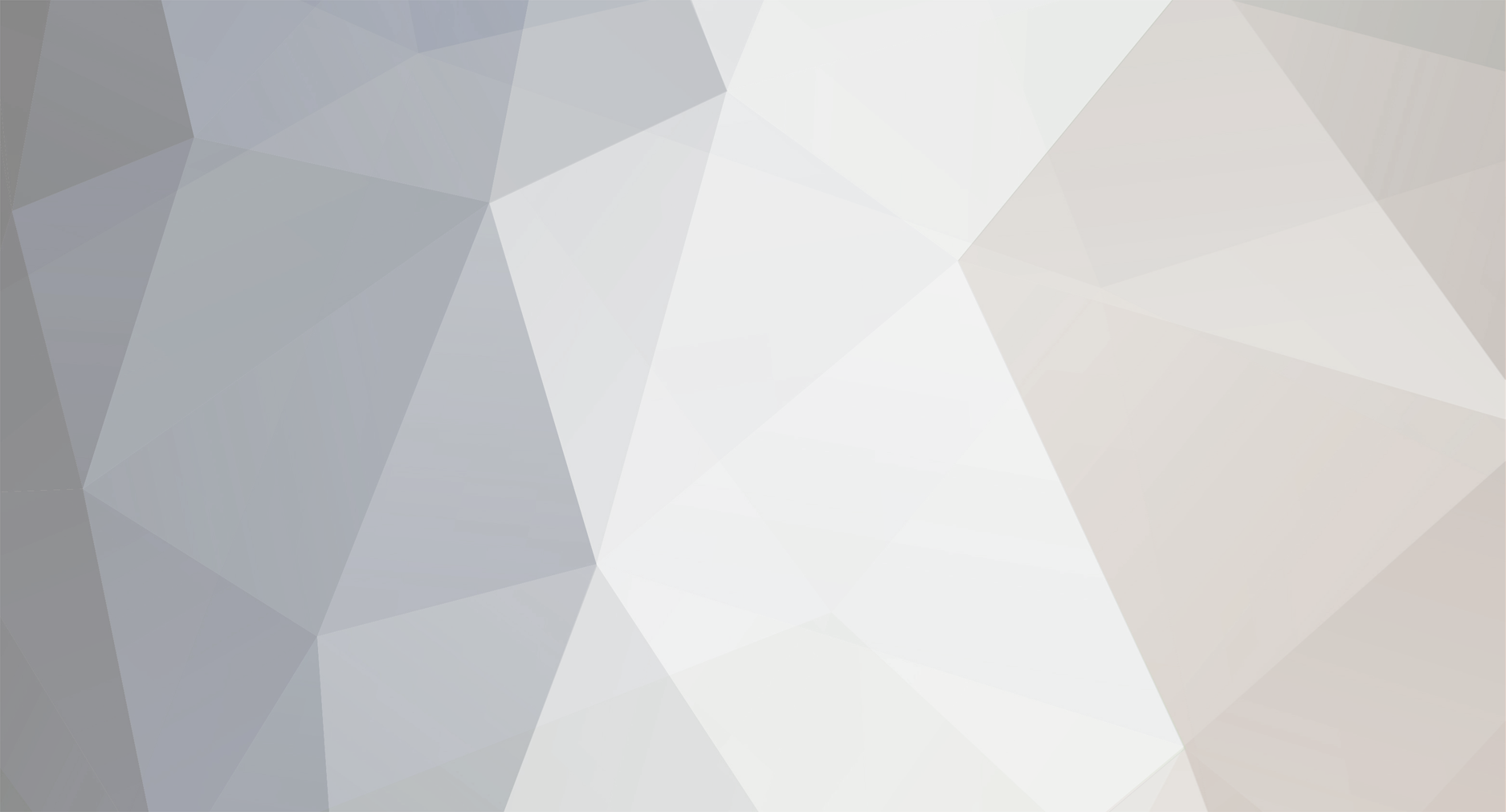
wildteen88
Staff Alumni-
Posts
10,480 -
Joined
-
Last visited
Never
Everything posted by wildteen88
-
In sessions.php simply do: <?php // always call session_start() before using sessions session_start(); // add all $_POST data to session $_SESSION = $_POST; ?> Data saved to session! <a href="session2.php">Display Session</a> Now create another file, call this session2.php and add the following code: <?php session_start(); echo 'Your name is <b>' . $_SESSION['name'] . '</b> and you are <b>' . $_SESSION['gender'] . '</b><br />'; echo 'You have <b>' . ( ($_SESSION['purpose'] == 'YES') ? 'a' ? 'no' ) . '</b> purpose here<br />'; echo 'Your favourite programming language is <b>' . $_SESSION['language'] . '</b>'; ?>
-
Hi, sorry I haven't replied. I have modified the script a little to how I think you wanted: <?php function keywordSearch(&$keywords) { global $keyword_count; $lines = file('search_data.txt'); $results = false; $symbols = array('[', ']', '-', '(', ')'); $replace = array('\[', '\]', '\-', '\(', '\)'); $colors = array('#FF0000', '#0000FF', '#00FF00', '#FFFF00'); $i = 0; foreach($lines as $line) { $keyword_found = false; foreach($keywords as $key => $keyword) { if(!isset($keyword_count[$keyword])) { $keyword_count[$keyword] = 0; } $keyword = str_replace($symbols, $replace, $keyword); if(eregi($keyword, $line)) { $keyword_found = true; // remove answer from end of line $line = eregi_replace("\*([a-z0-9 ]+)", '?' , $line); $line = str_replace($keyword, "<span style=\"color: {$colors[$key]}; font-weight: bold;\">$keyword</span>", $line); $results[$i] = $line; $keyword_count[$keyword]++; } } if($keyword_found) $i++; } return $results; } function displaySearchResults() { global $keyword_results, $keywords, $keyword_count; $output = '<p>The keywords "<i><b>'. implode('</b></i>", "<i><b>', $keywords) . '</b></i>" found ' . count($keyword_results) . " result(s):\n"; $output .= "<ol>\n <li>" . implode("</li>\n <li>", $keyword_results) . "</li>\n</ol>\n"; echo "<p>$output</p>"; } if(isset($_POST['submit'])) { if(!empty($_POST['keyword'])) { $_POST['keyword'] = str_replace(array("\r\n", "\r", "\n"), "\n", $_POST['keyword']); $keywords = explode("\n", $_POST['keyword']); $keyword_results = keywordSearch($keywords); displaySearchResults(); } else { echo 'Invalid search term'; } } ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> Keywords:<br /><textarea name="keyword" cols="40" rows="4"><?php echo isset($_POST['keyword']) ? $_POST['keyword'] : null; ?></textarea> <p><input type="submit" name="submit" value="Search" /></p> </form>
-
Both are correct however there are few notes you should consider.: 1. When using associative arrays always wrap the key within quotes, eg: $arr['key'] not $arr[key] although both return the same result they are in fact completely different. If you do not wrap keys within quotes PHP will think you are using a Constant rather than string, and so if you have error_reporting set high enough you may find PHP displaying the following notice don your page: Notice: Use of undefined constant key - assumed 'key' 2. If you are using an array within a string which is an associative array wrap the variable within curly braces: echo "<b>{$arr['key']}</b>" Otherwise you may get the following error message: Parse error: syntax error, unexpected T_ENCAPSED_AND_WHITESPACE, expecting T_STRING or T_VARIABLE or T_NUM_STRING
