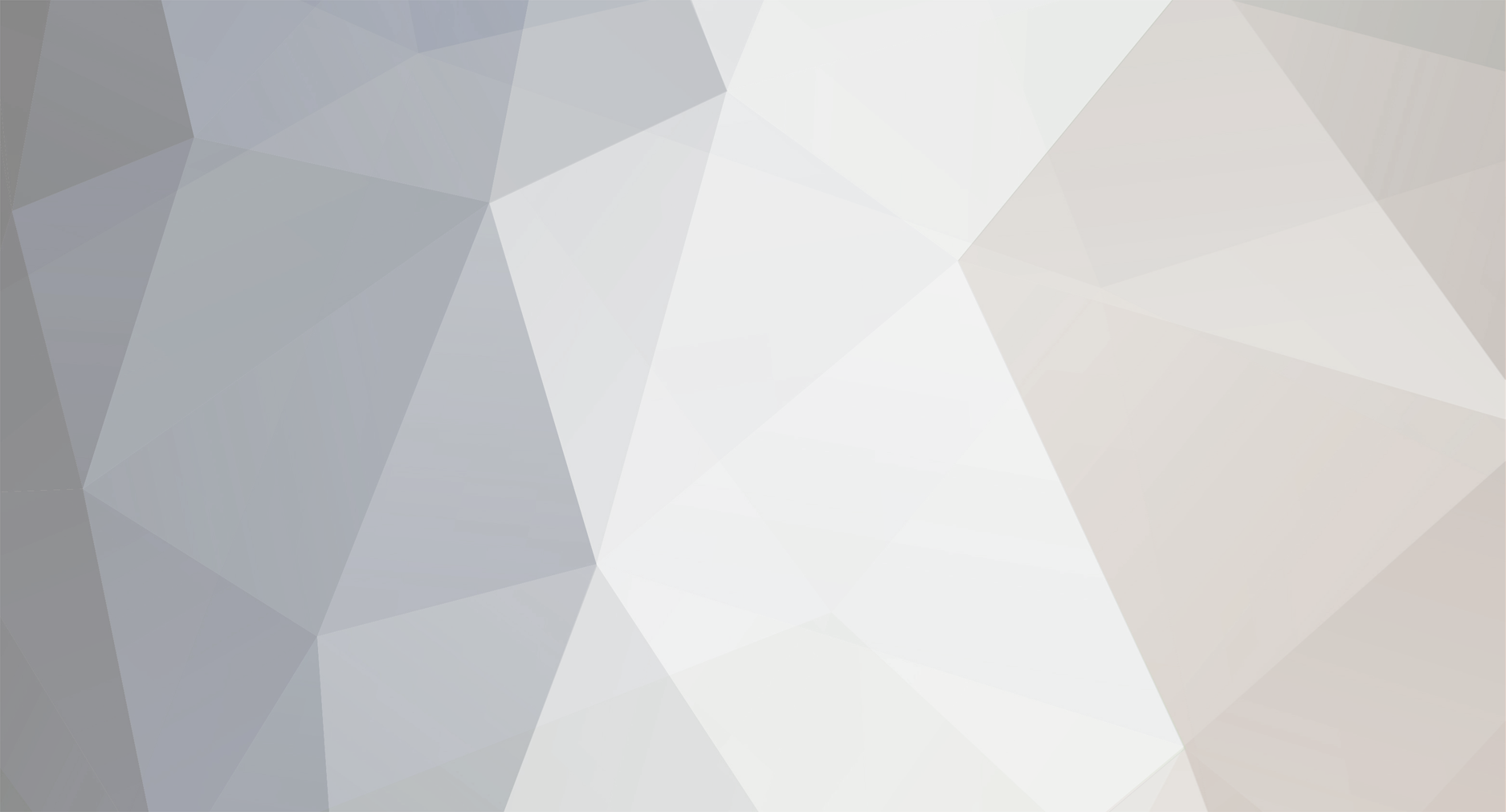
obsidian
Staff Alumni-
Posts
3,202 -
Joined
-
Last visited
Everything posted by obsidian
-
Look closely at these lines you posted: $_xml .='\t<marker address="'.$row['start_street'].'" postcode="'.$row['start_postcode'].'"; $_xml .=" lat="'.$row['start_lat'].'" lng="'.$row['start_long'].'" type="'.$row['start_type'].'">"; $_xml .="\t</marker>\r\n"; Your quotes are off, and they could well be causing issues. Try this instead: <?php $_xml .= "\t<marker address=\"{$row['start_street']}\" postcode=\"{$row['start_postcode']}"; $_xml .= " lat=\"{$row['start_lat']}\" lng=\"{$row['start_long']}\" type=\"{$row['start_type']}\">"; $_xml .= "\t</marker>\r\n"; ?> Check the rest of your strings, too, just to make sure your quotes aren't off like these were.
