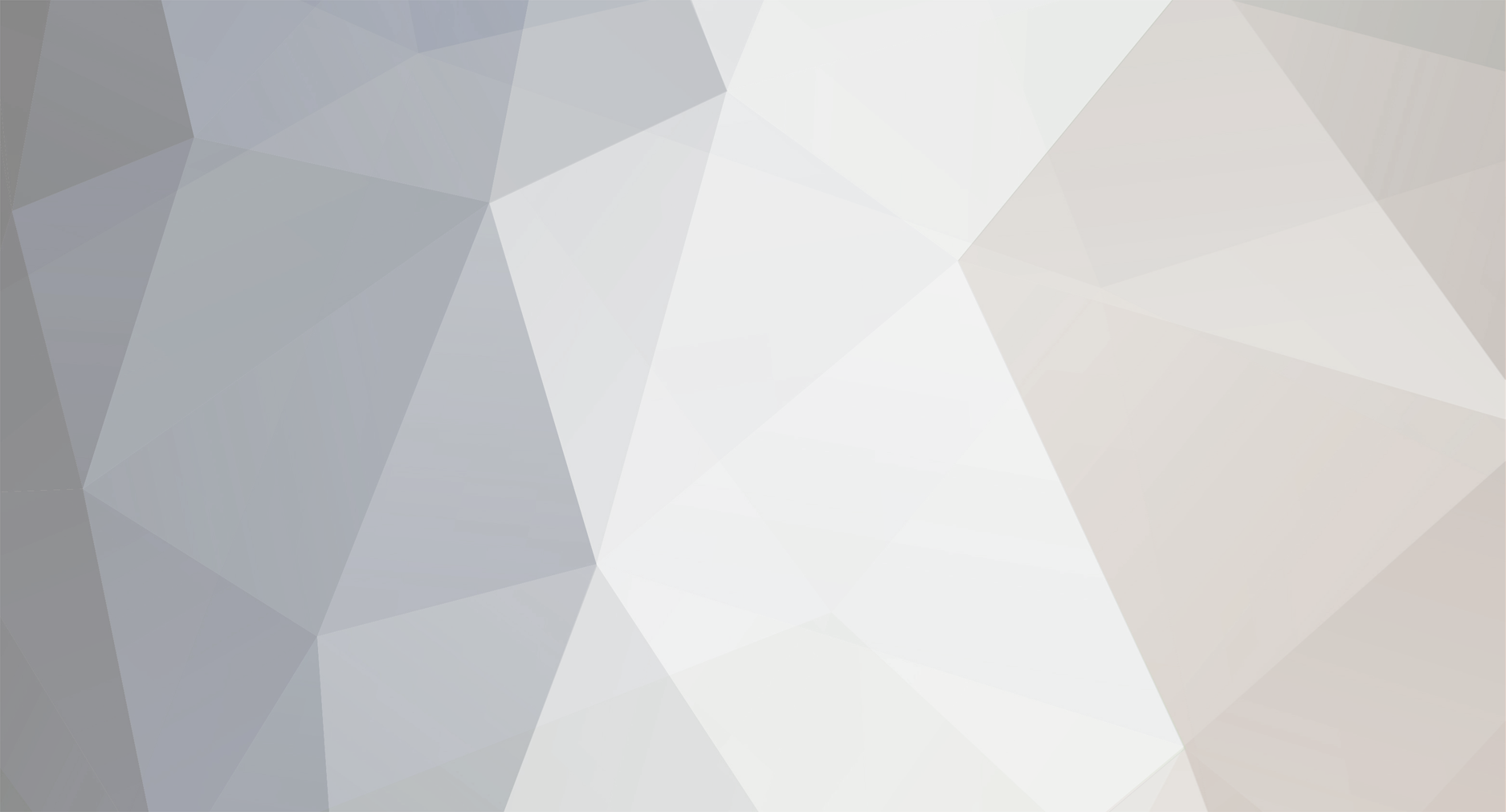
obsidian
Staff Alumni-
Posts
3,202 -
Joined
-
Last visited
Everything posted by obsidian
-
Yes, but you could even do unsigned for your ids to allow for even more room: CREATE TABLE friend_requests( from_id integer unsigned REFERENCES users(user_id), to_id integer unsigned REFERENCES users(user_id), status ENUM('pending', 'approved', 'denied') DEFAULT 'pending', PRIMARY KEY(from_id, to_id) );
-
Here is how I have set up this sort of thing in the past: USERS: ========== user_id username etc... FRIEND_REQUESTS: ================ from_id -- references USERS(user_id) to_id -- references USERS(user_id) status ENUM('pending', 'approved', 'denied') PRIMARY KEY(from_id, to_id) With this sort of setup, you can easily query someone's pending friend requests, but you also have control on how to treat the different requests based on their status. There is a ton more you can do with this type of thing, but hopefully this at least gives you a head start on it.