-
You can substitute the computers IP address with localhost instead if mysql is install locally. You only need to specifiy the ip address if MySQL is install remotely on another computer/server. Also always use mysql_error() function to retrieve errors from mysql. // mysql_connect connects to the database server and returns a link to the the resource $dblink = @mysql_connect("$dbhost","$dbpass") or die("<p><b>Could not connect to database server: ($dbhost)</b><br />" . mysql_error() . "</p>\n"); // mysql_select_db selects a database to use on the database server pointers to by $dblink // the @ sign before the command supresses any error messages @mysql_select_db ($dbname , $dblink) or die ("<p><b>Could not connect to database ($dbname)</b><br />" . mysql_error() . "</p>\n");
-
admin admin admin please help hacked account........
wildteen88 replied to redarrow's topic in PHPFreaks.com Website Feedback
This is an issue we had a few weeks back when the forum where hacked. The hackers had managed to install some form of backdoor script which allowed them changed users profiles to point all links to darkmindz.com -
Move this line: $canvas = imagecreatetruecolor($canvas_width, $canvas_height); before these lines: // copy the original image onto the canvas canvas, original and top/left co-ordinates // imagecopyresampled($canvas, $original_image, 0,0,0,0, $new_image_width, $new_image_height, $image_width, $image_height); and change $canvas_width and $canvas_height variables to $new_canvas_width and $new_canvas_height respectively.
-
Turn error reporting on if you can, by adding the following two lines to the top of your script ini_set('display_errors', 'On'); error_reporting(E_ALL); If that does work, add some debugging lines into your script: <?php ini_set('display_errors', 'On'); error_reporting(E_ALL); if($secret == "56") { echo 'Quering database...<br />'; $r = mysql_fetch_array(mysql_query("SELECT * FROM gallery WHERE ID='".$_GET[id]."'")); echo '<pre>$r equals ' . print_r($r, true) . '</pre>'; echo 'Deleting ' . $r[photo] . '... '; unlink($r[photo]) or die('FAILED'); echo ' SUCCESSFUL<br>'; echo 'Deleting from Database...'; mysql_query("DELETE FROM gallery WHERE ID='".$_GET[id]."'") or die('FAILDED. ' . mysql_error()); echo 'SUCCESSFULL';. //echo "<script>window.location = 'admin.php?p=admin_gallery';</script>"; } else { $content .= "You Must Be Logged In To Do That"; }?>
-
If you are using a custom session name, then you'll need to call session_name('your_sessions_name') before you call session_start()
-
I asumme change this line: if($taskID != "Save") to if($taskID != "Save" && $empID != '--Select an Employee--') Only guessin
-
That script will not work. You cannot use variable names that start with numbers. Also no where in that script you appear to add the image to the database.