-
Well, I'm not positive what "code" you are referring to, but there are a couple ways to alternate things. For instance, if you are wanting to alternate row colors, you'd simply have to do something like this: <?php $class = 'even'; while ($row = mysql_fetch_assoc($res)) { $class = $class == 'even' ? 'odd' : 'even'; // Here's where the magic happens echo "<tr class=\"$class\">\n"; // Display your table record here echo "</tr>\n"; } ?> However, if you are actually looking at handling the PHP differently for the separate records, you may want to try something like this: <?php $i = 0; while ($row = mysql_fetch_assoc($res)) { ++$i; if ($i % 2 == 0) // Here are your even records { } else // Here are your odd records { } } ?> Hope this helps!
-
Get on Facebook or Myspace (*shudder*) and search for people in the networks in which you are interested in conversing. I have had a similar interest, and I have been able to make some contacts at MS Game Studio, 3 Rings, and Acclaim as well as a contact at Google. I'm sure if you are persistent enough, you'll be able to get some contacts. Keep in mind that their time is money, and they're not going to want to just "shoot the breeze" with you, but I've found that most people in roles such as those of which you speak are more than willing to discuss valid questions if you present yourself in an intelligible way and are willing to wait for a response. Another place to start building connections is http://www.linkedin.com. I'm not a big fan of social networking sites, but they can indeed get you some legitimate contacts when used appropriately. Good luck.
-
Make note of the FORMAT of the DATETIME field. It accepts YYYY-MM-DD HH:MM:SS as the value, so you will need to convert and combine you existing data in order to get it to fit. This is definitely worth the effort.
-
You just need to properly sanitize your user input. For instance, if you are expecting a username to be 20 characters containing alphanumeric characters with dashes and underscores, you can just match it against a regular expression like /^[\da-z-_]{6,20}$/ before you throw it into your query. If you do need to allow for characters that could be used for SQL injection attempts, just be sure to properly escape the variables using mysql_real_escape_string(). That's a great start, at least.
-
SQL injection is possible any time you are using unsanitized user input in a query. Whether that comes from $_GET, $_POST or something else entirely is up to you and your script. As to whether it is possible in $_GET: yes, if you are using $_GET variables directly in a query without cleaning them up.
-
Because you are using pg_query, it simply is returning a resource, so you need to do a comparison like this: <?php if (pg_num_rows($result) > 0) { // Records returned } ?>
-
What are you doing with the string? If you are writing this to a file in order to create an Excel readable CSV file, check out the fgetcsv() and fputcsv() functions. Otherwise, you'd need something like this: <?php $arr = array('0010', '0020', '0030', '0040', '0050', '0060'); foreach ($arr as $k => $v) { $arr[$k] = '"{$v}"'; } echo implode(',' $arr); ?>
-
I got around 70 on this one. It's a bit frustrating, because I'm in the habit of hitting backspace any time I know I've made a mistake, so that logs me with another error lol.
-
You won't know how many people subscribe since that is simply a local setting in their application. All you can do is do an IP tracking or other method of figuring out your unique visitors, but as far as subscriptions, that won't be possible.
-
Right. Create a PHP page that checks the session first and then sets the header('Content-type: text/xml') so that readers will acknowledge the valid XML document.
-
There's not much to critique yet, but as for layout, it looks fine, and all the sections are lining up appropriately. Win XP Pro SP2 FF2.x
-
If you are using a DATE data type for stock_Date, it is already in the YYYY-MM-DD format by default. If, to be sure, you wrap the column in the DATE() function as I have in my previous post, it will assure that you have it in the YYYY-MM-DD format like your $dt variable. You could even just do it all with MySQL: SELECT * FROM pls WHERE stock_DATE = CURDATE();
-
Try something like this: <?php $query = mysql_query("SELECT * FROM pls WHERE DATE(stock_Date) = '" . date('Y-m-d') . "'"); ?> The DATE() function around stock_Date might not be needed, but I placed it there because I don't know if your data type is DATE or DATETIME.