-
I think your choice of terms between "table" and "database" may not be clear. Typically speaking, a given application will be run from a single database with multiple tables. Your tables need to be normalized as much as possible, which will then require joining tables to gather your data.
-
As I mentioned before, I am not trying to convince someone to change their opinions about something as much as trying to help developers hone their skills and have an understanding of the different options. Now, to answer your question, yes, in most cases it is much better to use the appropriate tools for the job, and it is not always simply options A and B to consider. Consider the date and time function limitations within PHP before 5.1, for instance. With those limitations, a user can only handle dates from 1970 through until the present, because the negative integers were not handled appropriately to calculate back before the UNIX epoch. Now, with your recommended solution, no issues are encountered in the processing of an ordering script because people can obviously not place an order in the past. So, what happens when a user gets into the habit of that method and then has to deal with ages on a PHP4 install? Then, like it or not, they will need to know of another appropriate tool to use. In this case, we have a tool designed specifically for date and time calculations. Besides the obvious limitation differences between the two methods, we come to the actual optimization process. Grant it, as someone is learning, the speed or optimization of a script is not priority #1, but if you ever get working on a site with millions of records and page hits, optimization becomes key, and when you are having to query for the data initially and then translate it with PHP, you'll find by running benchmark testing that in most cases, using the MySQL date/time functions, you will save yourself processing time since you can simply dump the results rather than having to parse them a second time. In summary, and as I mentioned in my previous post, they are both legitimate ways to handle the problem at hand, but part of becoming proficient at any language is understanding where the limitations are or where tweaks can be made to optimize for a given situation. Hope this helps clarify
-
You actually don't need the "FROM" clause, since you would have already selected a DB to use. Try this: <?php $sql = mysql_query("SHOW TABLES"); while ($row = mysql_fetch_row($sql)) { echo $row[0] . "<br />\n"; } ?> Hope that helps.
-
I agree that it may not always be the best, but it goes beyond opinion when you look at processing and optimization of scripts. What I am recommending is for someone to make themselves a better coder by learning to use the tools properly, and then they can make their own decision on how to do it based on each individual situation.
-
I would disagree with that sentiment. There is a reason that MySQL has date and time types. It's much better off to learn the tools and use them as intended. It will really save you time in the long run.
-
That is a DATETIME column. So, to display it with PHP, you could just do this: <?php $sql = mysql_query("SELECT date_column FROM myTable"); while ($row = mysql_fetch_assoc($sql)) { $formatted = date('Y-m-d', strtotime($row['date_column'])); echo "$formatted<br />\n"; } ?> ...or, let MySQL do the formatting... <?php $sql = mysql_query("SELECT DATE_FORMAT(date_column,'%c/%e/%Y') AS formatted FROM my_table"); while ($row = mysql_fetch_assoc($sql)) { echo "{$row['formatted']}<br />\n"; } ?>
-
What is the actual data type in the database? Is it a true DATE or DATETIME field? If so, you can either use the DATE_FORMAT function within MySQL to retrieve it in the desired format, or you can do what I showed in the first post to the raw returned value.
-
Just an FYI, you don't need to do a trim within the empty() call, since empty interprets empty space as well.