-
The unlink function will delete files yes. However if you install your script on a *nix based server your script will need to have the neccesary (chmod) permissions in order to delete files.
-
Look into using JOINS Using JOINS allows you to query multiple tables at one. Rather using multiple queries.
-
how to implement cookies
wildteen88 replied to vishallokhande's topic in PHP Installation and Configuration
What? Are you telling me that or are you answering to your own question? -
You can delete the image from the database and the filesystem at the same time. On the page that deletes the image from the database I assume you provide some form of image id, ie your link will be delete.php?image_id=xxx (xxx representing a number which is associated to the image stored in the database). If thats the case, then before you delete the image from the database then do a simple database query to return the image from the database, then delete the image from filesystem using unlink and run another query to delete the image from the database. $imageID = $_GET['image_id']; $qry = "SELECT image_path FROM image_table WHERE image_id='$imageID'"; list($image_path) = mysql_fetch_row(mysql_query($qry)); // delete image unlink($image_path); // delete image from database; mysql_query("DELETE FROM image_table WHERE image_id='$imageID'");
-
There is no issues with using if/else statements in functions. You most probably have a syntax issue which is causing your function to fail.
-
Both php set-ups are basically the same. I say go for the upgrade.
-
PHP Scripts not interpreted in Apache 2.2.2
wildteen88 replied to nicholasis23's topic in PHP Installation and Configuration
Probably your browser caching the page. If you press Ctrl + F5 whilst in your browser, it will force it to load a new instance of the page rather than the page loaded in the cache. Glad everything is working now. -
PHP Scripts not interpreted in Apache 2.2.2
wildteen88 replied to nicholasis23's topic in PHP Installation and Configuration
No, this is handled by Apache. No, PHP is compatible with Apache2.2.x I don't think so. If Norton was interfering then Apache will fail to work. Seems strange to me. There must be something else interfering. Can you link your httpd.conf and php.ini here so I can have a look. -
They are not the same, print_r is to display the contents of an array or object. read the manual on what each do.
-
Modified the code: <?php function keywordSearch($keyword) { $results = false; $lines = file('search_data.txt'); $symbols = array('[', ']', '-', '(', ')'); $replace = array('\[', '\]', '\-', '\(', '\)'); $keyword = str_replace($symbols, $replace, $keyword); foreach($lines as $line) { if(eregi($keyword, $line)) { $line = str_replace($keyword, "<b>$keyword</b>", $line); $results[] = $line; } } return $results; } function displaySearchResults() { global $keyword_results, $keyword; $output = ''; foreach($keyword_results as $keyword => $results) { $output .= '<p>The search term "<i><b>'. $keyword . '</b></i>" found '; if(is_array($results)) { $output .= count($results) . ' search result(s):'; $output .= '<ol><li>' . implode('</li><li>', $results) . '</li></ol>'; } else { $output .= "no search results</p>\n\n"; } } echo $output; } if(isset($_POST['submit'])) { if(!empty($_POST['keyword']) && strlen($_POST['keyword']) > 3) { $_POST['keyword'] = str_replace(array("\r\n", "\r", "\n"), "\n", $_POST['keyword']); $keyword_search = explode("\n", $_POST['keyword']); if(is_array($keyword_search)) { foreach($keyword_search as $keyword) { if(strlen($keyword) > 3) $keyword_results[$keyword] = keywordSearch($keyword); } } else { $keyword_results[$keyword_search] = keywordSearch($keyword_search); } displaySearchResults(); } else { echo 'Invalid search term'; } } ?> <form action="<?php echo $_SERVER['PHP_SELF']; ?>" method="post"> Keywords:<br /><textarea name="keyword" cols="40" rows="4"><?php echo isset($_POST['keyword']) ? $_POST['keyword'] : null; ?></textarea> <p><input type="submit" name="submit" value="Search" /></p> </form>
-
PHP Scripts not interpreted in Apache 2.2.2
wildteen88 replied to nicholasis23's topic in PHP Installation and Configuration
PHP maybe crashing when Apache starts up. This maybe due to a PHP configuration issue. You should have a php.ini file in the root of your PHP folder (C:/php). Rename this file to php.ini.old Restart Apache. Does your PHP scripts now work? If they do rename php.ini.old back to php.ini and open it for editing, search for a setting called display_startup_errors and change the default value Off to On (should be around line 377). Save the php.ini and restart Apache. PHP should display error prompts when Apache starts up. -
Function runs fine for me.
-
Because your are setting $this to false if the query fails it is breaking your code, change it to: function User ($id) { global $db; $this->id = intval($id); $this->objects = array(); $res = $db->GetRow("SELECT username, email FROM users WHERE id=".$this->id); if($res) { $this->name = $res[0]; $this->email = $res[1]; } }