-
In and of itself, that sets the variable something but doesn't assign a value to it, so it's pretty much worthless. If you are attempting to assign multiple values to a single variable, you may want to do something like this: URL: index.php?cat=1,2,3,4 <?php $cats = explode(',', $_GET['cat']); ?>
-
1. Yes. Try it out. Every time you run the assignment operator on a variable, you effectively overwrite the previous value. 2. First off, to retrieve information from the URL, you need to use the $_GET global. This is an array that is set up in PHP to automatically return any parameters passed via the URL. However, based on your sample, I would definitely encourage you to never pass user information such as password, SSN, etc through the URL. Always use POST (and preferably a secure server for the SSN and any other personal info). Check out this page of the PHP manual for details on the predefined variables including $_GET.
-
You need to check into some basic regular expressions matching. Here is an email matcher that is taken from this reference site. <?php if (!preg_match('|^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$|i', $email)) { // Not a valid email address } ?>
-
Ugh... my stupidity on that one. Try this as a fix: <?php $seed = "February 28, 2008"; $start = strtotime($seed); $end = strtotime("March 28, 2008"); $i = 0; while ($start < $end) { echo date('F j, Y', $start) . "<br />\n"; $i++; $start = strtotime("{$seed} +{$i} days"); } ?>
-
How about this: <?php $seed = "February 28, 2008"; $start = strtotime($seed); $end = strtotime("March 28, 2008"); $i = 0; while ($start <= $end) { $start = strtotime("{$seed} +{$i} days"); echo date('F j, Y', $start) . "<br />\n"; $i++; } ?>
-
Step #1: Change your dates to UNIX timestamps: <?php $hkLongDate = strtotime("02/25/2008"); $vtLongDate = strtotime("02/27/2008"); $totLongDate = strtotime("02/29/2008"); ?> Step #2: Create an associative array to tie the elements to their respective times: <?php $eles = array( array('time' => $hkLongDate, 'item' => $hkList), array('time' => $vtLongDate, 'item' => $vtList), array('time' => $totLongDate, 'item' => $totList) ); ?> Step #3: Sort the list based on the time and output: <?php // Get the columns for multisort foreach ($eles as $key => $row) { $times[$key] = $row['time']; $items[$key] = $row['item']; } array_multisort($times, SORT_ASC, $items, SORT_ASC, $eles); // Check the order: echo "<pre>\n"; print_r($eles); echo "</pre>\n"; ?>
-
Hard to say why checkdate allows that, but a much easier way to do what you are attempting is as follows. Now, this is assuming, based on your current code, that the year is in YYYY-MM-DD format (or any other human readable format, for that matter): <?php $date = isset($_GET['year']) ? $_GET['year'] : NULL; // default to null if (FALSE == ($ts = strtotime($date))) { // Invalid date provided } else { // Good to go! } ?>
-
You may need to share some code with us. To use checkdate(), you are required to provide a month, day and year. If the user is simply providing a year, you could get by with a much simpler check to see that their provided year contains only digits (or whatever). Then, you can build your dates and possibly use strtotime() for an easier comparison depending on what you are trying to do.
-
Not built in, no. Keep in mind that simply calling bold on a font is actually a different font set. For instance, with Arial, you have the plain arial.ttf file for normal font face, but for bold, you actually have arialbd.ttf. For italic, there is ariali.ttf and for bold-italic, there is arialbi.ttf. You would actually have to parse out the text, choose which font will accurately represent what you are after and continue your line with the new font. Do a Google search, and maybe there are some classes or tools out there that may help you along to getting where you want to be. Sounds like a very major undertaking to me, though. Good luck with it!
-
Your code seems to work just fine for me, unless you are actually wanting the HTML to be interpreted within your image? Are you wanting that text to be bold? If so, you'll have to build a full parser and select a bold font face for those areas where you want bold, etc. Check out the options for using TTF with this function: http://us3.php.net/imagettftext[/ur].
-
No, you're absolutely right. Within the processing script, you should have a predefined list of functions that can (and will) be performed by the script if the arguments are right. Another idea is to base64encode() all your arguments so that at a glance, they're a little more difficult to manipulate.