-
By default, the date fields in mysql are stored as YYYY-MM-DD, but any human readable format can be translated via the strtotime() function: <?php $ts = '2008-04-09'; echo date('m/d/Y', strtotime($ts)); ?>
-
[SOLVED] Need help grouping information from three tables
obsidian replied to zsazsageorge's topic in MySQL Help
That's what we're here for. You would do a similar join, but you would only have to do the join on two tables this time: SELECT b.author, b.title, r.review FROM books b INNER JOIN book_reviews r ON b.isbn = r.isbn Good luck! -
ENUM really wouldn't be the best way to go. If you are wanting the value to be treated as a number, you need to have a numeric type on the column. I would recommend simply having a float type with a DEFAULT of 6.25. If you do that, then you just don't have to insert a value into that column at all, and you can run aggregate functions on it (such as SUM).
-
[SOLVED] Need help grouping information from three tables
obsidian replied to zsazsageorge's topic in MySQL Help
Excellent. You actually need to have all 4 tables to get your user tied to the book title and author. Run this query in MySql query browser and see what you get: SELECT c.name, b.title, b.author FROM customers c INNER JOIN orders o ON c.customerid = o.customerid INNER JOIN order_items i ON o.orderid = i.orderid INNER JOIN books b ON i.isbn = b.isbn That should give you a list of customer names along with the author and title of the books they purchased. You may need to double check the capitalization of the tables and fields in the query as I left them all as lower case, but I hope this helps! -
Read this overview of SMTP injection[/ur]. If you don't sanitize your user inputs properly, they can actually overwrite hard coded headers because of the way the mail function processes. This article should give you some ideas on how to be sure to keep from being subject to SMTP injection.
-
FYI, as the manual suggests, the function is deprecated, but you can still run a mysql_query() function call: SHOW TABLES FROM db_name
-
[SOLVED] Need help grouping information from three tables
obsidian replied to zsazsageorge's topic in MySQL Help
Is there a table (something like order details) that actually shows which ISBN or book ID was purchased in a given order? Without that, it is impossible to tie the book back to the order. That has to be done in order to know who purchased each book... -
Usually, for something you're going to be querying against later, you would want to have a joining table that has the individual hobby interests in it: Users: user_id username etc... Hobbies: hobby_id title description etc... User_Hobbies: user_id hobby_id With that kind of table structure, you could pull all users with a specific interest by just doing a query on the hobby index in the User_Hobbies table. This is much less intensive than using a LIKE on a text column since it won't require a full table scan to complete (especially if it's indexed correctly). In addition, your table structure is more normalized, too ;-)
-
Also, check to see that you have the fopen URL wrappers turned on. If not, you won't be able to use getimagesize (or any other like functions) to open the source location via a URL.
-
Ditto the previous remarks. I, too, have often found their "solutions" at the top of my Google searches, but I'm not about to pay someone for a solution that I can't be guaranteed will fit my need anyway. That's one of my biggest gripes about them is that for any given question, there can be a myriad of answers, and when I can browse multiple answers elsewhere and choose the best for my needs, I'm not going to pay them to give me what they deem to be what I want to read.
-
Yes, but not directly. You have to go through a gateway that can resolve the phone number and pass the SMS message on to the phone. Check out this article on the matter for an idea. Another option would be to look at this list of provider gateways and send them as email via PHP instead of trying to hit the SMS gateway directly.
-
Templates Ugh... I can't stand using Dreamweaver templates. When you make a modification to one of the template components, Dreamweaver literally has to touch every single file and update the contents of that file, and then you have to push all the updated files to the server. That is such a wasted effort when you can simply use PHP includes or a true templating system, such as Smarty, to only have to change the content in one location. Period.
-
You don't need regexp at all for that. If you have all the functions in an array, just set up a matching replacement array like this and run str_replace on the whole string: <?php $functions = array('abs', 'random'); $replace = array(); foreach ($functions as $f) { $replace[] = '<b>' . $f . '</b>'; } $new_string = str_replace($functions, $replace, $old_string); echo $new_string; ?>
-
I use WAMP for dev, since my laptop runs XP, but my hosting is all on LAMP.
-
There is already a function that does this. Read up on highlight_string() and highlight_file(). <?php $string = "<?php echo 'Hello, world!'; ?>"; highlight_string($string); ?>